FFmpeg
|
libavcodec/avcodec.h
Go to the documentation of this file.
93 * 2. Give it a value which when taken as ASCII is recognized uniquely by a human as this specific codec.
480 AV_CODEC_ID_FIRST_UNKNOWN = 0x18000, ///< A dummy ID pointing at the start of various fake codecs.
490 AV_CODEC_ID_PROBE = 0x19000, ///< codec_id is not known (like AV_CODEC_ID_NONE) but lavf should attempt to identify it
496 AV_CODEC_ID_FFMETADATA = 0x21000, ///< Dummy codec for streams containing only metadata information.
555 * Required number of additionally allocated bytes at the end of the input bitstream for decoding.
606 AVCOL_PRI_BT470BG = 5, ///< also ITU-R BT601-6 625 / ITU-R BT1358 625 / ITU-R BT1700 625 PAL & SECAM
627 AVCOL_SPC_BT470BG = 5, ///< also ITU-R BT601-6 625 / ITU-R BT1358 625 / ITU-R BT1700 625 PAL & SECAM / IEC 61966-2-4 xvYCC601
628 AVCOL_SPC_SMPTE170M = 6, ///< also ITU-R BT601-6 525 / ITU-R BT1358 525 / ITU-R BT1700 NTSC / functionally identical to above
714 #define CODEC_FLAG_GLOBAL_HEADER 0x00400000 ///< Place global headers in extradata instead of every keyframe.
720 #define CODEC_FLAG_CLOSED_GOP 0x80000000
723 #define CODEC_FLAG2_LOCAL_HEADER 0x00000008 ///< Place global headers at every keyframe instead of in extradata.
724 #define CODEC_FLAG2_DROP_FRAME_TIMECODE 0x00002000 ///< timecode is in drop frame format. DEPRECATED!!!!
727 #define CODEC_FLAG2_CHUNKS 0x00008000 ///< Input bitstream might be truncated at a packet boundaries instead of only at frame boundaries.
886 int16_t position[3][2];
897 #define FF_BUFFER_TYPE_SHARED 4 ///< Buffer from somewhere else; don't deallocate image (data/base), all other tables are not shared.
898 #define FF_BUFFER_TYPE_COPY 8 ///< Just a (modified) copy of some other buffer, don't deallocate anything.
1163 * fourcc from the AVI stream header (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
1501 * Numerator and denominator must be relatively prime and smaller than 256 for some video standards.
1637 #define SLICE_FLAG_CODED_ORDER 0x0001 ///< draw_horiz_band() is called in coded order instead of display
1638 #define SLICE_FLAG_ALLOW_FIELD 0x0002 ///< allow draw_horiz_band() with field slices (MPEG2 field pics)
1639 #define SLICE_FLAG_ALLOW_PLANE 0x0004 ///< allow draw_horiz_band() with 1 component at a time (SVQ1)
2222 * Ratecontrol attempt to use, at least, <value> times the amount needed to prevent a vbv overflow.
2376 #define FF_BUG_OLD_MSMPEG4 2
2381 #define FF_BUG_AC_VLC 0 ///< Will be removed, libavcodec can now handle these non-compliant files by default.
2382 #define FF_BUG_QPEL_CHROMA 64
2390 #define FF_BUG_TRUNCATED 16384
2405 #define FF_COMPLIANCE_VERY_STRICT 2 ///< Strictly conform to an older more strict version of the spec or reference software.
2406 #define FF_COMPLIANCE_STRICT 1 ///< Strictly conform to all the things in the spec no matter what consequences.
2407 #define FF_COMPLIANCE_NORMAL 0
2615 int (*execute)(struct AVCodecContext *c, int (*func)(struct AVCodecContext *c2, void *arg), void *arg2, int *ret, int count, int size);
2626 * @param ret return values of executed functions, must have space for "count" values. May be NULL.
2630 * @return always 0 currently, but code should handle a future improvement where when any call to func
2635 int (*execute2)(struct AVCodecContext *c, int (*func)(struct AVCodecContext *c2, void *arg, int jobnr, int threadnr), void *arg2, int *ret, int count);
2828 #define FF_SUB_CHARENC_MODE_DO_NOTHING -1 ///< do nothing (demuxer outputs a stream supposed to be already in UTF-8, or the codec is bitmap for instance)
2830 #define FF_SUB_CHARENC_MODE_PRE_DECODER 1 ///< the AVPacket data needs to be recoded to UTF-8 before being fed to the decoder, requires iconv
2874 const AVRational *supported_framerates; ///< array of supported framerates, or NULL if any, array is terminated by {0,0}
2875 const enum AVPixelFormat *pix_fmts; ///< array of supported pixel formats, or NULL if unknown, array is terminated by -1
2876 const int *supported_samplerates; ///< array of supported audio samplerates, or NULL if unknown, array is terminated by 0
2877 const enum AVSampleFormat *sample_fmts; ///< array of supported sample formats, or NULL if unknown, array is terminated by -1
2878 const uint64_t *channel_layouts; ///< array of support channel layouts, or NULL if unknown. array is terminated by 0
2881 const AVProfile *profiles; ///< array of recognized profiles, or NULL if unknown, array is terminated by {FF_PROFILE_UNKNOWN}
3714 * @param[in,out] got_picture_ptr Zero if no frame could be decompressed, otherwise, it is nonzero.
3735 * @param[in,out] got_sub_ptr Zero if no subtitle could be decompressed, otherwise, it is nonzero.
3909 * @param buf_size input length, to signal EOF, this should be 0 (so that the last frame can be output).
3937 * @return 0 if the output buffer is a subset of the input, 1 if it is allocated and must be freed
4131 * @param filter_length length of each FIR filter in the filterbank relative to the cutoff frequency
4169 struct AVResampleContext *av_resample_init(int out_rate, int in_rate, int filter_length, int log2_phase_count, int linear, double cutoff);
4177 * @param update_ctx If this is 0 then the context will not be modified, that way several channels can be resampled with the same context.
4181 int av_resample(struct AVResampleContext *c, short *dst, short *src, int *consumed, int src_size, int dst_size, int update_ctx);
4187 * @param compensation_distance distance in output samples over which the compensation should be performed
4197 void av_resample_compensate(struct AVResampleContext *c, int sample_delta, int compensation_distance);
4286 int av_picture_pad(AVPicture *dst, const AVPicture *src, int height, int width, enum AVPixelFormat pix_fmt,
4387 * converting from some formats to other formats. avcodec_find_best_pix_fmt_of_2() selects which of
4390 * If one of the destination formats is AV_PIX_FMT_NONE the other pixel format (if valid) will be
4399 * dst_pix_fmt = avcodec_find_best_pix_fmt_of_2(dst_pix_fmt1, dst_pix_fmt2, src_pix_fmt, alpha, &loss);
4400 * dst_pix_fmt = avcodec_find_best_pix_fmt_of_2(dst_pix_fmt, dst_pix_fmt3, src_pix_fmt, alpha, &loss);
4407 * @param[in, out] loss_ptr Combination of loss flags. In: selects which of the losses to ignore, i.e.
