FFmpeg
|
libavcodec/utils.c
Go to the documentation of this file.
82 static inline int ff_fast_malloc(void *ptr, unsigned int *size, size_t min_size, int zero_realloc)
428 int ch = av_frame_get_channels(frame); //av_get_channel_layout_nb_channels(frame->channel_layout);
691 if ((ret = av_image_check_size(avctx->width, avctx->height, 0, avctx)) < 0 || avctx->pix_fmt<0) {
838 if (frame->data[0] && (frame->width != avctx->width || frame->height != avctx->height || frame->format != avctx->pix_fmt)) {
839 av_log(avctx, AV_LOG_WARNING, "Picture changed from size:%dx%d fmt:%s to size:%dx%d fmt:%s in reget buffer()\n",
840 frame->width, frame->height, av_get_pix_fmt_name(frame->format), avctx->width, avctx->height, av_get_pix_fmt_name(avctx->pix_fmt));
890 int avcodec_default_execute(AVCodecContext *c, int (*func)(AVCodecContext *c2, void *arg2), void *arg, int *ret, int count, int size)
902 int avcodec_default_execute2(AVCodecContext *c, int (*func)(AVCodecContext *c2, void *arg2, int jobnr, int threadnr), void *arg, int *ret, int count)
920 enum AVPixelFormat avcodec_default_get_format(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
1006 bit_rate = bits_per_sample ? ctx->sample_rate * ctx->channels * bits_per_sample : ctx->bit_rate;
1022 int attribute_align_arg ff_codec_open2_recursive(AVCodecContext *avctx, const AVCodec *codec, AVDictionary **options)
1034 int attribute_align_arg avcodec_open2(AVCodecContext *avctx, const AVCodec *codec, AVDictionary **options)
1096 // only call avcodec_set_dimensions() for non H.264/VP6F codecs so as not to overwrite previously setup dimensions
1145 codec2 = av_codec_is_encoder(codec) ? avcodec_find_encoder(codec->id) : avcodec_find_decoder(codec->id);
1160 av_log(avctx, AV_LOG_WARNING, "Warning: not compiled with thread support, using thread emulation\n");
1163 ff_unlock_avcodec(); //we will instanciate a few encoders thus kick the counter to prevent false detection of a problem
1276 av_log(avctx, AV_LOG_WARNING, "Bitrate %d is extremely low, maybe you mean %dk\n", avctx->bit_rate, avctx->bit_rate);
1387 av_fast_padded_malloc(&avctx->internal->byte_buffer, &avctx->internal->byte_buffer_size, size);
1525 av_log(avctx, AV_LOG_ERROR, "nb_samples (%d) != frame_size (%d) (avcodec_encode_audio2)\n", frame->nb_samples, avctx->frame_size);
1678 int attribute_align_arg avcodec_encode_video(AVCodecContext *avctx, uint8_t *buf, int buf_size,
1827 if ((ctx->pts_correction_num_faulty_pts<=ctx->pts_correction_num_faulty_dts || dts == AV_NOPTS_VALUE)
1916 if ((avctx->coded_width || avctx->coded_height) && av_image_check_size(avctx->coded_width, avctx->coded_height, 0, avctx))
1924 if ((avctx->codec->capabilities & CODEC_CAP_DELAY) || avpkt->size || (avctx->active_thread_type & FF_THREAD_FRAME)) {
1942 if (!picture->sample_aspect_ratio.num) picture->sample_aspect_ratio = avctx->sample_aspect_ratio;
2534 av_get_channel_layout_string(buf + strlen(buf), buf_size - strlen(buf), enc->channels, enc->channel_layout);
#define WRAP_PLANE(ref_out, data, data_size)
Definition: libavcodec/avcodec.h:367
Definition: libavcodec/avcodec.h:312
enum AVPixelFormat(* get_format)(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
callback to negotiate the pixelFormat
Definition: libavcodec/avcodec.h:1377
Number of sample formats. DO NOT USE if linking dynamically.
Definition: samplefmt.h:63
Definition: libavcodec/avcodec.h:437
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
planar YUV 4:2:2, 18bpp, (1 Cr & Cb sample per 2x1 Y samples), big-endian
Definition: pixfmt.h:158
const AVCodecDescriptor * codec_descriptor
AVCodecDescriptor Code outside libavcodec should access this field using: av_codec_{get,set}_codec_descriptor(avctx)
Definition: libavcodec/avcodec.h:2802
static AVCodec * find_encdec(enum AVCodecID id, int encoder)
Definition: libavcodec/utils.c:2360
Definition: libavcodec/avcodec.h:315
planar YUV 4:4:4,42bpp, (1 Cr & Cb sample per 1x1 Y samples), big-endian
Definition: pixfmt.h:231
Definition: libavcodec/avcodec.h:100
int ff_thread_video_encode_frame(AVCodecContext *avctx, AVPacket *pkt, const AVFrame *frame, int *got_packet_ptr)
Definition: frame_thread_encoder.c:216
Definition: libavcodec/avcodec.h:368
planar YUV 4:2:0,21bpp, (1 Cr & Cb sample per 2x2 Y samples), little-endian
Definition: pixfmt.h:224
int64_t pts_correction_num_faulty_dts
Number of incorrect PTS values so far.
Definition: libavcodec/avcodec.h:2810
void av_buffer_unref(AVBufferRef **buf)
Free a given reference and automatically free the buffer if there are no more references to it...
Definition: libavutil/buffer.c:105
void av_frame_set_best_effort_timestamp(AVFrame *frame, int64_t val)
const AVPixFmtDescriptor * av_pix_fmt_desc_get(enum AVPixelFormat pix_fmt)
Definition: pixdesc.c:1778
Definition: libavcodec/avcodec.h:303
Definition: pixfmt.h:67
void avcodec_default_release_buffer(AVCodecContext *s, AVFrame *pic)
Definition: libavcodec/utils.c:877
AVCodec * avcodec_find_encoder(enum AVCodecID id)
Find a registered encoder with a matching codec ID.
Definition: libavcodec/utils.c:2378
int av_samples_get_buffer_size(int *linesize, int nb_channels, int nb_samples, enum AVSampleFormat sample_fmt, int align)
Get the required buffer size for the given audio parameters.
