FFmpeg
|
ffmpeg_opt.c
Go to the documentation of this file.
412 static int copy_metadata(char *outspec, char *inspec, AVFormatContext *oc, AVFormatContext *ic, OptionsContext *o)
781 av_dict_set(&o->g->format_opts, "pixel_format", o->frame_pix_fmts[o->nb_frame_pix_fmts - 1].u.str, 0);
966 static OutputStream *new_output_stream(OptionsContext *o, AVFormatContext *oc, enum AVMediaType type, int source_index)
1029 st->codec->codec_type = type; // XXX hack, avcodec_get_context_defaults2() sets type to unknown for stream copy
1036 av_log(NULL, AV_LOG_WARNING, "Applying unspecific -frames to non video streams, maybe you meant -vframes ?\n");
1166 static OutputStream *new_video_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
1203 if (frame_size && av_parse_video_size(&video_enc->width, &video_enc->height, frame_size) < 0) {
1215 if (frame_pix_fmt && (video_enc->pix_fmt = av_get_pix_fmt(frame_pix_fmt)) == AV_PIX_FMT_NONE) {
1309 static OutputStream *new_audio_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
1344 if ((map->channel_idx == -1 || (ist->file_index == map->file_idx && ist->st->index == map->stream_idx)) &&
1372 static OutputStream *new_attachment_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
1380 static OutputStream *new_subtitle_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
1398 if (frame_size && av_parse_video_size(&subtitle_enc->width, &subtitle_enc->height, frame_size) < 0) {
1425 o->streamid_map = grow_array(o->streamid_map, sizeof(*o->streamid_map), &o->nb_streamid_map, idx+1);
1631 av_log(NULL, AV_LOG_FATAL, "Missing %s stream which is required by this ffm\n", av_get_media_type_string(ost->st->codec->codec_type));
1678 if (!o->subtitle_disable && (oc->oformat->subtitle_codec != AV_CODEC_ID_NONE || subtitle_codec_name)) {
1774 av_dict_set(&ost->st->metadata, "filename", (p && *p) ? p + 1 : o->attachments[i], AV_DICT_DONT_OVERWRITE);
1778 for (i = nb_output_streams - oc->nb_streams; i < nb_output_streams; i++) { //for all streams of this output file
1888 av_log(NULL, AV_LOG_FATAL, "Invalid input file index %d while processing metadata maps\n", in_file_index);
2094 av_dict_set(&o->g->format_opts, "packetsize", "2048", 0); // from www.mpucoder.com: DVD sectors contain 2048 bytes of data, this is also the size of one pack.
2095 av_dict_set(&o->g->format_opts, "muxrate", "10080000", 0); // from mplex project: data_rate = 1260000. mux_rate = data_rate * 8
2436 show_help_children(avfilter_get_class(), AV_OPT_FLAG_VIDEO_PARAM | AV_OPT_FLAG_AUDIO_PARAM | AV_OPT_FLAG_FILTERING_PARAM);
2443 av_log(NULL, AV_LOG_INFO, "usage: %s [options] [[infile options] -i infile]... {[outfile options] outfile}...\n", program_name);
2577 { "map_channel", HAS_ARG | OPT_EXPERT | OPT_PERFILE | OPT_OUTPUT, { .func_arg = opt_map_channel },
2578 "map an audio channel from one stream to another", "file.stream.channel[:syncfile.syncstream]" },
2586 { "t", HAS_ARG | OPT_TIME | OPT_OFFSET | OPT_INPUT | OPT_OUTPUT, { .off = OFFSET(recording_time) },
2651 { "copypriorss", OPT_INT | HAS_ARG | OPT_EXPERT | OPT_SPEC | OPT_OUTPUT, { .off = OFFSET(copy_prior_start) },
2668 { "filter_script", HAS_ARG | OPT_STRING | OPT_SPEC | OPT_OUTPUT, { .off = OFFSET(filter_scripts) },