4412 enum AVPixelFormat avcodec_find_best_pix_fmt_of_2(enum AVPixelFormat dst_pix_fmt1, enum AVPixelFormat dst_pix_fmt2,
4421 enum AVPixelFormat avcodec_find_best_pix_fmt2(enum AVPixelFormat dst_pix_fmt1, enum AVPixelFormat dst_pix_fmt2,
4426 enum AVPixelFormat avcodec_default_get_format(struct AVCodecContext *s, const enum AVPixelFormat * fmt);
4454 int avcodec_default_execute(AVCodecContext *c, int (*func)(AVCodecContext *c2, void *arg2),void *arg, int *ret, int count, int size);
4455 int avcodec_default_execute2(AVCodecContext *c, int (*func)(AVCodecContext *c2, void *arg2, int, int),void *arg, int *ret, int count);
4572 * @param ptr pointer to pointer to already allocated buffer, overwritten with pointer to new buffer
Definition: libavcodec/avcodec.h:162
Definition: libavcodec/avcodec.h:367
Definition: libavcodec/avcodec.h:312
codec_id is not known (like AV_CODEC_ID_NONE) but lavf should attempt to identify it ...
Definition: libavcodec/avcodec.h:490
Definition: libavcodec/avcodec.h:437
Definition: libavcodec/avcodec.h:271
Definition: libavcodec/avcodec.h:342
Definition: libavcodec/avcodec.h:410
unsigned int stream_codec_tag
fourcc from the AVI stream header (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A')...
Definition: libavcodec/avcodec.h:1168
const AVCodecDescriptor * codec_descriptor
AVCodecDescriptor Code outside libavcodec should access this field using: av_codec_{get,set}_codec_descriptor(avctx)
Definition: libavcodec/avcodec.h:2802
Definition: libavcodec/avcodec.h:279
Definition: libavcodec/avcodec.h:315
Definition: libavcodec/avcodec.h:100
Definition: libavcodec/avcodec.h:134
struct AVCodecParser AVCodecParser
Definition: libavcodec/avcodec.h:197
Definition: libavcodec/avcodec.h:368
int64_t pts_correction_num_faulty_dts
Number of incorrect PTS values so far.
Definition: libavcodec/avcodec.h:2810
Definition: libavcodec/avcodec.h:210
Definition: libavcodec/avcodec.h:202
Definition: libavcodec/avcodec.h:303
int avcodec_default_execute2(AVCodecContext *c, int(*func)(AVCodecContext *c2, void *arg2, int, int), void *arg, int *ret, int count)
void avcodec_default_release_buffer(AVCodecContext *s, AVFrame *pic)
Definition: libavcodec/utils.c:877
AVCodec * avcodec_find_encoder(enum AVCodecID id)
Find a registered encoder with a matching codec ID.
Definition: libavcodec/utils.c:2378
Definition: libavcodec/avcodec.h:448
Definition: libavcodec/avcodec.h:344
A dummy id pointing at the start of audio codecs.
Definition: libavcodec/avcodec.h:298
Definition: libavcodec/avcodec.h:170
Definition: libavcodec/avcodec.h:476
Definition: libavcodec/avcodec.h:439
Definition: libavcodec/avcodec.h:301
Definition: libavcodec/avcodec.h:463
int coded_width
Bitstream width / height, may be different from width/height e.g.
Definition: libavcodec/avcodec.h:1312
int av_lockmgr_register(int(*cb)(void **mutex, enum AVLockOp op))
Register a user provided lock manager supporting the operations specified by AVLockOp.
Definition: libavcodec/utils.c:2950
Definition: libavcodec/avcodec.h:407
Definition: libavcodec/avcodec.h:164
float border_masking
Border processing masking, raises the quantizer for mbs on the borders of the picture.
Definition: libavcodec/avcodec.h:1730
Definition: libavcodec/avcodec.h:232
int attribute_align_arg avcodec_decode_audio3(AVCodecContext *avctx, int16_t *samples, int *frame_size_ptr, AVPacket *avpkt)
Definition: libavcodec/utils.c:1985
void av_shrink_packet(AVPacket *pkt, int size)
Reduce packet size, correctly zeroing padding.
Definition: avpacket.c:97
Definition: libavcodec/avcodec.h:259
int64_t next_frame_offset
Definition: libavcodec/avcodec.h:3753
int max_b_frames
maximum number of B-frames between non-B-frames Note: The output will be delayed by max_b_frames+1 re...
Definition: libavcodec/avcodec.h:1385
Definition: libavcodec/avcodec.h:236
int rc_initial_buffer_occupancy
Number of bits which should be loaded into the rc buffer before decoding starts.
Definition: libavcodec/avcodec.h:2233
Definition: libavcodec/avcodec.h:474
Dummy codec for streams containing only metadata information.
Definition: libavcodec/avcodec.h:496
Definition: libavcodec/avcodec.h:173
Definition: libavcodec/avcodec.h:240
Definition: libavcodec/avcodec.h:186
const char * avcodec_configuration(void)
Return the libavcodec build-time configuration.
Definition: libavcodec/utils.c:2597
Definition: libavcodec/avcodec.h:166
Definition: libavcodec/avcodec.h:157
void av_codec_set_pkt_timebase(AVCodecContext *avctx, AVRational val)
Definition: libavcodec/avcodec.h:409
void avcodec_set_dimensions(AVCodecContext *s, int width, int height)
Definition: libavcodec/utils.c:177
int nb_colors
number of colors in pict, undefined when pict is not set
Definition: libavcodec/avcodec.h:3081
Definition: libavcodec/avcodec.h:475
int av_copy_packet(AVPacket *dst, AVPacket *src)
Copy packet, including contents.
Definition: avpacket.c:236
const char * avcodec_license(void)
Return the libavcodec license.
Definition: libavcodec/utils.c:2602
Definition: libavcodec/avcodec.h:276
Definition: libavcodec/avcodec.h:184
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
Definition: libavcodec/avcodec.h:112
int av_picture_crop(AVPicture *dst, const AVPicture *src, enum AVPixelFormat pix_fmt, int top_band, int left_band)
Crop image top and left side.
Definition: imgconvert.c:384
Definition: libavcodec/avcodec.h:177
Definition: libavcodec/avcodec.h:393
external API header
Definition: libavcodec/avcodec.h:424
int avpicture_layout(const AVPicture *src, enum AVPixelFormat pix_fmt, int width, int height, unsigned char *dest, int dest_size)
Copy pixel data from an AVPicture into a buffer, always assume a linesize alignment of 1...
Definition: avpicture.c:41
Definition: libavcodec/avcodec.h:158
Definition: libavcodec/avcodec.h:255
void av_fast_padded_malloc(void *ptr, unsigned int *size, size_t min_size)
Same behaviour av_fast_malloc but the buffer has additional FF_INPUT_BUFFER_PADDING_SIZE at the end w...
Definition: libavcodec/utils.c:101
Definition: libavcodec/avcodec.h:470
A dummy ID pointing at the start of various fake codecs.
Definition: libavcodec/avcodec.h:480
Definition: avisynth_c.h:503
Definition: libavcodec/avcodec.h:245
char * stats_in
pass2 encoding statistics input buffer Concatenated stuff from stats_out of pass1 should be placed he...
Definition: libavcodec/avcodec.h:2367
Definition: libavcodec/avcodec.h:115
Definition: libavcodec/avcodec.h:604
size_t av_get_codec_tag_string(char *buf, size_t buf_size, unsigned int codec_tag)
Put a string representing the codec tag codec_tag in buf.
Definition: libavcodec/utils.c:2436
Definition: libavcodec/avcodec.h:123
AVChromaLocation
X X 3 4 X X are luma samples, 1 2 1-6 are possible chroma positions X X 5 6 X 0 is undefined/unknown ...
Definition: libavcodec/avcodec.h:647
Definition: libavcodec/avcodec.h:260
Definition: libavcodec/avcodec.h:375
Definition: libavcodec/avcodec.h:251
Definition: libavcodec/avcodec.h:395
int avcodec_encode_audio2(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Encode a frame of audio.
