FFmpeg
|
Dirac Decoder. More...
#include "avcodec.h"
#include "dsputil.h"
#include "get_bits.h"
#include "bytestream.h"
#include "internal.h"
#include "golomb.h"
#include "dirac_arith.h"
#include "mpeg12data.h"
#include "dirac_dwt.h"
#include "dirac.h"
#include "diracdsp.h"
#include "videodsp.h"

Go to the source code of this file.
Data Structures | |
struct | DiracFrame |
struct | DiracBlock |
struct | SubBand |
struct | Plane |
struct | DiracContext |
struct | lowdelay_slice |
Macros | |
#define | MAX_DWT_LEVELS 5 |
The spec limits the number of wavelet decompositions to 4 for both level 1 (VC-2) and 128 (long-gop default). More... | |
#define | MAX_REFERENCE_FRAMES 8 |
The spec limits this to 3 for frame coding, but in practice can be as high as 6. More... | |
#define | MAX_DELAY 5 /* limit for main profile for frame coding (TODO: field coding) */ |
#define | MAX_FRAMES (MAX_REFERENCE_FRAMES + MAX_DELAY + 1) |
#define | MAX_QUANT 68 /* max quant for VC-2 */ |
#define | MAX_BLOCKSIZE 32 /* maximum xblen/yblen we support */ |
#define | DIRAC_REF_MASK_REF1 1 |
DiracBlock->ref flags, if set then the block does MC from the given ref. More... | |
#define | DIRAC_REF_MASK_REF2 2 |
#define | DIRAC_REF_MASK_GLOBAL 4 |
#define | DELAYED_PIC_REF 4 |
Value of Picture.reference when Picture is not a reference picture, but is held for delayed output. More... | |
#define | ff_emulated_edge_mc ff_emulated_edge_mc_8 /* Fix: change the calls to this function regarding bit depth */ |
#define | CALC_PADDING(size, depth) (((size + (1 << depth) - 1) >> depth) << depth) |
#define | DIVRNDUP(a, b) (((a) + (b) - 1) / (b)) |
#define | SIGN_CTX(x) (CTX_SIGN_ZERO + ((x) > 0) - ((x) < 0)) |
#define | CHECKEDREAD(dst, cond, errmsg) |
#define | ROLLOFF(i) |
#define | DATA_UNIT_HEADER_SIZE 13 |
Dirac Specification -> 9.6 Parse Info Header Syntax. More... | |
Typedefs | |
typedef struct SubBand | SubBand |
typedef struct Plane | Plane |
typedef struct DiracContext | DiracContext |
Enumerations | |
enum | dirac_parse_code { pc_seq_header = 0x00, pc_eos = 0x10, pc_aux_data = 0x20, pc_padding = 0x30 } |
Dirac Specification -> Parse code values. More... | |
enum | dirac_subband { subband_ll = 0, subband_hl = 1, subband_lh = 2, subband_hh = 3 } |
Functions | |
static int | divide3 (int x) |
static DiracFrame * | remove_frame (DiracFrame *framelist[], int picnum) |
static int | add_frame (DiracFrame *framelist[], int maxframes, DiracFrame *frame) |
static int | alloc_sequence_buffers (DiracContext *s) |
static void | free_sequence_buffers (DiracContext *s) |
static av_cold int | dirac_decode_init (AVCodecContext *avctx) |
static void | dirac_decode_flush (AVCodecContext *avctx) |
static av_cold int | dirac_decode_end (AVCodecContext *avctx) |
static void | coeff_unpack_arith (DiracArith *c, int qfactor, int qoffset, SubBand *b, IDWTELEM *buf, int x, int y) |
static int | coeff_unpack_golomb (GetBitContext *gb, int qfactor, int qoffset) |
static void | codeblock (DiracContext *s, SubBand *b, GetBitContext *gb, DiracArith *c, int left, int right, int top, int bottom, int blockcnt_one, int is_arith) |
Decode the coeffs in the rectangle defined by left, right, top, bottom [DIRAC_STD] 13.4.3.2 Codeblock unpacking loop. More... | |
static void | intra_dc_prediction (SubBand *b) |
Dirac Specification -> 13.3 intra_dc_prediction(band) More... | |
static av_always_inline void | decode_subband_internal (DiracContext *s, SubBand *b, int is_arith) |
Dirac Specification -> 13.4.2 Non-skipped subbands. More... | |
static int | decode_subband_arith (AVCodecContext *avctx, void *b) |
static int | decode_subband_golomb (AVCodecContext *avctx, void *arg) |
