FFmpeg
|
avformat.h
Go to the documentation of this file.
336 unsigned char *buf; /**< Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero. */
340 #define AVPROBE_SCORE_MAX 100 ///< maximum score, half of that is used for file-extension-based detection
341 #define AVPROBE_SCORE_RETRY (AVPROBE_SCORE_MAX/4)
353 #define AVFMT_TS_DISCONT 0x0200 /**< Format allows timestamp discontinuities. Note, muxers always require valid (monotone) timestamps */
357 #define AVFMT_NOBINSEARCH 0x2000 /**< Format does not allow to fallback to binary search via read_timestamp */
360 #define AVFMT_ALLOW_FLUSH 0x10000 /**< Format allows flushing. If not set, the muxer will not receive a NULL packet in the write_packet function. */
362 #define AVFMT_TS_NONSTRICT 0x8020000 //we try to be compatible to the ABIs of ffmpeg and major forks
445 int64_t *dts, int64_t *wall);
553 int64_t *pos, int64_t pos_limit);
573 int (*read_seek2)(struct AVFormatContext *s, int stream_index, int64_t min_ts, int64_t ts, int64_t max_ts, int flags);
583 AVSTREAM_PARSE_TIMESTAMPS, /**< full parsing and interpolation of timestamps for frames not starting on a packet boundary */
584 AVSTREAM_PARSE_FULL_ONCE, /**< full parsing and repack of the first frame only, only implemented for H.264 currently */
585 AVSTREAM_PARSE_FULL_RAW=MKTAG(0,'R','A','W'), /**< full parsing and repack with timestamp and position generation by parser for raw
591 int64_t pos;
593 * Timestamp in AVStream.time_base units, preferably the time from which on correctly decoded frames are available
595 * But demuxers can choose to store a different timestamp, if it is more convenient for the implementation or nothing better
600 int size:30; //Yeah, trying to keep the size of this small to reduce memory requirements (it is 24 vs. 32 bytes due to possible 8-byte alignment).
601 int min_distance; /**< Minimum distance between this and the previous keyframe, used to avoid unneeded searching. */
701 enum AVDiscard discard; ///< Selects which packets can be discarded at will and do not need to be demuxed.
753 int64_t duration_gcd;
756 int64_t codec_info_duration;
757 int64_t codec_info_duration_fields;
767 int64_t fps_last_dts;
783 int64_t first_dts;
784 int64_t cur_dts;
785 int64_t last_IP_pts;
807 int64_t interleaver_chunk_duration;
907 int64_t end_time;
932 };
1020 #define AVFMT_FLAG_GENPTS 0x0001 ///< Generate missing pts even if it requires parsing future frames.
1024 #define AVFMT_FLAG_NOFILLIN 0x0010 ///< Do not infer any values from other values, just return what is stored in the container
1025 #define AVFMT_FLAG_NOPARSE 0x0020 ///< Do not use AVParsers, you also must set AVFMT_FLAG_NOFILLIN as the fillin code works on frames and no parsing -> no frames. Also seeking to frames can not work if parsing to find frame boundaries has been disabled
1027 #define AVFMT_FLAG_CUSTOM_IO 0x0080 ///< The caller has supplied a custom AVIOContext, don't avio_close() it.
1030 #define AVFMT_FLAG_SORT_DTS 0x10000 ///< try to interleave outputted packets by dts (using this flag can slow demuxing down)
1031 #define AVFMT_FLAG_PRIV_OPT 0x20000 ///< Enable use of private options by delaying codec open (this could be made default once all code is converted)
1139 * Note, not all formats support this and unpredictable things may happen if it is used when not supported.
1147 * Note, not all formats support this and unpredictable things may happen if it is used when not supported.
1155 * Note, not all formats support this and unpredictable things may happen if it is used when not supported.
1275 enum AVDurationEstimationMethod av_fmt_ctx_get_duration_estimation_method(const AVFormatContext* ctx);
1507 int avformat_open_input(AVFormatContext **ps, const char *filename, AVInputFormat *fmt, AVDictionary **options);
1682 int avformat_seek_file(AVFormatContext *s, int stream_index, int64_t min_ts, int64_t ts, int64_t max_ts, int flags);
2116 AVRational av_guess_sample_aspect_ratio(AVFormatContext *format, AVStream *stream, AVFrame *frame);
struct AVOutputFormat AVOutputFormat
void av_url_split(char *proto, int proto_size, char *authorization, int authorization_size, char *hostname, int hostname_size, int *port_ptr, char *path, int path_size, const char *url)
Split a URL string into components.
