FFmpeg
|
#include "config.h"
#include <stdint.h>
#include <stdio.h>
#include <signal.h>
#include <pthread.h>
#include "cmdutils.h"
#include "libavformat/avformat.h"
#include "libavformat/avio.h"
#include "libavcodec/avcodec.h"
#include "libavfilter/avfilter.h"
#include "libavutil/avutil.h"
#include "libavutil/dict.h"
#include "libavutil/eval.h"
#include "libavutil/fifo.h"
#include "libavutil/pixfmt.h"
#include "libavutil/rational.h"
#include "libswresample/swresample.h"
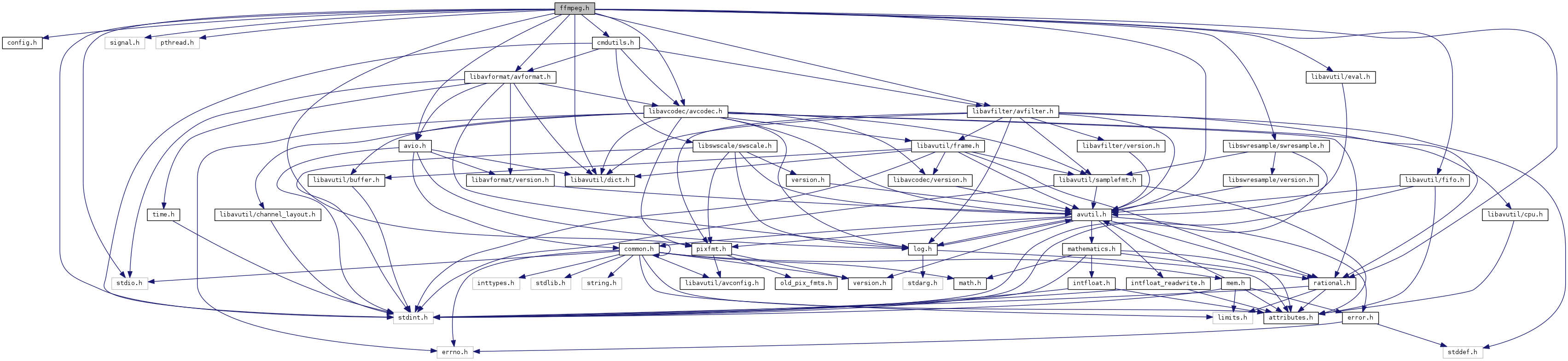
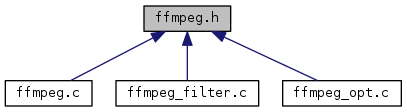
Go to the source code of this file.
Data Structures | |
struct | StreamMap |
struct | AudioChannelMap |
struct | OptionsContext |
struct | InputFilter |
struct | OutputFilter |
struct | FilterGraph |
struct | InputStream |
struct | InputStream::sub2video |
struct | InputFile |
struct | OutputStream |
struct | OutputFile |
Macros | |
#define | VSYNC_AUTO -1 |
#define | VSYNC_PASSTHROUGH 0 |
#define | VSYNC_CFR 1 |
#define | VSYNC_VFR 2 |
#define | VSYNC_DROP 0xff |
#define | MAX_STREAMS 1024 /* arbitrary sanity check value */ |
Typedefs | |
typedef struct StreamMap | StreamMap |
typedef struct OptionsContext | OptionsContext |
typedef struct InputFilter | InputFilter |
typedef struct OutputFilter | OutputFilter |
typedef struct FilterGraph | FilterGraph |
typedef struct InputStream | InputStream |
typedef struct InputFile | InputFile |
typedef struct OutputStream | OutputStream |
typedef struct OutputFile | OutputFile |
Enumerations | |
enum | forced_keyframes_const { FKF_N, FKF_N_FORCED, FKF_PREV_FORCED_N, FKF_PREV_FORCED_T, FKF_T, FKF_NB } |
Functions | |
void | term_init (void) |
void | term_exit (void) |
void | reset_options (OptionsContext *o, int is_input) |
void | show_usage (void) |
void | opt_output_file (void *optctx, const char *filename) |
void | assert_avoptions (AVDictionary *m) |
int | guess_input_channel_layout (InputStream *ist) |
enum AVPixelFormat | choose_pixel_fmt (AVStream *st, AVCodec *codec, enum AVPixelFormat target) |
void | choose_sample_fmt (AVStream *st, AVCodec *codec) |
int | configure_filtergraph (FilterGraph *fg) |
int | configure_output_filter (FilterGraph *fg, OutputFilter *ofilter, AVFilterInOut *out) |
int | ist_in_filtergraph (FilterGraph *fg, InputStream *ist) |
FilterGraph * | init_simple_filtergraph (InputStream *ist, OutputStream *ost) |
int | ffmpeg_parse_options (int argc, char **argv) |
Variables | |
const char *const | forced_keyframes_const_names [] |
InputStream ** | input_streams |
int | nb_input_streams |
InputFile ** | input_files |
int | nb_input_files |
OutputStream ** | output_streams |
