FFmpeg
|
pthread.c
Go to the documentation of this file.
103 pthread_mutex_t progress_mutex; ///< Mutex used to protect frame progress values and progress_cond.
235 c->rets[our_job%c->rets_count] = c->func ? c->func(avctx, (char*)c->args + our_job*c->job_size):
270 static int avcodec_thread_execute(AVCodecContext *avctx, action_func* func, void *arg, int *ret, int job_count, int job_size)
303 static int avcodec_thread_execute2(AVCodecContext *avctx, action_func2* func2, void *arg, int *ret, int job_count)
406 if (p->progress_used[i] && (p->got_frame || p->result<0 || avctx->codec_id != AV_CODEC_ID_H264)) {
427 * @param for_user 0 if the destination is a codec thread, 1 if the destination is the user's thread
1151 "Application has requested %d threads. Using a thread count greater than %d is not recommended.\n",
enum AVPixelFormat(* get_format)(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
callback to negotiate the pixelFormat
Definition: libavcodec/avcodec.h:1377
pthread_cond_t progress_cond
Used by child threads to wait for progress to change.
Definition: pthread.c:99
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
static av_always_inline int pthread_mutex_destroy(pthread_mutex_t *mutex)
Definition: os2threads.h:90
void av_buffer_unref(AVBufferRef **buf)
Free a given reference and automatically free the buffer if there are no more references to it...
Definition: libavutil/buffer.c:105
struct ThreadContext ThreadContext
void ff_thread_await_progress(ThreadFrame *f, int n, int field)
Wait for earlier decoding threads to finish reference pictures.
Definition: pthread.c:723
static av_always_inline int pthread_cond_wait(pthread_cond_t *cond, pthread_mutex_t *mutex)
Definition: os2threads.h:149
Context used by codec threads and stored in their AVCodecContext thread_opaque.
Definition: pthread.c:93
static attribute_align_arg void * frame_worker_thread(void *arg)
Codec worker thread.
Definition: pthread.c:372
int coded_width
Bitstream width / height, may be different from width/height e.g.
Definition: libavcodec/avcodec.h:1312
static int update_context_from_thread(AVCodecContext *dst, AVCodecContext *src, int for_user)
Update the next thread's AVCodecContext with values from the reference thread's context.
Definition: pthread.c:429
static int avcodec_thread_execute(AVCodecContext *avctx, action_func *func, void *arg, int *ret, int job_count, int job_size)
Definition: pthread.c:270
Set before the codec has called ff_thread_finish_setup().
Definition: pthread.c:117
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
os2threads to pthreads wrapper
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
static int thread_get_buffer_internal(AVCodecContext *avctx, ThreadFrame *f, int flags)
Definition: pthread.c:973
int bits_per_raw_sample
Bits per sample/pixel of internal libavcodec pixel/sample format.
Definition: libavcodec/avcodec.h:2553
pthread_cond_t input_cond
Used to wait for a new packet from the main thread.
Definition: pthread.c:98
int( action_func2)(AVCodecContext *c, void *arg, int jobnr, int threadnr)
Definition: pthread.c:70
Definition: libavcodec/avcodec.h:130
static av_always_inline int pthread_cond_destroy(pthread_cond_t *cond)
Definition: os2threads.h:120
void(* draw_horiz_band)(struct AVCodecContext *s, const AVFrame *src, int offset[AV_NUM_DATA_POINTERS], int y, int type, int height)
If non NULL, 'draw_horiz_band' is called by the libavcodec decoder to draw a horizontal band...
Definition: libavcodec/avcodec.h:1364
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
void av_fast_malloc(void *ptr, unsigned int *size, size_t min_size)
Allocate a buffer, reusing the given one if large enough.
Definition: libavcodec/utils.c:96
static int avcodec_thread_execute2(AVCodecContext *avctx, action_func2 *func2, void *arg, int *ret, int job_count)
Definition: pthread.c:303
#define CODEC_FLAG2_CHUNKS
Input bitstream might be truncated at a packet boundaries instead of only at frame boundaries...
Definition: libavcodec/avcodec.h:727
enum AVPixelFormat avcodec_default_get_format(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
Definition: libavcodec/utils.c:920
int ff_thread_decode_frame(AVCodecContext *avctx, AVFrame *picture, int *got_picture_ptr, AVPacket *avpkt)
Submit a new frame to a decoding thread.
Definition: pthread.c:632
void av_frame_move_ref(AVFrame *dst, AVFrame *src)
Move everythnig contained in src to dst and reset src.
Definition: frame.c:352
Definition: os2threads.h:41
static av_always_inline int pthread_cond_signal(pthread_cond_t *cond)
Definition: os2threads.h:127
enum AVPixelFormat ff_thread_get_format(AVCodecContext *avctx, const enum AVPixelFormat *fmt)
Wrapper around get_format() for frame-multithreaded codecs.
Definition: pthread.c:1040
static void copy(LZOContext *c, int cnt)
Copies bytes from input to output buffer with checking.
