FFmpeg
|
Types and functions for working with AVPacket. More...
![]() |
Data Structures | |
struct | AVPacket |
This structure stores compressed data. More... | |
Macros | |
#define | AV_PKT_FLAG_KEY 0x0001 |
The packet contains a keyframe. More... | |
#define | AV_PKT_FLAG_CORRUPT 0x0002 |
The packet content is corrupted. More... | |
Typedefs | |
typedef struct AVPacket | AVPacket |
This structure stores compressed data. More... | |
Functions | |
void | av_init_packet (AVPacket *pkt) |
Initialize optional fields of a packet with default values. More... | |
int | av_new_packet (AVPacket *pkt, int size) |
Allocate the payload of a packet and initialize its fields with default values. More... | |
void | av_shrink_packet (AVPacket *pkt, int size) |
Reduce packet size, correctly zeroing padding. More... | |
int | av_grow_packet (AVPacket *pkt, int grow_by) |
Increase packet size, correctly zeroing padding. More... | |
int | av_packet_from_data (AVPacket *pkt, uint8_t *data, int size) |
Initialize a reference-counted packet from av_malloc()ed data. More... | |
int | av_dup_packet (AVPacket *pkt) |
int | av_copy_packet (AVPacket *dst, AVPacket *src) |
Copy packet, including contents. More... | |
void | av_free_packet (AVPacket *pkt) |
Free a packet. More... | |
uint8_t * | av_packet_new_side_data (AVPacket *pkt, enum AVPacketSideDataType type, int size) |
Allocate new information of a packet. More... | |
int | av_packet_shrink_side_data (AVPacket *pkt, enum AVPacketSideDataType type, int size) |
Shrink the already allocated side data buffer. More... | |
uint8_t * | av_packet_get_side_data (AVPacket *pkt, enum AVPacketSideDataType type, int *size) |
Get side information from packet. More... | |
int | av_packet_merge_side_data (AVPacket *pkt) |
int | av_packet_split_side_data (AVPacket *pkt) |
Detailed Description
Types and functions for working with AVPacket.
Macro Definition Documentation
#define AV_PKT_FLAG_CORRUPT 0x0002 |
The packet content is corrupted.
Definition at line 1102 of file libavcodec/avcodec.h.
Referenced by aiff_read_packet(), apc_read_packet(), append_packet_chunked(), ff_pcm_read_packet(), ff_read_packet(), handle_packet(), mp3_read_packet(), new_pes_packet(), read_packet(), sol_read_packet(), vorbis_packet(), and vp8_handle_packet().
#define AV_PKT_FLAG_KEY 0x0001 |
The packet contains a keyframe.
Definition at line 1101 of file libavcodec/avcodec.h.
Referenced by a64multi_encode_frame(), ape_tag_read_field(), asf_read_picture(), asf_read_pts(), asf_write_packet(), av_read_frame(), avcodec_encode_audio(), avcodec_encode_audio2(), avcodec_encode_video(), avi_read_packet(), avi_write_packet(), avpriv_dv_produce_packet(), avs_read_audio_packet(), avs_read_video_packet(), avui_encode_frame(), bmp_encode_frame(), cdxl_read_packet(), compute_pkt_fields(), dc1394_read_common(), decode_frame(), dirac_gptopts(), dnxhd_encode_picture(), do_streamcopy(), do_video_out(), dv_extract_audio_info(), dv_write_pack(), ea_read_packet(), encode_frame(), encode_picture_lossless(), encode_picture_ls(), ff_asf_parse_packet(), ff_id3v2_parse_apic(), ff_mov_add_hinted_packet(), ff_mov_write_packet(), ff_MPV_encode_picture(), ff_rm_parse_packet(), ff_rm_retrieve_cache(), ff_subtitles_queue_insert(), ffm_read_packet(), ffm_write_packet(), flashsv2_encode_frame(), flashsv_decode_frame(), flashsv_encode_frame(), flv_data_packet(), flv_read_packet(), flv_write_packet(), framecrc_write_packet(), get_attachment(), get_needed_flags(), gif_encode_frame(), gif_read_packet(), h263_handle_packet(), hls_read_packet(), hls_write_packet(), http_prepare_data(), idcin_read_packet(), iff_read_packet(), img_read_packet(), ism_write_packet(), libopenjpeg_encode_frame(), libschroedinger_encode_frame(), lmlm4_read_packet(), lvf_read_packet(), lxf_read_packet(), main(), matroska_parse_block(), mkv_write_packet(), mkv_write_packet_internal(), modplug_read_packet(), mov_create_chapter_track(), mov_create_timecode_track(), mov_parse_vc1_frame(), mov_read_covr(), mov_read_packet(), mov_write_single_packet(), mpeg_mux_write_packet(), mpegts_write_flush(), mpegts_write_packet_internal(), msnwc_tcp_read_packet(), mv_read_packet(), nsv_read_chunk(), nut_read_packet(), nut_write_packet(), nuv_packet(), ogg_read_packet(), ogg_read_timestamp(), ogg_validate_keyframe(), ogg_write_packet(), ogm_packet(), old_dirac_gptopts(), pam_encode_frame(), parse_packet(), parse_picture(), pcx_encode_frame(), pkt_dump_internal(), pnm_encode_frame(), prores_encode_frame(), put_payload_header(), qt_rtp_parse_packet(), qtrle_encode_frame(), raw_encode(), read_frame(), read_frame_internal(), read_packet(), rm_write_audio(), rm_write_video(), roq_encode_frame(), rpl_read_packet(), search_hi_lo_keyframes(), seek_frame_generic(), seg_write_packet(), show_packet(), siff_read_packet(), smush_read_packet(), Stagefright_decode_frame(), storeframe(), sunrast_encode_frame(), svq1_encode_frame(), targa_encode_frame(), theora_gptopts(), tmv_read_packet(), utvideo_encode_frame(), v308_encode_frame(), v408_encode_frame(), v410_encode_frame(), vc1t_read_packet(), vc1test_write_packet(), vid_read_packet(), write_frame(), write_timestamp(), write_video_frame(), X264_frame(), XAVS_frame(), xbm_encode_frame(), xface_encode_frame(), xmv_fetch_video_packet(), xvid_encode_frame(), xwd_encode_frame(), y41p_encode_frame(), yop_read_packet(), yuv4_encode_frame(), and zerocodec_decode_frame().
Typedef Documentation
This structure stores compressed data.
It is typically exported by demuxers and then passed as input to decoders, or received as output from encoders and then passed to muxers.
For video, it should typically contain one compressed frame. For audio it may contain several compressed frames.
AVPacket is one of the few structs in FFmpeg, whose size is a part of public ABI. Thus it may be allocated on stack and no new fields can be added to it without libavcodec and libavformat major bump.
The semantics of data ownership depends on the buf or destruct (deprecated) fields. If either is set, the packet data is dynamically allocated and is valid indefinitely until av_free_packet() is called (which in turn calls av_buffer_unref()/the destruct callback to free the data). If neither is set, the packet data is typically backed by some static buffer somewhere and is only valid for a limited time (e.g. until the next read call when demuxing).
The side data is always allocated with av_malloc() and is freed in av_free_packet().
Enumeration Type Documentation
enum AVPacketSideDataType |
Definition at line 917 of file libavcodec/avcodec.h.
Enumerator | |
---|---|
AV_SIDE_DATA_PARAM_CHANGE_CHANNEL_COUNT | |
AV_SIDE_DATA_PARAM_CHANGE_CHANNEL_LAYOUT | |
AV_SIDE_DATA_PARAM_CHANGE_SAMPLE_RATE | |
AV_SIDE_DATA_PARAM_CHANGE_DIMENSIONS |
Definition at line 1104 of file libavcodec/avcodec.h.
