FFmpeg
|
#include "libavutil/opt.h"
#include "libavutil/bprint.h"
#include "libavutil/eval.h"
#include "libavutil/file.h"
#include "libavutil/intreadwrite.h"
#include "libavutil/avassert.h"
#include "avfilter.h"
#include "formats.h"
#include "internal.h"
#include "video.h"
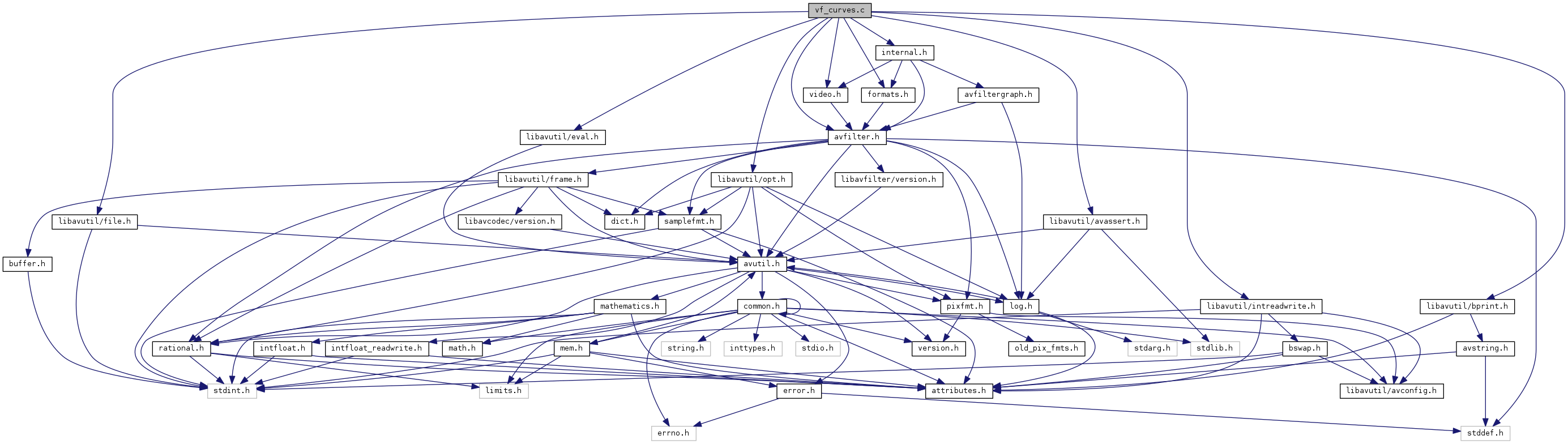
Go to the source code of this file.
Data Structures | |
struct | keypoint |
struct | CurvesContext |
Macros | |
#define | NB_COMP 3 |
#define | OFFSET(x) offsetof(CurvesContext, x) |
#define | FLAGS AV_OPT_FLAG_FILTERING_PARAM|AV_OPT_FLAG_VIDEO_PARAM |
#define | B 0 /* sub diagonal (below main) */ |
#define | M 1 /* main diagonal (center) */ |
#define | A 2 /* sup diagonal (above main) */ |
#define | READ16(dst) |
#define | SET_COMP_IF_NOT_SET(n, name) |
Functions | |
AVFILTER_DEFINE_CLASS (curves) | |
static struct keypoint * | make_point (double x, double y, struct keypoint *next) |
static int | parse_points_str (AVFilterContext *ctx, struct keypoint **points, const char *s) |
static int | get_nb_points (const struct keypoint *d) |
static int | interpolate (AVFilterContext *ctx, uint8_t *y, const struct keypoint *points) |
Natural cubic spline interpolation Finding curves using Cubic Splines notes by Steven Rauch and John Stockie. More... | |
static int | parse_psfile (AVFilterContext *ctx, const char *fname) |
static av_cold int | init (AVFilterContext *ctx) |
static int | query_formats (AVFilterContext *ctx) |
static int | filter_frame (AVFilterLink *inlink, AVFrame *in) |
Variables | |
static const AVOption | curves_options [] |
struct { | |
const char * r | |
const char * g | |
const char * b | |
const char * master | |
} | curves_presets [] |
static const AVFilterPad | curves_inputs [] |
static const AVFilterPad | curves_outputs [] |
AVFilter | avfilter_vf_curves |
Macro Definition Documentation
Referenced by interpolate().
#define B 0 /* sub diagonal (below main) */ |
Referenced by interpolate().
#define FLAGS AV_OPT_FLAG_FILTERING_PARAM|AV_OPT_FLAG_VIDEO_PARAM |
Definition at line 64 of file vf_curves.c.
#define M 1 /* main diagonal (center) */ |
Referenced by interpolate().
#define NB_COMP 3 |
Definition at line 37 of file vf_curves.c.
Referenced by filter_frame(), and init().
#define OFFSET | ( | x | ) | offsetof(CurvesContext, x) |
Definition at line 63 of file vf_curves.c.
#define READ16 | ( | dst | ) |
Referenced by parse_psfile().
Referenced by init().
Enumeration Type Documentation
enum preset |
Definition at line 39 of file vf_curves.c.
Function Documentation
AVFILTER_DEFINE_CLASS | ( | curves | ) |
|
static |
Definition at line 449 of file vf_curves.c.
|
static |
Definition at line 194 of file vf_curves.c.
Referenced by interpolate().
|
static |
Definition at line 359 of file vf_curves.c.
|
static |
Natural cubic spline interpolation Finding curves using Cubic Splines notes by Steven Rauch and John Stockie.
Definition at line 209 of file vf_curves.c.
Referenced by init().
Definition at line 123 of file vf_curves.c.
Referenced by parse_points_str().
|
static |
Definition at line 135 of file vf_curves.c.