2670 { "reinit_filter", HAS_ARG | OPT_INT | OPT_SPEC | OPT_INPUT, { .off = OFFSET(reinit_filters) },
2708 { "vn", OPT_VIDEO | OPT_BOOL | OPT_OFFSET | OPT_INPUT | OPT_OUTPUT,{ .off = OFFSET(video_disable) },
2778 { "an", OPT_AUDIO | OPT_BOOL | OPT_OFFSET | OPT_INPUT | OPT_OUTPUT,{ .off = OFFSET(audio_disable) },
2796 { "guess_layout_max", OPT_AUDIO | HAS_ARG | OPT_INT | OPT_SPEC | OPT_EXPERT | OPT_INPUT, { .off = OFFSET(guess_layout_max) },
2800 { "sn", OPT_SUBTITLE | OPT_BOOL | OPT_OFFSET | OPT_INPUT | OPT_OUTPUT, { .off = OFFSET(subtitle_disable) },
2802 { "scodec", OPT_SUBTITLE | HAS_ARG | OPT_PERFILE | OPT_INPUT | OPT_OUTPUT, { .func_arg = opt_subtitle_codec },
2804 { "stag", OPT_SUBTITLE | HAS_ARG | OPT_EXPERT | OPT_PERFILE | OPT_OUTPUT, { .func_arg = opt_old2new }
2806 { "fix_sub_duration", OPT_BOOL | OPT_EXPERT | OPT_SUBTITLE | OPT_SPEC | OPT_INPUT, { .off = OFFSET(fix_sub_duration) },
2808 { "canvas_size", OPT_SUBTITLE | HAS_ARG | OPT_STRING | OPT_SPEC | OPT_INPUT, { .off = OFFSET(canvas_sizes) },
2816 { "isync", OPT_BOOL | OPT_EXPERT, { &input_sync }, "this option is deprecated and does nothing", "" },
2819 { "muxdelay", OPT_FLOAT | HAS_ARG | OPT_EXPERT | OPT_OFFSET | OPT_OUTPUT, { .off = OFFSET(mux_max_delay) },
2821 { "muxpreload", OPT_FLOAT | HAS_ARG | OPT_EXPERT | OPT_OFFSET | OPT_OUTPUT, { .off = OFFSET(mux_preload) },
2824 { "bsf", HAS_ARG | OPT_STRING | OPT_SPEC | OPT_EXPERT | OPT_OUTPUT, { .off = OFFSET(bitstream_filters) },
2826 { "absf", HAS_ARG | OPT_AUDIO | OPT_EXPERT| OPT_PERFILE | OPT_OUTPUT, { .func_arg = opt_old2new },
2828 { "vbsf", OPT_VIDEO | HAS_ARG | OPT_EXPERT| OPT_PERFILE | OPT_OUTPUT, { .func_arg = opt_old2new },
2831 { "apre", HAS_ARG | OPT_AUDIO | OPT_EXPERT| OPT_PERFILE | OPT_OUTPUT, { .func_arg = opt_preset },
2833 { "vpre", OPT_VIDEO | HAS_ARG | OPT_EXPERT| OPT_PERFILE | OPT_OUTPUT, { .func_arg = opt_preset },
2835 { "spre", HAS_ARG | OPT_SUBTITLE | OPT_EXPERT| OPT_PERFILE | OPT_OUTPUT, { .func_arg = opt_preset },
2840 { "dcodec", HAS_ARG | OPT_DATA | OPT_PERFILE | OPT_EXPERT | OPT_INPUT | OPT_OUTPUT, { .func_arg = opt_data_codec },
2842 { "dn", OPT_BOOL | OPT_VIDEO | OPT_OFFSET | OPT_INPUT | OPT_OUTPUT, { .off = OFFSET(data_disable) },
int avio_open(AVIOContext **s, const char *url, int flags)
Create and initialize a AVIOContext for accessing the resource indicated by url.
Definition: aviobuf.c:799
int parse_optgroup(void *optctx, OptionGroup *g)
Parse an options group and write results into optctx.
Definition: cmdutils.c:376
int av_parse_ratio(AVRational *q, const char *str, int max, int log_offset, void *log_ctx)
Parse str and store the parsed ratio in q.
Definition: parseutils.c:43
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: libavcodec/avcodec.h:100
int64_t recording_time
desired length of the resulting file in microseconds == AV_TIME_BASE units
Definition: ffmpeg.h:371
static void choose_encoder(OptionsContext *o, AVFormatContext *s, OutputStream *ost)
Definition: ffmpeg_opt.c:949
int av_parse_video_rate(AVRational *rate, const char *arg)
Parse str and store the detected values in *rate.
Definition: parseutils.c:162
Definition: pixfmt.h:67
AVCodec * avcodec_find_encoder(enum AVCodecID id)
Find a registered encoder with a matching codec ID.
