FFmpeg
|
libavcodec/aacdec.c
Go to the documentation of this file.
158 if (*channels >= MAX_CHANNELS - (type == TYPE_CPE || (type == TYPE_SCE && ac->oc[1].m4ac.ps == 1))) {
564 if (ac->tags_mapped == tags_per_config[ac->oc[1].m4ac.chan_config] - 1 && (type == TYPE_LFE || type == TYPE_SCE)) {
597 * Decode an array of 4 bit element IDs, optionally interleaved with a stereo/mono switching bit.
647 av_log(avctx, AV_LOG_WARNING, "Sample rate index in program config element does not match the sample rate index configured by the container.\n");
664 if (get_bits_left(gb) < 4 * (num_front + num_side + num_back + num_lfe + num_assoc_data + num_cc)) {
877 ff_aac_spectral_bits[num], sizeof( ff_aac_spectral_bits[num][0]), sizeof( ff_aac_spectral_bits[num][0]), \
878 ff_aac_spectral_codes[num], sizeof(ff_aac_spectral_codes[num][0]), sizeof(ff_aac_spectral_codes[num][0]), \
956 ff_aac_scalefactor_bits, sizeof(ff_aac_scalefactor_bits[0]), sizeof(ff_aac_scalefactor_bits[0]),
957 ff_aac_scalefactor_code, sizeof(ff_aac_scalefactor_code[0]), sizeof(ff_aac_scalefactor_code[0]),
1005 for (sfb = 0; sfb < FFMIN(ics->max_sfb, ff_aac_pred_sfb_max[ac->oc[1].m4ac.sampling_index]); sfb++) {
1289 memset(cpe->ms_mask, 1, sizeof(cpe->ms_mask[0]) * cpe->ch[0].ics.num_window_groups * cpe->ch[0].ics.max_sfb);
1385 memset(coef + g * 128 + offsets[ics->max_sfb], 0, sizeof(float) * (c - offsets[ics->max_sfb]));
1670 * @param common_window Channels have independent [0], or shared [1], Individual Channel Stream information.
1671 * @param scale_flag scalable [1] or non-scalable [0] AAC (Unused until scalable AAC is implemented.)
1698 if (decode_scalefactors(ac, sce->sf, gb, global_gain, ics, sce->band_type, sce->band_type_run_end) < 0)
1721 if (decode_spectrum_and_dequant(ac, out, gb, sce->sf, pulse_present, &pulse, ics, sce->band_type) < 0)
1922 * Parse whether channels are to be excluded from Dynamic Range Compression; reference: table 4.53.
2038 av_log(ac->avctx, AV_LOG_ERROR, "SBR signaled to be not-present but was found in the bitstream.\n");
2042 av_log(ac->avctx, AV_LOG_ERROR, "Implicit SBR was found with a first occurrence after the first frame.\n");
2045 } else if (ac->oc[1].m4ac.ps == -1 && ac->oc[1].status < OC_LOCKED && ac->avctx->channels == 1) {
2071 * Decode Temporal Noise Shaping filter coefficients and apply all-pole filters; reference: 4.6.9.3.
2251 if ((ics->window_sequence[1] == ONLY_LONG_SEQUENCE || ics->window_sequence[1] == LONG_STOP_SEQUENCE) &&
2252 (ics->window_sequence[0] == ONLY_LONG_SEQUENCE || ics->window_sequence[0] == LONG_START_SEQUENCE)) {
2348 void (*apply_coupling_method)(AACContext *ac, SingleChannelElement *target, ChannelElement *cce, int index))
2498 av_log(ac->avctx, AV_LOG_ERROR, "invalid sampling rate index %d\n", ac->oc[1].m4ac.sampling_index);
2604 multiplier = (ac->oc[1].m4ac.sbr == 1) ? ac->oc[1].m4ac.ext_sample_rate > ac->oc[1].m4ac.sample_rate : 0;
3007 {"auto", "autoselection", 0, AV_OPT_TYPE_CONST, {.i64=-1}, INT_MIN, INT_MAX, AACDEC_FLAGS, "dual_mono_mode"},
3008 {"main", "Select Main/Left channel", 0, AV_OPT_TYPE_CONST, {.i64= 1}, INT_MIN, INT_MAX, AACDEC_FLAGS, "dual_mono_mode"},
3009 {"sub" , "Select Sub/Right channel", 0, AV_OPT_TYPE_CONST, {.i64= 2}, INT_MIN, INT_MAX, AACDEC_FLAGS, "dual_mono_mode"},
3010 {"both", "Select both channels", 0, AV_OPT_TYPE_CONST, {.i64= 0}, INT_MIN, INT_MAX, AACDEC_FLAGS, "dual_mono_mode"},
static int decode_fill(AACContext *ac, GetBitContext *gb, int len)
Definition: libavcodec/aacdec.c:1992
static int output_configure(AACContext *ac, uint8_t layout_map[MAX_ELEM_ID *4][3], int tags, enum OCStatus oc_type, int get_new_frame)
Configure output channel order based on the current program configuration element.