Definition: samplefmt.c:125
Definition: libavcodec/avcodec.h:344
A dummy id pointing at the start of audio codecs.
Definition: libavcodec/avcodec.h:298
planar YUV 4:2:2,28bpp, (1 Cr & Cb sample per 2x1 Y samples), little-endian
Definition: pixfmt.h:228
planar YUV 4:2:0, 15bpp, (1 Cr & Cb sample per 2x2 Y samples), little-endian
Definition: pixfmt.h:151
Definition: libavcodec/avcodec.h:301
#define CODEC_FLAG_PASS2
Use internal 2pass ratecontrol in second pass mode.
Definition: libavcodec/avcodec.h:705
#define CODEC_CAP_VARIABLE_FRAME_SIZE
Audio encoder supports receiving a different number of samples in each call.
Definition: libavcodec/avcodec.h:826
int coded_width
Bitstream width / height, may be different from width/height e.g.
Definition: libavcodec/avcodec.h:1312
int av_lockmgr_register(int(*cb)(void **mutex, enum AVLockOp op))
Register a user provided lock manager supporting the operations specified by AVLockOp.
Definition: libavcodec/utils.c:2950
planar YUV 4:4:4, 24bpp, (1 Cr & Cb sample per 1x1 Y samples)
Definition: pixfmt.h:73
misc image utilities
Definition: libavcodec/avcodec.h:164
int av_pix_fmt_count_planes(enum AVPixelFormat pix_fmt)
Definition: pixdesc.c:1818
int attribute_align_arg avcodec_decode_audio3(AVCodecContext *avctx, int16_t *samples, int *frame_size_ptr, AVPacket *avpkt)
Definition: libavcodec/utils.c:1985
AVBufferRef * buf[AV_NUM_DATA_POINTERS]
AVBuffer references backing the data for this frame.
Definition: frame.h:343
#define CODEC_FLAG_PASS1
Use internal 2pass ratecontrol in first pass mode.
Definition: libavcodec/avcodec.h:704
planar YUV 4:4:4, 27bpp, (1 Cr & Cb sample per 1x1 Y samples), big-endian
Definition: pixfmt.h:154
int rc_initial_buffer_occupancy
Number of bits which should be loaded into the rc buffer before decoding starts.
Definition: libavcodec/avcodec.h:2233
Definition: libavcodec/avcodec.h:240
planar YUV 4:4:4,36bpp, (1 Cr & Cb sample per 1x1 Y samples), big-endian
Definition: pixfmt.h:229
void av_opt_set_defaults(void *s)
Set the values of all AVOption fields to their default values.
Definition: opt.c:942
const char * avcodec_configuration(void)
Return the libavcodec build-time configuration.
Definition: libavcodec/utils.c:2597
Definition: libavcodec/avcodec.h:157
Definition: libavcodec/avcodec.h:409
void avcodec_set_dimensions(AVCodecContext *s, int width, int height)
Definition: libavcodec/utils.c:177
const char * avcodec_license(void)
Return the libavcodec license.
Definition: libavcodec/utils.c:2602
static int get_buffer_internal(AVCodecContext *avctx, AVFrame *frame, int flags)
Definition: libavcodec/utils.c:686
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
Definition: libavcodec/avcodec.h:112
static int pad_last_frame(AVCodecContext *s, AVFrame **dst, const AVFrame *src)
Pad last frame with silence.
Definition: libavcodec/utils.c:1428
Definition: libavcodec/avcodec.h:424
void avpriv_color_frame(AVFrame *frame, const int c[4])
Definition: libavcodec/utils.c:564
void av_fast_padded_malloc(void *ptr, unsigned int *size, size_t min_size)
Same behaviour av_fast_malloc but the buffer has additional FF_INPUT_BUFFER_PADDING_SIZE at the end w...
Definition: libavcodec/utils.c:101
A dummy ID pointing at the start of various fake codecs.
Definition: libavcodec/avcodec.h:480
planar YUV 4:2:0, 13.5bpp, (1 Cr & Cb sample per 2x2 Y samples), big-endian
Definition: pixfmt.h:148
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
size_t av_get_codec_tag_string(char *buf, size_t buf_size, unsigned int codec_tag)
Put a string representing the codec tag codec_tag in buf.
Definition: libavcodec/utils.c:2436
Definition: libavcodec/avcodec.h:375
int attribute_align_arg avcodec_encode_audio2(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Encode a frame of audio.
Definition: libavcodec/utils.c:1471
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
enum AVPixelFormat ff_thread_get_format(AVCodecContext *avctx, const enum AVPixelFormat *fmt)
Wrapper around get_format() for frame-multithreaded codecs.
Definition: libavcodec/utils.c:3047
int avcodec_decode_subtitle2(AVCodecContext *avctx, AVSubtitle *sub, int *got_sub_ptr, AVPacket *avpkt)
Decode a subtitle message.
Definition: libavcodec/utils.c:2209
Definition: libavcodec/avcodec.h:130
int block_align
number of bytes per packet if constant and known or 0 Used by some WAV based audio codecs...
Definition: libavcodec/avcodec.h:1898
Definition: libavcodec/avcodec.h:391
uint8_t log2_chroma_w
Amount to shift the luma width right to find the chroma width.
Definition: pixdesc.h:66
int av_bprint_finalize(AVBPrint *buf, char **ret_str)
Finalize a print buffer.
Definition: bprint.c:193
Definition: libavcodec/avcodec.h:346
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
Definition: libavcodec/avcodec.h:360
Definition: libavcodec/avcodec.h:402
void av_frame_set_pkt_duration(AVFrame *frame, int64_t val)
int avcodec_fill_audio_frame(AVFrame *frame, int nb_channels, enum AVSampleFormat sample_fmt, const uint8_t *buf, int buf_size, int align)
Fill AVFrame audio data and linesize pointers.
Definition: libavcodec/utils.c:326
Definition: libavcodec/avcodec.h:363
void avcodec_align_dimensions(AVCodecContext *s, int *width, int *height)
Modify width and height values so that they will result in a memory buffer that is acceptable for the...
