FFmpeg
|
ffprobe.c
Go to the documentation of this file.
82 #define SECTION_FLAG_IS_WRAPPER 1 ///< the section only contains other sections, but has no data at its own level
84 #define SECTION_FLAG_HAS_VARIABLE_FIELDS 4 ///< the section may contain a variable number of fields with variable keys.
86 int flags;
118 [SECTION_ID_FORMAT_TAGS] = { SECTION_ID_FORMAT_TAGS, "tags", SECTION_FLAG_HAS_VARIABLE_FIELDS, { -1 }, .element_name = "tag", .unique_name = "format_tags" },
119 [SECTION_ID_FRAMES] = { SECTION_ID_FRAMES, "frames", SECTION_FLAG_IS_ARRAY, { SECTION_ID_FRAME, -1 } },
121 [SECTION_ID_FRAME_TAGS] = { SECTION_ID_FRAME_TAGS, "tags", SECTION_FLAG_HAS_VARIABLE_FIELDS, { -1 }, .element_name = "tag", .unique_name = "frame_tags" },
122 [SECTION_ID_LIBRARY_VERSIONS] = { SECTION_ID_LIBRARY_VERSIONS, "library_versions", SECTION_FLAG_IS_ARRAY, { SECTION_ID_LIBRARY_VERSION, -1 } },
124 [SECTION_ID_PACKETS] = { SECTION_ID_PACKETS, "packets", SECTION_FLAG_IS_ARRAY, { SECTION_ID_PACKET, -1} },
125 [SECTION_ID_PACKETS_AND_FRAMES] = { SECTION_ID_PACKETS_AND_FRAMES, "packets_and_frames", SECTION_FLAG_IS_ARRAY, { SECTION_ID_PACKET, -1} },
131 [SECTION_ID_STREAMS] = { SECTION_ID_STREAMS, "streams", SECTION_FLAG_IS_ARRAY, { SECTION_ID_STREAM, -1 } },
132 [SECTION_ID_STREAM] = { SECTION_ID_STREAM, "stream", 0, { SECTION_ID_STREAM_DISPOSITION, SECTION_ID_STREAM_TAGS, -1 } },
133 [SECTION_ID_STREAM_DISPOSITION] = { SECTION_ID_STREAM_DISPOSITION, "disposition", 0, { -1 }, .unique_name = "stream_disposition" },
134 [SECTION_ID_STREAM_TAGS] = { SECTION_ID_STREAM_TAGS, "tags", SECTION_FLAG_HAS_VARIABLE_FIELDS, { -1 }, .element_name = "tag", .unique_name = "stream_tags" },
260 AVBPrint section_pbuf[SECTION_MAX_NB_LEVELS]; ///< generic print buffer dedicated to each section,
263 unsigned int nb_section_packet; ///< number of the packet section in case we are in "packets_and_frames" section
264 unsigned int nb_section_frame; ///< number of the frame section in case we are in "packets_and_frames" section
265 unsigned int nb_section_packet_frame; ///< nb_section_packet or nb_section_frame according if is_packets_and_frames
525 { "noprint_wrappers", "do not print headers and footers", OFFSET(noprint_wrappers), AV_OPT_TYPE_INT, {.i64=0}, 0, 1 },
526 { "nw", "do not print headers and footers", OFFSET(noprint_wrappers), AV_OPT_TYPE_INT, {.i64=0}, 0, 1 },
639 static const char *csv_escape_str(AVBPrint *dst, const char *src, const char sep, void *log_ctx)
657 static const char *none_escape_str(AVBPrint *dst, const char *src, const char sep, void *log_ctx)
677 {"item_sep", "set item separator", OFFSET(item_sep_str), AV_OPT_TYPE_STRING, {.str="|"}, CHAR_MIN, CHAR_MAX },
678 {"s", "set item separator", OFFSET(item_sep_str), AV_OPT_TYPE_STRING, {.str="|"}, CHAR_MIN, CHAR_MAX },
681 {"escape", "set escape mode", OFFSET(escape_mode_str), AV_OPT_TYPE_STRING, {.str="c"}, CHAR_MIN, CHAR_MAX },
682 {"e", "set escape mode", OFFSET(escape_mode_str), AV_OPT_TYPE_STRING, {.str="c"}, CHAR_MIN, CHAR_MAX },
683 {"print_section", "print section name", OFFSET(print_section), AV_OPT_TYPE_INT, {.i64=1}, 0, 1 },
695 av_log(wctx, AV_LOG_ERROR, "Item separator '%s' specified, but must contain a single character\n",
782 {"item_sep", "set item separator", OFFSET(item_sep_str), AV_OPT_TYPE_STRING, {.str=","}, CHAR_MIN, CHAR_MAX },
783 {"s", "set item separator", OFFSET(item_sep_str), AV_OPT_TYPE_STRING, {.str=","}, CHAR_MIN, CHAR_MAX },
