FFmpeg
|
aacpsdsp.c
Go to the documentation of this file.
static void ps_stereo_interpolate_c(float(*l)[2], float(*r)[2], float h[2][4], float h_step[2][4], int len)
Definition: aacpsdsp.c:134
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
static void ps_hybrid_synthesis_deint_c(float out[2][38][64], float(*in)[32][2], int i, int len)
Definition: aacpsdsp.c:80
Macro definitions for various function/variable attributes.
static void ps_mul_pair_single_c(float(*dst)[2], float(*src0)[2], float *src1, int n)
Definition: aacpsdsp.c:32
void(* stereo_interpolate[2])(float(*l)[2], float(*r)[2], float h[2][4], float h_step[2][4], int len)
Definition: aacpsdsp.h:45
void(* mul_pair_single)(float(*dst)[2], float(*src0)[2], float *src1, int n)
Definition: aacpsdsp.h:30
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
void(* hybrid_synthesis_deint)(float out[2][38][64], float(*in)[32][2], int i, int len)
Definition: aacpsdsp.h:37
Definition: aacpsdsp.h:28
static void ps_stereo_interpolate_ipdopd_c(float(*l)[2], float(*r)[2], float h[2][4], float h_step[2][4], int len)
Definition: aacpsdsp.c:165
static void ps_add_squares_c(float *dst, const float(*src)[2], int n)
Definition: aacpsdsp.c:25
static void ps_hybrid_analysis_c(float(*out)[2], float(*in)[2], const float(*filter)[8][2], int stride, int n)
Definition: aacpsdsp.c:42
void(* hybrid_analysis)(float(*out)[2], float(*in)[2], const float(*filter)[8][2], int stride, int n)
Definition: aacpsdsp.h:32
void(* hybrid_analysis_ileave)(float(*out)[32][2], float L[2][38][64], int i, int len)
Definition: aacpsdsp.h:35
void(* decorrelate)(float(*out)[2], float(*delay)[2], float(*ap_delay)[PS_QMF_TIME_SLOTS+PS_MAX_AP_DELAY][2], const float phi_fract[2], float(*Q_fract)[2], const float *transient_gain, float g_decay_slope, int len)
Definition: aacpsdsp.h:39
void(* add_squares)(float *dst, const float(*src)[2], int n)
Definition: aacpsdsp.h:29
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static void ps_decorrelate_c(float(*out)[2], float(*delay)[2], float(*ap_delay)[PS_QMF_TIME_SLOTS+PS_MAX_AP_DELAY][2], const float phi_fract[2], float(*Q_fract)[2], const float *transient_gain, float g_decay_slope, int len)
Definition: aacpsdsp.c:94
static void ps_hybrid_analysis_ileave_c(float(*out)[32][2], float L[2][38][64], int i, int len)
Definition: aacpsdsp.c:67
Generated on Wed May 7 2025 06:52:37 for FFmpeg by
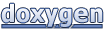