Definition: libavcodec/utils.c:311
static int volatile entangled_thread_counter
Definition: libavcodec/utils.c:58
void av_fast_malloc(void *ptr, unsigned int *size, size_t min_size)
Allocate a buffer, reusing the given one if large enough.
Definition: libavcodec/utils.c:96
int av_samples_fill_arrays(uint8_t **audio_data, int *linesize, const uint8_t *buf, int nb_channels, int nb_samples, enum AVSampleFormat sample_fmt, int align)
Fill plane data pointers and linesize for samples with sample format sample_fmt.
Definition: samplefmt.c:155
Public dictionary API.
planar YUV 4:2:0, 20bpp, (1 Cr & Cb sample per 2x2 Y & A samples)
Definition: pixfmt.h:105
enum AVPixelFormat avcodec_default_get_format(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
Definition: libavcodec/utils.c:920
AVComponentDescriptor comp[4]
Parameters that describe how pixels are packed.
Definition: pixdesc.h:86
Definition: samplefmt.h:50
AVOptions.
Definition: libavcodec/avcodec.h:340
Definition: libavcodec/avcodec.h:412
int avcodec_default_get_buffer(AVCodecContext *avctx, AVFrame *frame)
Definition: libavcodec/utils.c:661
int avcodec_default_reget_buffer(AVCodecContext *s, AVFrame *pic)
Definition: libavcodec/utils.c:884
planar YUV 4:4:4,36bpp, (1 Cr & Cb sample per 1x1 Y samples), little-endian
Definition: pixfmt.h:230
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
int ff_reget_buffer(AVCodecContext *avctx, AVFrame *frame)
Identical in function to av_frame_make_writable(), except it uses ff_get_buffer() to allocate the buf...
Definition: libavcodec/utils.c:868
Definition: libavcodec/avcodec.h:371
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define LICENSE_PREFIX
struct CompatReleaseBufPriv CompatReleaseBufPriv
Definition: libavcodec/avcodec.h:313
int attribute_align_arg avcodec_encode_video2(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Encode a frame of video.
Definition: libavcodec/utils.c:1713
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
void * frame_thread_encoder
Definition: libavcodec/internal.h:104
int ff_thread_decode_frame(AVCodecContext *avctx, AVFrame *picture, int *got_picture_ptr, AVPacket *avpkt)
Submit a new frame to a decoding thread.
Definition: pthread.c:632
Definition: libavcodec/avcodec.h:325
Definition: libavcodec/avcodec.h:332
void av_frame_move_ref(AVFrame *dst, AVFrame *src)
Move everythnig contained in src to dst and reset src.
Definition: frame.c:352
int attribute_align_arg ff_codec_open2_recursive(AVCodecContext *avctx, const AVCodec *codec, AVDictionary **options)
Call avcodec_open2 recursively by decrementing counter, unlocking mutex, calling the function and the...
Definition: libavcodec/utils.c:1022
void av_register_hwaccel(AVHWAccel *hwaccel)
Register the hardware accelerator hwaccel.
Definition: libavcodec/utils.c:2925
enum AVSampleFormat av_get_planar_sample_fmt(enum AVSampleFormat sample_fmt)
Get the planar alternative form of the given sample format.
Definition: samplefmt.c:82
planar YUV 4:4:0 full scale (JPEG), deprecated in favor of PIX_FMT_YUV440P and setting color_range ...
Definition: pixfmt.h:104
Definition: libavcodec/avcodec.h:434
int ff_init_buffer_info(AVCodecContext *avctx, AVFrame *frame)
does needed setup of pkt_pts/pos and such for (re)get_buffer();
Definition: libavcodec/utils.c:607
Definition: libavcodec/avcodec.h:428
planar YUV 4:2:2, 16bpp, full scale (JPEG), deprecated in favor of PIX_FMT_YUV422P and setting color_...
Definition: pixfmt.h:81
int64_t av_frame_get_pkt_duration(const AVFrame *frame)
int ff_match_2uint16(const uint16_t(*tab)[2], int size, int a, int b)
Return the index into tab at which {a,b} match elements {[0],[1]} of tab.
Definition: libavcodec/utils.c:2889
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
Definition: libavcodec/avcodec.h:110
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
Definition: libavcodec/avcodec.h:318
Definition: libavcodec/avcodec.h:3102
static void compat_release_buffer(void *opaque, uint8_t *data)
Definition: libavcodec/utils.c:679
AVCodec * avcodec_find_encoder_by_name(const char *name)
Find a registered encoder with the specified name.
Definition: libavcodec/utils.c:2383
void avcodec_align_dimensions2(AVCodecContext *s, int *width, int *height, int linesize_align[AV_NUM_DATA_POINTERS])
Modify width and height values so that they will result in a memory buffer that is acceptable for the...
Definition: libavcodec/utils.c:191
Definition: libavcodec/avcodec.h:415
static void * av_x_if_null(const void *p, const void *x)
Return x default pointer in case p is NULL.
Definition: avutil.h:250
int64_t av_rescale_q(int64_t a, AVRational bq, AVRational cq)
Rescale a 64-bit integer by 2 rational numbers.
Definition: mathematics.c:130
planar YUV 4:2:0, 13.5bpp, (1 Cr & Cb sample per 2x2 Y samples), little-endian
Definition: pixfmt.h:149
void av_dict_copy(AVDictionary **dst, AVDictionary *src, int flags)
Copy entries from one AVDictionary struct into another.
Definition: dict.c:176
Libavcodec version macros.
void av_frame_set_pkt_size(AVFrame *frame, int val)
av_cold int avcodec_close(AVCodecContext *avctx)
Close a given AVCodecContext and free all the data associated with it (but not the AVCodecContext its...
Definition: libavcodec/utils.c:2305
int av_new_packet(AVPacket *pkt, int size)
Allocate the payload of a packet and initialize its fields with default values.
Definition: avpacket.c:73
AVRational pkt_timebase
Timebase in which pkt_dts/pts and AVPacket.dts/pts are.
