FFmpeg
|
h264_refs.c
Go to the documentation of this file.
136 len += build_def_list(h->default_ref_list[list] + len, h->long_ref, 16, 1, h->picture_structure);
145 for (i = 0; h->default_ref_list[0][i].f.data[0] == h->default_ref_list[1][i].f.data[0] && i < lens[0]; i++);
154 len = build_def_list(h->default_ref_list[0], h->short_ref, h->short_ref_count, 0, h->picture_structure);
311 av_log(h->avctx, AV_LOG_ERROR, "Missing reference picture, default is %d\n", h->default_ref_list[list][0].poc);
345 h->luma_weight[16 + 2 * i][list][0] = h->luma_weight[16 + 2 * i + 1][list][0] = h->luma_weight[i][list][0];
346 h->luma_weight[16 + 2 * i][list][1] = h->luma_weight[16 + 2 * i + 1][list][1] = h->luma_weight[i][list][1];
348 h->chroma_weight[16 + 2 * i][list][j][0] = h->chroma_weight[16 + 2 * i + 1][list][j][0] = h->chroma_weight[i][list][j][0];
349 h->chroma_weight[16 + 2 * i][list][j][1] = h->chroma_weight[16 + 2 * i + 1][list][j][1] = h->chroma_weight[i][list][j][1];
633 av_log(h->avctx, AV_LOG_ERROR, "mmco: cannot assign current picture to 2 long term references\n");
640 av_log(h->avctx, AV_LOG_ERROR, "mmco: cannot assign current picture to short and long at the same time\n");
736 if(err >= 0 && h->long_ref_count==0 && h->short_ref_count<=2 && h->pps.ref_count[0]<=1 + (h->picture_structure != PICT_FRAME) && h->cur_pic_ptr->f.pict_type == AV_PICTURE_TYPE_I){
Picture default_ref_list[2][32]
base reference list for all slices of a coded picture
Definition: h264.h:526
static int pic_num_extract(H264Context *h, int pic_num, int *structure)
Extract structure information about the picture described by pic_num in the current decoding context ...
Definition: h264_refs.c:192
Definition: h264.h:243
Definition: h264.h:240
AVBufferRef * buf[AV_NUM_DATA_POINTERS]
AVBuffer references backing the data for this frame.
Definition: frame.h:343
static int check_opcodes(MMCO *mmco1, MMCO *mmco2, int n_mmcos)
Definition: h264_refs.c:516
int ff_h264_fill_default_ref_list(H264Context *h)
Fill the default_ref_list.
Definition: h264_refs.c:117
#define DELAYED_PIC_REF
Value of Picture.reference when Picture is not a reference picture, but is held for delayed output...
Definition: libavcodec/diracdec.c:73
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
void ff_h264_fill_mbaff_ref_list(H264Context *h)
Definition: h264_refs.c:327
int ff_h264_decode_ref_pic_list_reordering(H264Context *h)
Definition: h264_refs.c:205
int mmco_reset
h264 MMCO_RESET set this 1. Reordering code must not mix pictures before and after MMCO_RESET...
Definition: mpegvideo.h:162
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
int ff_h264_decode_ref_pic_marking(H264Context *h, GetBitContext *gb, int first_slice)
Definition: h264_refs.c:745
int ff_generate_sliding_window_mmcos(H264Context *h, int first_slice)
Definition: h264_refs.c:531
Definition: h264.h:244
H.264 / AVC / MPEG4 part10 codec.
Definition: h264.h:242
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
Definition: h264.h:241
simple assert() macros that are a bit more flexible than ISO C assert().
external API header
static int add_sorted(Picture **sorted, Picture **src, int len, int limit, int dir)
Definition: h264_refs.c:95
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
static Picture * remove_short(H264Context *h, int frame_num, int ref_mask)
Definition: h264_refs.c:424
static void remove_short_at_index(H264Context *h, int i)
Remove a picture from the short term reference list by its index in that list.
Definition: h264_refs.c:411
int ff_h264_execute_ref_pic_marking(H264Context *h, MMCO *mmco, int mmco_count)
Execute the reference picture marking (memory management control operations).
Definition: h264_refs.c:563
static int get_ue_golomb_31(GetBitContext *gb)
read unsigned exp golomb code, constraint to a max of 31.
Definition: golomb.h:100
int linesize[AV_NUM_DATA_POINTERS]
For video, size in bytes of each picture line.
Definition: frame.h:101
Definition: h264.h:239
int av_buffer_get_ref_count(const AVBufferRef *buf)
Definition: libavutil/buffer.c:133
Definition: h264.h:109
static int split_field_copy(Picture *dest, Picture *src, int parity, int id_add)
Definition: h264_refs.c:56
static Picture * find_short(H264Context *h, int frame_num, int *idx)
Find a Picture in the short term reference list by frame number.
Definition: h264_refs.c:389
Definition: get_bits.h:54
common internal api header.
static Picture * remove_long(H264Context *h, int i, int ref_mask)
Remove a picture from the long term reference list by its index in that list.
Definition: h264_refs.c:446
int pic_id
h264 pic_num (short -> no wrap version of pic_num, pic_num & max_pic_num; long -> long_pic_num) ...
Definition: mpegvideo.h:163
Definition: h264.h:245
static int unreference_pic(H264Context *h, Picture *pic, int refmask)
Mark a picture as no longer needed for reference.
Definition: h264_refs.c:366
exp golomb vlc stuff
static int build_def_list(Picture *def, Picture **in, int len, int is_long, int sel)
Definition: h264_refs.c:72
Generated on Mon Jun 30 2025 06:53:08 for FFmpeg by
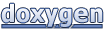