Referenced by init().
|
static |
Definition at line 305 of file vf_curves.c.
Referenced by init().
|
static |
Definition at line 442 of file vf_curves.c.
Variable Documentation
AVFilter avfilter_vf_curves |
Definition at line 508 of file vf_curves.c.
const char* b |
Definition at line 96 of file vf_curves.c.
Referenced by init(), and interpolate().
|
static |
Definition at line 491 of file vf_curves.c.
|
static |
Definition at line 65 of file vf_curves.c.
|
static |
Definition at line 500 of file vf_curves.c.
const { ... } curves_presets[] |
const char* g |
Definition at line 95 of file vf_curves.c.
Referenced by init().
const char* master |
Definition at line 97 of file vf_curves.c.
Referenced by init(), and revert_channel_correlation().
const char* r |
Definition at line 94 of file vf_curves.c.
Referenced by add_bell(), add_hfyu_left_prediction_bgr32_c(), alloc_frame_buffer(), av_des_mac(), av_find_nearest_q_idx(), av_inv_q(), av_realloc_f(), av_rescale_rnd(), avcodec_default_execute(), avcodec_default_execute2(), avfilter_graph_request_oldest(), avfilter_graph_send_command(), avpriv_set_systematic_pal2(), avs_get_row_size_p(), cache_read(), cdg_load_palette(), cdxl_decode_ham6(), cdxl_decode_ham8(), cinepak_decode_codebook(), cng_decode_frame(), color_distance(), compute_lpc_coefs(), config_output(), config_props(), convert_timestamp(), crypt(), dca_downmix(), DECLARE_ALIGNED(), decode_block(), decode_block_coeffs_internal(), decode_frame(), decode_hybrid(), decode_mvc2(), decode_pal(), decode_rbsp_trailing(), decode_refpass(), decode_rgb_frame(), decode_rice(), dither_int_to_float_triangular_c(), dshow_cycle_devices(), dshow_cycle_pins(), dshow_list_device_options(), dshow_open_device(), dshow_read_close(), dshow_read_header(), dv_read_seek(), dvbsub_init_decoder(), dvbsub_parse_clut_segment(), encode_dvb_subtitles(), encode_frame(), encode_rgb_frame(), encode_scalar(), encode_thread(), eval_expr(), expand_script(), expand_tseq(), ff_alsa_extend_reorder_buf(), ff_draw_supported_pixel_formats(), ff_gmc_c(), ff_init_cabac_states(), ff_lag_rac_init(), ff_mov_read_stsd_entries(), ff_parse_time_base(), ff_rac_terminate(), ff_rgb24toyv12_c(), ff_rtp_send_h264(), ff_rtp_send_mpegvideo(), file_read(), file_write(), fill_picture_parameters(), fill_slice_long(), filter(), filter_frame(), flic_decode_frame_8BPP(), frac64(), generate_interval(), generate_intervals(), generate_joint_tables(), generate_plateau(), generate_transition(), get_bits_left(), get_high_utility_cell(), get_sr_golomb_shorten(), get_strl(), get_symbol2(), guess_palette(), h263_handle_packet(), hpel_motion_search(), idcin_read_packet(), idct_col2(), idct_row(), import_palette(), init(), interpolate(), iterative_me(), lag_decode_frame(), lex_char(), lex_time(), ls_decode_line(), main(), make_cdt15_entry(), make_cdt16_entry(), make_cdt24_entry(), matroska_parse_laces(), mc_block(), modplug_read_header(), mp_yuv_to_rgb(), mpeg4_decode_sprite_trajectory(), mpegts_push_data(), mss2_blit_wmv9_template(), mss2_decode_frame(), mul32(), MULH(), opt_list(), paf_vid_decode(), paint_mouse_pointer(), parse_block_def(), parse_options(), parse_palette_segment(), parse_script(), parse_synth_channel(), parse_synth_def(), parse_time_sequence(), parse_timestamp(), pix_abs16x16_x2_mvi(), png_filter_row(), pop_integer(), pow_poly(), process_ipmovie_chunk(), ps_stereo_interpolate_c(), ps_stereo_interpolate_ipdopd_c(), push_integer(), put_pixel(), put_symbol2(), qpel_motion_search(), qtrle_decode_24bpp(), read_var_block_data(), read_whole_file(), request_frame(), restore_rgb_planes(), rgb12to15(), rgb24_to_yuv420p(), rgb24to15(), rgb24to15_c(), rgb24to16(), rgb24to16_c(), rgb24tobgr15(), rgb24tobgr15_c(), rgb24tobgr16(), rgb24tobgr16_c(), ring2_test(), roundToInt16(), rtmp_close(), rtmp_get_file_handle(), rtmp_open(), rtmp_read(), rtmp_read_pause(), rtmp_read_seek(), rtmp_write(), rtsp_read_packet(), rv30_decode_init(), rv40_decode_init(), sad_hpel_motion_search(), sbg_read_header(), sbg_read_probe(), search_keyval(), set_palette(), smacker_decode_bigtree(), smacker_decode_tree(), stereo_processing(), sub_left_prediction_bgr32(), sub_left_prediction_rgb24(), subtitle_thread(), super2xsai(), swri_rematrix_init(), sws_scale(), tta_decode_frame(), update_palette_index(), vmd_decode(), vmdvideo_decode_init(), vqa_decode_chunk(), wavesynth_decode(), wavesynth_init(), xan_decode_frame(), xface_encode_frame(), yuv2rgb_1_c_template(), yuv2rgb_2_c_template(), yuv2rgb_write(), yuv2rgb_write_full(), yuv2rgb_X_c_template(), and yuv_a_to_rgba().
Generated on Wed May 8 2024 06:52:21 for FFmpeg by
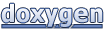