FFmpeg
|
golomb.h
Go to the documentation of this file.
397 av_log(NULL, AV_LOG_DEBUG, "%5d %2d %3d ue @%5d in %s %s:%d\n", bits, len, i, pos, file, func, line);
413 av_log(NULL, AV_LOG_DEBUG, "%5d %2d %3d se @%5d in %s %s:%d\n", bits, len, i, pos, file, func, line);
427 av_log(NULL, AV_LOG_DEBUG, "%5d %2d %3d te @%5d in %s %s:%d\n", bits, len, i, pos, file, func, line);
509 static inline void set_ur_golomb_jpegls(PutBitContext *pb, int i, int k, int limit, int esc_len){
static unsigned int show_bits_long(GetBitContext *s, int n)
Show 0-32 bits.
Definition: get_bits.h:352
static int get_sr_golomb_flac(GetBitContext *gb, int k, int limit, int esc_len)
read signed golomb rice code (flac).
Definition: golomb.h:358
static void set_ur_golomb(PutBitContext *pb, int i, int k, int limit, int esc_len)
write unsigned golomb rice code (ffv1).
Definition: golomb.h:493
Definition: put_bits.h:41
static void set_ue_golomb(PutBitContext *pb, int i)
write unsigned exp golomb code.
Definition: golomb.h:442
const int8_t ff_interleaved_se_golomb_vlc_code[256]
Definition: golomb.c:138
const uint8_t ff_interleaved_golomb_vlc_len[256]
Definition: golomb.c:100
static int get_te0_golomb(GetBitContext *gb, int range)
read unsigned truncated exp golomb code.
Definition: golomb.h:153
static int get_ur_golomb_jpegls(GetBitContext *gb, int k, int limit, int esc_len)
read unsigned golomb rice code (jpegls).
Definition: golomb.h:294
const uint8_t ff_interleaved_dirac_golomb_vlc_code[256]
Definition: golomb.c:157
#define av_assert2(cond)
assert() equivalent, that does lie in speed critical code.
Definition: avassert.h:63
static void set_te_golomb(PutBitContext *pb, int i, int range)
write truncated unsigned exp golomb code.
Definition: golomb.h:465
bitstream reader API header.
static int get_ur_golomb(GetBitContext *gb, int k, int limit, int esc_len)
read unsigned golomb rice code (ffv1).
Definition: golomb.h:261
Definition: graph2dot.c:48
static void put_bits(J2kEncoderContext *s, int val, int n)
put n times val bit
Definition: j2kenc.c:160
FFmpeg Automated Testing Environment ************************************Table of Contents *****************FFmpeg Automated Testing Environment Introduction Using FATE from your FFmpeg source directory Submitting the results to the FFmpeg result aggregation server FATE makefile targets and variables Makefile targets Makefile variables Examples Introduction **************FATE is an extended regression suite on the client side and a means for results aggregation and presentation on the server side The first part of this document explains how you can use FATE from your FFmpeg source directory to test your ffmpeg binary The second part describes how you can run FATE to submit the results to FFmpeg s FATE server In any way you can have a look at the publicly viewable FATE results by visiting this as it can be seen if some test on some platform broke with their recent contribution This usually happens on the platforms the developers could not test on The second part of this document describes how you can run FATE to submit your results to FFmpeg s FATE server If you want to submit your results be sure to check that your combination of OS and compiler is not already listed on the above mentioned website In the third part you can find a comprehensive listing of FATE makefile targets and variables Using FATE from your FFmpeg source directory **********************************************If you want to run FATE on your machine you need to have the samples in place You can get the samples via the build target fate rsync Use this command from the top level source this will cause FATE to fail NOTE To use a custom wrapper to run the pass target exec to configure or set the TARGET_EXEC Make variable Submitting the results to the FFmpeg result aggregation server ****************************************************************To submit your results to the server you should run fate through the shell script tests fate sh from the FFmpeg sources This script needs to be invoked with a configuration file as its first argument tests fate sh path to fate_config A configuration file template with comments describing the individual configuration variables can be found at doc fate_config sh template Create a configuration that suits your based on the configuration template The slot configuration variable can be any string that is not yet but it is suggested that you name it adhering to the following pattern< arch >< os >< compiler >< compiler version > The configuration file itself will be sourced in a shell therefore all shell features may be used This enables you to setup the environment as you need it for your build For your first test runs the fate_recv variable should be empty or commented out This will run everything as normal except that it will omit the submission of the results to the server The following files should be present in $workdir as specified in the configuration file
Definition: fate.txt:34
static void set_ur_golomb_jpegls(PutBitContext *pb, int i, int k, int limit, int esc_len)
write unsigned golomb rice code (jpegls).
Definition: golomb.h:509
static void set_sr_golomb_flac(PutBitContext *pb, int i, int k, int limit, int esc_len)
write signed golomb rice code (flac).
Definition: golomb.h:548
static unsigned get_ue_golomb_long(GetBitContext *gb)
Read an unsigned Exp-Golomb code in the range 0 to UINT32_MAX-1.
Definition: golomb.h:85
static void set_se_golomb(PutBitContext *pb, int i)
write signed exp golomb code.
Definition: golomb.h:476
static int get_ue_golomb_31(GetBitContext *gb)
read unsigned exp golomb code, constraint to a max of 31.
Definition: golomb.h:100
const uint8_t ff_interleaved_ue_golomb_vlc_code[256]
Definition: golomb.c:119
int(* func)(AVBPrint *dst, const char *in, const char *arg)
Definition: libavcodec/jacosubdec.c:70
static void set_sr_golomb(PutBitContext *pb, int i, int k, int limit, int esc_len)
write signed golomb rice code (ffv1).
Definition: golomb.h:536
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
Definition: get_bits.h:54
static int get_te_golomb(GetBitContext *gb, int range)
read unsigned truncated exp golomb code.
Definition: golomb.h:164
static unsigned int get_ur_golomb_shorten(GetBitContext *gb, int k)
read unsigned golomb rice code (shorten).
Definition: golomb.h:366
static int get_sr_golomb(GetBitContext *gb, int k, int limit, int esc_len)
read signed golomb rice code (ffv1).
Definition: golomb.h:345
static int get_sr_golomb_shorten(GetBitContext *gb, int k)
read signed golomb rice code (shorten).
Definition: golomb.h:373
bitstream writer API
Generated on Thu May 29 2025 06:52:48 for FFmpeg by
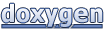