Definition: libavcodec/utils.c:1471
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
void avcodec_register_all(void)
Register all the codecs, parsers and bitstream filters which were enabled at configuration time...
Definition: allcodecs.c:67
Definition: libavcodec/avcodec.h:234
int dts_ref_dts_delta
Offset of the current timestamp against last timestamp sync point in units of AVCodecContext.time_base.
Definition: libavcodec/avcodec.h:3842
int avcodec_decode_subtitle2(AVCodecContext *avctx, AVSubtitle *sub, int *got_sub_ptr, AVPacket *avpkt)
Decode a subtitle message.
Definition: libavcodec/utils.c:2209
Definition: libavcodec/avcodec.h:130
An AV_PKT_DATA_H263_MB_INFO side data packet contains a number of structures with info about macroblo...
Definition: libavcodec/avcodec.h:957
Definition: libavcodec/avcodec.h:466
int avcodec_copy_context(AVCodecContext *dest, const AVCodecContext *src)
Copy the settings of the source AVCodecContext into the destination AVCodecContext.
Definition: libavcodec/options.c:182
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
Definition: libavcodec/avcodec.h:391
Definition: libavcodec/avcodec.h:413
Definition: libavcodec/avcodec.h:397
int avpicture_fill(AVPicture *picture, const uint8_t *ptr, enum AVPixelFormat pix_fmt, int width, int height)
Fill in the AVPicture fields, always assume a linesize alignment of 1.
Definition: avpicture.c:34
Definition: libavcodec/avcodec.h:346
uint64_t vbv_delay
VBV delay coded in the last frame (in periods of a 27 MHz clock).
Definition: libavcodec/avcodec.h:2784
Definition: libavcodec/avcodec.h:194
int scenechange_threshold
scene change detection threshold 0 is default, larger means fewer detected scene changes.
Definition: libavcodec/avcodec.h:1678
Definition: libavcodec/avcodec.h:653
Definition: libavcodec/avcodec.h:406
Definition: libavcodec/avcodec.h:154
Definition: libavcodec/avcodec.h:401
Definition: libavcodec/avcodec.h:275
Definition: libavcodec/avcodec.h:215
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
Definition: libavcodec/avcodec.h:187
int avcodec_get_pix_fmt_loss(enum AVPixelFormat dst_pix_fmt, enum AVPixelFormat src_pix_fmt, int has_alpha)
Compute what kind of losses will occur when converting from one specific pixel format to another...
Definition: imgconvert.c:207
Definition: libavcodec/avcodec.h:417
Definition: libavcodec/avcodec.h:273
Definition: libavcodec/avcodec.h:360
enum AVAudioServiceType audio_service_type
Type of service that the audio stream conveys.
Definition: libavcodec/avcodec.h:1936
const AVCodecDescriptor * avcodec_descriptor_next(const AVCodecDescriptor *prev)
Iterate over all codec descriptors known to libavcodec.
Definition: codec_desc.c:2576
Definition: libavcodec/avcodec.h:402
void av_picture_copy(AVPicture *dst, const AVPicture *src, enum AVPixelFormat pix_fmt, int width, int height)
Copy image src to dst.
Definition: avpicture.c:72
Definition: libavcodec/avcodec.h:425
int avcodec_fill_audio_frame(AVFrame *frame, int nb_channels, enum AVSampleFormat sample_fmt, const uint8_t *buf, int buf_size, int align)
Fill AVFrame audio data and linesize pointers.
Definition: libavcodec/utils.c:326
Definition: libavcodec/avcodec.h:605
Definition: libavcodec/avcodec.h:456
const AVClass * avcodec_get_class(void)
Get the AVClass for AVCodecContext.
Definition: libavcodec/options.c:239
Definition: libavcodec/avcodec.h:419
Definition: libavcodec/avcodec.h:363
void avcodec_align_dimensions(AVCodecContext *s, int *width, int *height)
Modify width and height values so that they will result in a memory buffer that is acceptable for the...
Definition: libavcodec/utils.c:311
Definition: libavcodec/avcodec.h:149
void av_fast_malloc(void *ptr, unsigned int *size, size_t min_size)
Allocate a buffer, reusing the given one if large enough.
Definition: libavcodec/utils.c:96
Public dictionary API.
the pkt_dts and pkt_pts fields in AVFrame will work as usual Restrictions on codec whose streams don t reset across will not work because their bitstreams cannot be decoded in parallel *The contents of buffers must not be read before as well as code calling get_buffer()
int bit_rate_tolerance
number of bits the bitstream is allowed to diverge from the reference.
Definition: libavcodec/avcodec.h:1200
Definition: libavcodec/avcodec.h:282
Definition: libavcodec/avcodec.h:625
enum AVPixelFormat avcodec_default_get_format(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
Definition: libavcodec/utils.c:920
the pkt_dts and pkt_pts fields in AVFrame will work as usual Restrictions on codec whose streams don t reset across will not work because their bitstreams cannot be decoded in parallel *The contents of buffers must not be read before as well as code calling up to before the decode process starts Call have update_thread_context() run it in the next thread.If the codec allocates writable tables in its init()
Definition: libavcodec/avcodec.h:144
int av_parser_change(AVCodecParserContext *s, AVCodecContext *avctx, uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size, int keyframe)
Definition: parser.c:169
int me_range
maximum motion estimation search range in subpel units If 0 then no limit.
Definition: libavcodec/avcodec.h:1614
Definition: libavcodec/avcodec.h:117
unsigned int avcodec_pix_fmt_to_codec_tag(enum AVPixelFormat pix_fmt)
Return a value representing the fourCC code associated to the pixel format pix_fmt, or 0 if no associated fourCC code can be found.
Definition: raw.c:199
Definition: libavcodec/avcodec.h:219
Definition: libavcodec/avcodec.h:340
Definition: libavcodec/avcodec.h:412
float b_quant_factor
qscale factor between IP and B-frames If > 0 then the last P-frame quantizer will be used (q= lastp_q...
Definition: libavcodec/avcodec.h:1394
int avcodec_default_get_buffer(AVCodecContext *avctx, AVFrame *frame)
Definition: libavcodec/utils.c:661
Definition: libavcodec/avcodec.h:473
Definition: libavcodec/avcodec.h:224
Definition: libavcodec/avcodec.h:383
Definition: libavcodec/avcodec.h:246
int avcodec_default_reget_buffer(AVCodecContext *s, AVFrame *pic)
Definition: libavcodec/utils.c:884
Definition: libavcodec/avcodec.h:293
Definition: libavcodec/avcodec.h:113
int av_packet_from_data(AVPacket *pkt, uint8_t *data, int size)
Initialize a reference-counted packet from av_malloc()ed data.
Definition: avpacket.c:136
Definition: libavcodec/avcodec.h:371
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
int scenechange_factor
Multiplied by qscale for each frame and added to scene_change_score.
Definition: libavcodec/avcodec.h:1793
Definition: libavcodec/avcodec.h:313
Definition: libavcodec/avcodec.h:208
int avcodec_encode_video2(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Encode a frame of video.
Definition: libavcodec/utils.c:1713
also ITU-R BT470M / ITU-R BT1700 625 PAL & SECAM
Definition: libavcodec/avcodec.h:616
static enum AVPixelFormat get_format(struct AVCodecContext *avctx, const enum AVPixelFormat *fmt)
Definition: vda_h264_dec.c:61
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
Definition: libavcodec/avcodec.h:325
Definition: libavcodec/avcodec.h:332
Definition: libavcodec/avcodec.h:124
Definition: libavcodec/avcodec.h:223
void av_register_hwaccel(AVHWAccel *hwaccel)
Register the hardware accelerator hwaccel.