Definition: libavcodec/utils.c:2378
Definition: dict.h:80
static int opt_data_frames(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2149
AVIOInterruptCB interrupt_callback
Custom interrupt callbacks for the I/O layer.
Definition: avformat.h:1125
int avio_close_dyn_buf(AVIOContext *s, uint8_t **pbuffer)
Return the written size and a pointer to the buffer.
Definition: aviobuf.c:988
int av_parse_video_size(int *width_ptr, int *height_ptr, const char *str)
Parse str and put in width_ptr and height_ptr the detected values.
Definition: parseutils.c:131
static void init_output_filter(OutputFilter *ofilter, OptionsContext *o, AVFormatContext *oc)
Definition: ffmpeg_opt.c:1515
#define CODEC_FLAG_PASS2
Use internal 2pass ratecontrol in second pass mode.
Definition: libavcodec/avcodec.h:705
void * av_realloc_f(void *ptr, size_t nelem, size_t elsize)
Allocate or reallocate a block of memory.
Definition: mem.c:168
external API header
int avformat_open_input(AVFormatContext **ps, const char *filename, AVInputFormat *fmt, AVDictionary **options)
Open an input stream and read the header.
Definition: libavformat/utils.c:614
static void assert_file_overwrite(const char *filename)
Definition: ffmpeg_opt.c:659
int avformat_query_codec(AVOutputFormat *ofmt, enum AVCodecID codec_id, int std_compliance)
Test if the given container can store a codec.
Definition: libavformat/utils.c:4182
#define CODEC_FLAG_PASS1
Use internal 2pass ratecontrol in first pass mode.
Definition: libavcodec/avcodec.h:704
int split_commandline(OptionParseContext *octx, int argc, char *argv[], const OptionDef *options, const OptionGroupDef *groups, int nb_groups)
Split the commandline into an intermediate form convenient for further processing.
Definition: cmdutils.c:678
void avfilter_inout_free(AVFilterInOut **inout)
Free the supplied list of AVFilterInOut and set *inout to NULL.
Definition: graphparser.c:175
Definition: ffmpeg.h:190
Definition: rtspcodes.h:52
int check_stream_specifier(AVFormatContext *s, AVStream *st, const char *spec)
Check if the given stream matches a stream specifier.
Definition: cmdutils.c:1828
enum AVMediaType avfilter_pad_get_type(const AVFilterPad *pads, int pad_idx)
Get the type of an AVFilterPad.
Definition: avfilter.c:842
static int sync(AVFormatContext *s, uint8_t *header)
Read input until we find the next ident.
Definition: lxfdec.c:85
static int opt_audio_filters(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2277
static int read_ffserver_streams(OptionsContext *o, AVFormatContext *s, const char *filename)
Definition: ffmpeg_opt.c:1472
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
external API header
Definition: cmdutils.h:144
static int opt_filter_complex(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2348
Definition: cmdutils.h:256
static int copy_chapters(InputFile *ifile, OutputFile *ofile, int copy_metadata)
Definition: ffmpeg_opt.c:1430
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
struct AVStream::@125 * info
Definition: cmdutils.h:133
static OutputStream * new_output_stream(OptionsContext *o, AVFormatContext *oc, enum AVMediaType type, int source_index)
Definition: ffmpeg_opt.c:966
Definition: avformat.h:456
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
AVDictionaryEntry * av_dict_get(AVDictionary *m, const char *key, const AVDictionaryEntry *prev, int flags)
Get a dictionary entry with matching key.
Definition: dict.c:39
int avcodec_copy_context(AVCodecContext *dest, const AVCodecContext *src)
Copy the settings of the source AVCodecContext into the destination AVCodecContext.
Definition: libavcodec/options.c:182
AVDictionary * filter_codec_opts(AVDictionary *opts, enum AVCodecID codec_id, AVFormatContext *s, AVStream *st, AVCodec *codec)
Filter out options for given codec.
Definition: cmdutils.c:1836
static int opt_preset(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2175
Definition: ffmpeg.h:276
static int opt_data_codec(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:210
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
#define MATCH_PER_STREAM_OPT(name, type, outvar, fmtctx, st)
Definition: ffmpeg_opt.c:44
void uninit_parse_context(OptionParseContext *octx)
Free all allocated memory in an OptionParseContext.
Definition: cmdutils.c:651
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
static int opt_audio_qscale(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2342
const AVClass * avcodec_get_class(void)
Get the AVClass for AVCodecContext.
