FFmpeg
|
#include <stdint.h>
#include "config.h"
#include "libavutil/common.h"
#include "libavutil/libm.h"
#include "libavutil/log.h"
#include "libavutil/mem.h"
#include "libavutil/samplefmt.h"
#include "audio_convert.h"
#include "audio_data.h"
#include "dither.h"
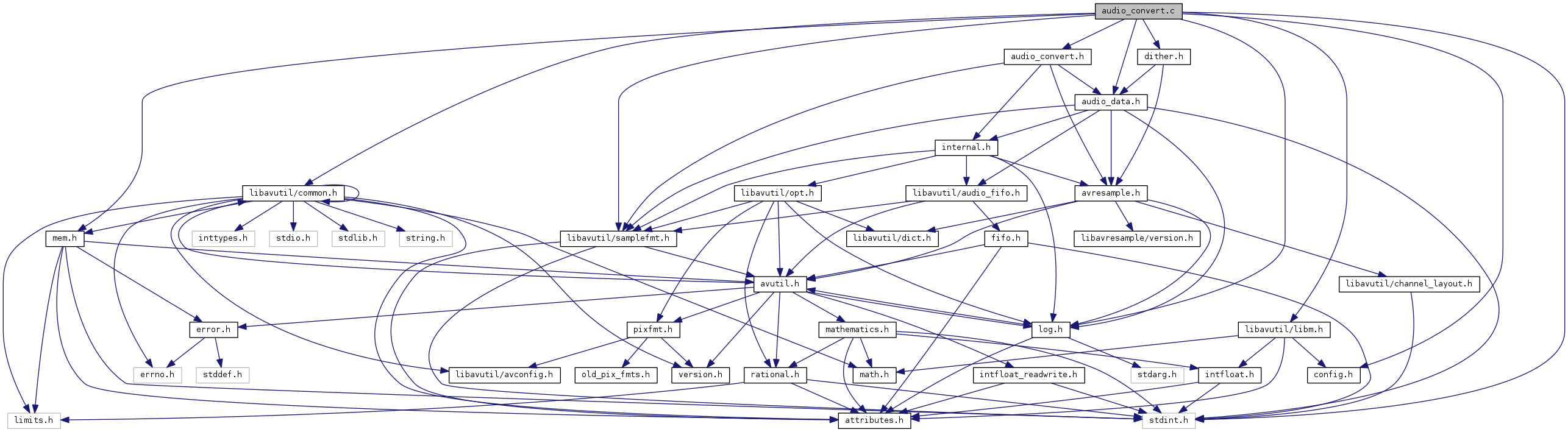
Go to the source code of this file.
Data Structures | |
struct | AudioConvert |
Macros | |
#define | CONV_FUNC_NAME(dst_fmt, src_fmt) conv_ ## src_fmt ## _to_ ## dst_fmt |
#define | CONV_LOOP(otype, expr) |
#define | CONV_FUNC_FLAT(ofmt, otype, ifmt, itype, expr) |
#define | CONV_FUNC_INTERLEAVE(ofmt, otype, ifmt, itype, expr) |
#define | CONV_FUNC_DEINTERLEAVE(ofmt, otype, ifmt, itype, expr) |
#define | CONV_FUNC_GROUP(ofmt, otype, ifmt, itype, expr) |
#define | SET_CONV_FUNC_GROUP(ofmt, ifmt) |
Typedefs | |
typedef void( | conv_func_flat) (uint8_t *out, const uint8_t *in, int len) |
typedef void( | conv_func_interleave) (uint8_t *out, uint8_t *const *in, int len, int channels) |
typedef void( | conv_func_deinterleave) (uint8_t **out, const uint8_t *in, int len, int channels) |
Enumerations | |
enum | ConvFuncType { CONV_FUNC_TYPE_FLAT, CONV_FUNC_TYPE_INTERLEAVE, CONV_FUNC_TYPE_DEINTERLEAVE } |
Functions | |
void | ff_audio_convert_set_func (AudioConvert *ac, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int ptr_align, int samples_align, const char *descr, void *conv) |
Set conversion function if the parameters match. More... | |
CONV_FUNC_GROUP (AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)<< 8) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32 | |
if (ac->has_optimized_func) | |
av_dlog (ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr) | |
Variables | |
int32_t | |
AV_SAMPLE_FMT_U8 | |
uint8_t | |
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> | dc |
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> | out |
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> | in |
else | |
return | |
Macro Definition Documentation
#define CONV_FUNC_DEINTERLEAVE | ( | ofmt, | |
otype, | |||
ifmt, | |||
itype, | |||
expr | |||
) |
Definition at line 171 of file audio_convert.c.
#define CONV_FUNC_FLAT | ( | ofmt, | |
otype, | |||
ifmt, | |||
itype, | |||
expr | |||
) |
Definition at line 143 of file audio_convert.c.
#define CONV_FUNC_GROUP | ( | ofmt, | |
otype, | |||
ifmt, | |||
itype, | |||
expr | |||
) |
Definition at line 187 of file audio_convert.c.
#define CONV_FUNC_INTERLEAVE | ( | ofmt, | |
otype, | |||
ifmt, | |||
itype, | |||
expr | |||
) |
Definition at line 155 of file audio_convert.c.
#define CONV_FUNC_NAME | ( | dst_fmt, | |
src_fmt | |||
) | conv_ ## src_fmt ## _to_ ## dst_fmt |
Definition at line 134 of file audio_convert.c.
#define CONV_LOOP | ( | otype, | |
expr | |||
) |
Definition at line 136 of file audio_convert.c.
#define SET_CONV_FUNC_GROUP | ( | ofmt, | |
ifmt | |||
) |
Typedef Documentation
Definition at line 45 of file audio_convert.c.
Definition at line 40 of file audio_convert.c.
Definition at line 42 of file audio_convert.c.
Enumeration Type Documentation
enum ConvFuncType |
Enumerator | |
---|---|
CONV_FUNC_TYPE_FLAT | |
CONV_FUNC_TYPE_INTERLEAVE | |
CONV_FUNC_TYPE_DEINTERLEAVE |
Definition at line 34 of file audio_convert.c.
Function Documentation
av_dlog | ( | ac-> | avr, |
"%d samples - audio_convert: %s to %s (%s)\n" | , | ||
len | , | ||
av_get_sample_fmt_name(ac->in_fmt) | , | ||
av_get_sample_fmt_name(ac->out_fmt) | , | ||
use_generic?ac->func_descr_generic:ac-> | func_descr | ||
) |
Referenced by amr_decode_fix_avctx(), asf_read_frame_header(), asf_read_header(), asf_read_metadata(), av_interleaved_write_frame(), av_new_program(), avi_load_index(), avi_read_header(), avi_read_idx1(), avi_read_packet(), avi_read_seek(), avi_sync(), avi_write_packet(), avpriv_mpegaudio_decode_header(), avresample_convert(), avresample_open(), build_table(), codec2subblock(), compute_bit_allocation(), compute_pkt_fields(), compute_pkt_fields2(), compute_scale_factors(), compute_target_delay(), cook_decode_frame(), create_map(), dca_subframe_header(), decode(), decode_audio_specific_config(), decode_band(), decode_codestream(), decode_coeffs(), decode_dvd_subtitles(), decode_format80(), decode_frame(), decode_frame_header(), decode_frame_headers(), decode_init(), decode_init_static(), decode_line(), decode_packet(), decode_pic_hdr(), decode_rle(), decode_slice(), decode_subframe(), decode_tilehdr(), dnxhd_decode_frame(), dnxhd_decode_header(), do_video_out(), dprint_options(), dprint_specific_config(), dv1394_read_packet(), dv_decode_ac(), dv_decode_video_segment(), dvbsub_decode(), dvbsub_parse(), dvbsub_parse_clut_segment(), dvbsub_parse_page_segment(), dvbsub_parse_pixel_data_block(), dvbsub_parse_region_segment(), dvdsub_decode(), encode_bitstream(), encode_line(), encode_picture(), encode_thread(), estimate_timings(), evolve(), fbdev_read_packet(), ff_asf_get_packet(), ff_asf_parse_packet(), ff_audio_mix(), ff_audio_resample(), ff_combine_frame(), ff_estimate_b_frame_motion(), ff_gen_search(), ff_h263_decode_picture_header(), ff_h264_decode_mb_cabac(), ff_init_vlc_sparse(), ff_jpegls_decode_lse(), ff_jpegls_reset_coding_parameters(), ff_mjpeg_decode_sof(), ff_mov_read_chan(), ff_mov_read_stsd_entries(), ff_mp4_read_dec_config_descr(), ff_mp4_read_descr(), ff_MPV_frame_start(), ff_msmpeg4_decode_block(), ff_msmpeg4_decode_picture_header(), ff_msmpeg4_encode_picture_header(), ff_parse_mpeg2_descriptor(), ff_rate_estimate_qscale(), ff_rm_read_mdpr_codecdata(), ff_rtp_check_and_send_back_rr(), ff_rtp_send_data(), ff_rtsp_open_transport_ctx(), ff_rtsp_tcp_read_packet(), ff_seek_frame_binary(), ff_vaapi_common_end_frame(), ff_vbv_update(), ff_vc1_parse_frame_header(), ff_wma_init(), ff_wmv2_decode_mb(), ffm_read_packet(), ffm_seek(), ffm_seek1(), filter_frame(), find_marker(), flashsv_decode_frame(), flashsv_encode_frame(), flic_decode_frame_15_16BPP(), flush_packet(), flv_read_packet(), get_dts(), get_http_header_data(), get_packet_size(), get_sockaddr(), get_tcp_server_response(), get_vlc_symbol(), gif_read_extension(), gif_read_header1(), gif_read_image(), grab_read_header(), h261_decode_frame(), h263p_decode_umotion(), handle_buffered_output(), handle_packet_stream_changing_type(), handle_packets(), http_read(), http_read_header(), huffman_decode(), if(), imc_get_coeffs(), init_pass2(), ipvideo_decode_block_opcode_0x2(), ipvideo_decode_block_opcode_0x3(), ipvideo_decode_block_opcode_0x4(), ipvideo_decode_block_opcode_0x5(), ipvideo_decode_block_opcode_0x6_16(), ipvideo_decode_opcodes(), ivi_decode_blocks(), lavfi_read_packet(), load_input_picture(), load_ipmovie_packet(), mjpeg_decode_scan(), mms_open(), mms_read(), mms_safe_send_recv(), mmsh_open_internal(), modify_qscale(), mov_build_index(), mov_find_next_sample(), mov_read_ctts(), mov_read_default(), mov_read_dref(), mov_read_elst(), mov_read_enda(), mov_read_hdlr(), mov_read_header(), mov_read_moof(), mov_read_mvhd(), mov_read_packet(), mov_read_stsc(), mov_read_stss(), mov_read_stsz(), mov_read_stts(), mov_read_tfhd(), mov_read_trun(), mov_read_udta_string(), mov_seek_stream(), movie_push_frame(), mp3lame_encode_frame(), mp_decode_layer2(), mp_decode_layer3(), MPA_encode_init(), mpeg1_decode_block_intra(), mpeg2_decode