Definition: lzo.c:79
static av_always_inline void avcodec_thread_park_workers(ThreadContext *c, int thread_count)
Definition: pthread.c:243
int bits_per_coded_sample
bits per sample/pixel from the demuxer (needed for huffyuv).
Definition: libavcodec/avcodec.h:2546
static void validate_thread_parameters(AVCodecContext *avctx)
Set the threading algorithms used.
Definition: pthread.c:1131
enum AVChromaLocation chroma_sample_location
This defines the location of chroma samples.
Definition: libavcodec/avcodec.h:1843
Context stored in the client AVCodecContext thread_opaque.
Definition: pthread.c:147
AVCodecContext * avctx
Context used to decode packets passed to this thread.
Definition: pthread.c:105
void ff_thread_finish_setup(AVCodecContext *avctx)
If the codec defines update_thread_context(), call this when they are ready for the next thread to st...
Definition: pthread.c:741
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
PerThreadContext * prev_thread
The last thread submit_packet() was called on.
Definition: pthread.c:149
uint8_t * buf
backup storage for packet data when the input packet is not refcounted
Definition: pthread.c:108
Multithreading support functions.
void * av_fast_realloc(void *ptr, unsigned int *size, size_t min_size)
Reallocate the given block if it is not large enough, otherwise do nothing.
Definition: libavcodec/utils.c:63
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
int is_copy
Whether the parent AVCodecContext is a copy of the context which had init() called on it...
Definition: libavcodec/internal.h:63
static void frame_thread_free(AVCodecContext *avctx, int thread_count)
Definition: pthread.c:774
int active_thread_type
Which multithreading methods are in use by the codec.
Definition: libavcodec/avcodec.h:2594
static void park_frame_worker_threads(FrameThreadContext *fctx, int thread_count)
Waits for all threads to finish.
Definition: pthread.c:757
AVBufferRef * buf
A reference to the reference-counted buffer where the packet data is stored.
Definition: libavcodec/avcodec.h:1034
simple assert() macros that are a bit more flexible than ISO C assert().
void * thread_opaque
thread opaque Can be used by execute() to store some per AVCodecContext stuff.
Definition: libavcodec/avcodec.h:2643
Definition: frame_thread_encoder.c:48
external API header
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
int dtg_active_format
DTG active format information (additional aspect ratio information only used in DVB MPEG-2 transport ...
Definition: libavcodec/avcodec.h:1598
pthread_cond_t output_cond
Used by the main thread to wait for frames to finish.
Definition: pthread.c:100
#define CODEC_CAP_AUTO_THREADS
Codec supports avctx->thread_count == 0 (auto).
Definition: libavcodec/avcodec.h:822
enum AVColorPrimaries color_primaries
Chromaticity coordinates of the source primaries.
Definition: libavcodec/avcodec.h:1815
static av_always_inline int pthread_join(pthread_t thread, void **value_ptr)
Definition: os2threads.h:76
static av_always_inline int pthread_mutex_init(pthread_mutex_t *mutex, const pthread_mutexattr_t *attr)
Definition: os2threads.h:83
static int update_context_from_user(AVCodecContext *dst, AVCodecContext *src)
Update the next thread's AVCodecContext with values set by the user.
Definition: pthread.c:483
int64_t reordered_opaque
opaque 64bit number (generally a PTS) that will be reordered and output in AVFrame.reordered_opaque
Definition: libavcodec/avcodec.h:2477
int ticks_per_frame
For some codecs, the time base is closer to the field rate than the frame rate.
Definition: libavcodec/avcodec.h:1262
Definition: thread.h:35
int thread_count
thread count is used to decide how many independent tasks should be passed to execute() ...
Definition: libavcodec/avcodec.h:2575
int got_frame
The output of got_picture_ptr from the last avcodec_decode_video() call.
Definition: pthread.c:112
pthread_mutex_t progress_mutex
Mutex used to protect frame progress values and progress_cond.
Definition: pthread.c:103
int(* update_thread_context)(AVCodecContext *dst, const AVCodecContext *src)
Copy necessary context variables from a previous thread context to the current one.
Definition: libavcodec/avcodec.h:2909
static av_always_inline int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void *), void *arg)
Definition: os2threads.h:62
int(* execute2)(struct AVCodecContext *c, int(*func)(struct AVCodecContext *c2, void *arg, int jobnr, int threadnr), void *arg2, int *ret, int count)
The codec may call this to execute several independent things.
Definition: libavcodec/avcodec.h:2635
struct PerThreadContext PerThreadContext
Context used by codec threads and stored in their AVCodecContext thread_opaque.
int avcodec_default_get_buffer2(AVCodecContext *s, AVFrame *frame, int flags)
The default callback for AVCodecContext.get_buffer2().
Definition: libavcodec/utils.c:586
#define FF_THREAD_SLICE
Decode more than one part of a single frame at once.
Definition: libavcodec/avcodec.h:2587
Definition: libavcodec/internal.h:56
AVBufferRef * av_buffer_alloc(int size)
Allocate an AVBuffer of the given size using av_malloc().