Function Documentation
Copy packet, including contents.
- Returns
- 0 on success, negative AVERROR on fail
Definition at line 236 of file avpacket.c.
Referenced by aiff_write_packet(), ff_subtitles_queue_read_packet(), flush_packet(), gif_write_packet(), read_thread(), tee_write_packet(), and write_packet().
int av_dup_packet | ( | AVPacket * | pkt | ) |
- Warning
- This is a hack - the packet memory allocation stuff is broken. The packet is allocated if it was not really allocated.
Definition at line 221 of file avpacket.c.
Referenced by av_read_frame(), avcodec_encode_audio2(), avcodec_encode_video2(), avformat_find_stream_info(), ff_interleave_add_packet(), input_thread(), packet_queue_put(), parse_packet(), tee_write_packet(), and worker().
Free a packet.
- Parameters
-
pkt packet to free
Definition at line 242 of file avpacket.c.
Referenced by adx_read_packet(), aiff_write_trailer(), amr_read_packet(), append_packet_chunked(), asf_read_header(), asf_read_picture(), asf_read_pts(), asf_reset_header(), asfrtp_parse_packet(), audio_decode_frame(), audio_encode_example(), audio_read_packet(), av_interleaved_write_frame(), av_packet_merge_side_data(), av_write_trailer(), avcodec_decode_subtitle2(), avcodec_encode_audio2(), avcodec_encode_video2(), avi_read_close(), avi_read_packet(), avs_read_video_packet(), cdxl_read_packet(), cin_read_packet(), dfa_read_packet(), do_audio_out(), do_video_out(), dxa_read_packet(), ea_read_packet(), encode_frame(), estimate_timings_from_pts(), extract_mpeg4_header(), ff_asf_parse_packet(), ff_free_stream(), ff_raw_read_partial_packet(), ff_read_packet(), ff_rm_free_rmstream(), ff_spdif_read_packet(), ff_subtitles_queue_clean(), ffm_read_packet(), flic_read_packet(), flush_packet(), fourxm_read_packet(), free_input_threads(), free_packet_buffer(), free_packet_list(), free_pkt_fifo(), free_variant_list(), g723_1_read_packet(), gsm_read_packet(), hls_read_seek(), http_prepare_data(), idcin_read_packet(), ilbc_read_packet(), img_read_packet(), interleave_packet(), load_ipmovie_packet(), lxf_read_packet(), main(), matroska_clear_queue(), matroska_decode_buffer(), matroska_parse_frame(), mkv_write_packet(), mkv_write_trailer(), mmap_read_frame(), modplug_read_packet(), mov_write_subtitle_end_packet(), movie_push_frame(), mp3_queue_flush(), mpc_read_packet(), mpc_read_seek(), mpegts_get_dts(), mpegts_raw_read_packet(), mpegts_read_packet(), mxf_interleave_get_packet(), nc_read_packet(), nsv_read_close(), nuv_packet(), packet_queue_flush(), packet_queue_put(), parse_packet(), process_input(), pulse_read_packet(), qt_rtp_parse_packet(), read_frame_internal(), read_packet(), read_packets(), read_thread(), recode_subtitle(), rl2_read_packet(), rm_assemble_video_frame(), rm_read_packet(), rpl_read_packet(), sap_fetch_packet(), search_hi_lo_keyframes(), seek_frame_generic(), seek_subtitle(), str_read_close(), str_read_packet(), stream_component_close(), subtitle_thread(), thp_read_packet(), video_encode_example(), video_thread(), vivo_read_packet(), vmd_read_packet(), vqf_read_packet(), wc3_read_close(), write_frame(), write_trailer(), wv_read_packet(), wv_read_seek(), xiph_handle_packet(), xvid_encode_frame(), yop_read_close(), yop_read_packet(), and yop_read_seek().
int av_grow_packet | ( | AVPacket * | pkt, |
int | grow_by | ||
) |
Increase packet size, correctly zeroing padding.
