FFmpeg
|
opt.c
Go to the documentation of this file.
49 if (!strcmp(o->name, name) && (!unit || (o->unit && !strcmp(o->unit, unit))) && (o->flags & mask) == flags)
92 static int write_number(void *obj, const AVOption *o, void *dst, double num, int den, int64_t intnum)
182 static int set_string_number(void *obj, void *target_obj, const AVOption *o, const char *val, void *dst)
214 int res = av_expr_parse_and_eval(&d, buf, const_names, const_values, NULL, NULL, NULL, NULL, NULL, 0, obj);
243 int av_set_string3(void *obj, const char *name, const char *val, int alloc, const AVOption **o_out)
520 int av_opt_set_sample_fmt(void *obj, const char *name, enum AVSampleFormat fmt, int search_flags)
522 return set_format(obj, name, fmt, search_flags, AV_OPT_TYPE_SAMPLE_FMT, "sample", AV_SAMPLE_FMT_NB);
531 const char *av_get_string(void *obj, const char *name, const AVOption **o_out, char *buf, int buf_len)
551 case AV_OPT_TYPE_RATIONAL: snprintf(buf, buf_len, "%d/%d", ((AVRational*)dst)->num, ((AVRational*)dst)->den);break;
587 case AV_OPT_TYPE_RATIONAL: ret = snprintf(buf, sizeof(buf), "%d/%d", ((AVRational*)dst)->num, ((AVRational*)dst)->den);break;
609 ret = snprintf(buf, sizeof(buf), "%s", (char *)av_x_if_null(av_get_pix_fmt_name(*(enum AVPixelFormat *)dst), "none"));
612 ret = snprintf(buf, sizeof(buf), "%s", (char *)av_x_if_null(av_get_sample_fmt_name(*(enum AVSampleFormat *)dst), "none"));
630 static int get_number(void *obj, const char *name, const AVOption **o_out, double *num, int *den, int64_t *intnum,
727 int av_opt_get_image_size(void *obj, const char *name, int search_flags, int *w_out, int *h_out)
779 int av_opt_get_pixel_fmt(void *obj, const char *name, int search_flags, enum AVPixelFormat *out_fmt)
784 int av_opt_get_sample_fmt(void *obj, const char *name, int search_flags, enum AVSampleFormat *out_fmt)
1006 av_log(s, AV_LOG_DEBUG, "AVOption type %d of option %s not implemented yet\n", opt->type, opt->name);
1046 av_log(ctx, AV_LOG_ERROR, "Missing key or no key/value separator found after key '%s'\n", key);
1307 int av_opt_query_ranges_default(AVOptionRanges **ranges_arg, void *obj, const char *key, int flags)
1415 {"string", "set string", OFFSET(string), AV_OPT_TYPE_STRING, {.str = "default"}, CHAR_MIN, CHAR_MAX },
1417 {"cool", "set cool flag ", 0, AV_OPT_TYPE_CONST, {.i64 = TEST_FLAG_COOL}, INT_MIN, INT_MAX, 0, "flags" },
1418 {"lame", "set lame flag ", 0, AV_OPT_TYPE_CONST, {.i64 = TEST_FLAG_LAME}, INT_MIN, INT_MAX, 0, "flags" },
1419 {"mu", "set mu flag ", 0, AV_OPT_TYPE_CONST, {.i64 = TEST_FLAG_MU}, INT_MIN, INT_MAX, 0, "flags" },
1421 {"pix_fmt", "set pixfmt", OFFSET(pix_fmt), AV_OPT_TYPE_PIXEL_FMT, {.i64 = AV_PIX_FMT_NONE}, -1, AV_PIX_FMT_NB-1},
1422 {"sample_fmt", "set samplefmt", OFFSET(sample_fmt), AV_OPT_TYPE_SAMPLE_FMT, {.i64 = AV_SAMPLE_FMT_NONE}, -1, AV_SAMPLE_FMT_NB-1},
1423 {"video_rate", "set videorate", OFFSET(video_rate), AV_OPT_TYPE_VIDEO_RATE, {.str = "25"}, 0, 0 },
1424 {"duration", "set duration", OFFSET(duration), AV_OPT_TYPE_DURATION, {.i64 = 0}, 0, INT64_MAX},
static int read_number(const AVOption *o, void *dst, double *num, int *den, int64_t *intnum)
Definition: opt.c:73
Number of sample formats. DO NOT USE if linking dynamically.