786 {"escape", "set escape mode", OFFSET(escape_mode_str), AV_OPT_TYPE_STRING, {.str="csv"}, CHAR_MIN, CHAR_MAX },
787 {"e", "set escape mode", OFFSET(escape_mode_str), AV_OPT_TYPE_STRING, {.str="csv"}, CHAR_MIN, CHAR_MAX },
788 {"print_section", "print section name", OFFSET(print_section), AV_OPT_TYPE_INT, {.i64=1}, 0, 1 },
820 {"sep_char", "set separator", OFFSET(sep_str), AV_OPT_TYPE_STRING, {.str="."}, CHAR_MIN, CHAR_MAX },
822 {"hierarchical", "specify if the section specification should be hierarchical", OFFSET(hierarchical), AV_OPT_TYPE_INT, {.i64=1}, 0, 1 },
823 {"h", "specify if the section specification should be hierarchical", OFFSET(hierarchical), AV_OPT_TYPE_INT, {.i64=1}, 0, 1 },
834 av_log(wctx, AV_LOG_ERROR, "Item separator '%s' specified, but must contain a single character\n",
927 .flags = WRITER_FLAG_DISPLAY_OPTIONAL_FIELDS|WRITER_FLAG_PUT_PACKETS_AND_FRAMES_IN_SAME_CHAPTER,
942 {"hierarchical", "specify if the section specification should be hierarchical", OFFSET(hierarchical), AV_OPT_TYPE_INT, {.i64=1}, 0, 1 },
943 {"h", "specify if the section specification should be hierarchical", OFFSET(hierarchical), AV_OPT_TYPE_INT, {.i64=1}, 0, 1 },
1031 .flags = WRITER_FLAG_DISPLAY_OPTIONAL_FIELDS|WRITER_FLAG_PUT_PACKETS_AND_FRAMES_IN_SAME_CHAPTER,
1211 {"fully_qualified", "specify if the output should be fully qualified", OFFSET(fully_qualified), AV_OPT_TYPE_INT, {.i64=0}, 0, 1 },
1212 {"q", "specify if the output should be fully qualified", OFFSET(fully_qualified), AV_OPT_TYPE_INT, {.i64=0}, 0, 1 },
1213 {"xsd_strict", "ensure that the output is XSD compliant", OFFSET(xsd_strict), AV_OPT_TYPE_INT, {.i64=0}, 0, 1 },
1214 {"x", "ensure that the output is XSD compliant", OFFSET(xsd_strict), AV_OPT_TYPE_INT, {.i64=0}, 0, 1 },
1229 "XSD-compliant output selected but option '%s' was selected, XML output may be non-compliant.\n" \
1422 static void show_packet(WriterContext *w, AVFormatContext *fmt_ctx, AVPacket *pkt, int packet_idx)
1476 print_duration_time("pkt_duration_time", av_frame_get_pkt_duration(frame), &stream->time_base);
1477 if (av_frame_get_pkt_pos (frame) != -1) print_fmt ("pkt_pos", "%"PRId64, av_frame_get_pkt_pos(frame));
1479 if (av_frame_get_pkt_size(frame) != -1) print_fmt ("pkt_size", "%d", av_frame_get_pkt_size(frame));
1708 if (nb_streams_frames[stream_idx]) print_fmt ("nb_read_frames", "%"PRIu64, nb_streams_frames[stream_idx]);
1710 if (nb_streams_packets[stream_idx]) print_fmt ("nb_read_packets", "%"PRIu64, nb_streams_packets[stream_idx]);
2188 "set the output printing format (available formats are: default, compact, csv, flat, ini, json, xml)", "format" },
2190 { "select_streams", OPT_STRING | HAS_ARG, {(void*)&stream_specifier}, "select the specified streams", "stream_specifier" },
2191 { "sections", OPT_EXIT, {.func_arg = opt_sections}, "print sections structure and section information, and exit" },
2202 { "count_frames", OPT_BOOL, {(void*)&do_count_frames}, "count the number of frames per stream" },
2203 { "count_packets", OPT_BOOL, {(void*)&do_count_packets}, "count the number of packets per stream" },
2210 { "default", HAS_ARG | OPT_AUDIO | OPT_VIDEO | OPT_EXPERT, {.func_arg = opt_default}, "generic catch all option", "" },
2305 av_log(NULL, AV_LOG_ERROR, "Use -h to get full help or, even better, run 'man %s'.\n", program_name);
codec_id is not known (like AV_CODEC_ID_NONE) but lavf should attempt to identify it ...