Definition: libavcodec/avcodec.h:2793
const uint64_t * channel_layouts
array of support channel layouts, or NULL if unknown. array is terminated by 0
Definition: libavcodec/avcodec.h:2878
planar YUV 4:4:4, 30bpp, (1 Cr & Cb sample per 1x1 Y samples), little-endian
Definition: pixfmt.h:157
AVHWAccel * av_hwaccel_next(AVHWAccel *hwaccel)
If hwaccel is NULL, returns the first registered hardware accelerator, if hwaccel is non-NULL...
Definition: libavcodec/utils.c:2934
Definition: dict.c:28
planar YUV 4:2:2 24bpp, (1 Cr & Cb sample per 2x1 Y & A samples)
Definition: pixfmt.h:219
Definition: libavcodec/avcodec.h:306
void av_log_missing_feature(void *avc, const char *feature, int want_sample)
Definition: libavcodec/utils.c:2897
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
int av_get_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2690
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
AVDictionary ** avpriv_frame_get_metadatap(AVFrame *frame)
Definition: frame.c:49
uint8_t log2_chroma_h
Amount to shift the luma height right to find the chroma height.
Definition: pixdesc.h:75
int attribute_align_arg avcodec_decode_video2(AVCodecContext *avctx, AVFrame *picture, int *got_picture_ptr, const AVPacket *avpkt)
Decode the video frame of size avpkt->size from avpkt->data into picture.
Definition: libavcodec/utils.c:1901
Definition: libavcodec/avcodec.h:390
#define CODEC_CAP_PARAM_CHANGE
Codec supports changed parameters at any point.
Definition: libavcodec/avcodec.h:818
Multithreading support functions.
void * av_fast_realloc(void *ptr, unsigned int *size, size_t min_size)
Reallocate the given block if it is not large enough, otherwise do nothing.
Definition: libavcodec/utils.c:63
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
Definition: libavcodec/avcodec.h:378
An AV_PKT_DATA_PARAM_CHANGE side data packet is laid out as follows:
Definition: libavcodec/avcodec.h:936
AVCodec * av_codec_next(const AVCodec *c)
If c is NULL, returns the first registered codec, if c is non-NULL, returns the next registered codec...
Definition: libavcodec/utils.c:128
#define CODEC_CAP_SMALL_LAST_FRAME
Codec can be fed a final frame with a smaller size.
Definition: libavcodec/avcodec.h:775
int av_pix_fmt_get_chroma_sub_sample(enum AVPixelFormat pix_fmt, int *h_shift, int *v_shift)
Utility function to access log2_chroma_w log2_chroma_h from the pixel format AVPixFmtDescriptor.
Definition: pixdesc.c:1806
Definition: libavcodec/avcodec.h:239
int64_t pts_correction_last_pts
Number of incorrect DTS values so far.
Definition: libavcodec/avcodec.h:2811
int active_thread_type
Which multithreading methods are in use by the codec.
Definition: libavcodec/avcodec.h:2594
void av_frame_set_pkt_pos(AVFrame *frame, int64_t val)
void av_dict_free(AVDictionary **pm)
Free all the memory allocated for an AVDictionary struct and all keys and values. ...
Definition: dict.c:162
int av_get_channel_layout_nb_channels(uint64_t channel_layout)
Return the number of channels in the channel layout.
Definition: channel_layout.c:191
AVBufferRef * buf
A reference to the reference-counted buffer where the packet data is stored.
Definition: libavcodec/avcodec.h:1034
void av_frame_set_channels(AVFrame *frame, int val)
planar YUV 4:2:2, 20bpp, (1 Cr & Cb sample per 2x1 Y samples), little-endian
Definition: pixfmt.h:153
int av_frame_is_writable(AVFrame *frame)
Check if the frame data is writable.
Definition: frame.c:361
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
planar YUV 4:2:2,28bpp, (1 Cr & Cb sample per 2x1 Y samples), big-endian
Definition: pixfmt.h:227
int64_t av_gcd(int64_t a, int64_t b)
Return the greatest common divisor of a and b.
Definition: mathematics.c:55
enum AVMediaType avcodec_get_type(enum AVCodecID codec_id)
Get the type of the given codec.
Definition: libavcodec/utils.c:3082
int avpriv_set_systematic_pal2(uint32_t pal[256], enum AVPixelFormat pix_fmt)
Definition: imgutils.c:150
AVBufferRef * av_buffer_create(uint8_t *data, int size, void(*free)(void *opaque, uint8_t *data), void *opaque, int flags)
Create an AVBuffer from an existing array.
Definition: libavutil/buffer.c:27
int av_buffer_realloc(AVBufferRef **pbuf, int size)
Reallocate a given buffer.
Definition: libavutil/buffer.c:156
void * thread_opaque
thread opaque Can be used by execute() to store some per AVCodecContext stuff.
Definition: libavcodec/avcodec.h:2643
external API header
AVHWAccel * ff_find_hwaccel(enum AVCodecID codec_id, enum AVPixelFormat pix_fmt)
Return the hardware accelerated codec for codec codec_id and pixel format pix_fmt.
Definition: libavcodec/utils.c:2939
void av_image_copy(uint8_t *dst_data[4], int dst_linesizes[4], const uint8_t *src_data[4], const int src_linesizes[4], enum AVPixelFormat pix_fmt, int width, int height)
Copy image in src_data to dst_data.
Definition: imgutils.c:257
Definition: libavcodec/avcodec.h:464
const AVCodecDescriptor * avcodec_descriptor_get(enum AVCodecID id)
Definition: codec_desc.c:2566
planar YUV 4:2:2, 16bpp, (1 Cr & Cb sample per 2x1 Y samples)
Definition: pixfmt.h:72
int props
Codec properties, a combination of AV_CODEC_PROP_* flags.
Definition: libavcodec/avcodec.h:524
int av_reduce(int *dst_num, int *dst_den, int64_t num, int64_t den, int64_t max)
Reduce a fraction.
Definition: rational.c:36
int ff_frame_thread_encoder_init(AVCodecContext *avctx, AVDictionary *options)
Definition: frame_thread_encoder.c:119
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
#define FF_MAX_EXTRADATA_SIZE
Maximum size in bytes of extradata.
Definition: libavcodec/internal.h:160
AVFrame * avcodec_alloc_frame(void)
Allocate an AVFrame and set its fields to default values.