Definition: samplefmt.h:63
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: opt.h:223
static void callback(void *priv_data, int index, uint8_t *buf, int buf_size, int64_t time)
Definition: dshow.c:200
int av_parse_video_rate(AVRational *rate, const char *arg)
Parse str and store the detected values in *rate.
Definition: parseutils.c:162
Definition: pixfmt.h:67
Definition: dict.h:80
int av_opt_set_q(void *obj, const char *name, AVRational val, int search_flags)
Definition: opt.c:404
int av_parse_video_size(int *width_ptr, int *height_ptr, const char *str)
Parse str and put in width_ptr and height_ptr the detected values.
Definition: parseutils.c:131
void * av_opt_child_next(void *obj, void *prev)
Iterate over AVOptions-enabled children of obj.
Definition: opt.c:1270
int av_parse_time(int64_t *timeval, const char *timestr, int duration)
Parse timestr and return in *time a corresponding number of microseconds.
Definition: parseutils.c:530
static int get_key(const char **ropts, const char *delim, char **rkey)
Read a key from a string.
Definition: opt.c:1099
if max(w)>1 w=0.9 *w/max(w)
Definition: opt.h:222
double value_max
For string ranges this represents the min/max length, for dimensions this represents the min/max pixe...
Definition: opt.h:303
const AVClass * av_opt_child_class_next(const AVClass *parent, const AVClass *prev)
Iterate over potential AVOptions-enabled children of parent.
Definition: opt.c:1278
void av_opt_set_defaults(void *s)
Set the values of all AVOption fields to their default values.
Definition: opt.c:942
Definition: opt.h:231
int av_set_options_string(void *ctx, const char *opts, const char *key_val_sep, const char *pairs_sep)
Parse the key/value pairs list in opts.
Definition: opt.c:1062
external API header
int av_opt_set_bin(void *obj, const char *name, const uint8_t *val, int len, int search_flags)
Definition: opt.c:409
Accept to parse a value without a key; the key will then be returned as NULL.
Definition: opt.h:511
int av_opt_get_q(void *obj, const char *name, int search_flags, AVRational *out_val)
Definition: opt.c:711
static void opt_list(void *obj, void *av_log_obj, const char *unit, int req_flags, int rej_flags)
Definition: opt.c:821
AVDictionaryEntry * av_dict_get(AVDictionary *m, const char *key, const AVDictionaryEntry *prev, int flags)
Get a dictionary entry with matching key.
Definition: dict.c:39
Definition: opt.h:221
const char * av_get_string(void *obj, const char *name, const AVOption **o_out, char *buf, int buf_len)
Definition: opt.c:531
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
int av_opt_get_video_rate(void *obj, const char *name, int search_flags, AVRational *out_val)
Definition: opt.c:745
int av_opt_set_from_string(void *ctx, const char *opts, const char *const *shorthand, const char *key_val_sep, const char *pairs_sep)
Parse the key-value pairs list in opts.
Definition: opt.c:1142
static int get_format(void *obj, const char *name, int search_flags, int *out_fmt, enum AVOptionType type, const char *desc)
Definition: opt.c:761
Public dictionary API.
Definition: opt.h:229
Definition: samplefmt.h:50
AVOptions.
const struct AVOption * option
a pointer to the first option specified in the class if any or NULL
Definition: log.h:68
const AVOption * av_set_double(void *obj, const char *name, double n)
Definition: opt.c:369
int av_opt_set_double(void *obj, const char *name, double val, int search_flags)
Definition: opt.c:399
AVRational av_get_q(void *obj, const char *name, const AVOption **o_out)
Definition: opt.c:661
Definition: opt.h:226
double component_max
For string this represents the unicode range for chars, 0-127 limits to ASCII.