static void | decode_component (DiracContext *s, int comp) |
Dirac Specification -> [DIRAC_STD] 13.4.1 core_transform_data() More... | |
static void | lowdelay_subband (DiracContext *s, GetBitContext *gb, int quant, int slice_x, int slice_y, int bits_end, SubBand *b1, SubBand *b2) |
static int | decode_lowdelay_slice (AVCodecContext *avctx, void *arg) |
Dirac Specification -> 13.5.2 Slices. More... | |
static void | decode_lowdelay (DiracContext *s) |
Dirac Specification -> 13.5.1 low_delay_transform_data() More... | |
static void | init_planes (DiracContext *s) |
static int | dirac_unpack_prediction_parameters (DiracContext *s) |
Unpack the motion compensation parameters Dirac Specification -> 11.2 Picture prediction data. More... | |
static int | dirac_unpack_idwt_params (DiracContext *s) |
Dirac Specification -> 11.3 Wavelet transform data. More... | |
static int | pred_sbsplit (uint8_t *sbsplit, int stride, int x, int y) |
static int | pred_block_mode (DiracBlock *block, int stride, int x, int y, int refmask) |
static void | pred_block_dc (DiracBlock *block, int stride, int x, int y) |
static void | pred_mv (DiracBlock *block, int stride, int x, int y, int ref) |
static void | global_mv (DiracContext *s, DiracBlock *block, int x, int y, int ref) |
static void | decode_block_params (DiracContext *s, DiracArith arith[8], DiracBlock *block, int stride, int x, int y) |
static void | propagate_block_data (DiracBlock *block, int stride, int size) |
Copies the current block to the other blocks covered by the current superblock split mode. More... | |
static int | dirac_unpack_block_motion_data (DiracContext *s) |
Dirac Specification -> More... | |
static int | weight (int i, int blen, int offset) |
static void | init_obmc_weight_row (Plane *p, uint8_t *obmc_weight, int stride, int left, int right, int wy) |
static void | init_obmc_weight (Plane *p, uint8_t *obmc_weight, int stride, int left, int right, int top, int bottom) |
static void | init_obmc_weights (DiracContext *s, Plane *p, int by) |
static int | mc_subpel (DiracContext *s, DiracBlock *block, const uint8_t *src[5], int x, int y, int ref, int plane) |
For block x,y, determine which of the hpel planes to do bilinear interpolation from and set src[] to the location in each hpel plane to MC from. More... | |
static void | add_dc (uint16_t *dst, int dc, int stride, uint8_t *obmc_weight, int xblen, int yblen) |
static void | block_mc (DiracContext *s, DiracBlock *block, uint16_t *mctmp, uint8_t *obmc_weight, int plane, int dstx, int dsty) |
static void | mc_row (DiracContext *s, DiracBlock *block, uint16_t *mctmp, int plane, int dsty) |
static void | select_dsp_funcs (DiracContext *s, int width, int height, int xblen, int yblen) |
static void | interpolate_refplane (DiracContext *s, DiracFrame *ref, int plane, int width, int height) |
static int | dirac_decode_frame_internal (DiracContext *s) |
Dirac Specification -> 13.0 Transform data syntax. More... | |
static int | dirac_decode_picture_header (DiracContext *s) |
Dirac Specification -> 11.1.1 Picture Header. More... | |
static int | get_delayed_pic (DiracContext *s, AVFrame *picture, int *got_frame) |
static int | dirac_decode_data_unit (AVCodecContext *avctx, const uint8_t *buf, int size) |
static int | dirac_decode_frame (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *pkt) |
Variables | |
static const uint8_t | default_qmat [][4][4] |
static const int | qscale_tab [MAX_QUANT+1] |
static const int | qoffset_intra_tab [MAX_QUANT+1] |
static const int | qoffset_inter_tab [MAX_QUANT+1] |
static const uint8_t | epel_weights [4][4][4] |
AVCodec | ff_dirac_decoder |
Detailed Description
Dirac Decoder.