Definition: libavcodec/aacdec.c:423
static float * VMUL4S(float *dst, const float *v, unsigned idx, unsigned sign, const float *scale)
Definition: libavcodec/aacdec.c:1335
float(* scalarproduct_float)(const float *v1, const float *v2, int len)
Calculate the scalar product of two vectors of floats.
Definition: float_dsp.h:161
AAC decoder data.
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
static void imdct_and_windowing(AACContext *ac, SingleChannelElement *sce)
Conduct IMDCT and windowing.
Definition: libavcodec/aacdec.c:2225
uint8_t use_kb_window[2]
If set, use Kaiser-Bessel window, otherwise use a sinus window.
Definition: aac.h:160
static int parse_adts_frame_header(AACContext *ac, GetBitContext *gb)
Definition: libavcodec/aacdec.c:2421
static int decode_audio_specific_config(AACContext *ac, AVCodecContext *avctx, MPEG4AudioConfig *m4ac, const uint8_t *data, int bit_size, int sync_extension)
Decode audio specific configuration; reference: table 1.13.
Definition: libavcodec/aacdec.c:775
Definition: aac.h:72
static void push_output_configuration(AACContext *ac)
Save current output configuration if and only if it has been locked.
Definition: libavcodec/aacdec.c:397
static void apply_intensity_stereo(AACContext *ac, ChannelElement *cpe, int ms_present)
intensity stereo decoding; reference: 4.6.8.2.3
Definition: libavcodec/aacdec.c:1763
av_cold void ff_kbd_window_init(float *window, float alpha, int n)
Generate a Kaiser-Bessel Derived Window.
Definition: kbdwin.c:26
Definition: opt.h:222
static float * VMUL2S(float *dst, const float *v, unsigned idx, unsigned sign, const float *scale)
Definition: libavcodec/aacdec.c:1318
Definition: libavcodec/aacdec.c:207
static int decode_ics_info(AACContext *ac, IndividualChannelStream *ics, GetBitContext *gb)
Decode Individual Channel Stream info; reference: table 4.6.
Definition: libavcodec/aacdec.c:1028
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
#define AACDEC_FLAGS
AVOptions for Japanese DTV specific extensions (ADTS only)
Definition: libavcodec/aacdec.c:3001
static int assign_pair(struct elem_to_channel e2c_vec[MAX_ELEM_ID], uint8_t(*layout_map)[3], int offset, uint64_t left, uint64_t right, int pos)
Definition: libavcodec/aacdec.c:214
uint8_t ms_mask[128]
Set if mid/side stereo is used for each scalefactor window band.
Definition: aac.h:251
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
static void apply_independent_coupling(AACContext *ac, SingleChannelElement *target, ChannelElement *cce, int index)
Apply independent channel coupling (applied after IMDCT).
Definition: libavcodec/aacdec.c:2326
static int frame_configure_elements(AVCodecContext *avctx)
Definition: libavcodec/aacdec.c:176
static int decode_pce(AVCodecContext *avctx, MPEG4AudioConfig *m4ac, uint8_t(*layout_map)[3], GetBitContext *gb)
Decode program configuration element; reference: table 4.2.
Definition: libavcodec/aacdec.c:635
Dynamic Range Control - decoded from the bitstream but not processed further.
Definition: aac.h:190
Reference: libavcodec/aacdec.c.
static av_always_inline void predict(PredictorState *ps, float *coef, int output_enable)
Definition: libavcodec/aacdec.c:1611
enum RawDataBlockType type[8]
Type of channel element to be coupled - SCE or CPE.