- Parameters
-
pkt packet grow_by number of bytes by which to increase the size of the packet
Definition at line 105 of file avpacket.c.
Referenced by append_packet_chunked(), ff_subtitles_queue_insert(), and matroska_decode_buffer().
Initialize optional fields of a packet with default values.
Note, this does not touch the data and size members, which have to be initialized separately.
- Parameters
-
pkt packet
Definition at line 56 of file avpacket.c.
Referenced by audio_decode_example(), audio_encode_example(), av_get_packet(), av_new_packet(), avcodec_encode_audio(), avcodec_encode_audio2(), avcodec_encode_video(), avcodec_encode_video2(), avformat_find_stream_info(), avi_write_packet(), avpriv_dv_produce_packet(), dc1394_read_common(), do_audio_out(), do_streamcopy(), do_subtitle_out(), do_video_out(), dv_extract_audio_info(), encode_frame(), ff_alloc_packet2(), ff_id3v2_parse_apic(), ff_interleave_packet_per_dts(), ff_mov_add_hinted_packet(), ff_read_packet(), ff_rtp_finalize_packet(), flush_encoders(), main(), mkv_write_header(), mov_write_subtitle_end_packet(), movie_push_frame(), mpegts_get_dts(), mpegts_write_packet_internal(), mxf_interleave_get_packet(), new_pes_packet(), output_packet(), parse_packet(), parse_picture(), qt_rtp_parse_packet(), read_frame_internal(), read_packets(), read_thread(), reset_packet(), sp5x_decode_frame(), v4l2_read_packet(), video_decode_example(), video_encode_example(), wc3_read_header(), worker(), write_audio_frame(), write_video_frame(), and x11grab_read_packet().
int av_new_packet | ( | AVPacket * | pkt, |
int | size | ||
) |
Allocate the payload of a packet and initialize its fields with default values.
- Parameters
-
pkt packet size wanted payload size
- Returns
- 0 if OK, AVERROR_xxx otherwise
Definition at line 73 of file avpacket.c.
Referenced by aac_parse_packet(), amr_handle_packet(), amr_read_packet(), ape_read_packet(), audio_read_packet(), av_grow_packet(), avs_read_video_packet(), bmv_read_packet(), callback(), cdxl_read_packet(), cin_read_packet(), decode_frame(), dxa_read_packet(), fbdev_read_packet(), ff_alloc_packet2(), ff_asf_parse_packet(), ff_h263_handle_packet(), ff_interleave_new_audio_packet(), ff_raw_read_partial_packet(), ff_rm_retrieve_cache(), ff_spdif_read_packet(), ff_subtitles_queue_insert(), ffm_read_packet(), film_read_packet(), flic_read_packet(), fourxm_read_packet(), g723_1_read_packet(), grab_read_packet(), h264_handle_packet(), iff_read_packet(), ilbc_read_packet(), img_read_packet(), latm_parse_packet(), lavfi_read_packet(), load_ipmovie_packet(), lxf_read_packet(), matroska_parse_frame(), matroska_parse_rm_audio(), mmap_read_frame(), modplug_read_packet(), mpc_read_packet(), mpeg_parse_packet(), mpegts_raw_read_packet(), nut_read_packet(), nuv_packet(), ogg_read_packet(), pulse_read_packet(), qdm2_restore_block(), qt_rtp_parse_packet(), read_frame(), read_packet(), recode_subtitle(), return_stored_frame(), rm_assemble_video_frame(), rm_read_audio_stream_info(), roq_read_packet(), rtp_parse_packet_internal(), sbg_read_packet(), seq_read_packet(), siff_read_packet(), smacker_read_packet(), store_packet(), str_read_packet(), supply_new_packets(), swf_read_packet(), videostream_cb(), vmd_read_packet(), vqf_read_packet(), wsaud_read_packet(), wv_read_packet(), xiph_handle_packet(), and yop_read_packet().