Definition in file libavcodec/diracdec.c.
Macro Definition Documentation
Definition at line 77 of file libavcodec/diracdec.c.
Referenced by alloc_sequence_buffers(), and init_planes().
#define CHECKEDREAD | ( | dst, | |
cond, | |||
errmsg | |||
) |
Referenced by dirac_unpack_idwt_params().
#define DATA_UNIT_HEADER_SIZE 13 |
Dirac Specification -> 9.6 Parse Info Header Syntax.
parse_info() 4 byte start code + byte parse code + 4 byte size + 4 byte previous size
Definition at line 1742 of file libavcodec/diracdec.c.
Referenced by dirac_decode_data_unit(), and dirac_decode_frame().
#define DELAYED_PIC_REF 4 |
Value of Picture.reference when Picture is not a reference picture, but is held for delayed output.
Definition at line 73 of file libavcodec/diracdec.c.
Referenced by decode_frame(), decode_postinit(), decode_slice_header(), dirac_decode_frame(), dirac_decode_picture_header(), get_delayed_pic(), pic_is_unused(), and unreference_pic().
#define DIRAC_REF_MASK_GLOBAL 4 |
Definition at line 67 of file libavcodec/diracdec.c.
Referenced by decode_block_params(), and pred_mv().
#define DIRAC_REF_MASK_REF1 1 |
DiracBlock->ref flags, if set then the block does MC from the given ref.
Definition at line 65 of file libavcodec/diracdec.c.
Referenced by decode_block_params().
#define DIRAC_REF_MASK_REF2 2 |
Definition at line 66 of file libavcodec/diracdec.c.
Referenced by decode_block_params().
Definition at line 80 of file libavcodec/diracdec.c.
Referenced by alloc_sequence_buffers(), and dirac_unpack_block_motion_data().
#define ff_emulated_edge_mc ff_emulated_edge_mc_8 /* Fix: change the calls to this function regarding bit depth */ |
Definition at line 75 of file libavcodec/diracdec.c.
Referenced by mc_subpel().
#define MAX_BLOCKSIZE 32 /* maximum xblen/yblen we support */ |
Definition at line 60 of file libavcodec/diracdec.c.
Referenced by add_dc(), alloc_sequence_buffers(), dirac_unpack_prediction_parameters(), ff_mlp_filter_channel(), filter_channel(), init_obmc_weights(), and read_major_sync().
Definition at line 57 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame().
#define MAX_DWT_LEVELS 5 |
The spec limits the number of wavelet decompositions to 4 for both level 1 (VC-2) and 128 (long-gop default).
5 decompositions is the maximum before >16-bit buffers are needed. Schroedinger allows this for DD 9,7 and 13,7 wavelets only, limiting the others to 4 decompositions (or 3 for the fidelity filter).
We use this instead of MAX_DECOMPOSITIONS to save some memory.
Definition at line 51 of file libavcodec/diracdec.c.
Referenced by alloc_sequence_buffers(), decode_component(), and dirac_unpack_idwt_params().
#define MAX_FRAMES (MAX_REFERENCE_FRAMES + MAX_DELAY + 1) |
Definition at line 58 of file libavcodec/diracdec.c.
Referenced by dirac_decode_data_unit(), dirac_decode_frame(), dirac_decode_picture_header(), and free_sequence_buffers().
Definition at line 59 of file libavcodec/diracdec.c.
Referenced by codeblock(), and lowdelay_subband().
#define MAX_REFERENCE_FRAMES 8 |
The spec limits this to 3 for frame coding, but in practice can be as high as 6.
Definition at line 56 of file libavcodec/diracdec.c.