Definition: libavcodec/utils.c:2925
Definition: libavcodec/avcodec.h:434
Definition: libavcodec/avcodec.h:652
Definition: libavcodec/avcodec.h:353
int mb_threshold
Macroblock threshold below which the user specified macroblock types will be used.
Definition: libavcodec/avcodec.h:1701
Definition: libavcodec/avcodec.h:428
Definition: libavcodec/avcodec.h:452
Definition: libavcodec/avcodec.h:169
Definition: libavcodec/avcodec.h:229
Definition: libavcodec/avcodec.h:274
Definition: libavcodec/avcodec.h:128
const AVCodecDescriptor * av_codec_get_codec_descriptor(const AVCodecContext *avctx)
const AVClass * avcodec_get_frame_class(void)
Get the AVClass for AVFrame.
Definition: libavcodec/options.c:266
Definition: libavcodec/avcodec.h:648
void avpicture_free(AVPicture *picture)
Free a picture previously allocated by avpicture_alloc().
Definition: avpicture.c:67
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
Definition: libavcodec/avcodec.h:4535
Definition: libavcodec/avcodec.h:110
Definition: libavcodec/avcodec.h:472
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
struct AVBitStreamFilterContext * next
Definition: libavcodec/avcodec.h:4531
enum AVChromaLocation chroma_sample_location
This defines the location of chroma samples.
Definition: libavcodec/avcodec.h:1843
Definition: libavcodec/avcodec.h:189
Definition: libavcodec/avcodec.h:318
Definition: libavcodec/avcodec.h:486
Definition: libavcodec/avcodec.h:400
Definition: libavcodec/avcodec.h:3102
Definition: libavcodec/avcodec.h:242
Definition: libavcodec/avcodec.h:209
Definition: libavcodec/avcodec.h:247
AVCodec * avcodec_find_encoder_by_name(const char *name)
Find a registered encoder with the specified name.
Definition: libavcodec/utils.c:2383
Definition: libavcodec/avcodec.h:127
void avcodec_align_dimensions2(AVCodecContext *s, int *width, int *height, int linesize_align[AV_NUM_DATA_POINTERS])
Modify width and height values so that they will result in a memory buffer that is acceptable for the...
Definition: libavcodec/utils.c:191
Definition: libavcodec/avcodec.h:415
Definition: libavcodec/avcodec.h:426
Definition: libavcodec/avcodec.h:212
Libavcodec version macros.
Definition: libavcodec/avcodec.h:103
Definition: libavcodec/avcodec.h:206
int avcodec_close(AVCodecContext *avctx)
Close a given AVCodecContext and free all the data associated with it (but not the AVCodecContext its...
Definition: libavcodec/utils.c:2305
int av_new_packet(AVPacket *pkt, int size)
Allocate the payload of a packet and initialize its fields with default values.
Definition: avpacket.c:73
AVRational pkt_timebase
Timebase in which pkt_dts/pts and AVPacket.dts/pts are.
Definition: libavcodec/avcodec.h:2793
no search, that is use 0,0 vector whenever one is needed
Definition: libavcodec/avcodec.h:576
Definition: libavcodec/avcodec.h:126
const uint64_t * channel_layouts
array of support channel layouts, or NULL if unknown. array is terminated by 0
Definition: libavcodec/avcodec.h:2878
AVHWAccel * av_hwaccel_next(AVHWAccel *hwaccel)
If hwaccel is NULL, returns the first registered hardware accelerator, if hwaccel is non-NULL...
Definition: libavcodec/utils.c:2934
Definition: dict.c:28
Definition: libavcodec/avcodec.h:111
Definition: libavcodec/avcodec.h:306
Definition: libavcodec/avcodec.h:106
Definition: libavcodec/avcodec.h:250
const AVCodecDefault * defaults
Private codec-specific defaults.
Definition: libavcodec/avcodec.h:2915
Definition: libavcodec/avcodec.h:626
Definition: libavcodec/avcodec.h:155
Definition: libavcodec/avcodec.h:179
void av_log_missing_feature(void *avc, const char *feature, int want_sample)
Definition: libavcodec/utils.c:2897
int av_get_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2690
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
Definition: libavcodec/avcodec.h:418
Definition: libavcodec/avcodec.h:3057
Definition: libavcodec/avcodec.h:918
Definition: libavcodec/avcodec.h:421
int avcodec_decode_video2(AVCodecContext *avctx, AVFrame *picture, int *got_picture_ptr, const AVPacket *avpkt)
Decode the video frame of size avpkt->size from avpkt->data into picture.
Definition: libavcodec/utils.c:1901
Definition: libavcodec/avcodec.h:390
Definition: libavcodec/avcodec.h:295
void * av_fast_realloc(void *ptr, unsigned int *size, size_t min_size)
Reallocate the given block if it is not large enough, otherwise do nothing.
Definition: libavcodec/utils.c:63
Definition: libavcodec/avcodec.h:378
An AV_PKT_DATA_PARAM_CHANGE side data packet is laid out as follows:
Definition: libavcodec/avcodec.h:936
Definition: libavcodec/avcodec.h:225
Definition: libavcodec/avcodec.h:217
Definition: libavcodec/avcodec.h:132
Definition: libavcodec/internal.h:112
AVCodec * av_codec_next(const AVCodec *c)
If c is NULL, returns the first registered codec, if c is non-NULL, returns the next registered codec...
Definition: libavcodec/utils.c:128
Definition: libavcodec/avcodec.h:239
int64_t pts_correction_last_pts
Number of incorrect DTS values so far.
Definition: libavcodec/avcodec.h:2811
int active_thread_type
Which multithreading methods are in use by the codec.
Definition: libavcodec/avcodec.h:2594
Definition: libavcodec/avcodec.h:181
int64_t convergence_duration
Time difference in AVStream->time_base units from the pts of this packet to the point at which the ou...
Definition: libavcodec/avcodec.h:1099
Definition: libavcodec/avcodec.h:385
void av_bitstream_filter_close(AVBitStreamFilterContext *bsf)
Definition: bitstream_filter.c:53
struct AVCodecParserContext AVCodecParserContext
preferred ID for decoding MPEG audio layer 1, 2 or 3
Definition: libavcodec/avcodec.h:382
AVBufferRef * buf
A reference to the reference-counted buffer where the packet data is stored.
Definition: libavcodec/avcodec.h:1034
struct AVSubtitle AVSubtitle
these buffered frames must be flushed immediately if a new input produces new the filter must not call request_frame to get more It must just process the frame or queue it The task of requesting more frames is left to the filter s request_frame method or the application If a filter has several the filter must be ready for frames arriving randomly on any input any filter with several inputs will most likely require some kind of queuing mechanism It is perfectly acceptable to have a limited queue and to drop frames when the inputs are too unbalanced request_frame This method is called when a frame is wanted on an output For an input
Definition: filter_design.txt:216
Definition: libavcodec/avcodec.h:118
Definition: libavcodec/avcodec.h:484
Definition: libavcodec/avcodec.h:609
enum AVMediaType avcodec_get_type(enum AVCodecID codec_id)
Get the type of the given codec.
Definition: libavcodec/utils.c:3082
Definition: libavcodec/avcodec.h:237
AVBitStreamFilter * av_bitstream_filter_next(AVBitStreamFilter *f)
Definition: bitstream_filter.c:28
Definition: libavcodec/avcodec.h:182
float i_quant_factor
qscale factor between P and I-frames If > 0 then the last p frame quantizer will be used (q= lastp_q*...