Initialize a reference-counted packet from av_malloc()ed data.
- Parameters
-
pkt packet to be initialized. This function will set the data, size, buf and destruct fields, all others are left untouched. data Data allocated by av_malloc() to be used as packet data. If this function returns successfully, the data is owned by the underlying AVBuffer. The caller may not access the data through other means. size size of data in bytes, without the padding. I.e. the full buffer size is assumed to be size + FF_INPUT_BUFFER_PADDING_SIZE.
- Returns
- 0 on success, a negative AVERROR on error
Definition at line 136 of file avpacket.c.
Referenced by ff_rtp_finalize_packet(), and qt_rtp_parse_packet().
uint8_t* av_packet_get_side_data | ( | AVPacket * | pkt, |
enum AVPacketSideDataType | type, | ||
int * | size | ||
) |
Get side information from packet.
- Parameters
-
pkt packet type desired side information type size pointer for side information size to store (optional)
- Returns
- pointer to data if present or NULL otherwise
Definition at line 289 of file avpacket.c.
Referenced by aac_decode_frame(), aac_decode_frame_int(), add_metadata_from_side_data(), apply_param_change(), avcodec_decode_audio4(), cinepak_decode_frame(), decode_frame(), decode_tag(), flush_packet(), idcin_decode_frame(), ipvideo_decode_frame(), msrle_decode_frame(), msvideo1_decode_frame(), qtrle_decode_frame(), raw_decode(), rtp_write_packet(), smc_decode_frame(), srt_decode_frame(), and srt_write_packet().
int av_packet_merge_side_data | ( | AVPacket * | pkt | ) |
Definition at line 306 of file avpacket.c.
Referenced by avcodec_encode_video2(), ff_read_packet(), and split_write_packet().
uint8_t* av_packet_new_side_data | ( | AVPacket * | pkt, |
enum AVPacketSideDataType | type, | ||
int | size | ||
) |
Allocate new information of a packet.
- Parameters
-
pkt packet type side information type size side information size
- Returns
- pointer to fresh allocated data or NULL otherwise
Definition at line 264 of file avpacket.c.
Referenced by av_read_frame(), avi_read_packet(), ff_add_param_change(), ff_asf_parse_packet(), ff_MPV_encode_picture(), flv_read_packet(), gif_encode_frame(), gif_image_write_image(), idcin_read_packet(), lavfi_read_packet(), load_ipmovie_packet(), matroska_parse_frame(), mov_read_packet(), read_frame(), srt_read_header(), and swf_read_packet().
int av_packet_shrink_side_data | ( | AVPacket * | pkt, |
enum AVPacketSideDataType | type, | ||
int | size | ||
) |
Shrink the already allocated side data buffer.
- Parameters
-
pkt packet type side information type size new side information size
- Returns
- 0 on success, < 0 on failure
Definition at line 386 of file avpacket.c.
Referenced by ff_MPV_encode_picture().
int av_packet_split_side_data | ( | AVPacket * | pkt | ) |
Definition at line 344 of file avpacket.c.
Referenced by avcodec_decode_audio4(), avcodec_decode_subtitle2(), avcodec_decode_video2(), and split_write_packet().
Reduce packet size, correctly zeroing padding.
- Parameters
-
pkt packet size new size
Definition at line 97 of file avpacket.c.
Referenced by append_packet_chunked(), cdxl_read_packet(), cin_read_packet(), ff_asf_parse_packet(), ff_raw_read_partial_packet(), libopus_encode(), mxf_decrypt_triplet(), mxf_get_d10_aes3_packet(), nuv_packet(), and yop_read_packet().
Generated on Wed Apr 16 2025 06:53:30 for FFmpeg by
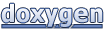