Definition: libavcodec/avcodec.h:490
const struct section * section[SECTION_MAX_NB_LEVELS]
section per each level
Definition: ffprobe.c:259
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
static char * value_string(char *buf, int buf_size, struct unit_value uv)
Definition: ffprobe.c:167
unsigned int nb_item[SECTION_MAX_NB_LEVELS]
number of the item printed in the given section, starting from 0
Definition: ffprobe.c:256
Definition: libavcodec/avcodec.h:100
void(* print_string)(WriterContext *wctx, const char *, const char *)
Definition: ffprobe.c:238
Definition: ffprobe.c:933
static void show_stream(WriterContext *w, AVFormatContext *fmt_ctx, int stream_idx)
Definition: ffprobe.c:1594
static void json_print_int(WriterContext *wctx, const char *key, long long int value)
Definition: ffprobe.c:1170
static void default_print_section_header(WriterContext *wctx)
Definition: ffprobe.c:544
Definition: dict.h:80
static int opt_input_file_i(void *optctx, const char *opt, const char *arg)
Definition: ffprobe.c:2100
static int opt_format(void *optctx, const char *opt, const char *arg)
Definition: ffprobe.c:1980
static void writer_print_rational(WriterContext *wctx, const char *key, AVRational q, char sep)
Definition: ffprobe.c:410
static int opt_show_format_entry(void *optctx, const char *opt, const char *arg)
Definition: ffprobe.c:2074
int avformat_open_input(AVFormatContext **ps, const char *filename, AVInputFormat *fmt, AVDictionary **options)
Open an input stream and read the header.
Definition: libavformat/utils.c:614
static const char * xml_escape_str(AVBPrint *dst, const char *src, void *log_ctx)
Definition: ffprobe.c:1248
static void json_print_section_footer(WriterContext *wctx)
Definition: ffprobe.c:1125
Definition: opt.h:222
static void writer_print_integer(WriterContext *wctx, const char *key, long long int val)
Definition: ffprobe.c:385
AVRational av_guess_sample_aspect_ratio(AVFormatContext *format, AVStream *stream, AVFrame *frame)
Guess the sample aspect ratio of a frame, based on both the stream and the frame aspect ratio...
Definition: libavformat/utils.c:4268
void av_opt_set_defaults(void *s)
Set the values of all AVOption fields to their default values.
Definition: opt.c:942
int repeat_pict
When decoding, this signals how much the picture must be delayed.
Definition: frame.h:265
int av_set_options_string(void *ctx, const char *opts, const char *key_val_sep, const char *pairs_sep)
Parse the key/value pairs list in opts.
Definition: opt.c:1062
void show_banner(int argc, char **argv, const OptionDef *options)
Print the program banner to stderr.
Definition: cmdutils.c:1055
Definition: cmdutils.h:144
Definition: avisynth_c.h:503
static int writer_open(WriterContext **wctx, const Writer *writer, const char *args, const struct section *sections, int nb_sections)
Definition: ffprobe.c:298
void avdevice_register_all(void)
Initialize libavdevice and register all the input and output devices.
Definition: alldevices.c:40
size_t av_get_codec_tag_string(char *buf, size_t buf_size, unsigned int codec_tag)
Put a string representing the codec tag codec_tag in buf.