Definition: libavcodec/avcodec.h:1431
int av_packet_shrink_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int size)
Shrink the already allocated side data buffer.
Definition: avpacket.c:386
Definition: libavcodec/avcodec.h:129
Definition: libavcodec/avcodec.h:471
void * thread_opaque
thread opaque Can be used by execute() to store some per AVCodecContext stuff.
Definition: libavcodec/avcodec.h:2643
Definition: libavcodec/avcodec.h:445
Definition: libavcodec/avcodec.h:3883
Definition: libavcodec/avcodec.h:227
Definition: libavcodec/avcodec.h:464
struct AVBitStreamFilter AVBitStreamFilter
const AVCodecDescriptor * avcodec_descriptor_get(enum AVCodecID id)
Definition: codec_desc.c:2566
Definition: libavcodec/avcodec.h:352
Definition: libavcodec/avcodec.h:176
Definition: libavcodec/avcodec.h:481
Definition: libavcodec/avcodec.h:235
int props
Codec properties, a combination of AV_CODEC_PROP_* flags.
Definition: libavcodec/avcodec.h:524
int intra_dc_precision
precision of the intra DC coefficient - 8
Definition: libavcodec/avcodec.h:1708
int me_penalty_compensation
Definition: libavcodec/avcodec.h:1751
Definition: libavcodec/avcodec.h:137
AVFrame * avcodec_alloc_frame(void)
Allocate an AVFrame and set its fields to default values.
Definition: libavcodec/utils.c:951
int dtg_active_format
DTG active format information (additional aspect ratio information only used in DVB MPEG-2 transport ...
Definition: libavcodec/avcodec.h:1598
Definition: libavcodec/avcodec.h:579
Definition: libavcodec/avcodec.h:280
Definition: libavcodec/avcodec.h:141
Definition: libavcodec/avcodec.h:362
Definition: libavcodec/avcodec.h:483
Definition: libavcodec/avcodec.h:263
Definition: libavcodec/avcodec.h:188
struct RcOverride RcOverride
Definition: libavcodec/avcodec.h:156
audio channel layout utility functions
enum AVPixelFormat * pix_fmts
array of supported pixel formats, or NULL if unknown, array is terminated by -1
Definition: libavcodec/avcodec.h:2875
Definition: libavcodec/avcodec.h:228
Definition: libavcodec/avcodec.h:314
Definition: libavcodec/avcodec.h:461
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
Definition: libavcodec/avcodec.h:411
Definition: libavcodec/avcodec.h:178
int av_get_exact_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2619
Definition: libavcodec/avcodec.h:192
AVCodecContext * avcodec_alloc_context3(const AVCodec *codec)
Allocate an AVCodecContext and set its fields to default values.
Definition: libavcodec/options.c:148
int av_parser_parse2(AVCodecParserContext *s, AVCodecContext *avctx, uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size, int64_t pts, int64_t dts, int64_t pos)
Parse a packet.
Definition: parser.c:112
Definition: libavcodec/avcodec.h:252
Definition: libavcodec/avcodec.h:257
Definition: libavcodec/avcodec.h:268
AVBitStreamFilterContext * av_bitstream_filter_init(const char *name)
Definition: bitstream_filter.c:38
Definition: libavcodec/avcodec.h:256
Definition: libavcodec/avcodec.h:249
Definition: libavcodec/avcodec.h:203
Definition: libavcodec/avcodec.h:1121
float rc_max_available_vbv_use
Ratecontrol attempt to use, at maximum, of what can be used without an underflow. ...
Definition: libavcodec/avcodec.h:2219
float rc_min_vbv_overflow_use
Ratecontrol attempt to use, at least, times the amount needed to prevent a vbv overflow.
Definition: libavcodec/avcodec.h:2226
Definition: libavcodec/avcodec.h:146
int64_t convergence_duration
Time difference in stream time base units from the pts of this packet to the point at which the outpu...
Definition: libavcodec/avcodec.h:3815
Definition: libavcodec/avcodec.h:121
Definition: libavcodec/avcodec.h:302
Definition: libavcodec/avcodec.h:159
Definition: libavcodec/avcodec.h:108
void av_register_bitstream_filter(AVBitStreamFilter *bsf)
Definition: bitstream_filter.c:33
Definition: libavcodec/avcodec.h:321
float rc_qsquish
ratecontrol qmin qmax limiting method 0-> clipping, 1-> use a nice continuous function to limit qscal...
Definition: libavcodec/avcodec.h:2164
Definition: libavcodec/avcodec.h:152
Definition: libavcodec/avcodec.h:109
Definition: libavcodec/avcodec.h:107
const AVClass * avcodec_get_subtitle_rect_class(void)
Get the AVClass for AVSubtitleRect.
Definition: libavcodec/options.c:291
Definition: libavcodec/avcodec.h:284
Definition: libavcodec/avcodec.h:248
Definition: libavcodec/avcodec.h:394
int avcodec_decode_audio4(AVCodecContext *avctx, AVFrame *frame, int *got_frame_ptr, const AVPacket *avpkt)
Decode the audio frame of size avpkt->size from avpkt->data into frame.
Definition: libavcodec/utils.c:2033
Definition: libavcodec/avcodec.h:432
const AVProfile * profiles
array of recognized profiles, or NULL if unknown, array is terminated by {FF_PROFILE_UNKNOWN} ...
Definition: libavcodec/avcodec.h:2881
int64_t reordered_opaque
opaque 64bit number (generally a PTS) that will be reordered and output in AVFrame.reordered_opaque
Definition: libavcodec/avcodec.h:2477
int refcounted_frames
If non-zero, the decoded audio and video frames returned from avcodec_decode_video2() and avcodec_dec...
Definition: libavcodec/avcodec.h:2131
Definition: libavcodec/avcodec.h:290
Definition: libavcodec/avcodec.h:213
int ticks_per_frame
For some codecs, the time base is closer to the field rate than the frame rate.
Definition: libavcodec/avcodec.h:1262
int skip_top
Number of macroblock rows at the top which are skipped.
Definition: libavcodec/avcodec.h:1715
Definition: libavcodec/avcodec.h:449
void av_log_ask_for_sample(void *avc, const char *msg,...)
Definition: libavcodec/utils.c:2907
int last_predictor_count
amount of previous MV predictors (2a+1 x 2a+1 square)
Definition: libavcodec/avcodec.h:1560
Definition: libavcodec/avcodec.h:388
Definition: libavcodec/avcodec.h:241
Definition: libavcodec/avcodec.h:243
void avcodec_get_chroma_sub_sample(enum AVPixelFormat pix_fmt, int *h_shift, int *v_shift)
Utility function to access log2_chroma_w log2_chroma_h from the pixel format AVPixFmtDescriptor.
Definition: imgconvert.c:65
Note except for filters that can have queued request_frame does not push and as a the filter_frame method will be called and do the work Legacy the filter_frame method was split
Definition: filter_design.txt:263
Definition: libavcodec/avcodec.h:459
Definition: libavcodec/avcodec.h:266
Definition: libavcodec/avcodec.h:343
Definition: libavcodec/avcodec.h:300
Definition: libavcodec/avcodec.h:387
Definition: libavcodec/avcodec.h:125
Definition: libavcodec/avcodec.h:405
Definition: libavcodec/avcodec.h:220
const AVCodecDescriptor * avcodec_descriptor_get_by_name(const char *name)
Definition: codec_desc.c:2585
Definition: libavcodec/avcodec.h:265
Definition: libavcodec/avcodec.h:1119
Definition: libavcodec/avcodec.h:216
Definition: libavcodec/avcodec.h:3747
const char * avcodec_get_name(enum AVCodecID id)
Get the name of a codec.