Definition: libavformat/utils.c:3830
full parsing and interpolation of timestamps for frames not starting on a packet boundary ...
Definition: avformat.h:584
struct AVInputFormat AVInputFormat
Buffered I/O operations.
attribute_deprecated int av_demuxer_open(AVFormatContext *ic)
Definition: libavformat/utils.c:451
int av_interleaved_write_frame(AVFormatContext *s, AVPacket *pkt)
Write a packet to an output media file ensuring correct interleaving.
Definition: mux.c:726
int av_add_index_entry(AVStream *st, int64_t pos, int64_t timestamp, int size, int distance, int flags)
Add an index entry into a sorted list.
Definition: libavformat/utils.c:1768
int avformat_write_header(AVFormatContext *s, AVDictionary **options)
Allocate the stream private data and write the stream header to an output media file.
Definition: mux.c:383
int av_write_frame(AVFormatContext *s, AVPacket *pkt)
Write a packet to an output media file.
Definition: mux.c:504
int avformat_open_input(AVFormatContext **ps, const char *filename, AVInputFormat *fmt, AVDictionary **options)
Open an input stream and read the header.
Definition: libavformat/utils.c:614
int avformat_query_codec(AVOutputFormat *ofmt, enum AVCodecID codec_id, int std_compliance)
Test if the given container can store a codec.
Definition: libavformat/utils.c:4182
static int write_packet(AVFormatContext *s, AVPacket *pkt)
Definition: libavformat/assenc.c:63
static int read_seek(AVFormatContext *ctx, int stream_index, int64_t timestamp, int flags)
Definition: libcdio.c:153
AVRational av_guess_sample_aspect_ratio(AVFormatContext *format, AVStream *stream, AVFrame *frame)
Guess the sample aspect ratio of a frame, based on both the stream and the frame aspect ratio...
Definition: libavformat/utils.c:4268
void av_stream_set_r_frame_rate(AVStream *s, AVRational r)
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
void av_pkt_dump2(FILE *f, AVPacket *pkt, int dump_payload, AVStream *st)
Send a nice dump of a packet to the specified file stream.
Definition: libavformat/utils.c:3811
int av_get_output_timestamp(struct AVFormatContext *s, int stream, int64_t *dts, int64_t *wall)
Get timing information for the data currently output.
Definition: mux.c:811
AVInputFormat * av_probe_input_format3(AVProbeData *pd, int is_opened, int *score_ret)
Guess the file format.
Definition: libavformat/utils.c:352
Definition: avformat.h:456
int priv_data_size
Size of private data so that it can be allocated in the wrapper.
Definition: avformat.h:505
enum AVDurationEstimationMethod av_fmt_ctx_get_duration_estimation_method(const AVFormatContext *ctx)
Returns the method used to set ctx->duration.
Definition: libavformat/options.c:115
AVRational av_guess_frame_rate(AVFormatContext *ctx, AVStream *stream, AVFrame *frame)
Guess the frame rate, based on both the container and codec information.
Definition: libavformat/utils.c:4291
Public dictionary API.
const struct AVCodecTag * avformat_get_riff_video_tags(void)
Definition: libavformat/utils.c:4259
enum AVCodecID av_codec_get_id(const struct AVCodecTag *const *tags, unsigned int tag)
Get the AVCodecID for the given codec tag tag.
unsigned avformat_version(void)
Return the LIBAVFORMAT_VERSION_INT constant.
Definition: libavformat/utils.c:58
const char * avformat_license(void)
Return the libavformat license.
Definition: libavformat/utils.c:69
int priv_data_size
size of private data so that it can be allocated in the wrapper
Definition: avformat.h:419
int avformat_match_stream_specifier(AVFormatContext *s, AVStream *st, const char *spec)
Check if the stream st contained in s is matched by the stream specifier spec.
Definition: libavformat/utils.c:4307
AVDurationEstimationMethod
The duration of a video can be estimated through various ways, and this enum can be used to know how ...