Definition: libavcodec/utils.c:2436
static void json_print_item_str(WriterContext *wctx, const char *key, const char *value)
Definition: ffprobe.c:1147
Definition: avformat.h:456
Timecode helpers header.
static void show_frame(WriterContext *w, AVFrame *frame, AVStream *stream, AVFormatContext *fmt_ctx)
Definition: ffprobe.c:1457
static const char * json_escape_str(AVBPrint *dst, const char *src, void *log_ctx)
Definition: ffprobe.c:1065
static void xml_print_section_footer(WriterContext *wctx)
Definition: ffprobe.c:1308
static void mark_section_show_entries(SectionID section_id, int show_all_entries, AVDictionary *entries)
Definition: ffprobe.c:1990
void av_log_set_callback(void(*callback)(void *, int, const char *, va_list))
Definition: log.c:279
AVDictionaryEntry * av_dict_get(AVDictionary *m, const char *key, const AVDictionaryEntry *prev, int flags)
Get a dictionary entry with matching key.
Definition: dict.c:39
Definition: ffprobe.c:97
AVDictionary * filter_codec_opts(AVDictionary *opts, enum AVCodecID codec_id, AVFormatContext *s, AVStream *st, AVCodec *codec)
Filter out options for given codec.
Definition: cmdutils.c:1836
int av_bprint_finalize(AVBPrint *buf, char **ret_str)
Finalize a print buffer.
Definition: bprint.c:193
char * av_timecode_make_mpeg_tc_string(char *buf, uint32_t tc25bit)
Get the timecode string from the 25-bit timecode format (MPEG GOP format).
Definition: libavutil/timecode.c:130
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
#define AV_DICT_DONT_STRDUP_KEY
Take ownership of a key that's been allocated with av_malloc() and children.
Definition: dict.h:69
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
static void writer_print_section_header(WriterContext *wctx, int section_id)
Definition: ffprobe.c:344
static void compact_print_section_footer(WriterContext *wctx)
Definition: ffprobe.c:732
Public dictionary API.
unsigned int nb_section_packet
number of the packet section in case we are in "packets_and_frames" section
Definition: ffprobe.c:263
static void ini_print_section_header(WriterContext *wctx)
Definition: ffprobe.c:976
Definition: ffprobe.c:662
Definition: ffprobe.c:78
void log_callback_help(void *ptr, int level, const char *fmt, va_list vl)
Trivial log callback.
Definition: cmdutils.c:95
int opt_default(void *optctx, const char *opt, const char *arg)
Fallback for options that are not explicitly handled, these will be parsed through AVOptions...
Definition: cmdutils.c:483
Definition: ffprobe.c:111
Definition: ffprobe.c:244
AVOptions.
int flags
Can use flags: AVFMT_NOFILE, AVFMT_NEEDNUMBER, AVFMT_SHOW_IDS, AVFMT_GENERIC_INDEX, AVFMT_TS_DISCONT, AVFMT_NOBINSEARCH, AVFMT_NOGENSEARCH, AVFMT_NO_BYTE_SEEK, AVFMT_SEEK_TO_PTS.
Definition: avformat.h:475
Definition: ffprobe.c:109
static const char * flat_escape_value_str(AVBPrint *dst, const char *src)
Definition: ffprobe.c:858
static void writer_print_time(WriterContext *wctx, const char *key, int64_t ts, const AVRational *time_base, int is_duration)
Definition: ffprobe.c:419
static void show_streams(WriterContext *w, AVFormatContext *fmt_ctx)
Definition: ffprobe.c:1744
static void compact_print_section_header(WriterContext *wctx)
Definition: ffprobe.c:712
int avformat_match_stream_specifier(AVFormatContext *s, AVStream *st, const char *spec)
Check if the stream st contained in s is matched by the stream specifier spec.
Definition: libavformat/utils.c:4307
#define AV_LOG_SKIP_REPEATED
Skip repeated messages, this requires the user app to use av_log() instead of (f)printf as the 2 woul...
Definition: log.h:219
static int match_section(const char *section_name, int show_all_entries, AVDictionary *entries)
Definition: ffprobe.c:2005
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
void init_opts(void)
Initialize the cmdutils option system, in particular allocate the *_opts contexts.
Definition: cmdutils.c:74
Definition: ffprobe.c:226
Definition: opt.h:226
#define PRINT_DISPOSITION(flagname, name)
void parse_options(void *optctx, int argc, char **argv, const OptionDef *options, void(*parse_arg_function)(void *, const char *))
Definition: cmdutils.c:345
int avformat_network_init(void)
Do global initialization of network components.
Definition: libavformat/utils.c:4196
char av_get_picture_type_char(enum AVPictureType pict_type)
Return a single letter to describe the given picture type pict_type.