Definition: libavcodec/utils.c:951
#define FF_COMPLIANCE_EXPERIMENTAL
Allow nonstandardized experimental things.
Definition: libavcodec/avcodec.h:2409
int av_image_check_size(unsigned int w, unsigned int h, int log_offset, void *log_ctx)
Check if the given dimension of an image is valid, meaning that all bytes of the image can be address...
Definition: imgutils.c:231
static int update_frame_pool(AVCodecContext *avctx, AVFrame *frame)
Definition: libavcodec/utils.c:362
Definition: libavcodec/avcodec.h:156
audio channel layout utility functions
enum AVPixelFormat * pix_fmts
array of supported pixel formats, or NULL if unknown, array is terminated by -1
Definition: libavcodec/avcodec.h:2875
int av_frame_get_channels(const AVFrame *frame)
Definition: libavcodec/avcodec.h:314
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
int av_samples_set_silence(uint8_t **audio_data, int offset, int nb_samples, int nb_channels, enum AVSampleFormat sample_fmt)
Fill an audio buffer with silence.
Definition: samplefmt.c:249
#define FF_MIN_BUFFER_SIZE
minimum encoding buffer size Used to avoid some checks during header writing.
Definition: libavcodec/avcodec.h:568
Definition: libavcodec/avcodec.h:411
AVBufferRef ** extended_buf
For planar audio which requires more than AV_NUM_DATA_POINTERS AVBufferRef pointers, this array will hold all the references which cannot fit into AVFrame.buf.
Definition: frame.h:357
planar YUV 4:2:0, 12bpp, full scale (JPEG), deprecated in favor of PIX_FMT_YUV420P and setting color_...
Definition: pixfmt.h:80
int av_get_exact_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2619
static int is_hwaccel_pix_fmt(enum AVPixelFormat pix_fmt)
Definition: libavcodec/utils.c:914
Definition: libavcodec/avcodec.h:146
int ff_alloc_packet2(AVCodecContext *avctx, AVPacket *avpkt, int size)
Check AVPacket size and/or allocate data.
Definition: libavcodec/utils.c:1377
#define CODEC_CAP_AUTO_THREADS
Codec supports avctx->thread_count == 0 (auto).
Definition: libavcodec/avcodec.h:822
Definition: libavcodec/avcodec.h:302
Definition: libavcodec/avcodec.h:321
Definition: libavcodec/avcodec.h:152
void ff_thread_finish_setup(AVCodecContext *avctx)
If the codec defines update_thread_context(), call this when they are ready for the next thread to st...
Definition: libavcodec/utils.c:3063
int attribute_align_arg avcodec_decode_audio4(AVCodecContext *avctx, AVFrame *frame, int *got_frame_ptr, const AVPacket *avpkt)
Decode the audio frame of size avpkt->size from avpkt->data into frame.
Definition: libavcodec/utils.c:2033
const AVProfile * profiles
array of recognized profiles, or NULL if unknown, array is terminated by {FF_PROFILE_UNKNOWN} ...
Definition: libavcodec/avcodec.h:2881
int64_t reordered_opaque
opaque 64bit number (generally a PTS) that will be reordered and output in AVFrame.reordered_opaque
Definition: libavcodec/avcodec.h:2477
static int video_get_buffer(AVCodecContext *s, AVFrame *pic)
Definition: libavcodec/utils.c:511
int refcounted_frames
If non-zero, the decoded audio and video frames returned from avcodec_decode_video2() and avcodec_dec...
Definition: libavcodec/avcodec.h:2131
planar YUV 4:2:0,18bpp, (1 Cr & Cb sample per 2x2 Y samples), little-endian
Definition: pixfmt.h:222
Definition: libavcodec/avcodec.h:449
planar YUV 4:2:0, 15bpp, (1 Cr & Cb sample per 2x2 Y samples), big-endian
Definition: pixfmt.h:150
Definition: thread.h:35
void av_log_ask_for_sample(void *avc, const char *msg,...)
Definition: libavcodec/utils.c:2907
static int audio_get_buffer(AVCodecContext *avctx, AVFrame *frame)
Definition: libavcodec/utils.c:466
#define FF_SUB_CHARENC_MODE_AUTOMATIC
libavcodec will select the mode itself
Definition: libavcodec/avcodec.h:2829
Definition: libavcodec/avcodec.h:343
const char * av_get_sample_fmt_name(enum AVSampleFormat sample_fmt)
Return the name of sample_fmt, or NULL if sample_fmt is not recognized.
Definition: samplefmt.c:47
static int ff_fast_malloc(void *ptr, unsigned int *size, size_t min_size, int zero_realloc)
Definition: libavcodec/utils.c:82
Definition: libavcodec/avcodec.h:300
planar YUV 4:2:2, 18bpp, (1 Cr & Cb sample per 2x1 Y samples), little-endian
Definition: pixfmt.h:159
Definition: libavcodec/avcodec.h:125
Definition: libavcodec/avcodec.h:405
#define AVERROR_EXPERIMENTAL
Requested feature is flagged experimental. Set strict_std_compliance if you really want to use it...
Definition: error.h:72
const char * avcodec_get_name(enum AVCodecID id)
Get the name of a codec.
Definition: libavcodec/utils.c:2416
int thread_count
thread count is used to decide how many independent tasks should be passed to execute() ...
Definition: libavcodec/avcodec.h:2575
Definition: libavcodec/avcodec.h:341
Definition: libavcodec/avcodec.h:145
Definition: libavcodec/utils.c:666
Definition: libavcodec/avcodec.h:357
void avcodec_flush_buffers(AVCodecContext *avctx)
Flush buffers, should be called when seeking or when switching to a different stream.
Definition: libavcodec/utils.c:2608
Definition: libavcodec/avcodec.h:304
int format
format of the frame, -1 if unknown or unset Values correspond to enum AVPixelFormat for video frames...
Definition: frame.h:134
const char * av_get_profile_name(const AVCodec *codec, int profile)
Return a name for the specified profile, if available.
Definition: libavcodec/utils.c:2567
int attribute_align_arg avcodec_encode_video(AVCodecContext *avctx, uint8_t *buf, int buf_size, const AVFrame *pict)
Definition: libavcodec/utils.c:1678
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
av_cold int ff_codec_close_recursive(AVCodecContext *avctx)
Call avcodec_close recursively, counterpart to avcodec_open2_recursive.
