FFmpeg
|
alacenc.c
Go to the documentation of this file.
447 const enum AlacRawDataBlockType *ch_elements = ff_alac_channel_elements[s->avctx->channels - 1];
static void av_unused put_bits32(PutBitContext *s, uint32_t value)
Definition: put_bits.h:182
Definition: frame.h:76
Definition: put_bits.h:41
Definition: lpc.h:50
int ff_lpc_calc_coefs(LPCContext *s, const int32_t *samples, int blocksize, int min_order, int max_order, int precision, int32_t coefs[][MAX_LPC_ORDER], int *shift, enum FFLPCType lpc_type, int lpc_passes, int omethod, int max_shift, int zero_shift)
Definition: lpc.c:170
#define COPY_SAMPLES(type)
static void write_element_header(AlacEncodeContext *s, enum AlacRawDataBlockType element, int instance)
Definition: alacenc.c:130
Definition: libavcodec/avcodec.h:2854
Definition: libavcodec/avcodec.h:397
static void write_element(AlacEncodeContext *s, enum AlacRawDataBlockType element, int instance, const uint8_t *samples0, const uint8_t *samples1)
Definition: alacenc.c:360
Definition: samplefmt.h:50
static void alac_linear_predictor(AlacEncodeContext *s, int ch)
Definition: alacenc.c:252
struct AlacLPCContext AlacLPCContext
static void calc_predictor_params(AlacEncodeContext *s, int ch)
Definition: alacenc.c:149
static int alac_encode_frame(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Definition: alacenc.c:602
struct RiceContext RiceContext
Definition: avutil.h:144
Definition: alacenc.c:53
Definition: alacenc.c:46
#define FF_INPUT_BUFFER_PADDING_SIZE
Definition: libavcodec/avcodec.h:561
static av_always_inline int get_max_frame_size(int frame_size, int ch, int bps)
Definition: alacenc.c:474
const uint64_t ff_alac_channel_layouts[ALAC_MAX_CHANNELS+1]
Definition: alac_data.c:35
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Definition: libavcodec/utils.c:1377
int av_get_bytes_per_sample(enum AVSampleFormat sample_fmt)
Definition: samplefmt.c:104
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip please avoid problems through this extra level of scrutiny For cosmetics only commits you should e g by running git config global user name My Name git config global user email my email which is either set in your personal configuration file through git config core editor or set by one of the following environment VISUAL or EDITOR Log messages should be concise but descriptive Explain why you made a what you did will be obvious from the changes themselves most of the time Saying just bug fix or is bad Remember that people of varying skill levels look at and educate themselves while reading through your code Don t include filenames in log Git provides that information Possibly make the commit message have a descriptive first an empty line and then a full description The first line will be used to name the patch by git format patch Renaming moving copying files or contents of making those normal commits mv cp path file otherpath otherfile git add[-A] git commit Do not rename or copy files of which you are not the maintainer without discussing it on the mailing list first Reverting broken commits git revert< commit > git revert will generate a revert commit This will not make the faulty commit disappear from the history git reset< commit > git reset will uncommit the changes till< commit > rewriting the current branch history git commit amend allows to amend the last commit details quickly git rebase i origin master will replay local commits over the main repository allowing to merge or remove some of them in the process Note that the commit amend and rebase rewrite history
Definition: git-howto.txt:153
Definition: libavcodec/avcodec.h:1134
static void init_sample_buffers(AlacEncodeContext *s, int channels, uint8_t const *samples[2])
Definition: alacenc.c:80
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
const uint8_t ff_alac_channel_layout_offsets[ALAC_MAX_CHANNELS][ALAC_MAX_CHANNELS]
Definition: alac_data.c:24
av_cold int ff_lpc_init(LPCContext *s, int blocksize, int max_order, enum FFLPCType lpc_type)
Definition: lpc.c:258
static int write_frame(AlacEncodeContext *s, AVPacket *avpkt, uint8_t *const *samples)
Definition: alacenc.c:443
enum AlacRawDataBlockType ff_alac_channel_elements[ALAC_MAX_CHANNELS][5]
Definition: alac_data.c:47
static int estimate_stereo_mode(int32_t *left_ch, int32_t *right_ch, int n)
Definition: alacenc.c:179
static av_cold int alac_encode_close(AVCodecContext *avctx)
Definition: alacenc.c:480
static void init_put_bits(PutBitContext *s, uint8_t *buffer, int buffer_size)
Definition: put_bits.h:54
Definition: alacenc.c:59
struct AlacEncodeContext AlacEncodeContext
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
Definition: libavcodec/avcodec.h:1028
static void alac_stereo_decorrelation(AlacEncodeContext *s)
Definition: alacenc.c:212
static void encode_scalar(AlacEncodeContext *s, int x, int k, int write_sample_size)
Definition: alacenc.c:102
Generated on Wed Aug 6 2025 06:53:25 for FFmpeg by
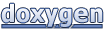