Definition: libavutil/utils.c:76
static int check_section_show_entries(int section_id)
Definition: ffprobe.c:2215
int64_t av_frame_get_pkt_duration(const AVFrame *frame)
const AVClass * avformat_get_class(void)
Get the AVClass for AVFormatContext.
Definition: libavformat/options.c:120
void parse_loglevel(int argc, char **argv, const OptionDef *options)
Find the '-loglevel' option in the command line args and apply it.
Definition: cmdutils.c:459
external API header
void show_help_default(const char *opt, const char *arg)
Per-fftool specific help handler.
Definition: ffprobe.c:2106
void show_help_options(const OptionDef *options, const char *msg, int req_flags, int rej_flags, int alt_flags)
Print help for all options matching specified flags.
Definition: cmdutils.c:147
static const char * c_escape_str(AVBPrint *dst, const char *src, const char sep, void *log_ctx)
Apply C-language-like string escaping.
Definition: ffprobe.c:616
static void xml_print_str(WriterContext *wctx, const char *key, const char *value)
Definition: ffprobe.c:1327
int children_ids[SECTION_MAX_NB_CHILDREN+1]
list of children section IDS, terminated by -1
Definition: ffprobe.c:87
int duration
Duration of this packet in AVStream->time_base units, 0 if unknown.
Definition: libavcodec/avcodec.h:1073
struct DefaultContext DefaultContext
static void json_print_section_header(WriterContext *wctx)
Definition: ffprobe.c:1087
void(* print_section_footer)(WriterContext *wctx)
Definition: ffprobe.c:235
static void ffprobe_show_program_version(WriterContext *w)
Definition: ffprobe.c:1933
Definition: ffprobe.c:102
AVDictionary ** setup_find_stream_info_opts(AVFormatContext *s, AVDictionary *codec_opts)
Setup AVCodecContext options for avformat_find_stream_info().
Definition: cmdutils.c:1892
Definition: ffprobe.c:112
static void * av_x_if_null(const void *p, const void *x)
Return x default pointer in case p is NULL.
Definition: avutil.h:250
void av_dump_format(AVFormatContext *ic, int index, const char *url, int is_output)
Definition: libavformat/utils.c:3599
void av_dict_copy(AVDictionary **dst, AVDictionary *src, int flags)
Copy entries from one AVDictionary struct into another.
Definition: dict.c:176
Main libavdevice API header.
int avcodec_close(AVCodecContext *avctx)
Close a given AVCodecContext and free all the data associated with it (but not the AVCodecContext its...
Definition: libavcodec/utils.c:2305
libswresample public header
static int opt_show_versions(const char *opt, const char *arg)
Definition: ffprobe.c:2154
Definition: dict.c:28
Definition: ffprobe.c:108
const int program_birth_year
program birth year, defined by the program for show_banner()
Definition: ffprobe.c:48
int av_get_bits_per_sample(enum AVCodecID codec_id)
Return codec bits per sample.
Definition: libavcodec/utils.c:2690
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static void read_packets(WriterContext *w, AVFormatContext *fmt_ctx)
Definition: ffprobe.c:1561
int avcodec_decode_video2(AVCodecContext *avctx, AVFrame *picture, int *got_picture_ptr, const AVPacket *avpkt)
Decode the video frame of size avpkt->size from avpkt->data into picture.
Definition: libavcodec/utils.c:1901
#define AV_BPRINT_SIZE_UNLIMITED
Convenience macros for special values for av_bprint_init() size_max parameter.
Definition: bprint.h:89
static int opt_pretty(void *optctx, const char *opt, const char *arg)
Definition: ffprobe.c:2116
void av_bprint_init(AVBPrint *buf, unsigned size_init, unsigned size_max)
Init a print buffer.
Definition: bprint.c:68
#define SECTION_FLAG_HAS_VARIABLE_FIELDS
the section may contain a variable number of fields with variable keys.
Definition: ffprobe.c:84
int64_t convergence_duration
Time difference in AVStream->time_base units from the pts of this packet to the point at which the ou...
Definition: libavcodec/avcodec.h:1099
Definition: ffprobe.c:162
void av_dict_free(AVDictionary **pm)
Free all the memory allocated for an AVDictionary struct and all keys and values. ...
Definition: dict.c:162
const AVOption * av_opt_next(void *obj, const AVOption *last)
Iterate over all AVOptions belonging to obj.
