FFmpeg
|
libavutil/utils.c
Go to the documentation of this file.
36 av_assert0(AV_PIX_FMT_VDA_VLD == 81); //check if the pix fmt enum has not had anything inserted or removed by mistake
44 av_log(NULL, AV_LOG_FATAL, "Libavutil has been build with a broken binutils, please upgrade binutils and rebuild\n");
const char * avutil_configuration(void)
Return the libavutil build-time configuration.
Definition: libavutil/utils.c:53
external API header
#define LICENSE_PREFIX
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
char av_get_picture_type_char(enum AVPictureType pict_type)
Return a single letter to describe the given picture type pict_type.
Definition: libavutil/utils.c:76
unsigned avutil_version(void)
Return the LIBAVUTIL_VERSION_INT constant.
Definition: libavutil/utils.c:30
unsigned av_int_list_length_for_size(unsigned elsize, const void *list, uint64_t term)
Compute the length of an integer list.
Definition: libavutil/utils.c:90
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
#define LIST_LENGTH(type)
const char * av_get_media_type_string(enum AVMediaType media_type)
Return a string describing the media_type enum, NULL if media_type is unknown.
Definition: libavutil/utils.c:64
Definition: avutil.h:143
Definition: avutil.h:146
Generated on Thu May 29 2025 06:52:54 for FFmpeg by
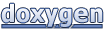