Definition: libavcodec/options.c:239
int avio_check(const char *url, int flags)
Return AVIO_FLAG_* access flags corresponding to the access permissions of the resource in url...
Definition: avio.c:365
#define CODEC_FLAG_PSNR
error[?] variables will be set during encoding.
Definition: libavcodec/avcodec.h:708
Definition: cmdutils.h:241
#define AVFMT_FLAG_NONBLOCK
Do not block when reading packets from input.
Definition: avformat.h:1024
int flags
can use flags: AVFMT_NOFILE, AVFMT_NEEDNUMBER, AVFMT_RAWPICTURE, AVFMT_GLOBALHEADER, AVFMT_NOTIMESTAMPS, AVFMT_VARIABLE_FPS, AVFMT_NODIMENSIONS, AVFMT_NOSTREAMS, AVFMT_ALLOW_FLUSH, AVFMT_TS_NONSTRICT
Definition: avformat.h:397
int opt_default(void *optctx, const char *opt, const char *arg)
Fallback for options that are not explicitly handled, these will be parsed through AVOptions...
Definition: cmdutils.c:483
Definition: samplefmt.h:50
AVOptions.
static int open_input_file(OptionsContext *o, const char *filename)
Definition: ffmpeg_opt.c:716
#define CODEC_FLAG_GLOBAL_HEADER
Place global headers in extradata instead of every keyframe.
Definition: libavcodec/avcodec.h:714
static int opt_vsync(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2283
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
Definition: ffmpeg.h:213
static int opt_vstats(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2126
AVFormatContext * avformat_alloc_context(void)
Allocate an AVFormatContext.
Definition: libavformat/options.c:106
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
const AVClass * avformat_get_class(void)
Get the AVClass for AVFormatContext.
Definition: libavformat/options.c:120
void show_help_options(const OptionDef *options, const char *msg, int req_flags, int rej_flags, int alt_flags)
Print help for all options matching specified flags.
Definition: cmdutils.c:147
void avio_write(AVIOContext *s, const unsigned char *buf, int size)
Definition: aviobuf.c:173
Definition: ffmpeg.h:59
struct AVBitStreamFilterContext * next
Definition: libavcodec/avcodec.h:4531
int64_t filter_in_rescale_delta_last
Definition: ffmpeg.h:232
#define AV_OPT_FLAG_ENCODING_PARAM
a generic parameter which can be set by the user for muxing or encoding
Definition: opt.h:281
int avio_read(AVIOContext *s, unsigned char *buf, int size)
Read size bytes from AVIOContext into buf.
Definition: aviobuf.c:478
static int opt_video_codec(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:198
static int opt_qscale(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2244
AVDictionary ** setup_find_stream_info_opts(AVFormatContext *s, AVDictionary *codec_opts)
Setup AVCodecContext options for avformat_find_stream_info().
Definition: cmdutils.c:1892
AVCodec * avcodec_find_encoder_by_name(const char *name)
Find a registered encoder with the specified name.
Definition: libavcodec/utils.c:2383
static OutputStream * new_subtitle_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
Definition: ffmpeg_opt.c:1380
int avformat_alloc_output_context2(AVFormatContext **ctx, AVOutputFormat *oformat, const char *format_name, const char *filename)
Allocate an AVFormatContext for an output format.
Definition: mux.c:125
int64_t av_rescale_q(int64_t a, AVRational bq, AVRational cq)
Rescale a 64-bit integer by 2 rational numbers.
Definition: mathematics.c:130
void av_dump_format(AVFormatContext *ic, int index, const char *url, int is_output)
Definition: libavformat/utils.c:3599
void av_dict_copy(AVDictionary **dst, AVDictionary *src, int flags)
Copy entries from one AVDictionary struct into another.
Definition: dict.c:176
Definition: dict.c:28
static int opt_profile(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2259
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static int open_files(OptionGroupList *l, const char *inout, int(*open_file)(OptionsContext *, const char *))
Definition: ffmpeg_opt.c:2457
static int opt_subtitle_codec(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:204
#define AV_OPT_FLAG_FILTERING_PARAM
a generic parameter which can be set by the user for filtering
Definition: opt.h:287
static int opt_video_channel(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:180
static int opt_recording_timestamp(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:502
Definition: cmdutils.h:281
Definition: ffmpeg.h:73
int avio_close(AVIOContext *s)
Close the resource accessed by the AVIOContext s and free it.