Definition: opt.h:304
static int get_number(void *obj, const char *name, const AVOption **o_out, double *num, int *den, int64_t *intnum, int search_flags)
Definition: opt.c:630
int av_opt_set_video_rate(void *obj, const char *name, AVRational val, int search_flags)
Definition: opt.c:461
#define AV_OPT_FLAG_ENCODING_PARAM
a generic parameter which can be set by the user for muxing or encoding
Definition: opt.h:281
static void * av_x_if_null(const void *p, const void *x)
Return x default pointer in case p is NULL.
Definition: avutil.h:250
void *(* child_next)(void *obj, void *prev)
Return next AVOptions-enabled child or NULL.
Definition: log.h:96
int av_opt_get_pixel_fmt(void *obj, const char *name, int search_flags, enum AVPixelFormat *out_fmt)
Definition: opt.c:779
int av_expr_parse_and_eval(double *d, const char *s, const char *const *const_names, const double *const_values, const char *const *func1_names, double(*const *funcs1)(void *, double), const char *const *func2_names, double(*const *funcs2)(void *, double, double), void *opaque, int log_offset, void *log_ctx)
Parse and evaluate an expression.
Definition: eval.c:701
Definition: dict.c:28
int av_opt_set_pixel_fmt(void *obj, const char *name, enum AVPixelFormat fmt, int search_flags)
Definition: opt.c:515
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
void * av_opt_ptr(const AVClass *class, void *obj, const char *name)
Gets a pointer to the requested field in a struct.
Definition: opt.c:1285
#define AV_OPT_FLAG_FILTERING_PARAM
a generic parameter which can be set by the user for filtering
Definition: opt.h:287
int(* query_ranges)(struct AVOptionRanges **, void *obj, const char *key, int flags)
Callback to return the supported/allowed ranges.
Definition: log.h:125
Definition: opt.h:225
void av_dict_free(AVDictionary **pm)
Free all the memory allocated for an AVDictionary struct and all keys and values. ...
Definition: dict.c:162
const AVOption * av_opt_next(void *obj, const AVOption *last)
Iterate over all AVOptions belonging to obj.
Definition: opt.c:63
int av_opt_set_int(void *obj, const char *name, int64_t val, int search_flags)
Definition: opt.c:394
double av_get_double(void *obj, const char *name, const AVOption **o_out)
Definition: opt.c:650
char * av_get_token(const char **buf, const char *term)
Unescape the given string until a non escaped terminating char, and return the token corresponding to...
Definition: avstring.c:148
const AVOption * av_opt_find(void *obj, const char *name, const char *unit, int opt_flags, int search_flags)
Look for an option in an object.
Definition: opt.c:1223
static int set_string(void *obj, const AVOption *o, const char *val, uint8_t **dst)
Definition: opt.c:169
int av_opt_get_sample_fmt(void *obj, const char *name, int search_flags, enum AVSampleFormat *out_fmt)
Definition: opt.c:784
const AVOption * av_find_opt(void *v, const char *name, const char *unit, int mask, int flags)
Look for an option in obj.
Definition: opt.c:44
AVRational av_d2q(double d, int max)
Convert a double precision floating point number to a rational.
Definition: rational.c:106
#define AV_OPT_SEARCH_CHILDREN
Search in possible children of the given object first.
Definition: opt.h:538
int64_t av_get_int(void *obj, const char *name, const AVOption **o_out)
Definition: opt.c:675
#define av_err2str(errnum)
Convenience macro, the return value should be used only directly in function arguments but never stan...
Definition: error.h:110
static int parse_key_value_pair(void *ctx, const char **buf, const char *key_val_sep, const char *pairs_sep)
Store the value in the field in ctx that is named like key.
Definition: opt.c:1028
static int set_string_number(void *obj, void *target_obj, const AVOption *o, const char *val, void *dst)
Definition: opt.c:182
static int set_string_binary(void *obj, const AVOption *o, const char *val, uint8_t **dst)
Definition: opt.c:140
const struct AVClass *(* child_class_next)(const struct AVClass *prev)
Return an AVClass corresponding to the next potential AVOptions-enabled child.
Definition: log.h:106
const char * av_get_sample_fmt_name(enum AVSampleFormat sample_fmt)
Return the name of sample_fmt, or NULL if sample_fmt is not recognized.