_block_intra(), mpeg_decode_frame(), mpeg_decode_mb(), mpeg_decode_picture_coding_extension(), mpeg_decode_postinit(), mpeg_decode_quant_matrix_extension(), mpeg_decode_sequence_extension(), mpeg_decode_slice(), mpeg_mux_write_packet(), mpegps_read_dts(), mpegps_read_packet(), mpegts_open_section_filter(), mpegts_probe(), mpegts_push_data(), mpegts_read_header(), mpegts_write_packet_internal(), mpegvideo_parse(), msmpeg4v2_decode_motion(), msmpeg4v34_decode_mb(), mxf_interleave_get_packet(), mxf_parse_handle_essence(), mxf_parse_structural_metadata(), mxf_read_header(), mxf_read_index_table_segment(), mxf_read_local_tags(), mxf_read_packet_old(), mxf_read_partition_pack(), mxf_read_pixel_layout(), mxf_seek_to_previous_partition(), nsv_parse_NSVf_header(), nsv_parse_NSVs_header(), nsv_probe(), nsv_read_chunk(), nsv_read_header(), nsv_read_packet(), nsv_resync(), ogg_packet(), ogg_read_header(), old_codec37(), old_codec47(), output_packet(), parse_palette_segment(), parse_presentation_segment(), pat_cb(), pcm_bluray_decode_frame(), pcm_bluray_parse_header(), pmt_cb(), process_frame_obj(), process_ipmovie_chunk(), process_line(), put_vlc_symbol(), r3d_read_header(), r3d_read_rdvo(), r3d_read_red1(), r3d_read_reda(), r3d_read_redv(), r3d_read_reos(), r3d_seek(), read_atom(), read_braindead_odml_indx(), read_data_packet(), read_frame_header(), read_header(), read_huffman_tree(), realloc_buffer(), rm_read_dts(), rm_read_header(), rotate_bufs(), rtcp_send_sr(), rtmp_parse_result(), rtp_write_packet(), rtsp_read_request(), rtsp_read_setup(), rtsp_send_reply(), rv10_decode_frame(), rv10_decode_packet(), rv10_decode_picture_header(), sdt_cb(), seg_write_packet(), slice_decode_thread(), svq1_decode_block_intra(), svq1_decode_block_non_intra(), svq1_decode_delta_block(), svq1_decode_frame(), svq1_decode_frame_header(), swf_read_packet(), synchronize_audio(), try_filter_frame(), vaapi_h264_decode_slice(), vaapi_h264_end_frame(), vaapi_h264_start_frame(), vaapi_mpeg2_decode_slice(), vaapi_mpeg2_start_frame(), vaapi_mpeg4_decode_slice(), vaapi_mpeg4_start_frame(), vaapi_vc1_decode_slice(), vaapi_vc1_start_frame(), vc1_decode_frame(), vorbis_decode_frame(), vorbis_floor0_decode(), vorbis_floor1_decode(), vorbis_parse_audio_packet(), vorbis_parse_id_hdr(), vorbis_parse_setup_hdr_codebooks(), vorbis_parse_setup_hdr_floors(), vorbis_parse_setup_hdr_mappings(), vorbis_parse_setup_hdr_modes(), vorbis_parse_setup_hdr_residues(), vorbis_parse_setup_hdr_tdtransforms(), vorbis_residue_decode_internal(), and wma_decode_superframe().
CONV_FUNC_GROUP | ( | AV_SAMPLE_FMT_S16 | , |
int16_t | , | ||
AV_SAMPLE_FMT_U8 | , | ||
uint8_t | , | ||
(*(const uint8_t *) pi-0x80)<< | 8 | ||
) |
void ff_audio_convert_set_func | ( | AudioConvert * | ac, |
enum AVSampleFormat | out_fmt, | ||
enum AVSampleFormat | in_fmt, | ||
int | channels, | ||
int | ptr_align, | ||
int | samples_align, | ||
const char * | descr, | ||
void * | conv | ||
) |
Set conversion function if the parameters match.
This compares the parameters of the conversion function to the parameters in the AudioConvert context. If the parameters do not match, no changes are made to the active functions. If the parameters do match and the alignment is not constrained, the function is set as the generic conversion function. If the parameters match and the alignment is constrained, the function is set as the optimized conversion function.
- Parameters
-
ac AudioConvert context out_fmt output sample format in_fmt input sample format channels number of channels, or 0 for any number of channels ptr_align buffer pointer alignment, in bytes samples_align buffer size alignment, in samples descr function type description (e.g. "C" or "SSE") conv conversion function pointer
Definition at line 70 of file audio_convert.c.
Referenced by ff_audio_convert_init_arm(), and ff_audio_convert_init_x86().
if | ( | ac-> | has_optimized_func | ) |
Definition at line 329 of file audio_convert.c.
Variable Documentation
AV_SAMPLE_FMT_U8 |
Definition at line 194 of file audio_convert.c.
Referenced by av_audio_convert(), av_get_pcm_codec(), av_samples_set_silence(), dpcm_decode_init(), get(), get_format_from_sample_fmt(), lavfi_read_header(), PUT_FUNC(), query_formats(), sample_fmt_bits_per_sample(), set(), smka_decode_frame(), smka_decode_init(), swri_dither_init(), tta_decode_init(), vmdaudio_decode_init(), volume_init(), waveform_codec_id(), ws_snd_decode_init(), yae_downmix(), and yae_overlap_add().
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t, (*(const uint8_t *)pi - 0x80) * (1.0f / (1 << 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t, (*(const uint8_t *)pi - 0x80) * (1.0 / (1 << 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t, (*(const int16_t *)pi >> 8) + 0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t, *(const int16_t *)pi * (1.0f / (1 << 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t, *(const int16_t *)pi * (1.0 / (1 << 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t, (*(const int32_t *)pi >> 24) + 0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t, *(const int32_t *)pi * (1.0f / (1U << 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t, *(const int32_t *)pi * (1.0 / (1U << 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8( lrintf(*(const float *)pi * (1 << 7)) + 0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16( lrintf(*(const float *)pi * (1 << 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *)pi * (1U << 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8( lrint(*(const double *)pi * (1 << 7)) + 0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16( lrint(*(const double *)pi * (1 << 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *)pi * (1U << 31)))) #define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac) { } void ff_audio_convert_free(AudioConvert **ac) { if (!*ac) return; ff_dither_free(&(*ac)->dc); av_freep(ac); } AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map) { AudioConvert *ac; int in_planar, out_planar; ac = av_mallocz(sizeof(*ac)); if (!ac) return NULL; ac->avr = avr; ac->out_fmt = out_fmt; ac->in_fmt = in_fmt; ac->channels = channels; ac->apply_map = apply_map; if (avr->dither_method != AV_RESAMPLE_DITHER_NONE && av_get_packed_sample_fmt(out_fmt) == AV_SAMPLE_FMT_S16 && av_get_bytes_per_sample(in_fmt) > 2) { ac->dc = ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map); if (!ac->dc) { av_free(ac); return NULL; } return ac; } in_planar = av_sample_fmt_is_planar(in_fmt); out_planar = av_sample_fmt_is_planar(out_fmt); if (in_planar == out_planar) { ac->func_type = CONV_FUNC_TYPE_FLAT; ac->planes = in_planar ? ac->channels : 1; } else if (in_planar) ac->func_type = CONV_FUNC_TYPE_INTERLEAVE; else ac->func_type = CONV_FUNC_TYPE_DEINTERLEAVE; set_generic_function(ac); if (ARCH_ARM) ff_audio_convert_init_arm(ac); if (ARCH_X86) ff_audio_convert_init_x86(ac); return ac; } int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in) { int use_generic = 1; int len = in->nb_samples; int p; if (ac->dc) { av_dlog(ac->avr, "%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt)); return ff_convert_dither(ac-> dc |
Definition at line 194 of file audio_convert.c.
else |
Definition at line 380 of file audio_convert.c.