Definition: aac.h:216
struct AACContext AACContext
Definition: aac.h:87
static int decode_spectrum_and_dequant(AACContext *ac, float coef[1024], GetBitContext *gb, const float sf[120], int pulse_present, const Pulse *pulse, const IndividualChannelStream *ics, enum BandType band_type[120])
Decode spectral data; reference: table 4.50.
Definition: libavcodec/aacdec.c:1373
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
av_cold void ff_aac_sbr_ctx_close(SpectralBandReplication *sbr)
Close one SBR context.
Definition: aacsbr.c:167
void(* apply_tns)(float coef[1024], TemporalNoiseShaping *tns, IndividualChannelStream *ics, int decode)
Definition: aac.h:323
const uint16_t * swb_offset
table of offsets to the lowest spectral coefficient of a scalefactor band, sfb, for a particular wind...
Definition: aac.h:164
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
Definition: opt.h:229
void void avpriv_request_sample(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
static void apply_prediction(AACContext *ac, SingleChannelElement *sce)
Apply AAC-Main style frequency domain prediction.
Definition: libavcodec/aacdec.c:1645
static const uint8_t aac_channel_layout_map[7][5][3]
Definition: aacdectab.h:83
Definition: aac.h:71
Definition: samplefmt.h:50
AVOptions.
#define INIT_VLC_STATIC(vlc, bits, a, b, c, d, e, f, g, static_size)
Definition: get_bits.h:445
void(* vector_fmul)(float *dst, const float *src0, const float *src1, int len)
Calculate the product of two vectors of floats and store the result in a vector of floats...
Definition: float_dsp.h:38
Definition: libavcodec/avcodec.h:383
static int aac_decode_frame_int(AVCodecContext *avctx, void *data, int *got_frame_ptr, GetBitContext *gb, AVPacket *avpkt)
Definition: libavcodec/aacdec.c:2479
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
Definition: aac.h:61
int ff_decode_sbr_extension(AACContext *ac, SpectralBandReplication *sbr, GetBitContext *gb_host, int crc, int cnt, int id_aac)
Decode Spectral Band Replication extension data; reference: table 4.55.
Definition: aacsbr.c:1076
static int decode_band_types(AACContext *ac, enum BandType band_type[120], int band_type_run_end[120], GetBitContext *gb, IndividualChannelStream *ics)
Decode band types (section_data payload); reference: table 4.46.
Definition: libavcodec/aacdec.c:1101
SingleChannelElement * output_element[MAX_CHANNELS]
Points to each SingleChannelElement.
Definition: aac.h:303
static int decode_pulses(Pulse *pulse, GetBitContext *gb, const uint16_t *swb_offset, int num_swb)
Decode pulse data; reference: table 4.7.
Definition: libavcodec/aacdec.c:1212
static int count_paired_channels(uint8_t(*layout_map)[3], int tags, int pos, int *current)
Definition: libavcodec/aacdec.c:234
bitstream reader API header.
static int read_stream_mux_config(struct LATMContext *latmctx, GetBitContext *gb)
Definition: libavcodec/aacdec.c:2795
static av_cold int che_configure(AACContext *ac, enum ChannelPosition che_pos, int type, int id, int *channels)
Check for the channel element in the current channel position configuration.
Definition: libavcodec/aacdec.c:147
#define CODEC_FLAG_BITEXACT
Use only bitexact stuff (except (I)DCT).
Definition: libavcodec/avcodec.h:715
const float *const ff_aac_codebook_vector_vals[]
Definition: aactab.c:1052
static int decode_dynamic_range(DynamicRangeControl *che_drc, GetBitContext *gb)
Decode dynamic range information; reference: table 4.52.
Definition: libavcodec/aacdec.c:1945
static void apply_channel_coupling(AACContext *ac, ChannelElement *cc, enum RawDataBlockType type, int elem_id, enum CouplingPoint coupling_point, void(*apply_coupling_method)(AACContext *ac, SingleChannelElement *target, ChannelElement *cce, int index))
channel coupling transformation interface
Definition: libavcodec/aacdec.c:2345
Definition: aac.h:91
static av_cold int aac_decode_close(AVCodecContext *avctx)
Definition: libavcodec/aacdec.c:2697
void(* vector_fmul_window)(float *dst, const float *src0, const float *src1, const float *win, int len)
Overlap/add with window function.
