FFmpeg
|
h264.h
Go to the documentation of this file.
49 #define MAX_MBPAIR_SIZE (256*1024) // a tighter bound could be calculated if someone cares about a few bytes
142 SEI_PIC_STRUCT_TOP_BOTTOM_TOP = 5, ///< 5: top field, bottom field, top field repeated, in that order
143 SEI_PIC_STRUCT_BOTTOM_TOP_BOTTOM = 6, ///< 6: bottom field, top field, bottom field repeated, in that order
231 uint8_t chroma_qp_table[2][QP_MAX_NUM+1]; ///< pre-scaled (with chroma_qp_index_offset) version of qp_table
316 int8_t intra4x4_pred_mode_cache[5 * 8];
317 int8_t(*intra4x4_pred_mode);
369 uint32_t(*dequant4_coeff[6])[16];
370 uint32_t(*dequant8_coeff[6])[64];
414 int ref2frm[MAX_SLICES][2][64]; ///< reference to frame number lists, used in the loop filter, the first 2 are for -2,-1
423 DECLARE_ALIGNED(16, int16_t, mb)[16 * 48 * 2]; ///< as a dct coeffecient is int32_t in high depth, we need to reserve twice the space.
425 int16_t mb_padding[256 * 2]; ///< as mb is addressed by scantable[i] and scantable is uint8_t we can either check that i is not too large or ensure that there is some unused stuff after mb
433 /* 0x100 -> non null luma_dc, 0x80/0x40 -> non null chroma_dc (cb/cr), 0x?0 -> chroma_cbp(0, 1, 2), 0x0? luma_cbp */
659 extern const uint8_t ff_h264_chroma_qp[7][QP_MAX_NUM + 1]; ///< One chroma qp table for each possible bit depth (8-14).
void ff_h264_direct_dist_scale_factor(H264Context *const h)
Definition: h264_direct.c:51
Definition: videodsp.h:40
static av_always_inline int get_dct8x8_allowed(H264Context *h)
Definition: h264.h:966
int ff_h264_decode_mb_cabac(H264Context *h)
Decode a CABAC coded macroblock.
Definition: h264_cabac.c:1875
Definition: h264.h:243
Definition: h264.h:240
int single_decode_warning
1 if the single thread fallback warning has already been displayed, 0 otherwise.
Definition: h264.h:572
5: top field, bottom field, top field repeated, in that order
Definition: h264.h:142
int sei_cpb_removal_delay
cpb_removal_delay in picture timing SEI message, see H.264 C.1.2
Definition: h264.h:608
int ff_h264_check_intra4x4_pred_mode(H264Context *h)
Check if the top & left blocks are available if needed & change the dc mode so it only uses the avail...
Definition: h264.c:426
Definition: error_resilience.h:40
int ff_h264_get_slice_type(const H264Context *h)
Reconstruct bitstream slice_type.
Definition: h264.c:3894
Definition: h264.h:118
Definition: parser.h:28
mpegvideo header.
static av_always_inline void write_back_motion(H264Context *h, int mb_type)
Definition: h264.h:941
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
H.264 DSP functions.
void ff_h264_init_cabac_states(H264Context *h)
Definition: h264_cabac.c:1260
void ff_h264_free_context(H264Context *h)
Free any data that may have been allocated in the H264 context like SPS, PPS etc. ...
Definition: h264.c:4978
static av_always_inline int pred_intra_mode(H264Context *h, int n)
Get the predicted intra4x4 prediction mode.
Definition: h264.h:857
#define USES_LIST(a, list)
does this mb use listX, note does not work if subMBs
Definition: mpegvideo.h:156
int ff_h264_decode_ref_pic_list_reordering(H264Context *h)
Definition: h264_refs.c:205
int deblocking_filter_parameters_present
deblocking_filter_parameters_present_flag
Definition: h264.h:225
void ff_h264_reset_sei(H264Context *h)
Reset SEI values at the beginning of the frame.
Definition: h264_sei.c:40
Definition: h264.h:117
Definition: h264chroma.h:26
void ff_h264_fill_mbaff_ref_list(H264Context *h)
Definition: h264_refs.c:327
bitstream reader API header.
static void fill_rectangle(SDL_Surface *screen, int x, int y, int w, int h, int color, int update)
Definition: ffplay.c:489
Definition: h264.h:112
Definition: buffer_internal.h:74
Definition: h264.h:244
Definition: h264qpel.h:27
const uint8_t ff_h264_chroma_qp[7][QP_MAX_NUM+1]
One chroma qp table for each possible bit depth (8-14).