Definition: aviobuf.c:821
void av_dict_free(AVDictionary **pm)
Free all the memory allocated for an AVDictionary struct and all keys and values. ...
Definition: dict.c:162
int av_get_channel_layout_nb_channels(uint64_t channel_layout)
Return the number of channels in the channel layout.
Definition: channel_layout.c:191
static int opt_bitrate(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2232
int parse_option(void *optctx, const char *opt, const char *arg, const OptionDef *options)
Parse one given option.
Definition: cmdutils.c:312
Definition: graph2dot.c:48
static void parse_meta_type(char *arg, char *type, int *index, const char **stream_spec)
Parse a metadata specifier passed as 'arg' parameter.
Definition: ffmpeg_opt.c:385
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
static int opt_old2new(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2223
static int opt_sameq(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:171
static int copy_metadata(char *outspec, char *inspec, AVFormatContext *oc, AVFormatContext *ic, OptionsContext *o)
Definition: ffmpeg_opt.c:412
external API header
size_t av_strlcpy(char *dst, const char *src, size_t size)
Copy the string src to dst, but no more than size - 1 bytes, and null-terminate dst.
Definition: avstring.c:82
char * av_get_token(const char **buf, const char *term)
Unescape the given string until a non escaped terminating char, and return the token corresponding to...
Definition: avstring.c:148
Definition: ffmpeg.h:68
int intra_dc_precision
precision of the intra DC coefficient - 8
Definition: libavcodec/avcodec.h:1708
static AVDictionary * strip_specifiers(AVDictionary *dict)
Definition: ffmpeg_opt.c:154
const AVOption * av_opt_find(void *obj, const char *name, const char *unit, int opt_flags, int search_flags)
Look for an option in an object.
Definition: opt.c:1223
AVInputFormat * av_find_input_format(const char *short_name)
Find AVInputFormat based on the short name of the input format.
Definition: libavformat/utils.c:248
static int opt_video_filters(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2271
int void avio_flush(AVIOContext *s)
Force flushing of buffered data to the output s.
Definition: aviobuf.c:193
audio channel layout utility functions
int av_strcasecmp(const char *a, const char *b)
Locale-independent case-insensitive compare.
Definition: avstring.c:212
static void add_input_streams(OptionsContext *o, AVFormatContext *ic)
Definition: ffmpeg_opt.c:560
int av_get_exact_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2619
#define AV_OPT_SEARCH_CHILDREN
Search in possible children of the given object first.
Definition: opt.h:538
static int opt_progress(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2536
enum AVCodecID av_guess_codec(AVOutputFormat *fmt, const char *short_name, const char *filename, const char *mime_type, enum AVMediaType type)
Guess the codec ID based upon muxer and filename.
Definition: libavformat/utils.c:223
AVBitStreamFilterContext * av_bitstream_filter_init(const char *name)
Definition: bitstream_filter.c:38
static int open_output_file(OptionsContext *o, const char *filename)
Definition: ffmpeg_opt.c:1560
#define av_err2str(errnum)
Convenience macro, the return value should be used only directly in function arguments but never stan...
Definition: error.h:110
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
A list of option groups that all have the same group type (e.g.
Definition: cmdutils.h:274
static AVCodec * find_codec_or_die(const char *name, enum AVMediaType type, int encoder)
Definition: ffmpeg_opt.c:516
int opt_timelimit(void *optctx, const char *opt, const char *arg)
Limit the execution time.
Definition: cmdutils.c:949
const AVCodecDescriptor * avcodec_descriptor_get_by_name(const char *name)
Definition: codec_desc.c:2585
AVFilterContext * filter_ctx
filter context associated to this input/output
Definition: avfilter.h:1128
#define AV_DISPOSITION_ATTACHED_PIC
The stream is stored in the file as an attached picture/"cover art" (e.g.
Definition: avformat.h:627
Definition: ffmpeg_opt.c:2449
int av_opt_get_int(void *obj, const char *name, int search_flags, int64_t *out_val)
Definition: opt.c:687
Close file fclose(fid)
#define SET_DICT(type, meta, context, index)
enum AVPixelFormat choose_pixel_fmt(AVStream *st, AVCodec *codec, enum AVPixelFormat target)
Definition: ffmpeg_filter.c:38
AVCodec * avcodec_find_decoder(enum AVCodecID id)
Find a registered decoder with a matching codec ID.