Definition: libavcodec/utils.c:2416
int thread_count
thread count is used to decide how many independent tasks should be passed to execute() ...
Definition: libavcodec/avcodec.h:2575
Definition: libavcodec/avcodec.h:465
Definition: libavcodec/avcodec.h:254
Definition: libavcodec/avcodec.h:193
Definition: libavcodec/avcodec.h:143
Definition: libavcodec/avcodec.h:341
Definition: libavcodec/avcodec.h:145
Definition: libavcodec/avcodec.h:357
void avcodec_flush_buffers(AVCodecContext *avctx)
Flush buffers, should be called when seeking or when switching to a different stream.
Definition: libavcodec/utils.c:2608
Definition: libavcodec/avcodec.h:304
Definition: libavcodec/avcodec.h:147
FAKE codec to indicate a MPEG-4 Systems stream (only used by libavformat)
Definition: libavcodec/avcodec.h:494
enum AVPixelFormat avcodec_find_best_pix_fmt_of_2(enum AVPixelFormat dst_pix_fmt1, enum AVPixelFormat dst_pix_fmt2, enum AVPixelFormat src_pix_fmt, int has_alpha, int *loss_ptr)
Find the best pixel format to convert to given a certain source pixel format and a selection of two d...
Definition: imgconvert.c:218
attribute_deprecated enum AVPixelFormat avcodec_find_best_pix_fmt2(enum AVPixelFormat dst_pix_fmt1, enum AVPixelFormat dst_pix_fmt2, enum AVPixelFormat src_pix_fmt, int has_alpha, int *loss_ptr)
Definition: imgconvert.c:257
Definition: libavcodec/avcodec.h:261
const char * av_get_profile_name(const AVCodec *codec, int profile)
Return a name for the specified profile, if available.
Definition: libavcodec/utils.c:2567
int attribute_align_arg avcodec_encode_video(AVCodecContext *avctx, uint8_t *buf, int buf_size, const AVFrame *pict)
Definition: libavcodec/utils.c:1678
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
int pts_dts_delta
Presentation delay of current frame in units of AVCodecContext.time_base.
Definition: libavcodec/avcodec.h:3856
Definition: libavcodec/avcodec.h:289
int(* execute2)(struct AVCodecContext *c, int(*func)(struct AVCodecContext *c2, void *arg, int jobnr, int threadnr), void *arg2, int *ret, int count)
The codec may call this to execute several independent things.
Definition: libavcodec/avcodec.h:2635
Definition: libavcodec/avcodec.h:578
const char * long_name
Descriptive name for the codec, meant to be more human readable than name.
Definition: libavcodec/avcodec.h:2866
Definition: libavcodec/avcodec.h:654
Definition: libavcodec/avcodec.h:139
Definition: libavcodec/avcodec.h:485
int av_bitstream_filter_filter(AVBitStreamFilterContext *bsfc, AVCodecContext *avctx, const char *args, uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size, int keyframe)
Definition: bitstream_filter.c:61
Definition: libavcodec/avcodec.h:244
int avcodec_default_get_buffer2(AVCodecContext *s, AVFrame *frame, int flags)
The default callback for AVCodecContext.get_buffer2().
Definition: libavcodec/utils.c:586
Definition: libavcodec/avcodec.h:133
also ITU-R BT1361 / IEC 61966-2-4 xvYCC709 / SMPTE RP177 Annex B
Definition: libavcodec/avcodec.h:624
Definition: libavcodec/avcodec.h:218
void(* rtp_callback)(struct AVCodecContext *avctx, void *data, int size, int mb_nb)
Definition: libavcodec/avcodec.h:2328
int me_threshold
Motion estimation threshold below which no motion estimation is performed, but instead the user speci...
Definition: libavcodec/avcodec.h:1694
const AVRational * supported_framerates
array of supported framerates, or NULL if any, array is terminated by {0,0}
Definition: libavcodec/avcodec.h:2874
A list of zero terminated key/value strings.
Definition: libavcodec/avcodec.h:984
int(* end_frame)(AVCodecContext *avctx)
Called at the end of each frame or field picture.
Definition: libavcodec/avcodec.h:3024
also ITU-R BT601-6 625 / ITU-R BT1358 625 / ITU-R BT1700 625 PAL & SECAM
Definition: libavcodec/avcodec.h:606
Definition: libavcodec/internal.h:56
enum AVCodecID av_get_pcm_codec(enum AVSampleFormat fmt, int be)
Return the PCM codec associated with a sample format.
Definition: libavcodec/utils.c:2669
Definition: libavcodec/avcodec.h:222
Definition: libavcodec/avcodec.h:168
Definition: libavcodec/avcodec.h:438
Definition: libavcodec/avcodec.h:467
enum AVDiscard skip_idct
Skip IDCT/dequantization for selected frames.
Definition: libavcodec/avcodec.h:2742
Definition: libavcodec/avcodec.h:120
unsigned avcodec_get_edge_width(void)
Return the amount of padding in pixels which the get_buffer callback must provide around the edge of ...
Definition: libavcodec/utils.c:172
int frame_bits
number of bits used for the previously encoded frame
Definition: libavcodec/avcodec.h:2352
Plain text, the text field must be set by the decoder and is authoritative.
Definition: libavcodec/avcodec.h:3065
the pkt_dts and pkt_pts fields in AVFrame will work as usual Restrictions on codec whose streams don t reset across will not work because their bitstreams cannot be decoded in parallel *The contents of buffers must not be read before as well as code calling up to before the decode process starts Call have add an init_thread_copy() which re-allocates them for other threads.Add CODEC_CAP_FRAME_THREADS to the codec capabilities.There will be very little speed gain at this point but it should work.If there are inter-frame dependencies
Definition: libavcodec/avcodec.h:140
Definition: libavcodec/avcodec.h:221
AVCodec * avcodec_find_decoder(enum AVCodecID id)
Find a registered decoder with a matching codec ID.
Definition: libavcodec/utils.c:2397
Definition: libavcodec/avcodec.h:482
Definition: libavcodec/avcodec.h:384
Definition: libavcodec/avcodec.h:294
int cur_frame_start_index
Definition: libavcodec/avcodec.h:3775
Definition: libavcodec/avcodec.h:447
void avsubtitle_free(AVSubtitle *sub)
Free all allocated data in the given subtitle struct.
Definition: libavcodec/utils.c:2274
Definition: libavcodec/avcodec.h:305
Definition: libavcodec/avcodec.h:309
Definition: libavcodec/avcodec.h:674
Definition: libavcodec/avcodec.h:457
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
Definition: libavcodec/avcodec.h:171
Used by Dirac / VC-2 and H.264 FRext, see ITU-T SG16.
Definition: libavcodec/avcodec.h:630
Definition: libavcodec/avcodec.h:278
Definition: libavcodec/avcodec.h:422
float spatial_cplx_masking
spatial complexity masking (0-> disabled)
Definition: libavcodec/avcodec.h:1459
Definition: libavcodec/avcodec.h:319
Data found in BlockAdditional element of matroska container.
Definition: libavcodec/avcodec.h:1003
enum AVPixelFormat avcodec_find_best_pix_fmt_of_list(enum AVPixelFormat *pix_fmt_list, enum AVPixelFormat src_pix_fmt, int has_alpha, int *loss_ptr)
Find the best pixel format to convert to given a certain source pixel format.
Definition: imgconvert.c:264
Definition: libavcodec/avcodec.h:253
unsigned int av_xiphlacing(unsigned char *s, unsigned int v)
Encode extradata length to a buffer.