Definition: float_dsp.h:103
PredictorState predictor_state[MAX_PREDICTORS]
Definition: aac.h:240
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
static int decode_extension_payload(AACContext *ac, GetBitContext *gb, int cnt, ChannelElement *che, enum RawDataBlockType elem_type)
Decode extension data (incomplete); reference: table 4.51.
Definition: libavcodec/aacdec.c:2025
Definition: libavcodec/aacdec.c:2719
enum CouplingPoint coupling_point
The point during decoding at which coupling is applied.
Definition: aac.h:214
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
Definition: aac.h:62
Spectral Band Replication definitions and structures.
void(* mdct_calc)(struct FFTContext *s, FFTSample *output, const FFTSample *input)
Definition: fft.h:83
Definition: avutil.h:144
void(* apply_ltp)(AACContext *ac, SingleChannelElement *sce)
Definition: aac.h:322
static int latm_decode_frame(AVCodecContext *avctx, void *out, int *got_frame_ptr, AVPacket *avpkt)
Definition: libavcodec/aacdec.c:2925
void ff_sbr_apply(AACContext *ac, SpectralBandReplication *sbr, int id_aac, float *L, float *R)
Apply one SBR element to one AAC element.
Definition: aacsbr.c:1681
external API header
Definition: mpeg4audio.h:29
int prog_ref_level
A reference level for the long-term program audio level for all channels combined.
Definition: aac.h:198
Definition: get_bits.h:63
#define POW_SF2_ZERO
ff_aac_pow2sf_tab index corresponding to pow(2, 0);
Definition: aac_tablegen_decl.h:26
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
static int read_payload_length_info(struct LATMContext *ctx, GetBitContext *gb)
Definition: libavcodec/aacdec.c:2875
AAC Spectral Band Replication function declarations.
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
static void pop_output_configuration(AACContext *ac)
Restore the previous output configuration if and only if the current configuration is unlocked...
Definition: libavcodec/aacdec.c:408
static void reset_predictor_group(PredictorState *ps, int group_num)
Definition: libavcodec/aacdec.c:868
Definition: aac.h:100
Definition: aac.h:101
static void decode_mid_side_stereo(ChannelElement *cpe, GetBitContext *gb, int ms_present)
Decode Mid/Side data; reference: table 4.54.
Definition: libavcodec/aacdec.c:1281
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
AAC definitions and structures.
#define GET_VLC(code, name, gb, table, bits, max_depth)
If the vlc code is invalid and max_depth=1, then no bits will be removed.
Definition: get_bits.h:458
static void apply_dependent_coupling(AACContext *ac, SingleChannelElement *target, ChannelElement *cce, int index)
Apply dependent channel coupling (applied before IMDCT).
Definition: libavcodec/aacdec.c:2290
static int decode_drc_channel_exclusions(DynamicRangeControl *che_drc, GetBitContext *gb)
Parse whether channels are to be excluded from Dynamic Range Compression; reference: table 4...
Definition: libavcodec/aacdec.c:1926
Definition: aac.h:89
int interpolation_scheme
Indicates the interpolation scheme used in the SBR QMF domain.
Definition: aac.h:196
Definition: aac.h:64
static int decode_ga_specific_config(AACContext *ac, AVCodecContext *avctx, GetBitContext *gb, MPEG4AudioConfig *m4ac, int channel_config)
Decode GA "General Audio" specific configuration; reference: table 4.1.
Definition: libavcodec/aacdec.c:702
int ch_select[8]
[0] shared list of gains; [1] list of gains for right channel; [2] list of gains for left channel; [3...
Definition: aac.h:218
static void apply_mid_side_stereo(AACContext *ac, ChannelElement *cpe)
Mid/Side stereo decoding; reference: 4.6.8.1.3.
Definition: libavcodec/aacdec.c:1733
int frame_size
Number of samples per channel in an audio frame.
Definition: libavcodec/avcodec.h:1881
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
Definition: aac.h:63
static int compute_lpc_coefs(const LPC_TYPE *autoc, int max_order, LPC_TYPE *lpc, int lpc_stride, int fail, int normalize)
Levinson-Durbin recursion.
Definition: lpc.h:151
static int init_get_bits8(GetBitContext *s, const uint8_t *buffer, int byte_size)
Initialize GetBitContext.