Definition: h264_ps.c:75
Definition: h264.h:242
Definition: h264.h:241
void ff_h264_direct_ref_list_init(H264Context *const h)
Definition: h264_direct.c:103
static av_always_inline int get_chroma_qp(H264Context *h, int t, int qscale)
Get the chroma qp.
Definition: h264.h:849
int ff_h264_decode_extradata(H264Context *h, const uint8_t *buf, int size)
Definition: h264.c:1398
Definition: h264.h:116
int prev_interlaced_frame
Complement sei_pic_struct SEI_PIC_STRUCT_TOP_BOTTOM and SEI_PIC_STRUCT_BOTTOM_TOP indicate interlaced...
Definition: h264.h:591
static av_always_inline void write_back_motion_list(H264Context *h, int b_stride, int b_xy, int b8_xy, int mb_type, int list)
Definition: h264.h:906
useful rectangle filling function
void ff_h264_pred_direct_motion(H264Context *const h, int *mb_type)
Definition: h264_direct.c:623
Definition: cabac.h:42
void ff_h264_filter_mb(H264Context *h, int mb_x, int mb_y, uint8_t *img_y, uint8_t *img_cb, uint8_t *img_cr, unsigned int linesize, unsigned int uvlinesize)
Definition: h264_loopfilter.c:704
int initial_cpb_removal_delay_length
initial_cpb_removal_delay_length_minus1 + 1
Definition: h264.h:200
Definition: h264.h:119
Definition: h264.h:115
Definition: h264.h:113
uint8_t chroma_qp_table[2][QP_MAX_NUM+1]
pre-scaled (with chroma_qp_index_offset) version of qp_table
Definition: h264.h:231
int ff_h264_decode_picture_parameter_set(H264Context *h, int bit_length)
Decode PPS.
Definition: h264_ps.c:567
int ff_generate_sliding_window_mmcos(H264Context *h, int first_slice)
Definition: h264_refs.c:531
unsigned int topright_samples_available
Definition: h264.h:321
static av_always_inline void write_back_non_zero_count(H264Context *h)
Definition: h264.h:883
Definition: h264.h:110
int ff_h264_execute_ref_pic_marking(H264Context *h, MMCO *mmco, int mmco_count)
Execute the reference picture marking (memory management control operations).
Definition: h264_refs.c:563
user data registered by ITU-T Recommendation T.35
Definition: h264.h:128
int recovery_frame
recovery_frame is the frame_num at which the next frame should be fully constructed.
Definition: h264.h:624
Definition: h264.h:108
Definition: h264.h:239
Definition: h264.h:106
Definition: h264.h:109
int ff_h264_decode_ref_pic_marking(H264Context *h, GetBitContext *gb, int first_slice)
Definition: h264_refs.c:745
6: bottom field, top field, bottom field repeated, in that order
Definition: h264.h:143
H.264 / AVC / MPEG4 prediction functions.
void ff_h264_filter_mb_fast(H264Context *h, int mb_x, int mb_y, uint8_t *img_y, uint8_t *img_cb, uint8_t *img_cr, unsigned int linesize, unsigned int uvlinesize)
Definition: h264_loopfilter.c:416
Definition: get_bits.h:54
const uint8_t * ff_h264_decode_nal(H264Context *h, const uint8_t *src, int *dst_length, int *consumed, int length)
Decode a network abstraction layer unit.
Definition: h264.c:514
int sei_ct_type
Bit set of clock types for fields/frames in picture timing SEI message.
Definition: h264.h:598
static av_always_inline void write_back_intra_pred_mode(H264Context *h)
Definition: h264.h:872
Definition: h264.h:105
Definition: h264.h:114
int current_slice
current slice number, used to initialize slice_num of each thread/context
Definition: h264.h:556
Definition: h264.h:245
int ff_h264_fill_default_ref_list(H264Context *h)
Fill the default_ref_list.
Definition: h264_refs.c:117
Definition: h264.h:107
Definition: h264.h:111
int ff_h264_check_intra_pred_mode(H264Context *h, int mode, int is_chroma)
Check if the top & left blocks are available if needed & change the dc mode so it only uses the avail...
Definition: h264.c:473
void ff_h264_draw_horiz_band(H264Context *h, int y, int height)
Definition: h264.c:147
int sei_dpb_output_delay
dpb_output_delay in picture timing SEI message, see H.264 C.2.2
Definition: h264.h:603
Context Adaptive Binary Arithmetic Coder.
int neighbor_transform_size
number of neighbors (top and/or left) that used 8x8 dct
Definition: h264.h:344
Generated on Fri Aug 1 2025 06:53:41 for FFmpeg by
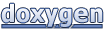