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t, (*(const uint8_t *)pi - 0x80) * (1.0f / (1 << 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t, (*(const uint8_t *)pi - 0x80) * (1.0 / (1 << 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t, (*(const int16_t *)pi >> 8) + 0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t, *(const int16_t *)pi * (1.0f / (1 << 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t, *(const int16_t *)pi * (1.0 / (1 << 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t, (*(const int32_t *)pi >> 24) + 0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t, *(const int32_t *)pi * (1.0f / (1U << 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t, *(const int32_t *)pi * (1.0 / (1U << 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8( lrintf(*(const float *)pi * (1 << 7)) + 0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16( lrintf(*(const float *)pi * (1 << 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *)pi * (1U << 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8( lrint(*(const double *)pi * (1 << 7)) + 0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16( lrint(*(const double *)pi * (1 << 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *)pi * (1U << 31)))) #define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac) { } void ff_audio_convert_free(AudioConvert **ac) { if (!*ac) return; ff_dither_free(&(*ac)->dc); av_freep(ac); } AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map) { AudioConvert *ac; int in_planar, out_planar; ac = av_mallocz(sizeof(*ac)); if (!ac) return NULL; ac->avr = avr; ac->out_fmt = out_fmt; ac->in_fmt = in_fmt; ac->channels = channels; ac->apply_map = apply_map; if (avr->dither_method != AV_RESAMPLE_DITHER_NONE && av_get_packed_sample_fmt(out_fmt) == AV_SAMPLE_FMT_S16 && av_get_bytes_per_sample(in_fmt) > 2) { ac->dc = ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map); if (!ac->dc) { av_free(ac); return NULL; } return ac; } in_planar = av_sample_fmt_is_planar(in_fmt); out_planar = av_sample_fmt_is_planar(out_fmt); if (in_planar == out_planar) { ac->func_type = CONV_FUNC_TYPE_FLAT; ac->planes = in_planar ? ac->channels : 1; } else if (in_planar) ac->func_type = CONV_FUNC_TYPE_INTERLEAVE; else ac->func_type = CONV_FUNC_TYPE_DEINTERLEAVE; set_generic_function(ac); if (ARCH_ARM) ff_audio_convert_init_arm(ac); if (ARCH_X86) ff_audio_convert_init_x86(ac); return ac; } int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in) { int use_generic = 1; int len = in->nb_samples; int p; if (ac->dc) { av_dlog(ac->avr, "%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt)); return ff_convert_dither(ac-> in |
Definition at line 194 of file audio_convert.c.
int32_t |
Definition at line 194 of file audio_convert.c.
Referenced by aacPlus_encode_frame(), add_bell(), adpcm_decode_frame(), alac_entropy_coder(), alac_linear_predictor(), alac_stereo_decorrelation(), allocate_buffers(), allocStuff(), ape_decode_frame(), ape_read_header(), ape_unpack_stereo(), apply_channel_coupling(), apply_unsharp(), av_audio_convert(), av_clipl_int32_c(), av_crc_init(), bgr24ToUV_c(), bgr24ToUV_half_c(), bgr24ToY_c(), bink_decode_plane(), binkb_decode_plane(), biweight_h264_W_altivec(), build_filter(), calc_predictor_params(), calculate_sign_huff(), channel_decorrelation(), chrRangeFromJpeg16_c(), chrRangeToJpeg16_c(), compute_imdct(), config(), copy_tag(), create_map(), dctcoef_get(), dctcoef_set(), DECLARE_ALIGNED(), decode(), decode_block(), decode_cabac_residual_internal(), decode_channel(), decode_const_block_data(), decode_element(), decode_frame(), decode_residual(), decode_subframe(), decode_thread(), decode_tile(), decode_var_block_data(), decorrelate(), decorrelate_stereo(), dxa_read_header(), encode_init(), encode_residual_ch(), entropy_decode_mono_3860(), entropy_decode_mono_3900(), entropy_decode_mono_3990(), entropy_decode_stereo_3860(), entropy_decode_stereo_3900(), entropy_decode_stereo_3930(), entropy_decode_stereo_3990(), estimate_stereo_mode(), ff_ac3_apply_rematrixing(), ff_eac3_apply_spectral_extension(), ff_ivi_inverse_haar_8x8(), ff_ivi_inverse_slant_4x4(), ff_ivi_inverse_slant_8x8(), ff_ivi_recompose53(), ff_ivi_recompose_haar(), ff_j_rev_dct(), ff_j_rev_dct4(), ff_mlp_filter_channel(), ff_mpadsp_apply_window_mips_fixed(), ff_print_debug_info2(), ff_rgb24toyv12_c(), ff_rtp_check_and_send_back_rr(), filter(), filter_3800(), filter_channel(), filter_fast_3320(), filter_frame(), finalize_packet(), flv_read_packet(), gbr24pToUV_half_c(), generate_wave_table(), get(), get_xbits(), h264_mp4toannexb_filter(), hScale16To19_c(), hScale8To19_c(), init_offset(), init_sample_buffers(), initFilter(), ivi_decode_blocks(), lfe_downsample(), long_filter_ehigh_3830(), long_filter_high_3800(), lpc_prediction(), lumRangeFromJpeg16_c(), lumRangeToJpeg16_c(), mov_read_elst(), mov_write_tapt_tag(), mp3_write_xing(), mp3lame_encode_frame(), mpeg1_decode_block_inter(), mpeg1_fast_decode_block_inter(), mpeg2_decode_block_non_intra(), mpeg2_fast_decode_block_non_intra(), msmpeg4_decode_dc(), multiple_resample(), nist_read_header(), output_data(), pcm_bluray_decode_frame(), pcm_decode_frame(), pcm_encode_frame(), pink_fill(), planar_rgb16_to_uv(), planar_rgb16_to_y(), planar_rgb_to_uv(), planar_rgb_to_y(), predictor_decode_mono_3800(), predictor_decode_mono_3930(), predictor_decode_mono_3950(), predictor_decode_stereo_3800(), predictor_decode_stereo_3930(), predictor_decode_stereo_3950(), predictor_update_3930(), predictor_update_filter(), process_chrominance(), put_subframe(), qmf_decompose(), qmf_init(), quantize_lpc_coefs(), ra144_encode_frame(), read_dct_coeffs(), read_filter_params(), read_mfra(), read_var_block_data(), rematrix_channels(), remove_wasted_bits(), request_frame(), revert_channel_correlation(), rgb24ToUV_c(), rgb24ToUV_half_c(), rgb24ToY_c(), rgb48ToUV_c_template(), rgb48ToUV_half_c_template(), rgb64funcs(), rgb64ToUV_c_template(), rgb64ToUV_half_c_template(), rgb64ToY_c_template(), rice_count_exact(), rnd_table_init(), rpl_read_header(), rtcp_update_jitter(), scalarproduct(), scalarproduct_and_madd_int16_altivec(), scalarproduct_int16_altivec(), scale_samples_s32(), set(), shorten_decode_frame(), smacker_read_header(), srt_read_header(), ssd_int8_vs_int16_altivec(), Stagefright_init(), svq1_encode_init(), svq3_decode_block(), svq3_mc_dir(), swri_get_dither(), sws_init_context(), sws_isSupportedOutput(), swScale(), tak_decode_frame(), tta_decode_frame(), tta_decode_init(), ttafilter_process(), update_md5_sum(), uyvytoyv12(), wavesynth_decode(), wavesynth_parse_extradata(), wavesynth_synth_sample(), wavpack_decode_block(), weight_h264_W_altivec(), write_element(), write_hint_packets(), write_subframes(), wv_unpack_mono(), wv_unpack_stereo(), x8_decode_intra_mb(), x8_loop_filter(), yuv2rgb48_1_c_template(), yuv2rgb48_2_c_template(), yuv2yuyv422_1(), yuvPlanartouyvy_c(), and yuvPlanartoyuy2_c().