Definition: libavcodec/utils.c:2875
struct AVBitStreamFilter * filter
Definition: libavcodec/avcodec.h:4529
Definition: libavcodec/avcodec.h:163
Definition: libavcodec/avcodec.h:487
void avcodec_get_frame_defaults(AVFrame *frame)
Set the fields of the given AVFrame to default values.
Definition: libavcodec/utils.c:927
Definition: libavcodec/avcodec.h:196
int(* func)(AVBPrint *dst, const char *in, const char *arg)
Definition: libavcodec/jacosubdec.c:70
Definition: libavcodec/avcodec.h:324
int avpicture_alloc(AVPicture *picture, enum AVPixelFormat pix_fmt, int width, int height)
Allocate memory for a picture.
Definition: avpicture.c:54
Definition: libavcodec/avcodec.h:403
float rc_buffer_aggressivity
Definition: libavcodec/avcodec.h:2205
Definition: libavcodec/avcodec.h:183
Recommmends skipping the specified number of samples.
Definition: libavcodec/avcodec.h:968
struct AVCodecDescriptor AVCodecDescriptor
This struct describes the properties of a single codec described by an AVCodecID. ...
Definition: libavcodec/avcodec.h:258
Definition: libavcodec/avcodec.h:172
Definition: libavcodec/avcodec.h:267
Definition: libavcodec/avcodec.h:270
discard useless packets like 0 size packets in avi
Definition: libavcodec/avcodec.h:595
refcounted data buffer API
Definition: libavcodec/avcodec.h:311
Definition: libavcodec/avcodec.h:262
Definition: libavcodec/avcodec.h:336
const char * name
Name of the codec described by this descriptor.
Definition: libavcodec/avcodec.h:516
int avcodec_open2(AVCodecContext *avctx, const AVCodec *codec, AVDictionary **options)
Initialize the AVCodecContext to use the given AVCodec.
Definition: libavcodec/utils.c:1034
Definition: libavcodec/avcodec.h:185
Definition: libavcodec/avcodec.h:381
Definition: libavcodec/avcodec.h:451
Definition: libavcodec/avcodec.h:420
Definition: libavcodec/avcodec.h:122
Definition: libavcodec/avcodec.h:372
int av_get_audio_frame_duration(AVCodecContext *avctx, int frame_bytes)
Return audio frame duration.
Definition: libavcodec/utils.c:2708
struct AVBitStreamFilterContext AVBitStreamFilterContext
float qcompress
amount of qscale change between easy & hard scenes (0.0-1.0)
Definition: libavcodec/avcodec.h:2134
Definition: libavcodec/avcodec.h:322
Definition: libavcodec/avcodec.h:281
Definition: libavcodec/avcodec.h:286
This struct describes the properties of a single codec described by an AVCodecID. ...
Definition: libavcodec/avcodec.h:508
Definition: libavcodec/avcodec.h:623
Definition: libavcodec/avcodec.h:231
Definition: libavcodec/avcodec.h:1118
struct AVSubtitleRect AVSubtitleRect
Definition: libavcodec/avcodec.h:167
Definition: libavcodec/avcodec.h:230
Definition: libavcodec/avcodec.h:431
Definition: libavcodec/avcodec.h:468
int global_quality
Global quality for codecs which cannot change it per frame.
Definition: libavcodec/avcodec.h:1208
int skip_bottom
Number of macroblock rows at the bottom which are skipped.
Definition: libavcodec/avcodec.h:1722
AVCodec * avcodec_find_decoder_by_name(const char *name)
Find a registered decoder with the specified name.
Definition: libavcodec/utils.c:2402
float temporal_cplx_masking
temporary complexity masking (0-> disabled)
Definition: libavcodec/avcodec.h:1452
Definition: libavcodec/avcodec.h:190
also ITU-R BT601-6 525 / ITU-R BT1358 525 / ITU-R BT1700 NTSC
Definition: libavcodec/avcodec.h:607
Definition: libavcodec/avcodec.h:191
Definition: libavcodec/avcodec.h:323
Definition: libavcodec/avcodec.h:1120
Definition: libavcodec/avcodec.h:199
Definition: libavcodec/avcodec.h:288
Definition: libavcodec/avcodec.h:161
Definition: libavcodec/avcodec.h:292
Definition: libavcodec/avcodec.h:320
Definition: libavcodec/avcodec.h:205
int gop_size
the number of pictures in a group of pictures, or 0 for intra_only
Definition: libavcodec/avcodec.h:1321
uint8_t max_lowres
maximum value for lowres supported by the decoder
Definition: libavcodec/avcodec.h:2879
Definition: libavcodec/avcodec.h:396
Definition: libavcodec/avcodec.h:1122
AVPacket * pkt
Current packet as passed into the decoder, to avoid having to pass the packet into every function...
Definition: libavcodec/avcodec.h:2776
Definition: libavcodec/avcodec.h:136
Definition: libavcodec/avcodec.h:460
Definition: libavcodec/avcodec.h:408
static int op(uint8_t **dst, const uint8_t *dst_end, GetByteContext *gb, int pixel, int count, int *x, int width, int linesize)
Perform decode operation.
Definition: libavcodec/anm.c:76
An AV_PKT_DATA_JP_DUALMONO side data packet indicates that the packet may contain "dual mono" audio s...
Definition: libavcodec/avcodec.h:978
Definition: libavcodec/avcodec.h:174
Definition: libavcodec/avcodec.h:469
int attribute_align_arg avcodec_encode_audio(AVCodecContext *avctx, uint8_t *buf, int buf_size, const short *samples)
Definition: libavcodec/utils.c:1600
Definition: libavcodec/avcodec.h:283
AVRational av_codec_get_pkt_timebase(const AVCodecContext *avctx)
Definition: libavcodec/avcodec.h:310
rational numbers
Definition: libavcodec/avcodec.h:264
Definition: libavcodec/avcodec.h:350
Definition: libavcodec/avcodec.h:618
void av_fast_padded_mallocz(void *ptr, unsigned int *size, size_t min_size)
Same behaviour av_fast_padded_malloc except that buffer will always be 0-initialized after call...
Definition: libavcodec/utils.c:113
int(* encode_sub)(AVCodecContext *, uint8_t *buf, int buf_size, const struct AVSubtitle *sub)
Definition: libavcodec/avcodec.h:2923
void av_codec_set_codec_descriptor(AVCodecContext *avctx, const AVCodecDescriptor *desc)
Definition: libavcodec/avcodec.h:299
Definition: libavcodec/avcodec.h:233
these buffered frames must be flushed immediately if a new input produces new output(Example:frame rate-doubling filter:filter_frame must(1) flush the second copy of the previous frame, if it is still there,(2) push the first copy of the incoming frame,(3) keep the second copy for later.) If the input frame is not enough to produce output
int workaround_bugs
Work around bugs in encoders which sometimes cannot be detected automatically.
Definition: libavcodec/avcodec.h:2374
Definition: libavcodec/avcodec.h:291
int av_grow_packet(AVPacket *pkt, int grow_by)
Increase packet size, correctly zeroing padding.
Definition: avpacket.c:105
void av_init_packet(AVPacket *pkt)
Initialize optional fields of a packet with default values.
Definition: avpacket.c:56
enum AVDiscard skip_loop_filter
Skip loop filtering for selected frames.
Definition: libavcodec/avcodec.h:2735
int thread_safe_callbacks
Set by the client if its custom get_buffer() callback can be called synchronously from another thread...