Definition: libavutil/buffer.c:65
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
int ff_init_buffer_info(AVCodecContext *s, AVFrame *frame)
does needed setup of pkt_pts/pos and such for (re)get_buffer();
Definition: libavcodec/utils.c:607
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
void avcodec_get_frame_defaults(AVFrame *frame)
Set the fields of the given AVFrame to default values.
Definition: libavcodec/utils.c:927
int(* get_buffer2)(struct AVCodecContext *s, AVFrame *frame, int flags)
This callback is called at the beginning of each frame to get data buffer(s) for it.
Definition: libavcodec/avcodec.h:2118
enum AVColorTransferCharacteristic color_trc
Color Transfer Characteristic.
Definition: libavcodec/avcodec.h:1822
int delaying
Set for the first N packets, where N is the number of threads.
Definition: pthread.c:156
int(* init_thread_copy)(AVCodecContext *)
If defined, called on thread contexts when they are created.
Definition: libavcodec/avcodec.h:2901
enum PerThreadContext::@83 state
#define copy_fields(s, e)
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
void ff_thread_flush(AVCodecContext *avctx)
Wait for decoding threads to finish and reset internal state.
Definition: pthread.c:931
int ff_thread_get_buffer(AVCodecContext *avctx, ThreadFrame *f, int flags)
Wrapper around get_buffer() for frame-multithreaded codecs.
Definition: pthread.c:1066
int allocate_progress
Whether to allocate progress for frame threading.
Definition: libavcodec/internal.h:78
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
AVFrame * released_buffers
Array of frames passed to ff_thread_release_buffer().
Definition: pthread.c:133
Set when the thread is awaiting a packet.
Definition: pthread.c:116
int64_t pkt_dts
DTS copied from the AVPacket that triggered returning this frame.
Definition: frame.h:171
AVPacket * pkt
Current packet as passed into the decoder, to avoid having to pass the packet into every function...
Definition: libavcodec/avcodec.h:2776
#define CODEC_CAP_SLICE_THREADS
Codec supports slice-based (or partition-based) multithreading.
Definition: libavcodec/avcodec.h:814
int(* decode)(AVCodecContext *, void *outdata, int *outdata_size, AVPacket *avpkt)
Definition: libavcodec/avcodec.h:2937
common internal api header.
common internal and external API header
#define CODEC_CAP_FRAME_THREADS
Codec supports frame-level multithreading.
Definition: libavcodec/avcodec.h:810
static int submit_packet(PerThreadContext *p, AVPacket *avpkt)
Definition: pthread.c:546
AVBufferRef * av_buffer_ref(AVBufferRef *buf)
Create a new reference to an AVBuffer.
Definition: libavutil/buffer.c:91
static av_always_inline int pthread_cond_init(pthread_cond_t *cond, const pthread_condattr_t *attr)
Definition: os2threads.h:111
int thread_safe_callbacks
Set by the client if its custom get_buffer() callback can be called synchronously from another thread...
Definition: libavcodec/avcodec.h:2604
struct FrameThreadContext FrameThreadContext
Context stored in the client AVCodecContext thread_opaque.
void ff_thread_report_progress(ThreadFrame *f, int n, int field)
Notify later decoding threads when part of their reference picture is ready.
Definition: pthread.c:705
Definition: w32pthreads.h:46
static av_always_inline int pthread_cond_broadcast(pthread_cond_t *cond)
Definition: os2threads.h:138
static av_always_inline int pthread_mutex_unlock(pthread_mutex_t *mutex)
Definition: os2threads.h:104
struct AVCodecInternal * internal
Private context used for internal data.
Definition: libavcodec/avcodec.h:1178
pthread_mutex_t mutex
Mutex used to protect the contents of the PerThreadContext.
Definition: pthread.c:102
w32threads to pthreads wrapper
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
static void release_delayed_buffers(PerThreadContext *p)
Releases the buffers that this decoding thread was the last user of.
Definition: pthread.c:527
Definition: avutil.h:143
static av_always_inline int pthread_mutex_lock(pthread_mutex_t *mutex)
Definition: os2threads.h:97
void ff_thread_release_buffer(AVCodecContext *avctx, ThreadFrame *f)
Wrapper around release_buffer() frame-for multithreaded codecs.
Definition: pthread.c:1074
int(* execute)(struct AVCodecContext *c, int(*func)(struct AVCodecContext *c2, void *arg), void *arg2, int *ret, int count, int size)
The codec may call this to execute several independent things.
Definition: libavcodec/avcodec.h:2615
int ff_thread_can_start_frame(AVCodecContext *avctx)
Definition: pthread.c:959
void * opaque
Private data of the user, can be used to carry app specific stuff.
Definition: libavcodec/avcodec.h:1185
int avcodec_default_execute(AVCodecContext *c, int(*func)(AVCodecContext *c2, void *arg2), void *arg, int *ret, int count, int size)
Definition: libavcodec/utils.c:890
Generated on Thu Jun 19 2025 06:53:12 for FFmpeg by
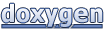