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t, (*(const uint8_t *)pi - 0x80) * (1.0f / (1 << 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t, (*(const uint8_t *)pi - 0x80) * (1.0 / (1 << 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t, (*(const int16_t *)pi >> 8) + 0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t, *(const int16_t *)pi * (1.0f / (1 << 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t, *(const int16_t *)pi * (1.0 / (1 << 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t, (*(const int32_t *)pi >> 24) + 0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t, *(const int32_t *)pi * (1.0f / (1U << 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t, *(const int32_t *)pi * (1.0 / (1U << 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8( lrintf(*(const float *)pi * (1 << 7)) + 0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16( lrintf(*(const float *)pi * (1 << 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *)pi * (1U << 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8( lrint(*(const double *)pi * (1 << 7)) + 0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16( lrint(*(const double *)pi * (1 << 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *)pi * (1U << 31)))) #define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac) { } void ff_audio_convert_free(AudioConvert **ac) { if (!*ac) return; ff_dither_free(&(*ac)->dc); av_freep(ac); } AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map) { AudioConvert *ac; int in_planar, out_planar; ac = av_mallocz(sizeof(*ac)); if (!ac) return NULL; ac->avr = avr; ac->out_fmt = out_fmt; ac->in_fmt = in_fmt; ac->channels = channels; ac->apply_map = apply_map; if (avr->dither_method != AV_RESAMPLE_DITHER_NONE && av_get_packed_sample_fmt(out_fmt) == AV_SAMPLE_FMT_S16 && av_get_bytes_per_sample(in_fmt) > 2) { ac->dc = ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map); if (!ac->dc) { av_free(ac); return NULL; } return ac; } in_planar = av_sample_fmt_is_planar(in_fmt); out_planar = av_sample_fmt_is_planar(out_fmt); if (in_planar == out_planar) { ac->func_type = CONV_FUNC_TYPE_FLAT; ac->planes = in_planar ? ac->channels : 1; } else if (in_planar) ac->func_type = CONV_FUNC_TYPE_INTERLEAVE; else ac->func_type = CONV_FUNC_TYPE_DEINTERLEAVE; set_generic_function(ac); if (ARCH_ARM) ff_audio_convert_init_arm(ac); if (ARCH_X86) ff_audio_convert_init_x86(ac); return ac; } int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in) { int use_generic = 1; int len = in->nb_samples; int p; if (ac->dc) { av_dlog(ac->avr, "%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt)); return ff_convert_dither(ac-> out |
Definition at line 194 of file audio_convert.c.
Referenced by altivec_uyvy_rgb32(), anti_sparseness(), audio_decode_frame(), av_base64_decode(), av_base64_encode(), av_get_token(), av_hmac_calc(), av_i2int(), av_int2i(), av_mlfg_get(), av_mul_i(), av_shr_i(), avcodec_decode_audio3(), avpriv_evaluate_lls(), avs_decode_frame(), cdg_scroll(), cdxl_decode_ham6(), cdxl_decode_ham8(), clear_index_entries(), copy_moof(), dc1394_read_common(), decode_frame(), decode_ics(), decode_postinit(), decorrelation(), doTest(), dpcm_decode_frame(), dump_attachment(), f_func(), ff_avc_find_startcode(), ff_avc_write_annexb_extradata(), ff_dct32_mips_float(), ff_filter_frame_framed(), ff_ref_fdct(), ff_ref_idct(), ff_rle_encode(), ff_srtp_encrypt(), ff_yuv2packedX_altivec(), filter(), filter_frame(), find_header_idx(), flac_decorrelate_indep_c(), flac_decorrelate_ls_c(), flac_decorrelate_ms_c(), flac_decorrelate_rs_c(), flv_data_packet(), g723_1_decode_frame(), geq_filter_frame(), get_delayed_pic(), grab_read_header(), gxf_resync_media(), h264_mp4toannexb_filter(), hybrid2_re(), hybrid4_8_12_cx(), hybrid6_cx(), imdct36_mips_fixed(), imdct_and_window(), imdct_and_windowing(), ir2_decode_plane(), ir2_decode_plane_inter(), iso88591_to_utf8(), log16(), matroska_decode_buffer(), md5_close(), mjpeg2jpeg_filter(), mjpeg_decode_app(), mss4_decode_dct_block(), mxf_interleave_get_packet(), open_output_file(), output_client_manifest(), output_server_manifest(), paf_vid_decode(), plot_spectrum_column(), pmt_cb(), predictor_calc_error(), print_formats(), ps_hybrid_analysis_c(), ps_hybrid_analysis_ileave_c(), put_main_header(), pxr24_uncompress(), qdm2_calculate_fft(), qdm2_decode_frame(), r3d_read_header(), ra288_decode_frame(), read_tree(), resample(), return_audio_frame(), rl2_rle_decode(), roq_dpcm_encode_frame(), sbr_hf_assemble(), sbr_make_f_tablelim(), scalarproduct(), scale_slice(), setup_array(), swr_convert(), swr_convert_internal(), swr_set_matrix(), targa_encode_frame(), targa_encode_normal(), targa_encode_rle(), twin_decode_frame(), WINDOW_FUNC(), write_fragment(), write_manifest(), x11grab_read_header(), zmbv_decode_xor_16(), zmbv_decode_xor_32(), and zmbv_decode_xor_8().
return |
Definition at line 413 of file audio_convert.c.
uint8_t |
Definition at line 194 of file audio_convert.c.
Referenced by __attribute__(), a64_compress_colram(), a64_write_header(), a64multi_encode_frame(), a64multi_init_encoder(), aac_adtstoasc_filter(), aac_decode_frame(), aac_decode_frame_int(), aac_decode_init(), aac_encode_init(), aac_sync(), aasc_decode_frame(), aasc_decode_init(), ac3_decode_frame(), ac3_decode_transform_coeffs_ch(), ac3_eac3_probe(), ac3_exponent_min_c(), ac3_sync(), add_entry(), add_frame_default(), add_hfyu_median_prediction_c(), add_lag_median_prediction(), add_metadata(), add_metadata_from_side_data(), add_yblock(), adpcm_compress_trellis(), adpcm_decode_frame(), adpcm_encode_frame(), adpcm_encode_init(), adts_aac_probe(), adts_write_packet(), adx_decode_frame(), adx_encode_frame(), alac_encode_init(), alloc_and_copy(), allocate_buffers(), amr_decode_fix_avctx(), amr_handle_packet(), amr_read_header(), amr_read_packet(), amrnb_decode_frame(), amrwb_decode_frame(), ape_decode_frame(), ape_tag_read_field(), apply_delogo(), apply_loop_filter(), apply_param_change(), apply_unsharp(), asf_read_picture(), asf_write_header1(), asfrtp_free_context(), asfrtp_parse_packet(), ass_get_duration(), ass_split_section(), at1_unpack_dequant(), atrac1_decode_frame(), atrac3_decode_frame(), atrac3_decode_init(), audio_decode_example(), audio_decode_frame(), audio_encode_example(), audio_write_packet(), aura_decode_frame(), av_aes_init(), av_audio_convert(), av_base64_decode(), av_base64_encode(), av_bitstream_filter_filter(), av_blowfish_crypt(), av_buffer_alloc(), av_buffer_realloc(), av_buffersrc_add_frame_internal(), av_crc(), av_des_mac(), av_dump_format(), av_fast_padded_malloc(), av_fast_padded_mallocz(), av_fifo_generic_read(), av_fifo_generic_write(), av_fifo_peek2(), av_find_info_tag(), av_frame_get_plane_buffer(), av_get_string(), av_hmac_calc(), av_hmac_final(), av_hmac_init(), av_i2int(), av_image_alloc(), av_image_copy_to_buffer(), av_image_fill_arrays(), av_image_get_buffer_size(), av_int_list_length_for_size(), av_lfg_init(), av_log(), av_lzo1x_decode(), av_md5_final(), av_md5_sum(), av_memcpy_backptr(), av_opencl_buffer_read_image(), av_opencl_buffer_write(), av_opencl_buffer_write_image(), av_opt_free(), av_opt_get(), av_opt_get_image_size(), av_opt_ptr(), av_opt_set(), av_opt_set_bin(), av_opt_set_image_size(), av_packet_merge_side_data(), av_packet_split_side_data(), av_parser_change(), av_parser_parse2(), av_picture_copy(), av_picture_pad(), av_probe_input_buffer(), av_probe_input_format3(), AV_RB16(), AV_RB24(), AV_RB32(), av_rc4_crypt(), av_rc4_init(), av_read