Referenced by dirac_decode_picture_header().
#define SIGN_CTX | ( | x | ) | (CTX_SIGN_ZERO + ((x) > 0) - ((x) < 0)) |
Definition at line 424 of file libavcodec/diracdec.c.
Referenced by coeff_unpack_arith().
Typedef Documentation
typedef struct DiracContext DiracContext |
Enumeration Type Documentation
enum dirac_parse_code |
Dirac Specification -> Parse code values.
9.6.1 Table 9.1
Enumerator | |
---|---|
pc_seq_header | |
pc_eos | |
pc_aux_data | |
pc_padding |
Definition at line 225 of file libavcodec/diracdec.c.
enum dirac_subband |
Enumerator | |
---|---|
subband_ll | |
subband_hl | |
subband_lh | |
subband_hh |
Definition at line 232 of file libavcodec/diracdec.c.
Function Documentation
|
static |
Definition at line 1426 of file libavcodec/diracdec.c.
Referenced by block_mc().
|
static |
Definition at line 306 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame(), and dirac_decode_picture_header().
|
static |
Definition at line 317 of file libavcodec/diracdec.c.
Referenced by dirac_decode_data_unit().
|
static |
Definition at line 1442 of file libavcodec/diracdec.c.
Referenced by mc_row().
|
inlinestatic |
Decode the coeffs in the rectangle defined by left, right, top, bottom [DIRAC_STD] 13.4.3.2 Codeblock unpacking loop.
Definition at line 475 of file libavcodec/diracdec.c.
Referenced by decode_subband_internal().
|
inlinestatic |
Definition at line 426 of file libavcodec/diracdec.c.
Referenced by codeblock().
|
inlinestatic |
Definition at line 458 of file libavcodec/diracdec.c.
Referenced by codeblock(), and lowdelay_subband().
|
static |
Definition at line 1133 of file libavcodec/diracdec.c.
Referenced by dirac_unpack_block_motion_data().
|
static |
Dirac Specification -> [DIRAC_STD] 13.4.1 core_transform_data()
Definition at line 610 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame_internal().
|
static |
Dirac Specification -> 13.5.1 low_delay_transform_data()
Definition at line 737 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame_internal().
|
static |
Dirac Specification -> 13.5.2 Slices.
slice(sx,sy)
Definition at line 695 of file libavcodec/diracdec.c.
Referenced by decode_lowdelay().
|
static |
Definition at line 591 of file libavcodec/diracdec.c.
Referenced by decode_component().
|
static |
Definition at line 598 of file libavcodec/diracdec.c.
Referenced by decode_component().
|
static |
Dirac Specification -> 13.4.2 Non-skipped subbands.
subband_coeffs()
Definition at line 558 of file libavcodec/diracdec.c.
Referenced by decode_subband_arith(), and decode_subband_golomb().
|
static |
Definition at line 1746 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame().
|
static |
Definition at line 418 of file libavcodec/diracdec.c.
|
static |
Definition at line 410 of file libavcodec/diracdec.c.
Referenced by dirac_decode_end().
|
static |
Definition at line 1832 of file libavcodec/diracdec.c.
|
static |
Dirac Specification -> 13.0 Transform data syntax.
transform_data()
Definition at line 1550 of file libavcodec/diracdec.c.
Referenced by dirac_decode_data_unit().
|
static |
Definition at line 393 of file libavcodec/diracdec.c.
|
static |
Dirac Specification -> 11.1.1 Picture Header.
picture_header()
Definition at line 1635 of file libavcodec/diracdec.c.
Referenced by dirac_decode_data_unit().
|
static |
Dirac Specification ->
- Block motion data syntax
Definition at line 1192 of file libavcodec/diracdec.c.
Referenced by dirac_decode_picture_header().
|
static |
Dirac Specification -> 11.3 Wavelet transform data.
wavelet_transform()
Definition at line 940 of file libavcodec/diracdec.c.