Definition: avformat.h:930
AVStream * avformat_new_stream(AVFormatContext *s, const AVCodec *c)
Add a new stream to a media file.
Definition: libavformat/utils.c:3362
int av_codec_get_tag2(const struct AVCodecTag *const *tags, enum AVCodecID id, unsigned int *tag)
Get the codec tag for the given codec id.
Definition: libavformat/internal.h:35
AVFormatContext * avformat_alloc_context(void)
Allocate an AVFormatContext.
Definition: libavformat/options.c:106
const char * avformat_configuration(void)
Return the libavformat build-time configuration.
Definition: libavformat/utils.c:64
Definition: avformat.h:1279
AVProgram * av_new_program(AVFormatContext *s, int id)
Definition: libavformat/utils.c:3421
int avformat_network_init(void)
Do global initialization of network components.
Definition: libavformat/utils.c:4196
const struct AVCodecTag * avformat_get_riff_audio_tags(void)
Definition: libavformat/utils.c:4263
int av_sdp_create(AVFormatContext *ac[], int n_files, char *buf, int size)
Generate an SDP for an RTP session.
Definition: sdp.c:703
int av_get_packet(AVIOContext *s, AVPacket *pkt, int size)
Allocate and read the payload of a packet and initialize its fields with default values.
Definition: libavformat/utils.c:328
int av_match_ext(const char *filename, const char *extensions)
Return a positive value if the given filename has one of the given extensions, 0 otherwise.
Definition: libavformat/utils.c:143
const AVClass * avformat_get_class(void)
Get the AVClass for AVFormatContext.
Definition: libavformat/options.c:120
static int interleave_packet(AVFormatContext *s, AVPacket *out, AVPacket *in, int flush)
Interleave an AVPacket correctly so it can be muxed.
Definition: mux.c:715
full parsing and repack with timestamp and position generation by parser for raw this assumes that ea...
Definition: avformat.h:586
Duration estimated from bitrate (less accurate)
Definition: avformat.h:933
int av_append_packet(AVIOContext *s, AVPacket *pkt, int size)
Read data and append it to the current content of the AVPacket.
Definition: libavformat/utils.c:338
int avformat_alloc_output_context2(AVFormatContext **ctx, AVOutputFormat *oformat, const char *format_name, const char *filename)
Allocate an AVFormatContext for an output format.
Definition: mux.c:125
Definition: avformat.h:581
void av_dump_format(AVFormatContext *ic, int index, const char *url, int is_output)
Definition: libavformat/utils.c:3599
int av_find_best_stream(AVFormatContext *ic, enum AVMediaType type, int wanted_stream_nb, int related_stream, AVCodec **decoder_ret, int flags)
Find the "best" stream in the file.
Definition: libavformat/utils.c:3189
AVProgram * av_find_program_from_stream(AVFormatContext *ic, AVProgram *last, int s)
Find the programs which belong to a given stream.
Definition: libavformat/utils.c:3172
Definition: dict.c:28
int av_index_search_timestamp(AVStream *st, int64_t timestamp, int flags)
Get the index for a specific timestamp.
Definition: libavformat/utils.c:1811
void av_hex_dump(FILE *f, const uint8_t *buf, int size)
Send a nice hexadecimal dump of a buffer to the specified file stream.
Definition: libavformat/utils.c:3768
int av_read_play(AVFormatContext *s)
Start playing a network-based stream (e.g.
Definition: libavformat/utils.c:3251
external API header
AVInputFormat * av_probe_input_format2(AVProbeData *pd, int is_opened, int *score_max)
Guess the file format.
Definition: libavformat/utils.c:398
The exact value of the fractional number is: 'val + num / den'.
Definition: avformat.h:322
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
struct AVChapter AVChapter
int av_read_pause(AVFormatContext *s)
Pause a network-based stream (e.g.
Definition: libavformat/utils.c:3260
AVInputFormat * av_find_input_format(const char *short_name)
Find AVInputFormat based on the short name of the input format.
Definition: libavformat/utils.c:248
enum AVCodecID av_guess_codec(AVOutputFormat *fmt, const char *short_name, const char *filename, const char *mime_type, enum AVMediaType type)
Guess the codec ID based upon muxer and filename.