Definition: libavcodec/avcodec.h:2604
int avcodec_get_context_defaults3(AVCodecContext *s, const AVCodec *codec)
Set the fields of the given AVCodecContext to default values corresponding to the given codec (defaul...
Definition: libavcodec/options.c:97
Definition: libavcodec/avcodec.h:207
Definition: libavcodec/avcodec.h:287
Definition: libavcodec/avcodec.h:238
void avcodec_string(char *buf, int buf_size, AVCodecContext *enc, int encode)
Definition: libavcodec/utils.c:2456
Definition: libavcodec/avcodec.h:150
Definition: libavcodec/avcodec.h:165
Definition: libavcodec/avcodec.h:151
Definition: libavcodec/avcodec.h:114
FAKE codec to indicate a raw MPEG-2 TS stream (only used by libavformat)
Definition: libavcodec/avcodec.h:492
Formatted text, the ass field must be set by the decoder and is authoritative.
Definition: libavcodec/avcodec.h:3071
Definition: libavcodec/avcodec.h:119
Definition: libavcodec/avcodec.h:450
pixel format definitions
Definition: libavcodec/avcodec.h:138
Definition: libavcodec/avcodec.h:160
Definition: libavcodec/avcodec.h:338
int error_rate
Simulates errors in the bitstream to test error concealment.
Definition: libavcodec/avcodec.h:2767
const int * supported_samplerates
array of supported audio samplerates, or NULL if unknown, array is terminated by 0 ...
Definition: libavcodec/avcodec.h:2876
Definition: libavcodec/avcodec.h:285
int av_picture_pad(AVPicture *dst, const AVPicture *src, int height, int width, enum AVPixelFormat pix_fmt, int padtop, int padbottom, int padleft, int padright, int *color)
Pad image.
Definition: imgconvert.c:415
Definition: libavcodec/avcodec.h:214
int avpicture_get_size(enum AVPixelFormat pix_fmt, int width, int height)
Calculate the size in bytes that a picture of the given width and height would occupy if stored in th...
Definition: avpicture.c:49
unsigned avcodec_version(void)
Return the LIBAVCODEC_VERSION_INT constant.
Definition: libavcodec/utils.c:2580
Definition: libavcodec/avcodec.h:427
Definition: libavcodec/avcodec.h:153
Definition: libavcodec/avcodec.h:440
Definition: libavcodec/avcodec.h:389
Definition: libavcodec/avcodec.h:354
int mv0_threshold
Note: Value depends upon the compare function used for fullpel ME.
Definition: libavcodec/avcodec.h:1801
Definition: libavcodec/avcodec.h:433
void avcodec_free_frame(AVFrame **frame)
Free the frame and any dynamically allocated objects in it, e.g.
Definition: libavcodec/utils.c:964
Definition: libavcodec/avcodec.h:446
static int get_buffer2(AVCodecContext *avctx, AVFrame *pic, int flag)
Definition: vda_h264_dec.c:79
Definition: libavcodec/avcodec.h:316
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
Definition: libavcodec/avcodec.h:175
void avcodec_register(AVCodec *codec)
Register the codec codec and initialize libavcodec.
Definition: libavcodec/utils.c:158
int repeat_pict
This field is used for proper frame duration computation in lavf.
Definition: libavcodec/avcodec.h:3765
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
also ITU-R BT1361 / IEC 61966-2-4 / SMPTE RP177 Annex B
Definition: libavcodec/avcodec.h:603
int64_t pts_correction_num_faulty_pts
Current statistics for PTS correction.
Definition: libavcodec/avcodec.h:2809
void(* init_static_data)(struct AVCodec *codec)
Initialize codec static data, called from avcodec_register().
Definition: libavcodec/avcodec.h:2920
uint8_t * av_packet_get_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int *size)
Get side information from packet.
Definition: avpacket.c:289
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
Definition: libavcodec/avcodec.h:307
Definition: libavcodec/avcodec.h:1117
Definition: libavcodec/avcodec.h:200
enum AVSampleFormat * sample_fmts
array of supported sample formats, or NULL if unknown, array is terminated by -1
Definition: libavcodec/avcodec.h:2877
Definition: libavcodec/avcodec.h:116
Definition: libavcodec/avcodec.h:436
also ITU-R BT601-6 625 / ITU-R BT1358 625 / ITU-R BT1700 625 PAL & SECAM / IEC 61966-2-4 xvYCC601 ...
Definition: libavcodec/avcodec.h:627
int me_method
Motion estimation algorithm used for video coding.
Definition: libavcodec/avcodec.h:1339
uint8_t * av_packet_new_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int size)
Allocate new information of a packet.
Definition: avpacket.c:264
Note except for filters that can have queued request_frame does not push and as a the filter_frame method will be called and do the work Legacy the filter_frame method was it was made of start_frame
Definition: filter_design.txt:263
Definition: libavcodec/avcodec.h:441
Definition: libavcodec/avcodec.h:226
Definition: libavcodec/avcodec.h:416
Definition: libavcodec/avcodec.h:636
Definition: libavcodec/avcodec.h:195
Definition: libavcodec/avcodec.h:423
Definition: libavcodec/avcodec.h:131
uint64_t request_channel_layout
Request decoder to use this channel layout if it can (0 for default)
Definition: libavcodec/avcodec.h:1929
Definition: libavcodec/avcodec.h:3076
Definition: libavcodec/avcodec.h:345
Definition: libavcodec/avcodec.h:180
Definition: libavcodec/avcodec.h:135
int avcodec_encode_subtitle(AVCodecContext *avctx, uint8_t *buf, int buf_size, const AVSubtitle *sub)
Definition: libavcodec/utils.c:1790
int key_frame
Set by parser to 1 for key frames and 0 for non-key frames.
Definition: libavcodec/avcodec.h:3796
Definition: libavcodec/avcodec.h:435
Definition: libavcodec/avcodec.h:148
int strict_std_compliance
strictly follow the standard (MPEG4, ...).
Definition: libavcodec/avcodec.h:2404
int dts_sync_point
Synchronization point for start of timestamp generation.
Definition: libavcodec/avcodec.h:3827
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
Definition: libavcodec/avcodec.h:386
A dummy ID pointing at the start of subtitle codecs.
Definition: libavcodec/avcodec.h:455
void * opaque
Private data of the user, can be used to carry app specific stuff.
Definition: libavcodec/avcodec.h:1185
Definition: libavcodec/avcodec.h:414
Definition: libavcodec/avcodec.h:377
Definition: libavcodec/avcodec.h:577
int avcodec_default_execute(AVCodecContext *c, int(*func)(AVCodecContext *c2, void *arg2), void *arg, int *ret, int count, int size)
Definition: libavcodec/utils.c:890
Definition: libavcodec/avcodec.h:272
Definition: libavcodec/avcodec.h:308
Definition: libavcodec/avcodec.h:201
Definition: libavcodec/avcodec.h:269
Definition: libavcodec/avcodec.h:404
Definition: libavcodec/avcodec.h:615
uint8_t * subtitle_header
Header containing style information for text subtitles.
Definition: libavcodec/avcodec.h:2759
Definition: libavcodec/avcodec.h:392
Definition: libavcodec/avcodec.h:204
Definition: libavcodec/avcodec.h:339
Definition: libavcodec/avcodec.h:211
Definition: libavcodec/avcodec.h:629
also ITU-R BT601-6 525 / ITU-R BT1358 525 / ITU-R BT1700 NTSC / functionally identical to above ...
Definition: libavcodec/avcodec.h:628
Generated on Mon Nov 18 2024 06:51:52 for FFmpeg by
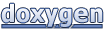