Definition: get_bits.h:410
#define AV_CH_LAYOUT_NATIVE
Channel mask value used for AVCodecContext.request_channel_layout to indicate that the user requests ...
Definition: channel_layout.h:78
Definition: aac.h:70
static void update_ltp(AACContext *ac, SingleChannelElement *sce)
Update the LTP buffer for next frame.
Definition: libavcodec/aacdec.c:2190
static int decode_ics(AACContext *ac, SingleChannelElement *sce, GetBitContext *gb, int common_window, int scale_flag)
Decode an individual_channel_stream payload; reference: table 4.44.
Definition: libavcodec/aacdec.c:1675
int ff_get_buffer(AVCodecContext *avctx, AVFrame *frame, int flags)
Get a buffer for a frame.
Definition: libavcodec/utils.c:823
void(* butterflies_float)(float *av_restrict v1, float *av_restrict v2, int len)
Calculate the sum and difference of two vectors of floats.
Definition: float_dsp.h:150
int avpriv_aac_parse_header(GetBitContext *gbc, AACADTSHeaderInfo *hdr)
Parse AAC frame header.
Definition: aacadtsdec.c:29
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static av_cold int latm_decode_init(AVCodecContext *avctx)
Definition: libavcodec/aacdec.c:2976
static void spectral_to_sample(AACContext *ac)
Convert spectral data to float samples, applying all supported tools as appropriate.
Definition: libavcodec/aacdec.c:2378
static av_always_inline int lcg_random(unsigned previous_val)
linear congruential pseudorandom number generator
Definition: libavcodec/aacdec.c:829
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
Recommmends skipping the specified number of samples.
Definition: libavcodec/avcodec.h:968
Definition: aac.h:90
static int read_audio_mux_element(struct LATMContext *latmctx, GetBitContext *gb)
Definition: libavcodec/aacdec.c:2896
static int latm_decode_audio_specific_config(struct LATMContext *latmctx, GetBitContext *gb, int asclen)
Definition: libavcodec/aacdec.c:2736
void(* vector_fmul_scalar)(float *dst, const float *src, float mul, int len)
Multiply a vector of floats by a scalar float.
Definition: float_dsp.h:69
static void apply_ltp(AACContext *ac, SingleChannelElement *sce)
Apply the long term prediction.
Definition: libavcodec/aacdec.c:2158
static float * VMUL2(float *dst, const float *v, unsigned idx, const float *scale)
Definition: libavcodec/aacdec.c:1294
int skip_samples
Number of audio samples to skip at the start of the next decoded frame.
Definition: libavcodec/internal.h:109
Definition: aac.h:92
av_cold void ff_aac_sbr_ctx_init(AACContext *ac, SpectralBandReplication *sbr)
Initialize one SBR context.
Definition: aacsbr.c:149
void av_frame_unref(AVFrame *frame)
Unreference all the buffers referenced by frame and reset the frame fields.
Definition: frame.c:330
static void reset_all_predictors(PredictorState *ps)
Definition: libavcodec/aacdec.c:845
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
void(* imdct_half)(struct FFTContext *s, FFTSample *output, const FFTSample *input)
Definition: fft.h:82
Definition: aac.h:88
void avpriv_report_missing_feature(void *avc, const char *msg,...) av_printf_format(2
Log a generic warning message about a missing feature.
Definition: libavcodec/avcodec.h:431
int band_top[17]
Indicates the top of the i-th DRC band in units of 4 spectral lines.
Definition: aac.h:197
static int decode_tns(AACContext *ac, TemporalNoiseShaping *tns, GetBitContext *gb, const IndividualChannelStream *ics)
Decode Temporal Noise Shaping data; reference: table 4.48.
Definition: libavcodec/aacdec.c:1239
static int decode_cpe(AACContext *ac, GetBitContext *gb, ChannelElement *cpe)
Decode a channel_pair_element; reference: table 4.4.
Definition: libavcodec/aacdec.c:1803
Definition: aacadtsdec.h:31
static av_always_inline void reset_predict_state(PredictorState *ps)
Definition: libavcodec/aacdec.c:835
An AV_PKT_DATA_JP_DUALMONO side data packet indicates that the packet may contain "dual mono" audio s...
Definition: libavcodec/avcodec.h:978
Definition: get_bits.h:54
common internal api header.