_frame(), av_read_image_line(), AV_RL16(), AV_RL24(), AV_RL32(), av_samples_alloc(), av_samples_fill_arrays(), av_sha_final(), av_size_mult(), av_strcasecmp(), av_strncasecmp(), av_tempfile(), av_tree_enumerate(), av_write_image_line(), av_xtea_crypt(), avcodec_decode_audio3(), avcodec_decode_audio4(), avcodec_encode_audio(), avcodec_fill_audio_frame(), avfilter_transform(), avg_no_rnd_vc1_chroma_mc8_c(), avi_read_header(), avi_read_packet(), avio_put_str16le(), avio_wb16(), avio_wb24(), avio_wb32(), avio_wl16(), avio_wl32(), avpicture_layout(), avpriv_color_frame(), avpriv_dv_produce_packet(), avpriv_vorbis_parse_extradata(), avpriv_vorbis_parse_reset(), avs_decode_frame(), avs_probe(), avs_read_packet(), avui_decode_frame(), avui_encode_frame(), backup_mb_border(), bethsoftvid_decode_frame(), bfi_decode_frame(), bfin_vp3_idct_add(), bfin_vp3_idct_put(), bidir_refine(), bink_decode_plane(), binkb_calc_quant(), binkb_decode_plane(), binkb_read_bundle(), bit8x8_c(), bitplane_decoding(), bktr_init(), blend_frame(), blend_image(), blend_subrect(), block_cmp(), block_mc(), blur(), blur_image(), blur_pixel(), blur_power(), bmp_decode_frame(), bmp_encode_frame(), bmv_aud_decode_frame(), buffer_needs_copy(), build_diff_map(), build_elision_headers(), build_huff(), build_huff_tree(), build_vlc(), calc_combed_score(), calc_diffs(), cavs_decode_frame(), cavs_idct8_add_c(), cbp_test(), cbr_bit_allocation(), cdata_probe(), cdg_border_preset(), cdg_decode_frame(), cdg_load_palette(), cdg_scroll(), cdg_tile_block(), cdxl_decode_frame(), cdxl_decode_ham6(), cdxl_decode_ham8(), celt_header(), channelmap_filter_frame(), check_bidir_mv(), check_format(), check_header_mismatch(), chomp_filter(), choose_pix_fmts(), chroma_4mv_motion(), chroma_4mv_motion_lowres(), cinaudio_decode_frame(), cinepak_decode(), cinepak_decode_codebook(), cinepak_decode_frame(), cinepak_decode_strip(), cinepak_decode_vectors(), cinvideo_decode_frame(), cllc_decode_frame(), cmap_read_palette(), cmp_direct_inline(), cmp_inline(), cmv_decode_frame(), cmv_decode_inter(), commit_bitstream_and_slice_buffer(), compare_fields(), compute_exp_strategy(), concat_read_header(), concatenate_packet(), config(), config_input(), config_out_props(), config_props(), config_video_output(), context_init(), convert_mask_to_strength_mask(), cook_decode_frame(), cook_decode_init(), copy(), copy_backptr(), copy_block(), copy_cell(), copy_frame(), copy_frame_default(), copy_input_samples(), copy_moof(), copy_output(), copy_picture_field(), copy_rectangle(), copy_region_enc(), core_yuv420_rgb(), count_colors(), count_frame_header(), count_pixels(), cpia_decode_frame(), create_iv(), create_vorbis_context(), cyuv_decode_frame(), dc1394_read_common(), dca_decode_frame(), dca_parse_params(), dct_error(), dct_get(), dct_quantize_bfin(), dct_quantize_refine(), dct_quantize_trellis_c(), dct_sad8x8_c(), DECLARE_ALIGNED(), decode(), decode_0(), decode_10bit_pulse(), decode_13(), decode_5(), decode_8(), decode_argb_frame(), decode_audio_block(), decode_audio_s16(), decode_band(), decode_band_hdr(), decode_band_structure(), decode_band_types(), decode_bdlt(), decode_block(), decode_block_coeffs(), decode_block_intra(), decode_bmv_frame(), decode_byterun(), decode_cabac_intra_mb_type(), decode_cabac_luma_residual(), decode_cabac_residual_internal(), decode_cell(), decode_cell_data(), decode_chunks(), decode_codestream(), decode_dc(), decode_dds1(), decode_deep_rle32(), decode_dsw1(), decode_dvd_subtitles(), decode_fill(), decode_flush(), decode_frame(), decode_frame_header(), decode_frame_headers(), decode_ga_specific_config(), decode_header(), decode_hextile(), decode_hybrid(), decode_i2_frame(), decode_i_frame(), decode_init(), decode_init_static(), decode_init_thread_copy(), decode_intra4x4_modes(), decode_lowdelay(), decode_luma_residual(), decode_main_header(), decode_mb_i(), decode_mvc1(), decode_mvc2(), decode_mvs(), decode_nal_units(), decode_p_frame(), decode_packet(), decode_picture_header(), decode_pixel_in_context(), decode_plane(), decode_region(), decode_region_intra(), decode_region_masked(), decode_residual_block(), decode_rgb24_frame(), decode_rle(), decode_scaling_list(), decode_scaling_matrices(), decode_sgirle8(), decode_slice(), decode_slice_header(), decode_slice_thread(), decode_splitmvs(), decode_str(), decode_tag(), decode_tdlt(), decode_text_chunk(), decode_thread(), decode_tile(), decode_tilehdr(), decode_tsw1(), decode_unregistered_user_data(), decode_vbmtree(), decode_wave_header(), decode_wdlt(), decrypt_init(), deInterlaceMedian(), delta_decode(), denoise_depth(), dequant(), dequant_lsps(), dering(), dering_altivec(), derive_key(), dfa_decode_frame(), dirac_combine_frame(), dirac_decode_frame(), dirac_decode_frame_internal(), dirac_unpack_block_motion_data(), dirac_unpack_prediction_parameters(), display_frame(), dnxhd_10bit_dct_quantize(), dnxhd_decode_dct_block(), dnxhd_decode_frame(), dnxhd_decode_header(), dnxhd_decode_macroblock(), dnxhd_encode_init(), dnxhd_encode_picture(), dnxhd_encode_thread(), dnxhd_get_blocks(), dnxhd_init_qmat(), dnxhd_mb_var_thread(), dnxhd_probe(), dnxhd_switch_matrix(), dnxhd_unquantize_c(), dnxhd_write_header(), do_adobe_auth(), do_llnw_auth(), do_streamcopy(), do_swap(), do_video_out(), doTest(), doVertDefFilter_altivec(), doVertLowPass_altivec(), dpcm_decode_frame(), draw_frame(), draw_glyphs(), draw_text(), drawline(), drawtext(), dts_probe(), duplicate(), dv_calc_mb_coordinates(), dv_decode_ac(), dv_decode_video_segment(), dv_encode_video_segment(), dv_extract_audio(), dv_extract_audio_info(), dv_extract_timecode(), dv_extract_video_info(), dv_init_enc_block(), dv_inject_metadata(), dv_read_timecode(), dv_write_pack(), dv_write_packet(), dvb_encode_rle2(), dvb_encode_rle4(), dvb_encode_rle8(), dvbsub_decode(), dvbsub_parse(), dvbsub_parse_clut_segment(), dvbsub_parse_object_segment(), dvbsub_parse_page_segment(), dvbsub_parse_pixel_data_block(), dvbsub_parse_region_segment(), dvbsub_read_8bit_string(), dvd_encode_rle(), dvdsub_decode(), dvvideo_decode_frame(), dxa_read_packet(), ebml_read_ascii(), ebml_read_num(), encode_15_7(), encode_422_bitstream(), encode_all_blocks(), encode_bgr(), encode_bitstream(), encode_block(), encode_dvb_subtitles(), encode_dvd_subtitles(), encode_exponents(), encode_exponents_blk_ch(), encode_frame(), encode_gbrp10(), encode_header(), encode_init(), encode_intervals(), encode_mb_hq(), encode_mb_internal(), encode_nals(), encode_picture(), encode_picture_lossless(), encode_picture_ls(), encode_plane(), encode_q_branch(), encode_rgb48_10bit(), encode_slice(), encode_slice_header(), encode_thread(), encrypt_counter(), escape124_decode_frame(), escape130_decode_frame(), escape_FF(), evolve(), evrc_decode_frame(), exponents_from_scale_factors(), extract_header(), extract_mpeg4_header(), f_func(), fade_plane(), fbdev_read_packet(), ff_ac3_apply_rematrixing(), ff_ac3_group_exponents(), ff_add_param_change(), ff_amf_tag_size(), ff_ape_parse_tag(), ff_asf_parse_packet(), ff_asfcrypt_dec(), ff_audio_data_add_to_fifo(), ff_audio_data_realloc(), ff_audio_mix(), ff_avc_find_startcode(), ff_avc_find_startcode_internal(), ff_avc_parse_nal_units(), ff_avc_write_annexb_extradata(), ff_bgmc_decode(), ff_big_add(), ff_big_div(), ff_big_mul(), ff_blend_mask(), ff_blend_rectangle(), ff_calculate_bounding_box(), ff_cavs_filter(), ff_check_pixfmt_descriptors(), ff_copy_dshow_media_type(), ff_copy_rectangle(), ff_copy_rectangle2(), ff_cos(), ff_dct_quantize_c(), ff_default_get