FFmpeg
|
libavformat API example. More...
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <math.h>
#include <libavutil/mathematics.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
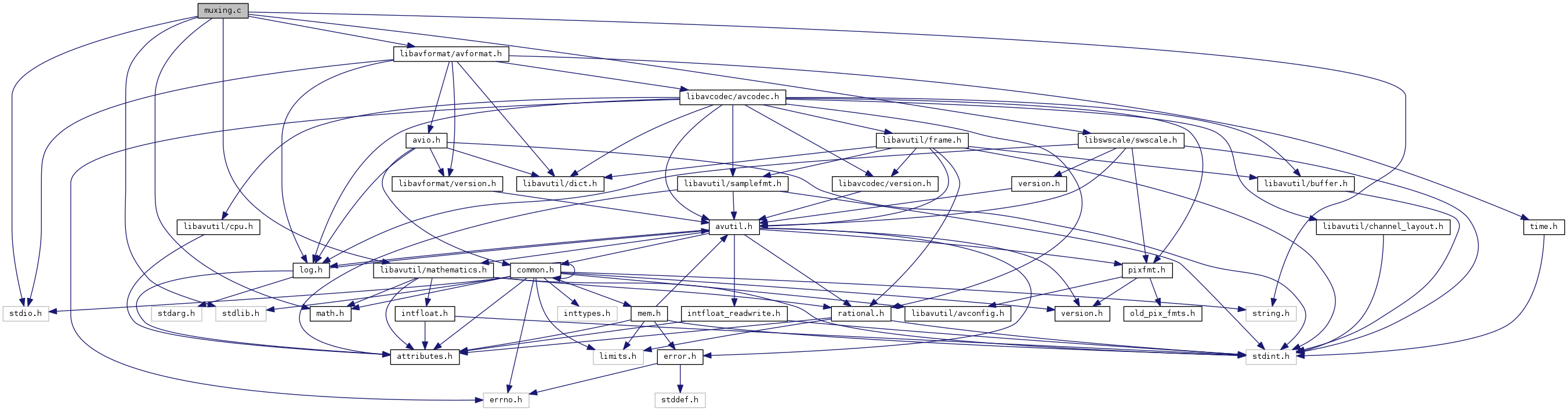
Go to the source code of this file.
Macros | |
#define | STREAM_DURATION 200.0 |
#define | STREAM_FRAME_RATE 25 /* 25 images/s */ |
#define | STREAM_NB_FRAMES ((int)(STREAM_DURATION * STREAM_FRAME_RATE)) |
#define | STREAM_PIX_FMT AV_PIX_FMT_YUV420P /* default pix_fmt */ |
Functions | |
static AVStream * | add_stream (AVFormatContext *oc, AVCodec **codec, enum AVCodecID codec_id) |
static void | open_audio (AVFormatContext *oc, AVCodec *codec, AVStream *st) |
static void | get_audio_frame (int16_t *samples, int frame_size, int nb_channels) |
static void | write_audio_frame (AVFormatContext *oc, AVStream *st) |
static void | close_audio (AVFormatContext *oc, AVStream *st) |
static void | open_video (AVFormatContext *oc, AVCodec *codec, AVStream *st) |
static void | fill_yuv_image (AVPicture *pict, int frame_index, int width, int height) |
static void | write_video_frame (AVFormatContext *oc, AVStream *st) |
static void | close_video (AVFormatContext *oc, AVStream *st) |
int | main (int argc, char **argv) |
Variables | |
static int | sws_flags = SWS_BICUBIC |
static float | t |
static float | tincr |
static float | tincr2 |
static int16_t * | samples |
static int | audio_input_frame_size |
static AVFrame * | frame |
static AVPicture | src_picture |
static AVPicture | dst_picture |
static int | frame_count |
Detailed Description
libavformat API example.
Output a media file in any supported libavformat format. The default codecs are used.
Definition in file muxing.c.
Macro Definition Documentation
#define STREAM_FRAME_RATE 25 /* 25 images/s */ |
Definition at line 43 of file muxing.c.
Referenced by add_stream().
#define STREAM_NB_FRAMES ((int)(STREAM_DURATION * STREAM_FRAME_RATE)) |
Definition at line 44 of file muxing.c.
Referenced by write_video_frame().
#define STREAM_PIX_FMT AV_PIX_FMT_YUV420P /* default pix_fmt */ |
Definition at line 45 of file muxing.c.
Referenced by add_stream().
Function Documentation
|
static |
|
static |
|
static |
Definition at line 279 of file muxing.c.
Referenced by write_video_frame().
|
static |
Definition at line 168 of file muxing.c.
Referenced by write_audio_frame().
|
static |
|
static |
|
static |
|
static |
Variable Documentation
|
static |
Definition at line 54 of file muxing.c.
Referenced by add_stream(), open_audio(), and write_audio_frame().
|
static |
Definition at line 233 of file muxing.c.
Referenced by open_video().
|
static |
Definition at line 232 of file muxing.c.
Referenced by write_audio_frame().
|
static |
Definition at line 234 of file muxing.c.
Referenced by main(), rl2_read_header(), video_decode_example(), write_video_frame(), and yop_read_seek().
|
static |
Definition at line 53 of file muxing.c.
Referenced by add_stream(), close_audio(), get_audio_frame(), open_audio(), and write_audio_frame().
|
static |
Definition at line 47 of file muxing.c.
Referenced by write_video_frame().
|
static |
Definition at line 52 of file muxing.c.
Referenced by add_stream(), get_audio_frame(), and open_audio().
|
static |
Definition at line 52 of file muxing.c.
Referenced by add_stream(), audio_encode_example(), fill_samples(), get_audio_frame(), and open_audio().
|
static |
Definition at line 52 of file muxing.c.
Referenced by add_stream(), get_audio_frame(), and open_audio().
Generated on Tue Jan 21 2025 06:52:35 for FFmpeg by
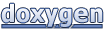