FFmpeg
|
libavcodec/options.c
Go to the documentation of this file.
247 {"best_effort_timestamp", "", FOFFSET(best_effort_timestamp), AV_OPT_TYPE_INT64, {.i64 = AV_NOPTS_VALUE }, INT64_MIN, INT64_MAX, 0},
250 {"sample_aspect_ratio", "", FOFFSET(sample_aspect_ratio), AV_OPT_TYPE_RATIONAL, {.dbl = 0 }, 0, INT_MAX, 0},
254 {"channel_layout", "", FOFFSET(channel_layout), AV_OPT_TYPE_INT64, {.i64 = 0 }, 0, INT64_MAX, 0},
enum AVPixelFormat(* get_format)(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
callback to negotiate the pixelFormat
Definition: libavcodec/avcodec.h:1377
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: opt.h:223
int avcodec_default_execute2(AVCodecContext *c, int(*func)(AVCodecContext *c2, void *arg2, int, int), void *arg, int *ret, int count)
Definition: pixfmt.h:67
memory handling functions
Definition: opt.h:222
void av_opt_set_defaults(void *s)
Set the values of all AVOption fields to their default values.
Definition: opt.c:942
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
int avcodec_copy_context(AVCodecContext *dest, const AVCodecContext *src)
Copy the settings of the source AVCodecContext into the destination AVCodecContext.
Definition: libavcodec/options.c:182
Definition: opt.h:221
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
const AVClass * avcodec_get_class(void)
Get the AVClass for AVCodecContext.
Definition: libavcodec/options.c:239
static const AVClass av_codec_context_class
Definition: libavcodec/options.c:77
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
enum AVPixelFormat avcodec_default_get_format(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
Definition: libavcodec/utils.c:920
Definition: samplefmt.h:50
static const AVOption subtitle_rect_options[]
Definition: libavcodec/options.c:273
AVOptions.
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
static const AVClass av_subtitle_rect_class
Definition: libavcodec/options.c:284
const AVClass * avcodec_get_frame_class(void)
Get the AVClass for AVFrame.
Definition: libavcodec/options.c:266
Definition: log.h:34
const AVCodecDefault * defaults
Private codec-specific defaults.
Definition: libavcodec/avcodec.h:2915
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Definition: libavcodec/internal.h:112
AVCodec * av_codec_next(const AVCodec *c)
If c is NULL, returns the first registered codec, if c is non-NULL, returns the next registered codec...
Definition: libavcodec/utils.c:128
static void * codec_child_next(void *obj, void *prev)
Definition: libavcodec/options.c:46
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
AVClassCategory category
Category used for visualization (like color) This is only set if the category is equal for all object...
Definition: log.h:113
void * thread_opaque
thread opaque Can be used by execute() to store some per AVCodecContext stuff.
Definition: libavcodec/avcodec.h:2643
external API header
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
AVCodecContext * avcodec_alloc_context3(const AVCodec *codec)
Allocate an AVCodecContext and set its fields to default values.
Definition: libavcodec/options.c:148
static const AVClass * codec_child_class_next(const AVClass *prev)
Definition: libavcodec/options.c:54
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
const AVClass * avcodec_get_subtitle_rect_class(void)
Get the AVClass for AVSubtitleRect.
Definition: libavcodec/options.c:291
int64_t reordered_opaque
opaque 64bit number (generally a PTS) that will be reordered and output in AVFrame.reordered_opaque
Definition: libavcodec/avcodec.h:2477
int(* execute2)(struct AVCodecContext *c, int(*func)(struct AVCodecContext *c2, void *arg, int jobnr, int threadnr), void *arg2, int *ret, int count)
The codec may call this to execute several independent things.
Definition: libavcodec/avcodec.h:2635
int avcodec_default_get_buffer2(AVCodecContext *s, AVFrame *frame, int flags)
The default callback for AVCodecContext.get_buffer2().
Definition: libavcodec/utils.c:586
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
int(* get_buffer2)(struct AVCodecContext *s, AVFrame *frame, int flags)
This callback is called at the beginning of each frame to get data buffer(s) for it.
Definition: libavcodec/avcodec.h:2118
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
Definition: log.h:35
int(* decode)(AVCodecContext *, void *outdata, int *outdata_size, AVPacket *avpkt)
Definition: libavcodec/avcodec.h:2937
common internal api header.
int avcodec_get_context_defaults3(AVCodecContext *s, const AVCodec *codec)
Set the fields of the given AVCodecContext to default values corresponding to the given codec (defaul...
Definition: libavcodec/options.c:97
#define alloc_and_copy_or_fail(obj, size, pad)
struct AVCodecInternal * internal
Private context used for internal data.
Definition: libavcodec/avcodec.h:1178
Definition: opt.h:227
Definition: avutil.h:143
Definition: avutil.h:146
int(* execute)(struct AVCodecContext *c, int(*func)(struct AVCodecContext *c2, void *arg), void *arg2, int *ret, int count, int size)
The codec may call this to execute several independent things.
Definition: libavcodec/avcodec.h:2615
int av_opt_set(void *obj, const char *name, const char *val, int search_flags)
Definition: opt.c:252
int avcodec_default_execute(AVCodecContext *c, int(*func)(AVCodecContext *c2, void *arg2), void *arg, int *ret, int count, int size)
Definition: libavcodec/utils.c:890
Generated on Wed Jun 18 2025 06:53:05 for FFmpeg by
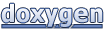