Definition: libavformat/utils.c:223
int av_find_default_stream_index(AVFormatContext *s)
Definition: libavformat/utils.c:1640
int av_probe_input_buffer(AVIOContext *pb, AVInputFormat **fmt, const char *filename, void *logctx, unsigned int offset, unsigned int max_probe_size)
Probe a bytestream to determine the input format.
Definition: libavformat/utils.c:471
Definition: avformat.h:591
Duration estimated from a stream with a known duration.
Definition: avformat.h:932
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
AVOutputFormat * av_guess_format(const char *short_name, const char *filename, const char *mime_type)
Return the output format in the list of registered output formats which best matches the provided par...
Definition: libavformat/utils.c:188
void av_register_input_format(AVInputFormat *format)
Definition: libavformat/utils.c:125
Libavformat version macros.
Definition: libavcodec/avcodec.h:3747
int av_get_frame_filename(char *buf, int buf_size, const char *path, int number)
Return in 'buf' the path with 'd' replaced by a number.
Definition: libavformat/utils.c:3684
AVOutputFormat * av_oformat_next(AVOutputFormat *f)
If f is NULL, returns the first registered output format, if f is non-NULL, returns the next register...
Definition: libavformat/utils.c:119
int avformat_queue_attached_pictures(AVFormatContext *s)
Definition: libavformat/utils.c:598
void av_register_output_format(AVOutputFormat *format)
Definition: libavformat/utils.c:134
int avformat_network_deinit(void)
Undo the initialization done by avformat_network_init.
Definition: libavformat/utils.c:4208
int av_filename_number_test(const char *filename)
Check whether filename actually is a numbered sequence generator.
Definition: libavformat/utils.c:346
struct AVPacketList AVPacketList
AVRational av_stream_get_r_frame_rate(const AVStream *s)
void avformat_free_context(AVFormatContext *s)
Free an AVFormatContext and all its streams.
Definition: libavformat/utils.c:3291
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
#define type
int av_read_frame(AVFormatContext *s, AVPacket *pkt)
Return the next frame of a stream.
Definition: libavformat/utils.c:1530
int avformat_seek_file(AVFormatContext *s, int stream_index, int64_t min_ts, int64_t ts, int64_t max_ts, int flags)
Seek to timestamp ts.
Definition: libavformat/utils.c:2146
full parsing and repack of the first frame only, only implemented for H.264 currently ...
Definition: avformat.h:585
int av_seek_frame(AVFormatContext *s, int stream_index, int64_t timestamp, int flags)
Seek to the keyframe at timestamp.
Definition: libavformat/utils.c:2136
Definition: avformat.h:918
int avformat_find_stream_info(AVFormatContext *ic, AVDictionary **options)
Read packets of a media file to get stream information.
Definition: libavformat/utils.c:2748
struct AVProbeData AVProbeData
This structure contains the data a format has to probe a file.
Definition: avformat.h:377
void avformat_close_input(AVFormatContext **s)
Close an opened input AVFormatContext.
Definition: libavformat/utils.c:3329
AVInputFormat * av_iformat_next(AVInputFormat *f)
If f is NULL, returns the first registered input format, if f is non-NULL, returns the next registere...
Definition: libavformat/utils.c:113
int av_write_trailer(AVFormatContext *s)
Write the stream trailer to an output media file and free the file private data.
Definition: mux.c:769
struct AVIndexEntry AVIndexEntry
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
void av_pkt_dump_log2(void *avcl, int level, AVPacket *pkt, int dump_payload, AVStream *st)
Send a nice dump of a packet to the log.
Definition: libavformat/utils.c:3824
void av_hex_dump_log(void *avcl, int level, const uint8_t *buf, int size)
Send a nice hexadecimal dump of a buffer to the log.
Definition: libavformat/utils.c:3773
unsigned int av_codec_get_tag(const struct AVCodecTag *const *tags, enum AVCodecID id)
Get the codec tag for the given codec id id.
AVInputFormat * av_probe_input_format(AVProbeData *pd, int is_opened)
Guess the file format.
Definition: libavformat/utils.c:409
void av_register_all(void)
Initialize libavformat and register all the muxers, demuxers and protocols.
Definition: allformats.c:52
#define MAX_STD_TIMEBASES
Stream information used internally by av_find_stream_info()
Definition: avformat.h:751
Generated on Tue Jul 1 2025 06:52:21 for FFmpeg by
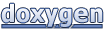