Definition: libavcodec/utils.c:2293
int avcodec_default_get_buffer2(AVCodecContext *avctx, AVFrame *frame, int flags)
The default callback for AVCodecContext.get_buffer2().
Definition: libavcodec/utils.c:586
int av_image_fill_pointers(uint8_t *data[4], enum AVPixelFormat pix_fmt, int height, uint8_t *ptr, const int linesizes[4])
Fill plane data pointers for an image with pixel format pix_fmt and height height.
Definition: imgutils.c:108
static int add_metadata_from_side_data(AVCodecContext *avctx, AVFrame *frame)
Definition: libavcodec/utils.c:1878
A list of zero terminated key/value strings.
Definition: libavcodec/avcodec.h:984
Definition: libavcodec/internal.h:56
enum AVCodecID av_get_pcm_codec(enum AVSampleFormat fmt, int be)
Return the PCM codec associated with a sample format.
Definition: libavcodec/utils.c:2669
int av_opt_set_dict(void *obj, AVDictionary **options)
Set all the options from a given dictionary on an object.
Definition: opt.c:1202
#define PIX_FMT_PLANAR
At least one pixel component is not in the first data plane.
Definition: pixdesc.h:93
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
planar YUV 4:4:4 32bpp, (1 Cr & Cb sample per 1x1 Y & A samples)
Definition: pixfmt.h:218
unsigned avcodec_get_edge_width(void)
Return the amount of padding in pixels which the get_buffer callback must provide around the edge of ...
Definition: libavcodec/utils.c:172
Descriptor that unambiguously describes how the bits of a pixel are stored in the up to 4 data planes...
Definition: pixdesc.h:55
AVCodec * avcodec_find_decoder(enum AVCodecID id)
Find a registered decoder with a matching codec ID.
Definition: libavcodec/utils.c:2397
static int recode_subtitle(AVCodecContext *avctx, AVPacket *outpkt, const AVPacket *inpkt)
Definition: libavcodec/utils.c:2152
Definition: libavcodec/avcodec.h:384
void avsubtitle_free(AVSubtitle *sub)
Free all allocated data in the given subtitle struct.
Definition: libavcodec/utils.c:2274
AVRational sample_aspect_ratio
Sample aspect ratio for the video frame, 0/1 if unknown/unspecified.
Definition: frame.h:154
Definition: libavcodec/avcodec.h:305
Definition: libavcodec/avcodec.h:309
int av_samples_copy(uint8_t **dst, uint8_t *const *src, int dst_offset, int src_offset, int nb_samples, int nb_channels, enum AVSampleFormat sample_fmt)
Copy samples from src to dst.
Definition: samplefmt.c:225
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
#define AV_CODEC_PROP_BITMAP_SUB
Subtitle codec is bitmap based Decoded AVSubtitle data can be read from the AVSubtitleRect->pict fiel...
Definition: libavcodec/avcodec.h:546
Definition: libavcodec/avcodec.h:319
void ff_packet_free_side_data(AVPacket *pkt)
Remove and free all side data from packet.
Definition: avpacket.c:31
AVBufferRef * av_buffer_allocz(int size)
Same as av_buffer_alloc(), except the returned buffer will be initialized to zero.
Definition: libavutil/buffer.c:81
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
static void avcodec_get_subtitle_defaults(AVSubtitle *sub)
Definition: libavcodec/utils.c:986
unsigned int av_xiphlacing(unsigned char *s, unsigned int v)
Encode extradata length to a buffer.
Definition: libavcodec/utils.c:2875
#define FF_SUB_CHARENC_MODE_DO_NOTHING
do nothing (demuxer outputs a stream supposed to be already in UTF-8, or the codec is bitmap for inst...
Definition: libavcodec/avcodec.h:2828
planar YUV 4:1:0, 9bpp, (1 Cr & Cb sample per 4x4 Y samples)
Definition: pixfmt.h:74
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
void avcodec_get_frame_defaults(AVFrame *frame)
Set the fields of the given AVFrame to default values.
Definition: libavcodec/utils.c:927
int64_t reordered_opaque
reordered opaque 64bit (generally an integer or a double precision float PTS but can be anything)...
Definition: frame.h:302
int(* func)(AVBPrint *dst, const char *in, const char *arg)
Definition: libavcodec/jacosubdec.c:70
Definition: libavcodec/avcodec.h:324
int(* get_buffer2)(struct AVCodecContext *s, AVFrame *frame, int flags)
This callback is called at the beginning of each frame to get data buffer(s) for it.
Definition: libavcodec/avcodec.h:2118
int av_image_fill_linesizes(int linesizes[4], enum AVPixelFormat pix_fmt, int width)
Fill plane linesizes for an image with pixel format pix_fmt and width width.
Definition: imgutils.c:86
Definition: libavcodec/avcodec.h:403
void av_buffer_pool_uninit(AVBufferPool **ppool)
Mark the pool as being available for freeing.
Definition: libavutil/buffer.c:236
int av_sample_fmt_is_planar(enum AVSampleFormat sample_fmt)
Check if the sample format is planar.
Definition: samplefmt.c:118
void ff_thread_release_buffer(AVCodecContext *avctx, ThreadFrame *f)
Wrapper around release_buffer() frame-for multithreaded codecs.
Definition: libavcodec/utils.c:3058
Recommmends skipping the specified number of samples.
Definition: libavcodec/avcodec.h:968
planar YUV 4:2:0,21bpp, (1 Cr & Cb sample per 2x2 Y samples), big-endian
Definition: pixfmt.h:223
uint16_t step_minus1
Number of elements between 2 horizontally consecutive pixels minus 1.
Definition: pixdesc.h:35
int skip_samples
Number of audio samples to skip at the start of the next decoded frame.
Definition: libavcodec/internal.h:109
Definition: libavcodec/avcodec.h:311
Definition: libavcodec/avcodec.h:336
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
const char * name
Name of the codec described by this descriptor.