_video_buffer(), ff_draw_color(), ff_draw_rectangle(), ff_draw_supported_pixel_formats(), ff_dvvideo_init(), ff_eac3_apply_spectral_extension(), ff_er_add_slice(), ff_er_frame_end(), ff_er_frame_start(), ff_estimate_motion_b(), ff_estimate_p_frame_motion(), ff_fill_line_with_color(), ff_fill_rectangle(), ff_filter_frame_framed(), ff_flac_write_header(), ff_generate_avci_extradata(), ff_get_audio_buffer(), ff_get_best_fcode(), ff_h261_loop_filter(), ff_h263_decode_frame(), ff_h263_find_resync_marker_reverse(), ff_h263_handle_packet(), ff_h263_loop_filter(), ff_h263_round_chroma(), ff_h264_alloc_tables(), ff_h264_chroma422_dc_dequant_idct(), ff_h264_decode_init(), ff_h264_decode_mb_cabac(), ff_h264_decode_mb_cavlc(), ff_h264_decode_nal(), ff_h264_filter_mb(), ff_h264_luma_dc_dequant_idct(), ff_id3v1_read(), ff_id3v2_parse(), ff_id3v2_read(), ff_id3v2_write_apic(), ff_init_cabac_states(), ff_init_range_decoder(), ff_init_rl(), ff_insert_pad(), ff_isom_write_avcc(), ff_ivi_decode_frame(), ff_j2k_ceildiv(), ff_j2k_getrefctxno(), ff_j2k_init_component(), ff_jpegls_decode_picture(), ff_load_image(), ff_log2_q15(), ff_lzw_decode(), ff_lzw_encode(), ff_mjpeg_decode_dht(), ff_mjpeg_decode_frame(), ff_mjpeg_find_marker(), ff_mlp_calculate_parity(), ff_mlp_checksum8(), ff_mms_asf_header_parser(), ff_mov_add_hinted_packet(), ff_mov_close_hinting(), ff_mov_iso639_to_lang(), ff_mov_read_stsd_entries(), ff_mov_write_packet(), ff_mpeg4_encode_mb(), ff_mpeg4_init_partitions(), ff_mpeg_unref_picture(), ff_MPV_encode_picture(), ff_msmpeg4_encode_block(), ff_msmpeg4_encode_mb(), ff_msmpeg4_pred_dc(), ff_mspel_motion(), ff_mss34_gen_quant_mat(), ff_opencl_transform(), ff_parse_mpeg2_descriptor(), ff_print_debug_info2(), ff_printGUID(), ff_put_v(), ff_rac_terminate(), ff_rdt_parse_packet(), ff_rle_encode(), ff_rm_read_mdpr_codecdata(), ff_rtjpeg_decode_frame_yuv420(), ff_rtmp_calc_digest(), ff_rtmp_packet_dump(), ff_rtmp_packet_read(), ff_rtmp_packet_read_internal(), ff_rtmp_packet_write(), ff_rtmpe_compute_secret_key(), ff_rtp_chain_mux_open(), ff_rtp_check_and_send_back_rr(), ff_rtp_send_aac(), ff_rtp_send_amr(), ff_rtp_send_h263(), ff_rtp_send_h263_rfc2190(), ff_rtp_send_h264(), ff_rtp_send_jpeg(), ff_rtp_send_mpegvideo(), ff_rtp_send_punch_packets(), ff_rtp_send_rtcp_feedback(), ff_rtp_send_xiph(), ff_rtsp_open_transport_ctx(), ff_rtsp_undo_setup(), ff_rv34_decode_frame(), ff_samples_to_time_base(), ff_scale_image(), ff_shrink22(), ff_shrink44(), ff_snow_inner_add_yblock(), ff_snow_pred_block(), ff_spdif_probe(), ff_srtp_decrypt(), ff_srtp_encrypt(), ff_srtp_set_crypto(), ff_svq3_luma_dc_dequant_idct_c(), ff_thread_video_encode_frame(), ff_vaapi_alloc_slice(), ff_vaapi_get_surface_id(), ff_vc1_decode_blocks(), ff_vda_create_decoder(), ff_vdpau_add_data_chunk(), ff_vdpau_get_surface_id(), ff_vf_get_image(), ff_vf_next_control(), ff_vf_next_put_image(), ff_vorbis_comment(), ff_vorbis_len2vlc(), ff_vp56_decode_frame(), ff_wma_init(), ff_wmv2_decode_mb(), ff_wmv2_encode_mb(), ffio_fdopen(), ffio_rewind_with_probe_data(), ffio_set_buf_size(), ffio_wfourcc(), ffm_read_write_index(), ffm_write_packet(), ffm_write_write_index(), ffmpeg_parse_options(), ffv1_init_slice_state(), fill_buffer(), fill_decode_caches(), fill_decode_neighbors(), fill_filter_caches(), fill_noise_buffer(), fill_picture(), fill_picture_monoblack(), fill_picture_rgb(), fill_rectangle(), fill_rgb2yuv_table(), fillPlane(), fillPlane16(), filter(), filter_common(), filter_edges(), filter_frame(), filter_line_c(), filter_mb(), filter_mb_dir(), filter_mbedge(), find_header_idx(), find_headers_search_validate(), find_marker(), find_new_headers(), find_smallest_bounding_rectangle(), flac_decode_frame(), flac_decode_init(), flac_encode_init(), flac_fifo_read(), flac_fifo_read_wrap(), flac_header(), flac_parse(), flac_read_header(), flac_write_block_comment(), flac_write_trailer(), flashsv_decode_block(), flashsv_encode_frame(), flic_decode_frame(), flic_decode_init(), flush(), flush_packet(), flush_put_bits(), flv_probe(), flv_read_packet(), flv_write_packet(), format_line(), free_fragment_if_needed(), free_frame_if_needed(), free_section(), g723_1_decode_frame(), g726_reset(), gather_data_for_cel(), gather_data_for_subcel(), gbr24ptopacked24(), gbr24ptopacked32(), gen_buffer_time(), gen_bytes_read(), gen_check_bw(), gen_connect(), gen_create_stream(), gen_delete_stream(), gen_fcpublish_stream(), gen_fcsubscribe_stream(), gen_fcunpublish_stream(), gen_play(), gen_pong(), gen_publish(), gen_release_stream(), gen_server_bw(), gen_swf_verification(), generate_joint_tables(), generate_new_codebooks(), geq_filter_frame(), get(), get16(), get8(), get_4block_rd(), get_bits1(), get_block(), get_block_rd(), get_che(), get_chunk_header(), get_dc(), get_exponent_dynamic(), get_format(), get_generic_seed(), get_line(), get_meta(), get_metadata(), get_metadata_size(), get_moov_size(), get_number(), get_packet(), get_packet_header(), get_packetheader(), get_private_data(), get_pts(), get_siz(), get_slice_data(), get_stats(), getpix(), getstr8(), gif_encode_frame(), gif_image_write_image(), gif_read_ext(), gif_read_header1(), gif_read_image(), gmc1_c(), gmc1_motion(), gmc_motion(), grow_array(), gsm_decode_frame(), guess_mv(), guess_palette(), gxf_probe(), gxf_write_header(), gxf_write_mpeg_auxiliary(), h261_decode_block(), h261_decode_frame(), h263_decode_block(), h263_free_context(), h263_handle_packet(), h263_mv4_search(), h264_handle_packet(), h264_mp4toannexb_filter(), h_block_filter(), halfpel_interpol(), handle_connection(), handle_invoke_error(), handle_invoke_status(), handle_notify(), handle_packet(), handle_packets(), hcscale(), hl_decode_mb(), hl_decode_mb_444(), hl_decode_mb_idct_luma(), hl_decode_mb_predict_luma(), hls_read_header(), html_color_parse(), http_get_file_handle(), http_parse_request(), http_seek(), http_send_data(), hyscale(), id3v2_put_ttag(), idcin_decode_frame(), idcin_read_packet(), idct_add(), idct_mb(), idct_put(), iff_probe(), iff_read_header(), iff_read_packet(), ilbc_decode_frame(), ilbc_read_header(), imc_decode_frame(), imc_read_level_coeffs(), imdct_and_window(), imx_dump_header(), init(), init_coef_vlc(), init_context_frame(), init_dequant4_coeff_table(), init_dequant8_coeff_table(), init_pattern_from_string(), init_ralf_vlc(), init_stream(), initFilter(), interlaced_search(), interpol(), INTERPOLATE_METHOD(), intra_pred_plane(), intra_predict(), ipmovie_probe(), ipmovie_read_header(), ipvideo_decode_frame(), ir2_decode_frame(), is_intra_more_likely(), iso88591_to_utf8(), iterative_me(), ivi_create_huff_from_desc(), ivi_decode_blocks(), jpeg_create_header(), jpeg_parse_packet(), jpeg_put_comments(), jpeg_table_header(), lag_decode_arith_plane(), lag_decode_frame(), lag_decode_prob(), lag_decode_zero_run_line(), lag_get_rac(), latm_free_context(), latm_parse_packet(), latm_write_packet(), lavfi_read_header(), lavfi_read_packet(), left_predict(), libAVMemInputPin_AddRef(), libAVMemInputPin_Destroy(), libAVMemInputPin_QueryInterface(), libAVMemInputPin_Receive(), libAVMemInputPin_Release(), libgsm_decode_frame(), libopenjpeg_copy_to_packed8(), libopenjpeg_copyto8(), libopenjpeg_decode_frame(), libopus_decode_init(), libopus_encode(), libopus_encode_init(), libopus_write_header(), libschroedinger_decode_frame(), libspeex_decode_frame(), linecpy(), ljpeg_decode_rgb_scan(), ljpeg_decode_yuv_scan(), load_cursor(), load_font_file(), load_input_picture(), load_ipmovie_packet(), load_mask(), load_textfile(), loas_probe