FFmpeg
|
ratecontrol.c
Go to the documentation of this file.
133 s->avctx->rc_max_available_vbv_use = av_clipf(s->avctx->rc_max_rate/(s->avctx->rc_buffer_size*get_fps(s->avctx)), 1.0/3, 1.0);
208 e += sscanf(p, " in:%*d out:%*d type:%d q:%f itex:%d ptex:%d mv:%d misc:%d fcode:%d bcode:%d mc-var:%d var:%d icount:%d skipcount:%d hbits:%d",
391 (rcc->i_cplx_sum[pict_type] + rcc->p_cplx_sum[pict_type]) / (double)rcc->frame_count[pict_type],
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
RateControlContext rc_context
contains stuff only accessed in ratecontrol.c
Definition: mpegvideo.h:512
double pass1_rc_eq_output_sum
sum of the output of the rc equation, this is used for normalization
Definition: ratecontrol.h:70
#define CODEC_FLAG_PASS2
Use internal 2pass ratecontrol in second pass mode.
Definition: libavcodec/avcodec.h:705
float border_masking
Border processing masking, raises the quantizer for mbs on the borders of the picture.
Definition: libavcodec/avcodec.h:1730
int rc_initial_buffer_occupancy
Number of bits which should be loaded into the rc buffer before decoding starts.
Definition: libavcodec/avcodec.h:2233
static void update_predictor(Predictor *p, double q, double var, double size)
Definition: ratecontrol.c:597
Definition: ratecontrol.h:35
char * stats_in
pass2 encoding statistics input buffer Concatenated stuff from stats_out of pass1 should be placed he...
Definition: libavcodec/avcodec.h:2367
Definition: libavcodec/avcodec.h:115
mpegvideo header.
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
int av_expr_parse(AVExpr **expr, const char *s, const char *const *const_names, const char *const *func1_names, double(*const *funcs1)(void *, double), const char *const *func2_names, double(*const *funcs2)(void *, double, double), int log_offset, void *log_ctx)
Parse an expression.
Definition: eval.c:640
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
static void get_qminmax(int *qmin_ret, int *qmax_ret, MpegEncContext *s, int pict_type)
Get the qmin & qmax for pict_type.
Definition: ratecontrol.c:473
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
int bit_rate_tolerance
number of bits the bitstream is allowed to diverge from the reference.
Definition: libavcodec/avcodec.h:1200
float b_quant_factor
qscale factor between IP and B-frames If > 0 then the last P-frame quantizer will be used (q= lastp_q...
Definition: libavcodec/avcodec.h:1394
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
char av_get_picture_type_char(enum AVPictureType pict_type)
Return a single letter to describe the given picture type pict_type.
Definition: libavutil/utils.c:76
static void adaptive_quantization(MpegEncContext *s, double q)
Definition: ratecontrol.c:609
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static double predict_size(Predictor *p, double q, double var)
Definition: ratecontrol.c:592
float i_quant_factor
qscale factor between P and I-frames If > 0 then the last p frame quantizer will be used (q= lastp_q*...
Definition: libavcodec/avcodec.h:1431
int ff_xvid_rate_control_init(MpegEncContext *s)
Definition: libxvid_rc.c:34
external API header
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
float rc_max_available_vbv_use
Ratecontrol attempt to use, at maximum, of what can be used without an underflow. ...
Definition: libavcodec/avcodec.h:2219
float rc_min_vbv_overflow_use
Ratecontrol attempt to use, at least, times the amount needed to prevent a vbv overflow.
Definition: libavcodec/avcodec.h:2226
float ff_rate_estimate_qscale(MpegEncContext *s, int dry_run)
Definition: ratecontrol.c:737
float rc_qsquish
ratecontrol qmin qmax limiting method 0-> clipping, 1-> use a nice continuous function to limit qscal...
Definition: libavcodec/avcodec.h:2164
int ticks_per_frame
For some codecs, the time base is closer to the field rate than the frame rate.
Definition: libavcodec/avcodec.h:1262
double pass1_wanted_bits
bits which should have been outputed by the pass1 code (including complexity init) ...
Definition: ratecontrol.h:71
void ff_xvid_rate_control_uninit(MpegEncContext *s)
Definition: libxvid_rc.c:137
1i.*Xphase exp()
#define CODEC_FLAG_NORMALIZE_AQP
Normalize adaptive quantization.
Definition: libavcodec/avcodec.h:711
void av_expr_free(AVExpr *e)
Free a parsed expression previously created with av_expr_parse().
Definition: eval.c:302
Definition: libavcodec/avcodec.h:674
float spatial_cplx_masking
spatial complexity masking (0-> disabled)
Definition: libavcodec/avcodec.h:1459
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
float rc_buffer_aggressivity
Definition: libavcodec/avcodec.h:2205
double last_qscale_for[5]
last qscale for a specific pict type, used for max_diff & ipb factor stuff
Definition: ratecontrol.h:73
float qcompress
amount of qscale change between easy & hard scenes (0.0-1.0)
Definition: libavcodec/avcodec.h:2134
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
float temporal_cplx_masking
temporary complexity masking (0-> disabled)
Definition: libavcodec/avcodec.h:1452
int mb_stride
mb_width+1 used for some arrays to allow simple addressing of left & top MBs without sig11 ...
Definition: mpegvideo.h:278
static double get_qscale(MpegEncContext *s, RateControlEntry *rce, double rate_factor, int frame_num)
Modify the bitrate curve from pass1 for one frame.
Definition: ratecontrol.c:355
Definition: ratecontrol.h:41
double av_expr_eval(AVExpr *e, const double *const_values, void *opaque)
Evaluate a previously parsed expression.
Definition: eval.c:691
ratecontrol header.
static double get_diff_limited_q(MpegEncContext *s, RateControlEntry *rce, double q)
Definition: ratecontrol.c:434
Note except for filters that can have queued request_frame does not push and as a the filter_frame method will be called and do the work Legacy the filter_frame method was it was made of start_frame
Definition: filter_design.txt:263
float ff_xvid_rate_estimate_qscale(MpegEncContext *s, int dry_run)
Definition: libxvid_rc.c:86
trying all byte sequences megabyte in length and selecting the best looking sequence will yield cases to try But a word about which is also called distortion Distortion can be quantified by almost any quality measurement one chooses the sum of squared differences is used but more complex methods that consider psychovisual effects can be used as well It makes no difference in this discussion First step
Definition: rate_distortion.txt:12
simple arithmetic expression evaluator
static double modify_qscale(MpegEncContext *s, RateControlEntry *rce, double q, int frame_num)
Definition: ratecontrol.c:501
Generated on Wed Jul 9 2025 06:53:37 for FFmpeg by
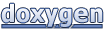