Definition: opt.c:63
static void default_print_int(WriterContext *wctx, const char *key, long long int value)
Definition: ffprobe.c:591
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
Definition: ffprobe.c:100
static void flat_print_str(WriterContext *wctx, const char *key, const char *value)
Definition: ffprobe.c:907
external API header
char * av_get_token(const char **buf, const char *term)
Unescape the given string until a non escaped terminating char, and return the token corresponding to...
Definition: avstring.c:148
Definition: ffprobe.c:1037
int av_reduce(int *dst_num, int *dst_den, int64_t num, int64_t den, int64_t max)
Reduce a fraction.
Definition: rational.c:36
#define SECTION_FLAG_IS_WRAPPER
the section only contains other sections, but has no data at its own level
Definition: ffprobe.c:82
static void writer_print_data(WriterContext *wctx, const char *name, uint8_t *data, int size)
Definition: ffprobe.c:445
static void show_format(WriterContext *w, AVFormatContext *fmt_ctx)
Definition: ffprobe.c:1754
AVInputFormat * av_find_input_format(const char *short_name)
Find AVInputFormat based on the short name of the input format.
Definition: libavformat/utils.c:248
Definition: ffprobe.c:107
AVBPrint section_pbuf[SECTION_MAX_NB_LEVELS]
generic print buffer dedicated to each section, used by various writers
Definition: ffprobe.c:260
int av_frame_get_channels(const AVFrame *frame)
static const char * csv_escape_str(AVBPrint *dst, const char *src, const char sep, void *log_ctx)
Quote fields containing special characters, check RFC4180.
Definition: ffprobe.c:639
static const Writer * registered_writers[MAX_REGISTERED_WRITERS_NB+1]
Definition: ffprobe.c:475
static void writer_print_string(WriterContext *wctx, const char *key, const char *val, int opt)
Definition: ffprobe.c:396
struct FlatContext FlatContext
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
Definition: ffprobe.c:99
static int probe_file(WriterContext *wctx, const char *filename)
Definition: ffprobe.c:1869
int avcodec_decode_audio4(AVCodecContext *avctx, AVFrame *frame, int *got_frame_ptr, const AVPacket *avpkt)
Decode the audio frame of size avpkt->size from avpkt->data into frame.
Definition: libavcodec/utils.c:2033
static void flat_print_int(WriterContext *wctx, const char *key, long long int value)
Definition: ffprobe.c:902
static int opt_sections(void *optctx, const char *opt, const char *arg)
Definition: ffprobe.c:2142
#define WRITER_FLAG_DISPLAY_OPTIONAL_FIELDS
Definition: ffprobe.c:223
struct Writer Writer
const char * av_get_sample_fmt_name(enum AVSampleFormat sample_fmt)
Return the name of sample_fmt, or NULL if sample_fmt is not recognized.
Definition: samplefmt.c:47
Definition: ffprobe.c:98
static void compact_print_str(WriterContext *wctx, const char *key, const char *value)
Definition: ffprobe.c:741
const char * long_name
Descriptive name for the format, meant to be more human-readable than name.
Definition: avformat.h:468
const char program_name[]
program name, defined by the program for show_version().
Definition: ffprobe.c:47
static void compact_print_int(WriterContext *wctx, const char *key, long long int value)
Definition: ffprobe.c:754
int format
format of the frame, -1 if unknown or unset Values correspond to enum AVPixelFormat for video frames...
Definition: frame.h:134
const char * av_get_profile_name(const AVCodec *codec, int profile)
Return a name for the specified profile, if available.
Definition: libavcodec/utils.c:2567
int avformat_network_deinit(void)
Undo the initialization done by avformat_network_init.
Definition: libavformat/utils.c:4208
external API header
const char * long_name
Descriptive name for the codec, meant to be more human readable than name.
Definition: libavcodec/avcodec.h:2866
unsigned int nb_section_frame
number of the frame section in case we are in "packets_and_frames" section
Definition: ffprobe.c:264
#define ERROR(...)
Definition: ffprobe.c:96
int av_frame_get_pkt_size(const AVFrame *frame)
static void xml_print_int(WriterContext *wctx, const char *key, long long int value)
Definition: ffprobe.c:1350
AVCodec * avcodec_find_decoder(enum AVCodecID id)
Find a registered decoder with a matching codec ID.