Definition: libavcodec/avcodec.h:516
int attribute_align_arg avcodec_open2(AVCodecContext *avctx, const AVCodec *codec, AVDictionary **options)
Initialize the AVCodecContext to use the given AVCodec.
Definition: libavcodec/utils.c:1034
void ff_thread_flush(AVCodecContext *avctx)
Wait for decoding threads to finish and reset internal state.
Definition: pthread.c:931
Definition: libavcodec/avcodec.h:381
Definition: libavcodec/avcodec.h:451
Definition: libavcodec/avcodec.h:372
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int av_get_audio_frame_duration(AVCodecContext *avctx, int frame_bytes)
Return audio frame duration.
Definition: libavcodec/utils.c:2708
Definition: libavcodec/avcodec.h:322
static enum AVCodecID remap_deprecated_codec_id(enum AVCodecID id)
Definition: libavcodec/utils.c:2348
size_t av_strlcat(char *dst, const char *src, size_t size)
Append the string src to the string dst, but to a total length of no more than size - 1 bytes...
Definition: avstring.c:92
This struct describes the properties of a single codec described by an AVCodecID. ...
Definition: libavcodec/avcodec.h:508
int64_t pkt_pts
PTS copied from the AVPacket that was decoded to produce this frame.
Definition: frame.h:164
int avpriv_bprint_to_extradata(AVCodecContext *avctx, struct AVBPrint *buf)
Finalize buf into extradata and set its size appropriately.
Definition: libavcodec/utils.c:3107
const char * av_get_media_type_string(enum AVMediaType media_type)
Return a string describing the media_type enum, NULL if media_type is unknown.
Definition: libavutil/utils.c:64
AVCodec * avcodec_find_decoder_by_name(const char *name)
Find a registered decoder with the specified name.
Definition: libavcodec/utils.c:2402
int av_frame_ref(AVFrame *dst, AVFrame *src)
Setup a new reference to the data described by an given frame.
Definition: frame.c:228
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
Definition: libavcodec/avcodec.h:323
planar YUV 4:4:4, 27bpp, (1 Cr & Cb sample per 1x1 Y samples), little-endian
Definition: pixfmt.h:155
Definition: libavcodec/avcodec.h:320
static int av_toupper(int c)
Locale-independent conversion of ASCII characters to uppercase.
Definition: avstring.h:206
uint8_t max_lowres
maximum value for lowres supported by the decoder
Definition: libavcodec/avcodec.h:2879
int64_t pkt_dts
DTS copied from the AVPacket that triggered returning this frame.
Definition: frame.h:171
AVPacket * pkt
Current packet as passed into the decoder, to avoid having to pass the packet into every function...
Definition: libavcodec/avcodec.h:2776
Definition: libavcodec/avcodec.h:408
static int op(uint8_t **dst, const uint8_t *dst_end, GetByteContext *gb, int pixel, int count, int *x, int width, int linesize)
Perform decode operation.
Definition: libavcodec/anm.c:76
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
planar YUV 4:2:2,24bpp, (1 Cr & Cb sample per 2x1 Y samples), little-endian
Definition: pixfmt.h:226
int attribute_align_arg avcodec_encode_audio(AVCodecContext *avctx, uint8_t *buf, int buf_size, const short *samples)
Definition: libavcodec/utils.c:1600
int(* decode)(AVCodecContext *, void *outdata, int *outdata_size, AVPacket *avpkt)
Definition: libavcodec/avcodec.h:2937
common internal api header.
AVBufferPool * av_buffer_pool_init(int size, AVBufferRef *(*alloc)(int size))
Allocate and initialize a buffer pool.
Definition: libavutil/buffer.c:206
Definition: libavcodec/avcodec.h:310
static void apply_param_change(AVCodecContext *avctx, AVPacket *avpkt)
Definition: libavcodec/utils.c:1836
static int64_t guess_correct_pts(AVCodecContext *ctx, int64_t reordered_pts, int64_t dts)
Attempt to guess proper monotonic timestamps for decoded video frames which might have incorrect time...
Definition: libavcodec/utils.c:1814
void av_fast_padded_mallocz(void *ptr, unsigned int *size, size_t min_size)
Same behaviour av_fast_padded_malloc except that buffer will always be 0-initialized after call...
Definition: libavcodec/utils.c:113
int(* encode_sub)(AVCodecContext *, uint8_t *buf, int buf_size, const struct AVSubtitle *sub)
Definition: libavcodec/avcodec.h:2923
AVBufferRef * av_buffer_ref(AVBufferRef *buf)
Create a new reference to an AVBuffer.
Definition: libavutil/buffer.c:91
#define CODEC_CAP_EXPERIMENTAL
Codec is experimental and is thus avoided in favor of non experimental encoders.
Definition: libavcodec/avcodec.h:796
Definition: libavcodec/avcodec.h:299
planar YUV 4:4:4, 24bpp, full scale (JPEG), deprecated in favor of PIX_FMT_YUV444P and setting color_...
Definition: pixfmt.h:82
int ff_thread_can_start_frame(AVCodecContext *avctx)
Definition: libavcodec/utils.c:3075
enum AVCodecID id
Codec implemented by the hardware accelerator.
Definition: libavcodec/avcodec.h:2969
void ff_thread_report_progress(ThreadFrame *f, int progress, int field)
Notify later decoding threads when part of their reference picture is ready.
Definition: libavcodec/utils.c:3067
planar YUV 4:1:1, 12bpp, (1 Cr & Cb sample per 4x1 Y samples)
Definition: pixfmt.h:75
void av_init_packet(AVPacket *pkt)
Initialize optional fields of a packet with default values.
Definition: avpacket.c:56
int avcodec_default_execute2(AVCodecContext *c, int(*func)(AVCodecContext *c2, void *arg2, int jobnr, int threadnr), void *arg, int *ret, int count)
Definition: libavcodec/utils.c:902
DSP utils.
void avcodec_string(char *buf, int buf_size, AVCodecContext *enc, int encode)
Definition: libavcodec/utils.c:2456
Definition: libavcodec/avcodec.h:450
static int reget_buffer_internal(AVCodecContext *avctx, AVFrame *frame)
Definition: libavcodec/utils.c:831
int(* encode2)(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Encode data to an AVPacket.
