FFmpeg
|
eval.c
Go to the documentation of this file.
55 static const AVClass class = { "Eval", av_default_item_name, NULL, LIBAVUTIL_VERSION_INT, offsetof(Parser,log_offset), offsetof(Parser,log_ctx) };
128 #define IS_IDENTIFIER_CHAR(c) ((c) - '0' <= 9U || (c) - 'a' <= 25U || (c) - 'A' <= 25U || (c) == '_')
153 double (*func0)(double);
173 case e_func2: return e->value * e->a.func2(p->opaque, eval_expr(p, e->param[0]), eval_expr(p, e->param[1]));
708 int ret = av_expr_parse(&e, s, const_names, func1_names, funcs1, func2_names, funcs2, log_offset, log_ctx);
783 "st(1, 1); st(2, 2); st(0, 1); while(lte(ld(0),10), st(3, ld(1)+ld(2)); st(1, ld(2)); st(2, ld(3)); st(0, ld(0)+1)); ld(3)",
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: eval.c:143
Definition: eval.c:142
Definition: eval.c:145
Definition: eval.c:144
Definition: eval.c:143
external API header
int av_expr_parse(AVExpr **expr, const char *s, const char *const *const_names, const char *const *func1_names, double(*const *funcs1)(void *, double), const char *const *func2_names, double(*const *funcs2)(void *, double, double), int log_offset, void *log_ctx)
Parse an expression.
Definition: eval.c:640
Definition: eval.c:148
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: eval.c:146
Definition: eval.c:148
Definition: eval.c:143
Definition: eval.c:146
Definition: eval.c:142
Definition: eval.c:143
Definition: eval.c:140
double strtod(const char *, char **)
Definition: eval.c:144
Definition: eval.c:148
Definition: eval.c:142
int av_expr_parse_and_eval(double *d, const char *s, const char *const *const_names, const double *const_values, const char *const *func1_names, double(*const *funcs1)(void *, double), const char *const *func2_names, double(*const *funcs2)(void *, double, double), void *opaque, int log_offset, void *log_ctx)
Parse and evaluate an expression.
Definition: eval.c:701
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: eval.c:146
Definition: eval.c:146
Definition: eval.c:147
Definition: eval.c:148
Definition: eval.c:144
int64_t av_gcd(int64_t a, int64_t b)
Return the greatest common divisor of a and b.
Definition: mathematics.c:55
enum AVExpr::@166 type
Definition: eval.c:144
struct Parser Parser
Definition: eval.c:147
Definition: eval.c:146
Definition: eval.c:146
Definition: eval.c:142
Definition: eval.c:146
double av_strtod(const char *numstr, char **tail)
Parse the string in numstr and return its value as a double.
Definition: eval.c:89
1i.*Xphase exp()
Definition: eval.c:144
void av_expr_free(AVExpr *e)
Free a parsed expression previously created with av_expr_parse().
Definition: eval.c:302
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
union AVExpr::@167 a
Definition: eval.c:148
Definition: eval.c:144
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
Definition: eval.c:143
attribute_deprecated const uint8_t av_reverse[256]
Reverse the order of the bits of an 8-bits unsigned integer.
Definition: mathematics.c:35
Definition: eval.c:147
Definition: eval.c:144
Definition: eval.c:142
Definition: eval.c:147
Definition: eval.c:145
common internal and external API header
Definition: eval.c:145
Definition: eval.c:146
Definition: eval.c:147
double av_expr_eval(AVExpr *e, const double *const_values, void *opaque)
Evaluate a previously parsed expression.
Definition: eval.c:691
printf("static const uint8_t my_array[100] = {\n")
Definition: eval.c:144
Definition: eval.c:148
static AVExpr * make_eval_expr(int type, int value, AVExpr *p0, AVExpr *p1)
Definition: eval.c:465
static const struct @165 constants[]
Definition: eval.c:145
simple arithmetic expression evaluator
Generated on Fri Aug 1 2025 06:53:40 for FFmpeg by
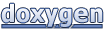