FFmpeg
|
frame_thread_encoder.c
Go to the documentation of this file.
216 int ff_thread_video_encode_frame(AVCodecContext *avctx, AVPacket *pkt, const AVFrame *frame, int *got_packet_ptr){
250 if(!c->finished_tasks[c->finished_task_index].outdata && (c->task_index - c->finished_task_index) % BUFFER_SIZE <= avctx->thread_count)
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
int ff_thread_video_encode_frame(AVCodecContext *avctx, AVPacket *pkt, const AVFrame *frame, int *got_packet_ptr)
Definition: frame_thread_encoder.c:216
static av_always_inline int pthread_mutex_destroy(pthread_mutex_t *mutex)
Definition: os2threads.h:90
struct ThreadContext ThreadContext
static av_always_inline int pthread_cond_wait(pthread_cond_t *cond, pthread_mutex_t *mutex)
Definition: os2threads.h:149
misc image utilities
os2threads to pthreads wrapper
static av_always_inline int pthread_cond_destroy(pthread_cond_t *cond)
Definition: os2threads.h:120
int av_fifo_generic_write(AVFifoBuffer *f, void *src, int size, int(*func)(void *, void *, int))
Feed data from a user-supplied callback to an AVFifoBuffer.
Definition: libavutil/fifo.c:96
#define CODEC_FLAG_INPUT_PRESERVED
The parent program guarantees that the input for B-frames containing streams is not written to for at...
Definition: libavcodec/avcodec.h:703
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
int avcodec_encode_video2(AVCodecContext *avctx, AVPacket *avpkt, const AVFrame *frame, int *got_packet_ptr)
Encode a frame of video.
Definition: libavcodec/utils.c:1713
void * frame_thread_encoder
Definition: libavcodec/internal.h:104
Definition: os2threads.h:41
static av_always_inline int pthread_cond_signal(pthread_cond_t *cond)
Definition: os2threads.h:127
void av_dict_copy(AVDictionary **dst, AVDictionary *src, int flags)
Copy entries from one AVDictionary struct into another.
Definition: dict.c:176
int avcodec_close(AVCodecContext *avctx)
Close a given AVCodecContext and free all the data associated with it (but not the AVCodecContext its...
Definition: libavcodec/utils.c:2305
Definition: dict.c:28
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
Multithreading support functions.
int active_thread_type
Which multithreading methods are in use by the codec.
Definition: libavcodec/avcodec.h:2594
int av_fifo_generic_read(AVFifoBuffer *f, void *dest, int buf_size, void(*func)(void *, void *, int))
Feed data from an AVFifoBuffer to a user-supplied callback.
Definition: libavutil/fifo.c:124
pthread_cond_t finished_task_cond
Definition: frame_thread_encoder.c:58
void av_dict_free(AVDictionary **pm)
Free all the memory allocated for an AVDictionary struct and all keys and values. ...
Definition: dict.c:162
simple assert() macros that are a bit more flexible than ISO C assert().
Definition: frame_thread_encoder.c:48
external API header
void av_image_copy(uint8_t *dst_data[4], int dst_linesizes[4], const uint8_t *src_data[4], const int src_linesizes[4], enum AVPixelFormat pix_fmt, int width, int height)
Copy image in src_data to dst_data.
Definition: imgutils.c:257
int ff_frame_thread_encoder_init(AVCodecContext *avctx, AVDictionary *options)
Definition: frame_thread_encoder.c:119
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
AVCodecContext * avcodec_alloc_context3(const AVCodec *codec)
Allocate an AVCodecContext and set its fields to default values.
Definition: libavcodec/options.c:148
static av_always_inline int pthread_join(pthread_t thread, void **value_ptr)
Definition: os2threads.h:76
static av_always_inline int pthread_mutex_init(pthread_mutex_t *mutex, const pthread_mutexattr_t *attr)
Definition: os2threads.h:83
int thread_count
thread count is used to decide how many independent tasks should be passed to execute() ...
Definition: libavcodec/avcodec.h:2575
Definition: fifo.h:31
static av_always_inline int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void *), void *arg)
Definition: os2threads.h:62
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
a very simple circular buffer FIFO implementation
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
int avcodec_open2(AVCodecContext *avctx, const AVCodec *codec, AVDictionary **options)
Initialize the AVCodecContext to use the given AVCodec.
Definition: libavcodec/utils.c:1034
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
AVFrame * av_frame_alloc(void)
Allocate an AVFrame and set its fields to default values.
Definition: frame.c:95
int av_fifo_size(AVFifoBuffer *f)
Return the amount of data in bytes in the AVFifoBuffer, that is the amount of data you can read from ...
Definition: libavutil/fifo.c:52
common internal api header.
void av_frame_free(AVFrame **frame)
Free the frame and any dynamically allocated objects in it, e.g.
Definition: frame.c:108
static av_always_inline int pthread_cond_init(pthread_cond_t *cond, const pthread_condattr_t *attr)
Definition: os2threads.h:111
void av_init_packet(AVPacket *pkt)
Initialize optional fields of a packet with default values.
Definition: avpacket.c:56
pthread_mutex_t finished_task_mutex
Definition: frame_thread_encoder.c:57
AVFifoBuffer * av_fifo_alloc(unsigned int size)
Initialize an AVFifoBuffer.
Definition: libavutil/fifo.c:25
Definition: w32pthreads.h:46
static av_always_inline int pthread_cond_broadcast(pthread_cond_t *cond)
Definition: os2threads.h:138
static av_always_inline int pthread_mutex_unlock(pthread_mutex_t *mutex)
Definition: os2threads.h:104
struct AVCodecInternal * internal
Private context used for internal data.
Definition: libavcodec/avcodec.h:1178
Definition: frame_thread_encoder.c:41
w32threads to pthreads wrapper
void ff_frame_thread_encoder_free(AVCodecContext *avctx)
Definition: frame_thread_encoder.c:194
static av_always_inline int pthread_mutex_lock(pthread_mutex_t *mutex)
Definition: os2threads.h:97
Generated on Fri Aug 1 2025 06:53:41 for FFmpeg by
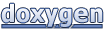