int | nb_output_streams |
OutputFile ** | output_files |
int | nb_output_files |
FilterGraph ** | filtergraphs |
int | nb_filtergraphs |
char * | vstats_filename |
float | audio_drift_threshold |
float | dts_delta_threshold |
float | dts_error_threshold |
int | audio_volume |
int | audio_sync_method |
int | video_sync_method |
int | do_benchmark |
int | do_benchmark_all |
int | do_deinterlace |
int | do_hex_dump |
int | do_pkt_dump |
int | copy_ts |
int | copy_tb |
int | debug_ts |
int | exit_on_error |
int | print_stats |
int | qp_hist |
int | stdin_interaction |
int | frame_bits_per_raw_sample |
AVIOContext * | progress_avio |
const AVIOInterruptCB | int_cb |
const OptionDef | options [] |
Macro Definition Documentation
Definition at line 56 of file ffmpeg.h.
Referenced by opt_streamid().
#define VSYNC_AUTO -1 |
Definition at line 50 of file ffmpeg.h.
Referenced by do_video_out(), opt_vsync(), and transcode_init().
#define VSYNC_CFR 1 |
Definition at line 52 of file ffmpeg.h.
Referenced by do_video_out(), opt_vsync(), and transcode_init().
#define VSYNC_DROP 0xff |
Definition at line 54 of file ffmpeg.h.
Referenced by do_video_out(), opt_vsync(), and write_frame().
#define VSYNC_PASSTHROUGH 0 |
Definition at line 51 of file ffmpeg.h.
Referenced by do_video_out(), opt_vsync(), and transcode_init().
#define VSYNC_VFR 2 |
Definition at line 53 of file ffmpeg.h.
Referenced by do_video_out(), and opt_vsync().
Typedef Documentation
typedef struct FilterGraph FilterGraph |
typedef struct InputFilter InputFilter |
typedef struct InputStream InputStream |
typedef struct OptionsContext OptionsContext |
typedef struct OutputFile OutputFile |
typedef struct OutputFilter OutputFilter |
typedef struct OutputStream OutputStream |
Enumeration Type Documentation
Function Documentation
void assert_avoptions | ( | AVDictionary * | m | ) |
Definition at line 510 of file ffmpeg.c.
Referenced by init_input_stream(), open_input_file(), and transcode_init().
enum AVPixelFormat choose_pixel_fmt | ( | AVStream * | st, |
AVCodec * | codec, | ||
enum AVPixelFormat | target | ||
) |
Definition at line 38 of file ffmpeg_filter.c.
Referenced by choose_pix_fmts(), and read_ffserver_streams().
Definition at line 71 of file ffmpeg_filter.c.
Referenced by read_ffserver_streams().
int configure_filtergraph | ( | FilterGraph * | fg | ) |
Definition at line 719 of file ffmpeg_filter.c.
Referenced by configure_complex_filters(), decode_audio(), decode_video(), and transcode_init().
int configure_output_filter | ( | FilterGraph * | fg, |
OutputFilter * | ofilter, | ||
AVFilterInOut * | out | ||
) |
Definition at line 483 of file ffmpeg_filter.c.