Definition: libavcodec/utils.c:2397
static void ini_print_str(WriterContext *wctx, const char *key, const char *value)
Definition: ffprobe.c:1009
unsigned int codec_tag
fourcc (LSB first, so "ABCD" -> ('D'<<24) + ('C'<<16) + ('B'<<8) + 'A').
Definition: libavcodec/avcodec.h:1160
#define WRITER_FLAG_PUT_PACKETS_AND_FRAMES_IN_SAME_CHAPTER
Definition: ffprobe.c:224
AVDictionary * av_frame_get_metadata(const AVFrame *frame)
unsigned int nb_section_packet_frame
nb_section_packet or nb_section_frame according if is_packets_and_frames
Definition: ffprobe.c:265
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
Replacements for frequently missing libm functions.
void avcodec_get_frame_defaults(AVFrame *frame)
Set the fields of the given AVFrame to default values.
Definition: libavcodec/utils.c:927
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
size_t av_strlcatf(char *dst, size_t size, const char *fmt,...)
Definition: avstring.c:100
Definition: ffprobe.c:110
int avcodec_open2(AVCodecContext *avctx, const AVCodec *codec, AVDictionary **options)
Initialize the AVCodecContext to use the given AVCodec.
Definition: libavcodec/utils.c:1034
void(* print_section_header)(WriterContext *wctx)
Definition: ffprobe.c:234
#define SECTION_FLAG_IS_ARRAY
the section contains an array of elements of the same type
Definition: ffprobe.c:83
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int av_read_frame(AVFormatContext *s, AVPacket *pkt)
Return the next frame of a stream.
Definition: libavformat/utils.c:1530
int64_t pkt_pts
PTS copied from the AVPacket that was decoded to produce this frame.
Definition: frame.h:164
static void writer_print_ts(WriterContext *wctx, const char *key, int64_t ts, int is_duration)
Definition: ffprobe.c:436
void * av_calloc(size_t nmemb, size_t size)
Allocate a block of nmemb * size bytes with alignment suitable for all memory accesses (including vec...
Definition: mem.c:213
const char * av_get_media_type_string(enum AVMediaType media_type)
Return a string describing the media_type enum, NULL if media_type is unknown.
Definition: libavutil/utils.c:64
static void ffprobe_show_library_versions(WriterContext *w)
Definition: ffprobe.c:1966
Definition: ffprobe.c:106
void(* print_integer)(WriterContext *wctx, const char *, long long int)
Definition: ffprobe.c:236
int64_t start_time
Decoding: position of the first frame of the component, in AV_TIME_BASE fractional seconds...
Definition: avformat.h:1001
static void flat_print_section_header(WriterContext *wctx)
Definition: ffprobe.c:876
int av_strerror(int errnum, char *errbuf, size_t errbuf_size)
Put a description of the AVERROR code errnum in errbuf.
Definition: error.c:53
static char * upcase_string(char *dst, size_t dst_size, const char *src)
Definition: ffprobe.c:535
void av_bprint_clear(AVBPrint *buf)
Reset the string to "" but keep internal allocated data.
Definition: bprint.c:185
char * av_strtok(char *s, const char *delim, char **saveptr)
Split the string into several tokens which can be accessed by successive calls to av_strtok()...
Definition: avstring.c:183
Definition: ffprobe.c:95
static int av_toupper(int c)
Locale-independent conversion of ASCII characters to uppercase.
Definition: avstring.h:206
int64_t pkt_dts
DTS copied from the AVPacket that triggered returning this frame.
Definition: frame.h:171
Main libavformat public API header.
void print_error(const char *filename, int err)
Print an error message to stderr, indicating filename and a human readable description of the error c...
Definition: cmdutils.c:982
static void xml_print_section_header(WriterContext *wctx)
Definition: ffprobe.c:1268
static void default_print_str(WriterContext *wctx, const char *key, const char *value)
Definition: ffprobe.c:582
const char *(* escape_str)(AVBPrint *dst, const char *src, const char sep, void *log_ctx)
Definition: ffprobe.c:669
int avformat_find_stream_info(AVFormatContext *ic, AVDictionary **options)
Read packets of a media file to get stream information.
Definition: libavformat/utils.c:2748
Definition: ffprobe.c:104
int64_t start_time
Decoding: pts of the first frame of the stream in presentation order, in stream time base...
