FFmpeg
|
#include <inttypes.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <libcrystalhd/bc_dts_types.h>
#include <libcrystalhd/bc_dts_defs.h>
#include <libcrystalhd/libcrystalhd_if.h>
#include "avcodec.h"
#include "h264.h"
#include "internal.h"
#include "libavutil/imgutils.h"
#include "libavutil/intreadwrite.h"
#include "libavutil/opt.h"
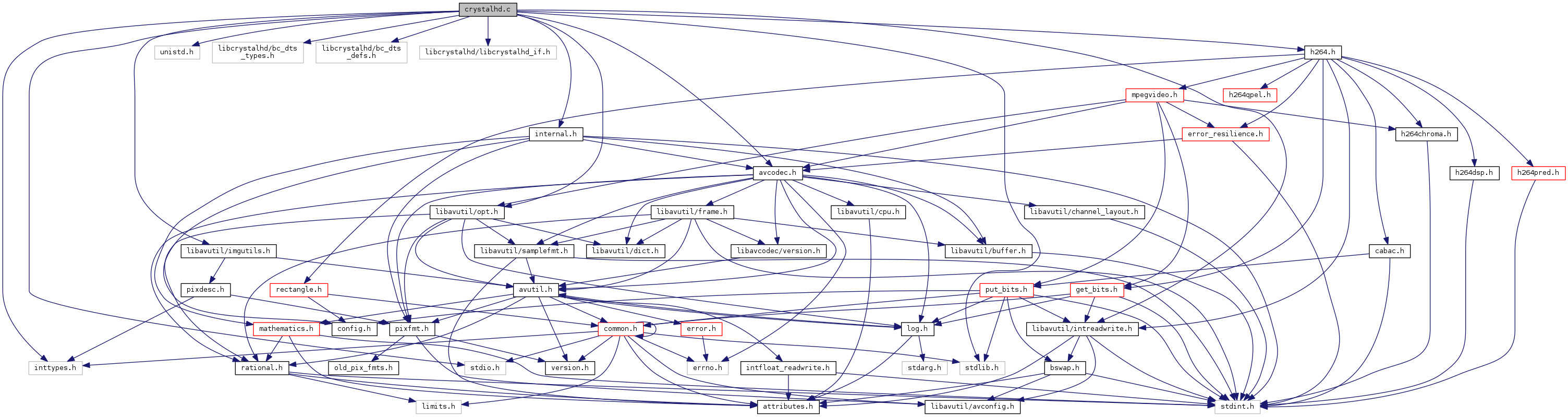
Go to the source code of this file.
Data Structures | |
struct | OpaqueList |
struct | CHDContext |
Macros | |
#define | _XOPEN_SOURCE 600 |
#define | OUTPUT_PROC_TIMEOUT 50 |
Timeout parameter passed to DtsProcOutput() in us. More... | |
#define | TIMESTAMP_UNIT 100000 |
Step between fake timestamps passed to hardware in units of 100ns. More... | |
#define | BASE_WAIT 10000 |
Initial value in us of the wait in decode() More... | |
#define | WAIT_UNIT 1000 |
Increment in us to adjust wait in decode() More... | |
Typedefs | |
typedef struct OpaqueList | OpaqueList |
Enumerations | |
enum | CopyRet { RET_ERROR = -1, RET_OK = 0, RET_COPY_AGAIN = 1, RET_SKIP_NEXT_COPY = 2, RET_COPY_NEXT_FIELD = 3 } |
Functions | |
static BC_MEDIA_SUBTYPE | id2subtype (CHDContext *priv, enum AVCodecID id) |
static void | print_frame_info (CHDContext *priv, BC_DTS_PROC_OUT *output) |
static uint64_t | opaque_list_push (CHDContext *priv, uint64_t reordered_opaque, uint8_t pic_type) |
static OpaqueList * | opaque_list_pop (CHDContext *priv, uint64_t fake_timestamp) |
static void | flush (AVCodecContext *avctx) |
static av_cold int | uninit (AVCodecContext *avctx) |
static av_cold int | init (AVCodecContext *avctx) |
static CopyRet | copy_frame (AVCodecContext *avctx, BC_DTS_PROC_OUT *output, void *data, int *got_frame) |
static CopyRet | receive_frame (AVCodecContext *avctx, void *data, int *got_frame) |
static int | decode (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt) |
Variables | |
static const AVOption | options [] |
Macro Definition Documentation
#define _XOPEN_SOURCE 600 |
Definition at line 76 of file crystalhd.c.
#define BASE_WAIT 10000 |
#define OUTPUT_PROC_TIMEOUT 50 |
Timeout parameter passed to DtsProcOutput() in us.
Definition at line 94 of file crystalhd.c.
Referenced by receive_frame().
#define TIMESTAMP_UNIT 100000 |
Step between fake timestamps passed to hardware in units of 100ns.
Definition at line 96 of file crystalhd.c.
Referenced by opaque_list_push().
#define WAIT_UNIT 1000 |
Increment in us to adjust wait in decode()
Definition at line 100 of file crystalhd.c.
Referenced by decode().
Typedef Documentation
typedef struct OpaqueList OpaqueList |
Enumeration Type Documentation
enum CopyRet |
Enumerator | |
---|---|
RET_ERROR | |
RET_OK | |
RET_COPY_AGAIN | |
RET_SKIP_NEXT_COPY | |
RET_COPY_NEXT_FIELD |
Definition at line 107 of file crystalhd.c.
Function Documentation
|
inlinestatic |
Definition at line 536 of file crystalhd.c.
Referenced by receive_frame().
|
static |
Definition at line 868 of file crystalhd.c.
Referenced by amr_decode_fix_avctx(), cyuv_decode_frame(), decode_end(), decode_flush(), eightsvx_decode_close(), flashsv_decode_end(), flush(), g726_reset(), gsm_flush(), libgsm_flush(), mvc_decode_end(), pnm_decode_frame(), predictor_calc_error(), read_access_unit(), show_formats(), sp5x_decode_frame(), vp3_init_thread_copy(), and vp8_free().
|
static |
Definition at line 316 of file crystalhd.c.
Referenced by decode().
|
inlinestatic |
Definition at line 167 of file crystalhd.c.
Referenced by init().
|
static |
Definition at line 379 of file crystalhd.c.
Referenced by decode().
|
static |
Definition at line 263 of file crystalhd.c.
Referenced by copy_frame().
|
static |
Definition at line 233 of file crystalhd.c.
Referenced by decode().
|
inlinestatic |
Definition at line 187 of file crystalhd.c.
Referenced by receive_frame().
|
inlinestatic |
Definition at line 732 of file crystalhd.c.
Referenced by decode().
|
static |
Definition at line 334 of file crystalhd.c.
Referenced by decode(), init(), and movie_request_frame().
Variable Documentation
|
static |
Definition at line 153 of file crystalhd.c.
Referenced by decode().
Generated on Wed Jun 18 2025 06:53:12 for FFmpeg by
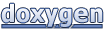