Referenced by dirac_decode_picture_header().
|
static |
Unpack the motion compensation parameters Dirac Specification -> 11.2 Picture prediction data.
picture_prediction()
Definition at line 828 of file libavcodec/diracdec.c.
Referenced by dirac_decode_picture_header().
|
inlinestatic |
Definition at line 283 of file libavcodec/diracdec.c.
Referenced by intra_dc_prediction(), and pred_block_dc().
|
static |
Definition at line 362 of file libavcodec/diracdec.c.
Referenced by dirac_decode_data_unit(), and dirac_decode_flush().
|
static |
Definition at line 1711 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame().
|
static |
Definition at line 1117 of file libavcodec/diracdec.c.
Referenced by decode_block_params().
|
static |
Definition at line 1273 of file libavcodec/diracdec.c.
Referenced by init_obmc_weights().
|
static |
Definition at line 1259 of file libavcodec/diracdec.c.
Referenced by init_obmc_weight().
|
static |
Definition at line 1292 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame_internal().
|
static |
Definition at line 775 of file libavcodec/diracdec.c.
Referenced by dirac_decode_picture_header().
|
static |
Definition at line 1514 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame_internal().
Dirac Specification -> 13.3 intra_dc_prediction(band)
Definition at line 534 of file libavcodec/diracdec.c.
Referenced by decode_lowdelay(), and decode_subband_internal().
|
static |
Definition at line 646 of file libavcodec/diracdec.c.
Referenced by decode_lowdelay_slice().
|
static |
Definition at line 1478 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame_internal().
|
static |
For block x,y, determine which of the hpel planes to do bilinear interpolation from and set src[] to the location in each hpel plane to MC from.
- Returns
- the index of the put_dirac_pixels_tab function to use 0 for 1 plane (fpel,hpel), 1 for 2 planes (qpel), 2 for 4 planes (qpel), and 3 for epel
Definition at line 1332 of file libavcodec/diracdec.c.
Referenced by block_mc().
|
inlinestatic |
Definition at line 1048 of file libavcodec/diracdec.c.
Referenced by decode_block_params().
|
inlinestatic |
Definition at line 1032 of file libavcodec/diracdec.c.
Referenced by decode_block_params().
|
inlinestatic |
Definition at line 1081 of file libavcodec/diracdec.c.
Referenced by decode_block_params(), decode_q_branch(), encode_q_branch(), encode_q_branch2(), and get_block_bits().
|
inlinestatic |
Definition at line 1018 of file libavcodec/diracdec.c.
Referenced by dirac_unpack_block_motion_data().
|
static |
Copies the current block to the other blocks covered by the current superblock split mode.
Definition at line 1173 of file libavcodec/diracdec.c.
Referenced by dirac_unpack_block_motion_data().
|
static |
Definition at line 288 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame(), and dirac_decode_picture_header().
|
static |
Definition at line 1494 of file libavcodec/diracdec.c.
Referenced by dirac_decode_frame_internal().
|
static |
Definition at line 1247 of file libavcodec/diracdec.c.
Referenced by dv_init_enc_block(), encode_mb_internal(), ff_lpc_calc_coefs(), guess_dc(), init_obmc_weight(), init_obmc_weight_row(), interpolate_lpc(), mc_block(), try_8x8basis(), and weight_h264_W_altivec().
Variable Documentation
|
static |
Definition at line 239 of file libavcodec/diracdec.c.
Referenced by dirac_unpack_idwt_params().
|
static |
Definition at line 1305 of file libavcodec/diracdec.c.
Referenced by mc_subpel().
AVCodec ff_dirac_decoder |
Definition at line 1927 of file libavcodec/diracdec.c.
|
static |
Definition at line 271 of file libavcodec/diracdec.c.
Referenced by codeblock().
|
static |
Definition at line 260 of file libavcodec/diracdec.c.
Referenced by codeblock(), and lowdelay_subband().
|
static |
Definition at line 249 of file libavcodec/diracdec.c.
Referenced by codeblock(), and lowdelay_subband().
Generated on Wed May 8 2024 06:52:17 for FFmpeg by
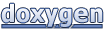