Referenced by configure_filtergraph(), and init_output_filter().
int ffmpeg_parse_options | ( | int | argc, |
char ** | argv | ||
) |
Definition at line 2490 of file ffmpeg_opt.c.
Referenced by main().
int guess_input_channel_layout | ( | InputStream * | ist | ) |
Definition at line 1464 of file ffmpeg.c.
Referenced by add_input_streams(), and decode_audio().
FilterGraph* init_simple_filtergraph | ( | InputStream * | ist, |
OutputStream * | ost | ||
) |
Referenced by transcode_init().
int ist_in_filtergraph | ( | FilterGraph * | fg, |
InputStream * | ist | ||
) |
Definition at line 800 of file ffmpeg_filter.c.
Referenced by decode_audio(), and decode_video().
void reset_options | ( | OptionsContext * | o, |
int | is_input | ||
) |
Definition at line 2440 of file ffmpeg_opt.c.
Referenced by main(), and show_help_default().
Definition at line 302 of file ffmpeg.c.
Referenced by assert_file_overwrite(), sigterm_handler(), term_init(), and transcode().
Definition at line 324 of file ffmpeg.c.
Referenced by assert_file_overwrite(), and main().
Variable Documentation
float audio_drift_threshold |
Definition at line 67 of file ffmpeg_opt.c.
Referenced by configure_input_audio_filter().
int audio_sync_method |
Definition at line 72 of file ffmpeg_opt.c.
Referenced by configure_input_audio_filter(), do_audio_out(), and write_frame().
int audio_volume |
Definition at line 71 of file ffmpeg_opt.c.
Referenced by configure_input_audio_filter(), configure_output_audio_filter(), and transcode_init().
int copy_tb |
Definition at line 80 of file ffmpeg_opt.c.
Referenced by transcode_init().
int copy_ts |
Definition at line 79 of file ffmpeg_opt.c.
Referenced by open_input_file(), and process_input().
int debug_ts |
Definition at line 81 of file ffmpeg_opt.c.
Referenced by decode_video(), do_audio_out(), do_video_out(), process_input(), and write_frame().
int do_benchmark |
Definition at line 75 of file ffmpeg_opt.c.
Referenced by exit_program(), and main().
int do_benchmark_all |
Definition at line 76 of file ffmpeg_opt.c.
Referenced by update_benchmark().
int do_deinterlace |
Definition at line 74 of file ffmpeg_opt.c.
Referenced by configure_input_video_filter().
int do_hex_dump |
Definition at line 77 of file ffmpeg_opt.c.
Referenced by check_keyboard_interaction(), and process_input().
int do_pkt_dump |
Definition at line 78 of file ffmpeg_opt.c.
Referenced by check_keyboard_interaction(), and process_input().
float dts_delta_threshold |
Definition at line 68 of file ffmpeg_opt.c.
Referenced by process_input().
float dts_error_threshold |
Definition at line 69 of file ffmpeg_opt.c.
Referenced by do_video_out(), and process_input().
int exit_on_error |
Definition at line 82 of file ffmpeg_opt.c.
Referenced by do_subtitle_out(), process_input(), and write_frame().
FilterGraph** filtergraphs |
Definition at line 155 of file ffmpeg.c.
Referenced by configure_complex_filters(), DEF_CHOOSE_FORMAT(), open_output_file(), opt_filter_complex(), and opt_filter_complex_script().
const char* const forced_keyframes_const_names[] |
Definition at line 111 of file ffmpeg.c.
Referenced by transcode_init().
int frame_bits_per_raw_sample |
Definition at line 86 of file ffmpeg_opt.c.
Referenced by new_video_stream(), and transcode_init().
InputFile** input_files |
Definition at line 147 of file ffmpeg.c.
Referenced by configure_input_video_filter(), init_input_filter(), open_input_file(), open_output_file(), opt_map(), opt_map_channel(), opt_target(), and sub2video_prepare().
InputStream** input_streams |
Definition at line 145 of file ffmpeg.c.