Definition: libavcodec/utils.c:2397
int av_filename_number_test(const char *filename)
Check whether filename actually is a numbered sequence generator.
Definition: libavformat/utils.c:346
Definition: libavcodec/avcodec.h:674
int configure_output_filter(FilterGraph *fg, OutputFilter *ofilter, AVFilterInOut *out)
Definition: ffmpeg_filter.c:483
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
a very simple circular buffer FIFO implementation
void show_help_default(const char *opt, const char *arg)
Per-fftool specific help handler.
Definition: ffmpeg_opt.c:2374
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
static int opt_map_channel(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:319
FILE * get_preset_file(char *filename, size_t filename_size, const char *preset_name, int is_path, const char *codec_name)
Get a file corresponding to a preset file.
Definition: cmdutils.c:1778
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static int opt_filter_complex_script(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2360
static char * get_ost_filters(OptionsContext *o, AVFormatContext *oc, OutputStream *ost)
Definition: ffmpeg_opt.c:1142
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
int64_t parse_time_or_die(const char *context, const char *timestr, int is_duration)
Parse a string specifying a time and return its corresponding value as a number of microseconds...
Definition: cmdutils.c:135
void * grow_array(void *array, int elem_size, int *size, int new_size)
Realloc array to hold new_size elements of elem_size.
Definition: cmdutils.c:1912
static OutputStream * new_audio_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
Definition: ffmpeg_opt.c:1309
int avio_open2(AVIOContext **s, const char *url, int flags, const AVIOInterruptCB *int_cb, AVDictionary **options)
Create and initialize a AVIOContext for accessing the resource indicated by url.
Definition: aviobuf.c:804
const char * name
Name of the codec described by this descriptor.
Definition: libavcodec/avcodec.h:516
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
misc parsing utilities
#define type
size_t av_strlcat(char *dst, const char *src, size_t size)
Append the string src to the string dst, but to a total length of no more than size - 1 bytes...
Definition: avstring.c:92
Definition: ffmpeg.h:367
static int opt_channel_layout(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2306
int avformat_seek_file(AVFormatContext *s, int stream_index, int64_t min_ts, int64_t ts, int64_t max_ts, int flags)
Seek to timestamp ts.
Definition: libavformat/utils.c:2146
static int opt_audio_codec(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:192
This struct describes the properties of a single codec described by an AVCodecID. ...
Definition: libavcodec/avcodec.h:508
double parse_number_or_die(const char *context, const char *numstr, int type, double min, double max)
Parse a string and return its corresponding value as a double.
Definition: cmdutils.c:114
static int opt_vstats_file(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2119
static AVCodec * choose_decoder(OptionsContext *o, AVFormatContext *s, AVStream *st)
Definition: ffmpeg_opt.c:545
int global_quality
Global quality for codecs which cannot change it per frame.
Definition: libavcodec/avcodec.h:1208
const char * av_get_media_type_string(enum AVMediaType media_type)
Return a string describing the media_type enum, NULL if media_type is unknown.
Definition: libavutil/utils.c:64
AVCodec * avcodec_find_decoder_by_name(const char *name)
Find a registered decoder with the specified name.
Definition: libavcodec/utils.c:2402
static OutputStream * new_video_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
Definition: ffmpeg_opt.c:1166
static int opt_attach(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:311
static void parse_matrix_coeffs(uint16_t *dest, const char *str)
Definition: ffmpeg_opt.c:1096
int64_t start_time
Decoding: position of the first frame of the component, in AV_TIME_BASE fractional seconds...
Definition: avformat.h:1001
int av_strerror(int errnum, char *errbuf, size_t errbuf_size)
Put a description of the AVERROR code errnum in errbuf.
Definition: error.c:53
static int opt_video_frames(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2137
char * av_strtok(char *s, const char *delim, char **saveptr)
Split the string into several tokens which can be accessed by successive calls to av_strtok()...
Definition: avstring.c:183
int gop_size
the number of pictures in a group of pictures, or 0 for intra_only
Definition: libavcodec/avcodec.h:1321
int av_strstart(const char *str, const char *pfx, const char **ptr)
Return non-zero if pfx is a prefix of str.
Definition: avstring.c:33
Main libavformat public API header.
void print_error(const char *filename, int err)
Print an error message to stderr, indicating filename and a human readable description of the error c...