(), loop_filter(), lsf_decode(), luma_abs_diff(), lxf_read_header(), m4sl_cb(), mace_decode_frame(), main(), make_digest_auth(), matroska_decode_buffer(), matroska_parse_cluster_incremental(), matroska_parse_frame(), matroska_parse_laces(), matroska_read_header(), mb_var_thread(), mc_block(), mc_dir_part(), mc_part_weighted(), mc_subpel(), md5_close(), md5_finish(), mdec_decode_block_intra(), median_predict(), mem2agpcpy_pic(), memcpy_pic2(), merge(), microdvd_probe(), mimic_decode_frame(), mix(), mjpeg2jpeg_filter(), mjpeg_decode_scan(), mjpeg_decode_scan_progressive_ac(), mjpega_dump_header(), mjpegb_decode_frame(), mkv_flush_dynbuf(), mkv_write_attachments(), mkv_write_block(), mkv_write_codecprivate(), mkv_write_header(), mkv_write_simpletag(), mlp_parse(), mm_decode_frame(), motion_arg(), motion_compensation(), mov_create_chapter_track(), mov_flush_fragment(), mov_parse_vc1_frame(), mov_read_chapters(), mov_read_cmov(), mov_read_dvc1(), mov_read_extradata(), mov_read_ftyp(), mov_read_mac_string(), mov_read_packet(), mov_read_sbgp(), mov_text_encode_init(), mov_write_ac3_tag(), mov_write_dvc1_structs(), mov_write_dvc1_tag(), mov_write_int8_metadata(), mov_write_isml_manifest(), mov_write_subtitle_end_packet(), mov_write_tfrf_tag(), mov_write_tfxd_tag(), mov_write_trailer(), mov_write_udta_tag(), mp3_header_compress(), mp3_header_decompress(), mp3_read_probe(), mp3_write_trailer(), mp3_write_xing(), mp_decode_frame(), mp_decode_layer1(), mp_decode_layer3(), mp_yuv_to_rgb(), mpc7_decode_frame(), mpc7_decode_init(), mpc8_decode_frame(), mpc8_parse_seektable(), mpc8_probe(), mpc_probe(), mpeg1_decode_block_inter(), mpeg1_decode_block_intra(), mpeg1_fast_decode_block_inter(), mpeg2_decode_block_intra(), mpeg2_decode_block_non_intra(), mpeg2_fast_decode_block_intra(), mpeg2_fast_decode_block_non_intra(), mpeg4_decode_block(), mpeg4_encode_block(), mpeg4_get_block_length(), mpeg_decode_frame(), mpeg_decode_postinit(), mpeg_decode_slice(), mpeg_decode_user_data(), mpeg_motion_internal(), mpeg_motion_lowres(), mpeg_mux_write_packet(), mpegps_read_pes_header(), mpegts_get_pcr(), mpegts_insert_null_packet(), mpegts_insert_pcr_only(), mpegts_push_data(), mpegts_raw_read_packet(), mpegts_read_header(), mpegts_write_packet_internal(), mpegts_write_pat(), mpegts_write_pes(), mpegts_write_pmt(), mpegts_write_sdt(), mpegts_write_section1(), mpegvideo_extract_headers(), mpjpeg_write_header(), mpjpeg_write_packet(), MPV_decode_mb_internal(), MPV_motion_internal(), MPV_motion_lowres(), msmpeg4v34_decode_mb(), msnwc_tcp_probe(), msrle_decode_8_16_24_32(), msrle_decode_frame(), mss1_decode_frame(), mss2_decode_frame(), mss3_decode_frame(), mss4_decode_dct_block(), mss4_decode_frame(), mss4_decode_image_block(), mss4_init_vlc(), msvideo1_decode_frame(), mszh_decomp(), mul_thrmat_c(), mxf_decrypt_triplet(), mxf_get_d10_aes3_packet(), mxf_parse_dnxhd_frame(), mxf_parse_dv_frame(), mxf_probe(), mxf_read_content_storage(), mxf_read_generic_descriptor(), mxf_read_material_package(), mxf_read_sequence(), mxf_read_source_package(), mxf_write_d10_audio_packet(), mxf_write_header(), mxf_write_multi_descriptor(), mxg_read_packet(), mxg_update_cache(), mxpeg_decode_frame(), mxpeg_decode_mxm(), nal_send(), next_byte(), nsv_read_chunk(), nuv_packet(), nuv_read_dts(), obmc_motion(), ogg_buffer_data(), ogg_build_flac_headers(), ogg_build_opus_headers(), ogg_build_speex_headers(), ogg_new_buf(), ogg_read_page(), ogg_replace_stream(), ogg_write_header(), ogg_write_page(), ogg_write_vorbiscomment(), oggvorbis_decode_init(), oggvorbis_encode_init(), ogm_dshow_header(), ogm_header(), ogm_packet(), old_codec1(), old_codec37(), old_codec47(), old_dirac_header(), oma_read_header(), oma_read_probe(), open_input_file(), open_null_ctx(), open_output_file(), opt_filter_complex_script(), opus_header(), opus_packet(), output_frame_end(), output_plane(), overlay_ass_image(), pack_yuv(), packedCopyWrapper(), packedtogbr24p(), pad_last_frame(), paf_aud_decode(), paf_vid_decode(), paint_mouse_pointer(), palToRgbWrapper(), palToUV_c(), pam_encode_frame(), parse_adts_frame_header(), parse_chunks(), parse_fmtp_config(), parse_fragment(), parse_media_type(), parse_nal_units(), parse_packed_headers(), parse_packet(), parse_palette_segment(), parse_pcr(), parse_picture(), parse_picture_segment(), parse_playlist(), parse_psfile(), parse_setup_header(), parse_timecode(), pat_cb(), pcm_bluray_decode_frame(), pcm_bluray_parse_header(), pcm_decode_frame(), pcm_encode_frame(), pcx_decode_frame(), pcx_encode_frame(), pcx_rle_encode(), picmemset(), picmemset_8bpp(), pix_abs16_xy2_c(), pix_abs16_y2_c(), pix_abs8_xy2_c(), pix_abs8_y2_c(), pix_abs8x8_mvi(), planarCopyWrapper(), planarRgbToRgbWrapper(), planarToNv12Wrapper(), planarToUyvyWrapper(), planarToYuy2Wrapper(), plot_spectrum_column(), pmt_cb(), png_choose_filter(), png_get_interlaced_row(), png_handle_row(), png_put_interlaced_row(), png_write_chunk(), png_write_row(), pnm_decode_frame(), pnm_encode_frame(), pnm_parse(), pop_integer(), postProcess(), pp_filter_frame(), pred16x16_tm_vp8_c(), pred4x4_tm_vp8_c(), pred8x8_tm_vp8_c(), pred_sbsplit(), pred_spatial_direct_motion(), predict_slice(), predict_slice_buffered(), predictor(), predictor_calc_error(), prefetch_motion(), prefetch_ppc(), probe(), probe_codec(), process_audio_header_elements(), process_block(), prores_encode_frame(), prores_encode_init(), prores_encode_picture(), psy_3gpp_init(), psy_3gpp_window(), ptx_decode_frame(), push_integer(), put16(), put_cursor(), put_ebml_id(), put_ebml_num(), put_ebml_uint(), put_main_header(), put_mspel8_mc10_c(), put_mspel8_mc10_sh4(), put_mspel8_mc12_c(), put_mspel8_mc12_sh4(), put_mspel8_mc22_c(), put_mspel8_mc22_sh4(), put_mspel8_mc30_c(), put_mspel8_mc30_sh4(), put_mspel8_mc32_c(), put_mspel8_mc32_sh4(), put_obmc(), put_packet(), put_pixels8x8_overlapped(), put_signed_pixels_clamped_c(), put_sot(), put_str16(), put_swf_matrix(), put_swf_rect(), put_xiph_codecpriv(), putstr8(), pxr24_uncompress(), qcelp_decode_frame(), qcelp_free_context(), qcp_read_header(), qdm2_decode_frame(), qdm2_decode_init(), qdm2_parse_config(), qdm2_parse_packet(), qdm2_parse_subpacket(), qdm2_restore_block(), qpeg_decode_inter(), qpel_motion(), qtrle_decode_frame(), qtrle_encode_line(), queue_frames(), ra144_decode_frame(), ra144_encode_frame(), ra288_decode_frame(), raw_decode(), rd8x8_c(), read_access_unit(), read_and_decode_spectrum(), read_arbitary(), read_argb_line(), read_audio_mux_element(), read_block_types(), read_chapter(), read_code_table(), read_colors(), read_connect(), read_dcs(), read_extra_header(), read_file(), read_frame(), read_gab2_sub(), read_header(), read_huffman_tables(), read_kuki_chunk(), read_motion_values(), read_mv_component(), read_old_huffman_tables(), read_packet(), read_patterns(), read_payload_length_info(), read_quant_table(), read_restart_header(), read_rgb24_component_line(), read_rle_sgi(), read_runs(), read_sbr_header(), read_sbr_invf(), read_shape_from_file(), read_tag(), read_tree(), read_ttag(), read_uncompressed_sgi(), read_xing_toc(), realloc_buffer(), realloc_section_array(), reconstruct_and_encode_image(), remove_extradata(), render_charset(), render_slice(), request_frame(), reset_block_bap(), residual_interp(), restore_median(), restore_median_il(), restore_rgb_planes(), rgb15to16(), rgb15to16_c(), rgb15to24(), rgb15to32(), rgb15to32_c(), rgb15tobgr24(), rgb15tobgr24_c(), rgb15tobgr32(), rgb16to15(), rgb16to15_c(), rgb16to24(), rgb16to32(), rgb16to32_c(), rgb16tobgr24(), rgb16tobgr24_c(), rgb16tobgr32(), rgb24to15(), rgb24to15_c(), rgb24to16(), rgb24to16_c(), rgb24tobgr15(), rgb24tobgr15_c(), rgb24tobgr16(), rgb24tobgr16_c(), rgb24tobgr24(), rgb24tobgr24_c(), rgb24tobgr32(), rgb24tobgr32_c(), rgb32to15(), rgb32to15_c(), rgb32to16(), rgb32to16_c(), rgb32tobgr15(), rgb32tobgr15_c(), rgb32tobgr16(), rgb32tobgr16_c(), rgb32tobgr24(), rgb32tobgr24_c(), rgbToPlanarRgbWrapper(), rgbToRgbWrapper(), rl2_decode_frame(), rl2_decode_init(), rl2_rle_decode(), rm_ac3_swap_bytes(), rm_write_audio(), roq_decode_frame(), roq_dpcm_encode_frame(), roq_write_header(), row_fdct_c(), rpza_decode_frame(), rtmp_calc_swf_verification(), rtmp_calc_swfhash(), rtmp_handshake(), rtmp_http_close(), rtmp_http_send_cmd(), rtmp_open(), rtmp_server_handshake(), rtmp_validate_digest(), rtmp_write(), rtp_asf_fix_header(), rtp_new_av_stream(), rtp_parse_one_packet(), rtp_write_packet(), rtsp_cmd_describe(), run_psnr(), rv10_decode_frame(), rv10_decode_init(), rv20_decode_picture_header(), rv30_loop_filter(), rv30_weak_loop_filter(), rv34_decode_inter_macroblock(), rv34_gen_vlc(), rv34_mc(), rv34_output_i16x16(), rv34_output_intra(), rv34_pred_4x4_block(), rv40_loop_filter(), rv40_loop_filter_strength(), rv40_weak_loop_filter(), s302m_decode_frame(), sad16_xy2_altivec(), sad16_y2_altivec(), sad_hpel_motion_search(), sample_queue_retain(), sap_fetch_packet(), sap_read_header(), scale_slice(), sdl_audio_callback(), sdp_parse_fmtp_config_h264(), sdt_cb(), send_invoke_response(), seqvideo_decode_frame(), set(), set_bandwidth(), set_format(), set_mvs(), set_number(), set_param(), set_string_binary(), setup_partitions(), shall_we_drop(), shift_data(), shorten_decode_frame(), show_stream(), shuffle_bytes_2103(), shuffle_bytes_2103_c(), sipr_decode_frame(), skeleton_header(), skip_check(), slice_decode_thread(), smacker_read_packet(), smc_decode_frame(), smka_decode_frame(), snow_horizontal_compose_liftS_lead_out(), softthresh_c(), sp5x_decode_frame(), spdif_header_eac3(), speex_header(), srt_decode_frame(), srt_read_header(), srt_write_packet(), Stagefright_decode_frame(), Stagefright_init(), store_slice2_c(), store_slice_c(), str_probe(), sub2video_copy_rect(), sub_hfyu_median_prediction_c(), sunrast_decode_frame(), sunrast_image_write_image(), super2xsai(), svq1_decode_block_intra(), svq1_decode_block_non_intra(), svq1_decode_frame(), svq1_decode_frame_header(), svq1_decode_init(), svq1_encode_init(), svq1_encode_plane(), svq1_motion_inter_4v_block(), svq1_motion_inter_block(), svq1_parse_string(), svq1_skip_block(), svq3_decode_block(), svq3_decode_frame(), svq3_decode_init(), svq3_decode_mb(), svq3_decode_slice_header(), svq3_extradata_free(), svq3_mc_dir_part(), svq3_parse_packet(), swf_probe(), swf_read_packet(), swf_write_header(), swr_convert(), swr_inject_silence(), swri_rematrix(), sws_scale(), swScale(), sync(), synth_block_fcb_acb(), tak_decode_frame(), tak_parse(), tak_read_header(), targa_decode_rle(), targa_encode_frame(), targa_encode_normal(), targa_encode_rle(), tcp_write_packet(), tempNoiseReducer(), test_motion(), tgq_decode_block(), tgq_decode_frame(), tgq_decode_init(), tgq_idct_put_mb(), tgq_idct_put_mb_dconly(), tgv_decode_frame(), tgv_decode_inter(), theora_header(), tiff_unpack_strip(), tm2_decode_blocks(), tmv_decode_frame(), to_meta_with_crop(), tqi_decode_frame(), tqi_idct_put(), truemotion1_decode_frame(), truemotion1_decode_header(), truespeech_decode_frame(), tscc2_decode_frame(), tta_check_crc64(), tta_decode_frame(), tta_probe(), twin_decode_frame(), txd_decode_frame(), udp_read(), ulti_convert_yuv(), ulti_decode_frame(), ulti_grad(), ulti_pattern(), unescape(), uninit_options(), unpack(), unpack_parse_unit(), unref_picture(), update_md5_sum(), upsample_plane_c(), usePal(), utvideo_decode_init(), utvideo_encode_frame(), utvideo_encode_init(), uyvyToYuv420Wrapper(), uyvyToYuv422Wrapper(), uyvytoyv12(), uyvytoyv12_unscaled(), v308_decode_frame(), v308_encode_frame(), v408_decode_frame(), v408_encode_frame(), v410_decode_frame(), v410_encode_frame(), v4l_mm_read_picture(), v_block_filter(), vaapi_vc1_start_frame(), vb_decode_framedata(), vble_decode_frame(), vble_restore_plane(), vble_unpack(), vc1_apply_p_h_loop_filter(), vc1_apply_p_v_loop_filter(), vc1_decode_frame(), vc1_decode_i_block(), vc1_decode_i_block_adv(), vc1_decode_i_blocks(), vc1_decode_i_blocks_adv(), vc1_decode_init(), vc1_extract_headers(), vc1_interp_mc(), vc1_mc_1mv(), vc1_mc_4mv_chroma(), vc1_mc_4mv_chroma4(), vc1_mc_4mv_luma(), vc1_pack_bitplanes(), vc1_pred_b_mv(), vcr1_decode_frame(), vertClassify_altivec(), very_broken_op(), vf_default_query_format(), video_decode_example(), video_encode_example(), vmdaudio_decode_frame(), vmdvideo_decode_frame(), vorbis_decode_frame(), vorbis_decode_init(), vorbis_encode_init(), vorbis_header(), vorbis_packet(), vorbis_parse_audio_packet(), vorbis_parse_setup_hdr_codebooks(), vorbis_parse_setup_hdr_residues(), vorbis_residue_decode_internal(), vp3_decode_frame(), vp3_dequant(), vp3_init_thread_copy(), vp56_mc(), vp56_parse_mb_type(), vp5_parse_coeff(), vp5_parse_coeff_models(), vp6_filter_diag2(), vp6_parse_coeff(), vp6_parse_coeff_huffman(), vp6_parse_vector_adjustment(), vp8_decode_mb_row_no_filter(), vp8_filter_mb_row(), vp8_free_buffer(), vp8_mc_chroma(), vp8_mc_luma(), vqf_read_header(), vu9_to_vu12(), vu9_to_vu12_c(), wavesynth_parse_extradata(), wavpack_decode_block(), wavpack_decode_frame(), wc3_read_header(), wc3_read_packet(), webp_decode_frame(), webvtt_probe(), wma_decode_init(), wma_decode_superframe(), wmv2_decode_inter_block(), wmv2_mspel8_h_lowpass(), wmv2_mspel8_v_lowpass(), write_audio_frame(), write_back_motion(), write_back_motion_list(), write_back_non_zero_count(), write_chapter(), write_codebooks(), write_element(), write_escape_str(), write_extradata(), write_frame(), write_globalinfo(), write_header(), write_header_chunk(), write_headers(), write_mb_info(), write_option(), write_packet(), write_quant_table(), write_streaminfo(), write_utf8(), write_video_frame(), writeout(), ws_snd_decode_frame(), wtvfile_open2(), wtvfile_open_sector(), X264_init(), x8_decode_intra_mb(), x8_get_ac_rlf(), x8_setup_spatial_compensation(), x8_setup_spatial_predictor(), xan_decode_chroma(), xan_decode_frame(), xan_decode_frame_type0(), xan_decode_frame_type1(), xan_unpack(), xan_unpack_luma(), xan_wc3_decode_frame(), XAVS_init(), xbm_decode_frame(), xbm_encode_frame(), xchg_mb_border(), xface_decode_frame(), xface_encode_frame(), xiph_parse_fmtp_pair(), xmv_fetch_video_packet(), xmv_process_packet_header(), xsub_encode(), xwd_decode_frame(), xwd_encode_frame(), y41p_decode_frame(), y41p_encode_frame(), yae_downmix(), yae_flush(), yae_load_data(), yae_load_frag(), yae_overlap_add(), yop_copy_previous_block(), yuv2mono_1_c_template(), yuv2mono_2_c_template(), yuv2mono_X_c_template(), yuv2nv12cX_c(), yuv2rgb_write(), yuv2yuyv422_1(), yuv420_bgr32(), yuv420_rgb32(), yuv422pToUyvyWrapper(), yuv422pToYuy2Wrapper(), yuv4_decode_frame(), yuv4_encode_frame(), yuv_a_to_rgba(), yuvPlanartouyvy_c(), yuvPlanartoyuy2_c(), yuy2toyv12(), yuyvToYuv420Wrapper(), yuyvToYuv422Wrapper(), yuyvtoyv12_unscaled(), yv12touyvy_unscaled_altivec(), yv12toyuy2_unscaled_altivec(), yvu9_to_yuy2(), yvu9_to_yuy2_c(), zero12v_decode_frame(), ZERO8x2(), zerocodec_decode_frame(), zmbv_decode_intra(), zmbv_decode_xor_16(), zmbv_decode_xor_32(), and zmbv_decode_xor_8().
Generated on Wed Apr 16 2025 06:53:24 for FFmpeg by
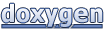