Referenced by add_input_streams(), init_input_filter(), new_audio_stream(), new_output_stream(), open_input_file(), and open_output_file().
const AVIOInterruptCB int_cb |
Definition at line 422 of file ffmpeg.c.
Referenced by dump_attachment(), get_preset_file_2(), open_input_file(), open_output_file(), opt_progress(), read_ffserver_streams(), transcode_init(), and url_alloc_for_protocol().
int nb_filtergraphs |
Definition at line 156 of file ffmpeg.c.
Referenced by check_keyboard_interaction(), configure_complex_filters(), decode_audio(), decode_video(), DEF_CHOOSE_FORMAT(), exit_program(), open_output_file(), opt_filter_complex(), opt_filter_complex_script(), and transcode_init().
int nb_input_files |
Definition at line 148 of file ffmpeg.c.
Referenced by add_input_streams(), dump_attachment(), exit_program(), free_input_threads(), get_input_packet(), init_input_filter(), init_input_threads(), main(), open_input_file(), open_output_file(), opt_map(), opt_map_channel(), opt_target(), reset_eagain(), and transcode_init().
int nb_input_streams |
Definition at line 146 of file ffmpeg.c.
Referenced by add_input_streams(), check_keyboard_interaction(), exit_program(), init_input_filter(), open_input_file(), open_output_file(), transcode(), and transcode_init().
int nb_output_files |
Definition at line 153 of file ffmpeg.c.
Referenced by exit_program(), get_ost_filters(), main(), new_output_stream(), open_output_file(), print_sdp(), transcode(), and transcode_init().
int nb_output_streams |
Definition at line 151 of file ffmpeg.c.
Referenced by check_keyboard_interaction(), choose_output(), exit_program(), flush_encoders(), got_eagain(), need_output(), new_output_stream(), open_output_file(), output_packet(), print_report(), process_input(), reap_filters(), reset_eagain(), transcode(), transcode_init(), and transcode_subtitles().
static const OptionDef options |
Definition at line 4697 of file ffserver.c.
Referenced by amr_decode_fix_avctx(), audio_read_close(), av_opt_freep_ranges(), avfilter_init_str(), decode_flush(), ff_rtsp_open_transport_ctx(), ff_samples_to_time_base(), ffmpeg_parse_options(), g726_reset(), main(), open_slave(), opt_audio_codec(), opt_audio_filters(), opt_audio_frames(), opt_audio_qscale(), opt_channel_layout(), opt_data_codec(), opt_data_frames(), opt_old2new(), opt_qscale(), opt_recording_timestamp(), opt_subtitle_codec(), opt_target(), opt_timecode(), opt_video_codec(), opt_video_filters(), opt_video_frames(), pp_get_mode_by_name_and_quality(), query_codec(), read_ffserver_streams(), show_codec_opts(), show_format_opts(), show_help_default(), uninit(), and uninit_options().
OutputFile** output_files |
Definition at line 152 of file ffmpeg.c.
Referenced by open_output_file().
OutputStream** output_streams |
Definition at line 150 of file ffmpeg.c.
Referenced by new_output_stream(), and open_output_file().
int print_stats |
Definition at line 83 of file ffmpeg_opt.c.
Referenced by print_report().
AVIOContext* progress_avio |
Definition at line 134 of file ffmpeg.c.
Referenced by opt_progress().
int qp_hist |
Definition at line 84 of file ffmpeg_opt.c.
Referenced by check_keyboard_interaction(), and print_report().
int stdin_interaction |
Definition at line 85 of file ffmpeg_opt.c.
Referenced by assert_file_overwrite(), open_input_file(), and transcode().
int video_sync_method |
Definition at line 73 of file ffmpeg_opt.c.
Referenced by do_video_out(), opt_vsync(), transcode_init(), and write_frame().
char* vstats_filename |
Definition at line 65 of file ffmpeg_opt.c.
Referenced by do_video_out(), do_video_stats(), exit_program(), flush_encoders(), and opt_vstats_file().
Generated on Fri Dec 20 2024 06:56:12 for FFmpeg by
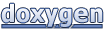