Definition: libavcodec/avcodec.h:2935
#define TAG_PRINT(x)
planar YUV 4:4:4,42bpp, (1 Cr & Cb sample per 1x1 Y samples), little-endian
Definition: pixfmt.h:232
Definition: libavcodec/avcodec.h:338
const int * supported_samplerates
array of supported audio samplerates, or NULL if unknown, array is terminated by 0 ...
Definition: libavcodec/avcodec.h:2876
struct AVCodecInternal * internal
Private context used for internal data.
Definition: libavcodec/avcodec.h:1178
unsigned avcodec_version(void)
Return the LIBAVCODEC_VERSION_INT constant.
Definition: libavcodec/utils.c:2580
Definition: libavcodec/avcodec.h:440
Definition: libavcodec/internal.h:37
void avcodec_free_frame(AVFrame **frame)
Free the frame and any dynamically allocated objects in it, e.g.
Definition: libavcodec/utils.c:964
void ff_thread_await_progress(ThreadFrame *f, int progress, int field)
Wait for earlier decoding threads to finish reference pictures.
Definition: libavcodec/utils.c:3071
struct AVPacket::@35 * side_data
Additional packet data that can be provided by the container.
Definition: libavcodec/avcodec.h:316
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
void avcodec_register(AVCodec *codec)
Register the codec codec and initialize libavcodec.
Definition: libavcodec/utils.c:158
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
void ff_frame_thread_encoder_free(AVCodecContext *avctx)
Definition: frame_thread_encoder.c:194
int64_t pts_correction_num_faulty_pts
Current statistics for PTS correction.
Definition: libavcodec/avcodec.h:2809
void(* init_static_data)(struct AVCodec *codec)
Initialize codec static data, called from avcodec_register().
Definition: libavcodec/avcodec.h:2920
Definition: avutil.h:143
uint8_t * av_packet_get_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int *size)
Get side information from packet.
Definition: avpacket.c:289
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
Definition: libavcodec/avcodec.h:307
int ff_thread_ref_frame(ThreadFrame *dst, ThreadFrame *src)
Definition: libavcodec/utils.c:3026
static int(* ff_lockmgr_cb)(void **mutex, enum AVLockOp op)
Definition: libavcodec/utils.c:59
static av_always_inline int64_t ff_samples_to_time_base(AVCodecContext *avctx, int64_t samples)
Rescale from sample rate to AVCodecContext.time_base.
Definition: libavcodec/internal.h:186
AVBufferRef * av_buffer_pool_get(AVBufferPool *pool)
Allocate a new AVBuffer, reusing an old buffer from the pool when available.
Definition: libavutil/buffer.c:329
enum AVSampleFormat * sample_fmts
array of supported sample formats, or NULL if unknown, array is terminated by -1
Definition: libavcodec/avcodec.h:2877
int ff_thread_get_buffer(AVCodecContext *avctx, ThreadFrame *f, int flags)
Wrapper around get_buffer() for frame-multithreaded codecs.
Definition: libavcodec/utils.c:3052
Definition: libavcodec/avcodec.h:436
const char * av_get_pix_fmt_name(enum AVPixelFormat pix_fmt)
Return the short name for a pixel format, NULL in case pix_fmt is unknown.
Definition: pixdesc.c:1700
const uint8_t * avpriv_find_start_code(const uint8_t *av_restrict p, const uint8_t *end, uint32_t *av_restrict state)
Definition: libavcodec/utils.c:3125
Definition: libavcodec/avcodec.h:441
Definition: avutil.h:146
Definition: libavcodec/avcodec.h:195
Definition: libavcodec/avcodec.h:423
Definition: libavcodec/avcodec.h:345
uint8_t * byte_buffer
temporary buffer used for encoders to store their bitstream
Definition: libavcodec/internal.h:101
int avcodec_encode_subtitle(AVCodecContext *avctx, uint8_t *buf, int buf_size, const AVSubtitle *sub)
Definition: libavcodec/utils.c:1790
#define AV_GET_BUFFER_FLAG_REF
The decoder will keep a reference to the frame and may reuse it later.
Definition: libavcodec/avcodec.h:909
int strict_std_compliance
strictly follow the standard (MPEG4, ...).
Definition: libavcodec/avcodec.h:2404
planar YUV 4:2:0,18bpp, (1 Cr & Cb sample per 2x2 Y samples), big-endian
Definition: pixfmt.h:221
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
planar YUV 4:4:4, 30bpp, (1 Cr & Cb sample per 1x1 Y samples), big-endian
Definition: pixfmt.h:156
A dummy ID pointing at the start of subtitle codecs.
Definition: libavcodec/avcodec.h:455
planar YUV 4:2:2,24bpp, (1 Cr & Cb sample per 2x1 Y samples), big-endian
Definition: pixfmt.h:225
Definition: libavcodec/avcodec.h:377
static void compat_free_buffer(void *opaque, uint8_t *data)
Definition: libavcodec/utils.c:671
int avcodec_default_execute(AVCodecContext *c, int(*func)(AVCodecContext *c2, void *arg2), void *arg, int *ret, int count, int size)
Definition: libavcodec/utils.c:890
Definition: libavcodec/avcodec.h:308
#define FF_SUB_CHARENC_MODE_PRE_DECODER
the AVPacket data needs to be recoded to UTF-8 before being fed to the decoder, requires iconv ...
Definition: libavcodec/avcodec.h:2830
Definition: libavcodec/avcodec.h:269
void av_get_channel_layout_string(char *buf, int buf_size, int nb_channels, uint64_t channel_layout)
Return a description of a channel layout.
Definition: channel_layout.c:182
int last_audio_frame
An audio frame with less than required samples has been submitted and padded with silence...
Definition: libavcodec/internal.h:92
uint8_t * subtitle_header
Header containing style information for text subtitles.
Definition: libavcodec/avcodec.h:2759
Definition: libavcodec/avcodec.h:339
planar YUV 4:2:2, 20bpp, (1 Cr & Cb sample per 2x1 Y samples), big-endian
Definition: pixfmt.h:152
Generated on Sat Jul 5 2025 06:54:53 for FFmpeg by
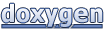