Definition: avformat.h:689
Definition: ffprobe.c:105
static void show_tags(WriterContext *wctx, AVDictionary *tags, int section_id)
Definition: ffprobe.c:1410
static void show_packet(WriterContext *w, AVFormatContext *fmt_ctx, AVPacket *pkt, int packet_idx)
Definition: ffprobe.c:1422
void av_init_packet(AVPacket *pkt)
Initialize optional fields of a packet with default values.
Definition: avpacket.c:56
Definition: ffprobe.c:809
void uninit_opts(void)
Uninitialize the cmdutils option system, in particular free the *_opts contexts and their contents...
Definition: cmdutils.c:82
struct AVInputFormat * iformat
Can only be iformat or oformat, not both at the same time.
Definition: avformat.h:957
static const char * flat_escape_key_str(AVBPrint *dst, const char *src, const char sep)
Definition: ffprobe.c:843
void avformat_close_input(AVFormatContext **s)
Close an opened input AVFormatContext.
Definition: libavformat/utils.c:3329
Definition: ffprobe.c:1199
struct CompactContext CompactContext
static int opt_show_entries(void *optctx, const char *opt, const char *arg)
Definition: ffprobe.c:2024
int av_opt_get(void *obj, const char *name, int search_flags, uint8_t **out_val)
Definition: opt.c:566
int top_field_first
If the content is interlaced, is top field displayed first.
Definition: frame.h:275
static av_always_inline int process_frame(WriterContext *w, AVFormatContext *fmt_ctx, AVFrame *frame, AVPacket *pkt)
Definition: ffprobe.c:1528
printf("static const uint8_t my_array[100] = {\n")
static int open_input_file(AVFormatContext **fmt_ctx_ptr, const char *filename)
Definition: ffprobe.c:1793
#define CHECK_COMPLIANCE(opt, opt_name)
int64_t av_frame_get_pkt_pos(const AVFrame *frame)
static const char * none_escape_str(AVBPrint *dst, const char *src, const char sep, void *log_ctx)
Definition: ffprobe.c:657
void show_help_children(const AVClass *class, int flags)
Show help for all options with given flags in class and all its children.
Definition: cmdutils.c:176
static void json_print_str(WriterContext *wctx, const char *key, const char *value)
Definition: ffprobe.c:1159
static void ini_print_int(WriterContext *wctx, const char *key, long long int value)
Definition: ffprobe.c:1020
union unit_value::@26 val
int64_t dts
Decompression timestamp in AVStream->time_base units; the time at which the packet is decompressed...
Definition: libavcodec/avcodec.h:1050
int bit_rate
Decoding: total stream bitrate in bit/s, 0 if not available.
Definition: avformat.h:1016
int64_t duration
Decoding: duration of the stream, in AV_TIME_BASE fractional seconds.
Definition: avformat.h:1009
int64_t av_frame_get_channel_layout(const AVFrame *frame)
static void default_print_section_footer(WriterContext *wctx)
Definition: ffprobe.c:569
Definition: avutil.h:143
Definition: ffprobe.c:515
const char * av_get_pix_fmt_name(enum AVPixelFormat pix_fmt)
Return the short name for a pixel format, NULL in case pix_fmt is unknown.
Definition: pixdesc.c:1700
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: avformat.h:679
Definition: ffprobe.c:101
static const Writer * writer_get_by_name(const char *name)
Definition: ffprobe.c:488
void av_register_all(void)
Initialize libavformat and register all the muxers, demuxers and protocols.
Definition: allformats.c:52
#define DEFINE_OPT_SHOW_SECTION(section, target_section_id)
Definition: ffprobe.c:2161
int64_t pts
Presentation timestamp in AVStream->time_base units; the time at which the decompressed packet will b...
Definition: libavcodec/avcodec.h:1044
static void writer_print_section_footer(WriterContext *wctx)
Definition: ffprobe.c:368
Definition: ffprobe.c:103
void av_bprint_channel_layout(struct AVBPrint *bp, int nb_channels, uint64_t channel_layout)
Append a description of a channel layout to a bprint buffer.
Definition: channel_layout.c:148
void av_bprint_chars(AVBPrint *buf, char c, unsigned n)
Append char c n times to a print buffer.
Definition: bprint.c:116
Generated on Wed May 7 2025 06:52:40 for FFmpeg by
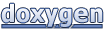