Definition: samplefmt.c:47
offset must point to a pointer immediately followed by an int for the length
Definition: opt.h:228
int av_opt_query_ranges_default(AVOptionRanges **ranges_arg, void *obj, const char *key, int flags)
Get a default list of allowed ranges for the given option.
Definition: opt.c:1307
int is_range
if set to 1 the struct encodes a range, if set to 0 a single value
Definition: opt.h:305
int av_opt_get_int(void *obj, const char *name, int search_flags, int64_t *out_val)
Definition: opt.c:687
Definition: opt.h:224
static int set_format(void *obj, const char *name, int fmt, int search_flags, enum AVOptionType type, const char *desc, int nb_fmts)
Definition: opt.c:478
void av_opt_freep_ranges(AVOptionRanges **rangesp)
Free an AVOptionRanges struct and set it to NULL.
Definition: opt.c:1374
int offset
The offset relative to the context structure where the option value is stored.
Definition: opt.h:264
int av_opt_set_dict(void *obj, AVDictionary **options)
Set all the options from a given dictionary on an object.
Definition: opt.c:1202
static int set_number(void *obj, const char *name, double num, int den, int64_t intnum, int search_flags)
Definition: opt.c:355
int av_opt_show2(void *obj, void *av_log_obj, int req_flags, int rej_flags)
Show the obj options.
Definition: opt.c:930
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
misc parsing utilities
#define type
Definition: opt.h:232
Definition: opt.h:234
int av_opt_query_ranges(AVOptionRanges **ranges_arg, void *obj, const char *key, int flags)
Get a list of allowed ranges for the given option.
Definition: opt.c:1293
common internal and external API header
int av_opt_get_double(void *obj, const char *name, int search_flags, double *out_val)
Definition: opt.c:699
#define AV_OPT_SEARCH_FAKE_OBJ
The obj passed to av_opt_find() is fake – only a double pointer to AVClass instead of a required poi...
Definition: opt.h:547
static int write_number(void *obj, const AVOption *o, void *dst, double num, int den, int64_t intnum)
Definition: opt.c:92
int av_set_string3(void *obj, const char *name, const char *val, int alloc, const AVOption **o_out)
Set the field of obj with the given name to value.
Definition: opt.c:243
int av_opt_get(void *obj, const char *name, int search_flags, uint8_t **out_val)
Definition: opt.c:566
int av_opt_get_key_value(const char **ropts, const char *key_val_sep, const char *pairs_sep, unsigned flags, char **rkey, char **rval)
Extract a key-value pair from the beginning of a string.
Definition: opt.c:1120
printf("static const uint8_t my_array[100] = {\n")
int av_opt_flag_is_set(void *obj, const char *field_name, const char *flag_name)
Check whether a particular flag is set in a flags field.
Definition: opt.c:789
number of pixel formats, DO NOT USE THIS if you want to link with shared libav* because the number of...
Definition: pixfmt.h:237
Definition: opt.h:227
const AVOption * av_opt_find2(void *obj, const char *name, const char *unit, int opt_flags, int search_flags, void **target_obj)
Look for an option in an object.
Definition: opt.c:1229
enum AVPixelFormat av_get_pix_fmt(const char *name)
Return the pixel format corresponding to name.
Definition: pixdesc.c:1712
const char * av_get_pix_fmt_name(enum AVPixelFormat pix_fmt)
Return the short name for a pixel format, NULL in case pix_fmt is unknown.
Definition: pixdesc.c:1700
int av_opt_set_sample_fmt(void *obj, const char *name, enum AVSampleFormat fmt, int search_flags)
Definition: opt.c:520
int av_opt_set_image_size(void *obj, const char *name, int w, int h, int search_flags)
Definition: opt.c:439
int av_opt_set(void *obj, const char *name, const char *val, int search_flags)
Definition: opt.c:252
enum AVSampleFormat av_get_sample_fmt(const char *name)
Return a sample format corresponding to name, or AV_SAMPLE_FMT_NONE on error.
Definition: samplefmt.c:54
simple arithmetic expression evaluator
int av_opt_get_image_size(void *obj, const char *name, int search_flags, int *w_out, int *h_out)
Definition: opt.c:727
Generated on Thu May 29 2025 06:52:51 for FFmpeg by
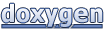