Definition: cmdutils.c:982
static int get_preset_file_2(const char *preset_name, const char *codec_name, AVIOContext **s)
Definition: ffmpeg_opt.c:923
static int opt_target(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:1980
Definition: avformat.h:918
static int opt_video_standard(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:186
#define AVFMT_NOFILE
Demuxer will use avio_open, no opened file should be provided by the caller.
Definition: avformat.h:345
int avformat_find_stream_info(AVFormatContext *ic, AVDictionary **options)
Read packets of a media file to get stream information.
Definition: libavformat/utils.c:2748
#define AV_OPT_SEARCH_FAKE_OBJ
The obj passed to av_opt_find() is fake – only a double pointer to AVClass instead of a required poi...
Definition: opt.h:547
AVRational time_base
time base in which the start/end timestamps are specified
Definition: avformat.h:920
Definition: avformat.h:377
int avcodec_get_context_defaults3(AVCodecContext *s, const AVCodec *codec)
Set the fields of the given AVCodecContext to default values corresponding to the given codec (defaul...
Definition: libavcodec/options.c:97
void avformat_close_input(AVFormatContext **s)
Close an opened input AVFormatContext.
Definition: libavformat/utils.c:3329
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel layout
Definition: filter_design.txt:12
Definition: ffmpeg.h:309
pixel format definitions
static int opt_timecode(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2295
static void dump_attachment(AVStream *st, const char *filename)
Definition: ffmpeg_opt.c:684
printf("static const uint8_t my_array[100] = {\n")
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip please avoid problems through this extra level of scrutiny For cosmetics only commits you should e g by running git config global user name My Name git config global user email my email which is either set in your personal configuration file through git config core editor or set by one of the following environment VISUAL or EDITOR Log messages should be concise but descriptive Explain why you made a what you did will be obvious from the changes themselves most of the time Saying just bug fix or is bad Remember that people of varying skill levels look at and educate themselves while reading through your code Don t include filenames in log Git provides that information Possibly make the commit message have a descriptive first line
Definition: git-howto.txt:153
uint64_t av_get_channel_layout(const char *name)
Return a channel layout id that matches name, or 0 if no match is found.
Definition: channel_layout.c:132
static int opt_streamid(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:1408
void show_help_children(const AVClass *class, int flags)
Show help for all options with given flags in class and all its children.
Definition: cmdutils.c:176
Definition: ffmpeg.h:200
union SpecifierOpt::@0 u
int64_t duration
Decoding: duration of the stream, in AV_TIME_BASE fractional seconds.
Definition: avformat.h:1009
int read_yesno(void)
Return a positive value if a line read from standard input starts with [yY], otherwise return 0...
Definition: cmdutils.c:1725
union OptionDef::@1 u
Definition: avutil.h:143
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
#define MATCH_PER_TYPE_OPT(name, type, outvar, fmtctx, mediatype)
Definition: ffmpeg_opt.c:56
static int opt_default_new(OptionsContext *o, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2155
enum AVPixelFormat av_get_pix_fmt(const char *name)
Return the pixel format corresponding to name.
Definition: pixdesc.c:1712
static int opt_map(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:216
static int opt_audio_frames(void *optctx, const char *opt, const char *arg)
Definition: ffmpeg_opt.c:2143
Definition: avutil.h:146
enum AVDiscard discard
Selects which packets can be discarded at will and do not need to be demuxed.
Definition: avformat.h:702
static OutputStream * new_attachment_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
Definition: ffmpeg_opt.c:1372
static void uninit_options(OptionsContext *o, int is_input)
Definition: ffmpeg_opt.c:99
int avio_closep(AVIOContext **s)
Close the resource accessed by the AVIOContext *s, free it and set the pointer pointing to it to NULL...
Definition: aviobuf.c:839
Definition: ffmpeg_opt.c:2448
int av_opt_set(void *obj, const char *name, const char *val, int search_flags)
Definition: opt.c:252
enum AVSampleFormat av_get_sample_fmt(const char *name)
Return a sample format corresponding to name, or AV_SAMPLE_FMT_NONE on error.
Definition: samplefmt.c:54
const AVClass * sws_get_class(void)
Get the AVClass for swsContext.
Definition: libswscale/options.c:78
const char program_name[]
program name, defined by the program for show_version().
Definition: ffmpeg.c:106
static OutputStream * new_data_stream(OptionsContext *o, AVFormatContext *oc, int source_index)
Definition: ffmpeg_opt.c:1359
Generated on Tue May 27 2025 07:04:03 for FFmpeg by
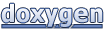