Single Channel Element - used for both SCE and LFE elements.
Definition: aac.h:227
#define CODEC_CAP_CHANNEL_CONF
Codec should fill in channel configuration and samplerate instead of container.
Definition: libavcodec/avcodec.h:800
static av_cold int aac_decode_init(AVCodecContext *avctx)
Definition: libavcodec/aacdec.c:883
static ChannelElement * get_che(AACContext *ac, int type, int elem_id)
Definition: libavcodec/aacdec.c:510
int avpriv_mpeg4audio_get_config(MPEG4AudioConfig *c, const uint8_t *buf, int bit_size, int sync_extension)
Parse MPEG-4 systems extradata to retrieve audio configuration.
Definition: mpeg4audio.c:81
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel layout
Definition: filter_design.txt:12
const uint16_t *const ff_aac_codebook_vector_idx[]
Definition: aactab.c:1061
static void windowing_and_mdct_ltp(AACContext *ac, float *out, float *in, IndividualChannelStream *ics)
Apply windowing and MDCT to obtain the spectral coefficient from the predicted sample by LTP...
Definition: libavcodec/aacdec.c:2132
void(* update_ltp)(AACContext *ac, SingleChannelElement *sce)
Definition: aac.h:327
static uint64_t sniff_channel_order(uint8_t(*layout_map)[3], int tags)
Definition: libavcodec/aacdec.c:264
av_cold void ff_fmt_convert_init(FmtConvertContext *c, AVCodecContext *avctx)
Definition: fmtconvert.c:79
struct AVCodecInternal * internal
Private context used for internal data.
Definition: libavcodec/avcodec.h:1178
static int decode_scalefactors(AACContext *ac, float sf[120], GetBitContext *gb, unsigned int global_gain, IndividualChannelStream *ics, enum BandType band_type[120], int band_type_run_end[120])
Decode scalefactors; reference: table 4.47.
Definition: libavcodec/aacdec.c:1150
void(* windowing_and_mdct_ltp)(AACContext *ac, float *out, float *in, IndividualChannelStream *ics)
Definition: aac.h:325
static int set_default_channel_config(AVCodecContext *avctx, uint8_t(*layout_map)[3], int *tags, int channel_config)
Set up channel positions based on a default channel configuration as specified in table 1...
Definition: libavcodec/aacdec.c:495
Definition: aac.h:69
static void decode_channel_map(uint8_t layout_map[][3], enum ChannelPosition type, GetBitContext *gb, int n)
Decode an array of 4 bit element IDs, optionally interleaved with a stereo/mono switching bit...
Definition: libavcodec/aacdec.c:601
static int skip_data_stream_element(AACContext *ac, GetBitContext *gb)
Skip data_stream_element; reference: table 4.10.
Definition: libavcodec/aacdec.c:977
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
uint8_t * av_packet_get_side_data(AVPacket *pkt, enum AVPacketSideDataType type, int *size)
Get side information from packet.
Definition: avpacket.c:289
static int decode_prediction(AACContext *ac, IndividualChannelStream *ics, GetBitContext *gb)
Definition: libavcodec/aacdec.c:994
uint8_t pi<< 24) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0f/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_U8, uint8_t,(*(const uint8_t *) pi-0x80)*(1.0/(1<< 7))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S16, int16_t,(*(const int16_t *) pi >> 8)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0f/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S16, int16_t,*(const int16_t *) pi *(1.0/(1<< 15))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_S32, int32_t,(*(const int32_t *) pi >> 24)+0x80) CONV_FUNC_GROUP(AV_SAMPLE_FMT_FLT, float, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0f/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_DBL, double, AV_SAMPLE_FMT_S32, int32_t,*(const int32_t *) pi *(1.0/(1U<< 31))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_FLT, float, av_clip_uint8(lrintf(*(const float *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_FLT, float, av_clip_int16(lrintf(*(const float *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_FLT, float, av_clipl_int32(llrintf(*(const float *) pi *(1U<< 31)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_U8, uint8_t, AV_SAMPLE_FMT_DBL, double, av_clip_uint8(lrint(*(const double *) pi *(1<< 7))+0x80)) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S16, int16_t, AV_SAMPLE_FMT_DBL, double, av_clip_int16(lrint(*(const double *) pi *(1<< 15)))) CONV_FUNC_GROUP(AV_SAMPLE_FMT_S32, int32_t, AV_SAMPLE_FMT_DBL, double, av_clipl_int32(llrint(*(const double *) pi *(1U<< 31))))#define SET_CONV_FUNC_GROUP(ofmt, ifmt) static void set_generic_function(AudioConvert *ac){}void ff_audio_convert_free(AudioConvert **ac){if(!*ac) return;ff_dither_free(&(*ac) ->dc);av_freep(ac);}AudioConvert *ff_audio_convert_alloc(AVAudioResampleContext *avr, enum AVSampleFormat out_fmt, enum AVSampleFormat in_fmt, int channels, int sample_rate, int apply_map){AudioConvert *ac;int in_planar, out_planar;ac=av_mallocz(sizeof(*ac));if(!ac) return NULL;ac->avr=avr;ac->out_fmt=out_fmt;ac->in_fmt=in_fmt;ac->channels=channels;ac->apply_map=apply_map;if(avr->dither_method!=AV_RESAMPLE_DITHER_NONE &&av_get_packed_sample_fmt(out_fmt)==AV_SAMPLE_FMT_S16 &&av_get_bytes_per_sample(in_fmt) > 2){ac->dc=ff_dither_alloc(avr, out_fmt, in_fmt, channels, sample_rate, apply_map);if(!ac->dc){av_free(ac);return NULL;}return ac;}in_planar=av_sample_fmt_is_planar(in_fmt);out_planar=av_sample_fmt_is_planar(out_fmt);if(in_planar==out_planar){ac->func_type=CONV_FUNC_TYPE_FLAT;ac->planes=in_planar?ac->channels:1;}else if(in_planar) ac->func_type=CONV_FUNC_TYPE_INTERLEAVE;else ac->func_type=CONV_FUNC_TYPE_DEINTERLEAVE;set_generic_function(ac);if(ARCH_ARM) ff_audio_convert_init_arm(ac);if(ARCH_X86) ff_audio_convert_init_x86(ac);return ac;}int ff_audio_convert(AudioConvert *ac, AudioData *out, AudioData *in){int use_generic=1;int len=in->nb_samples;int p;if(ac->dc){av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (dithered)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt));return ff_convert_dither(ac-> out
Definition: audio_convert.c:194
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
static void apply_tns(float coef[1024], TemporalNoiseShaping *tns, IndividualChannelStream *ics, int decode)
Decode Temporal Noise Shaping filter coefficients and apply all-pole filters; reference: 4...
Definition: libavcodec/aacdec.c:2076
static int decode_cce(AACContext *ac, GetBitContext *gb, ChannelElement *che)
Decode coupling_channel_element; reference: table 4.8.
Definition: libavcodec/aacdec.c:1854
void(* imdct_and_windowing)(AACContext *ac, SingleChannelElement *sce)
Definition: aac.h:321
static int aac_decode_frame(AVCodecContext *avctx, void *data, int *got_frame_ptr, AVPacket *avpkt)
Definition: libavcodec/aacdec.c:2639
void avpriv_float_dsp_init(AVFloatDSPContext *fdsp, int bit_exact)
Initialize a float DSP context.
Definition: float_dsp.c:118
Definition: aac.h:65
Definition: aac.h:99
AAC data declarations.
uint64_t request_channel_layout
Request decoder to use this channel layout if it can (0 for default)
Definition: libavcodec/avcodec.h:1929
static int count_channels(uint8_t(*layout)[3], int tags)
Definition: libavcodec/aacdec.c:123
void ff_init_ff_sine_windows(int index)
initialize the specified entry of ff_sine_windows
Definition: sinewin_tablegen.h:60
static void decode_ltp(LongTermPrediction *ltp, GetBitContext *gb, uint8_t max_sfb)
Decode Long Term Prediction data; reference: table 4.xx.
Definition: libavcodec/aacdec.c:1014
static float * VMUL4(float *dst, const float *v, unsigned idx, const float *scale)
Definition: libavcodec/aacdec.c:1305
void(* vector_fmul_reverse)(float *dst, const float *src0, const float *src1, int len)
Calculate the product of two vectors of floats, and store the result in a vector of floats...
Definition: float_dsp.h:140
Generated on Tue Jan 21 2025 06:52:17 for FFmpeg by
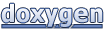