FFmpeg
|
dirac_dwt.h
Go to the documentation of this file.
42 typedef void (*vertical_compose_5tap)(IDWTELEM *b0, IDWTELEM *b1, IDWTELEM *b2, IDWTELEM *b3, IDWTELEM *b4, int width);
Definition: dirac_dwt.h:73
struct DWTContext DWTContext
Definition: dirac_dwt.h:72
void(* vertical_compose_2tap)(IDWTELEM *b0, IDWTELEM *b1, int width)
Definition: dirac_dwt.h:40
Definition: dirac_dwt.h:67
int ff_spatial_idwt2(IDWTELEM *buffer, int width, int height, int stride, enum dwt_type type, int decomposition_count, IDWTELEM *temp)
Definition: dirac_dwt.c:572
void(* vertical_compose_3tap)(IDWTELEM *b0, IDWTELEM *b1, IDWTELEM *b2, int width)
Definition: dirac_dwt.h:41
Definition: dirac_dwt.h:71
int ff_spatial_idwt_init2(DWTContext *d, IDWTELEM *buffer, int width, int height, int stride, enum dwt_type type, int decomposition_count, IDWTELEM *temp)
Definition: dirac_dwt.c:461
Definition: dirac_dwt.h:70
Definition: dirac_dwt.h:45
void(* vertical_compose_9tap)(IDWTELEM *dst, IDWTELEM *b[8], int width)
Definition: dirac_dwt.h:43
struct DWTCompose DWTCompose
void(* horizontal_compose)(IDWTELEM *b, IDWTELEM *tmp, int width)
Definition: dirac_dwt.h:60
Definition: dirac_dwt.h:75
#define type
Definition: dirac_dwt.h:74
void(* vertical_compose_5tap)(IDWTELEM *b0, IDWTELEM *b1, IDWTELEM *b2, IDWTELEM *b3, IDWTELEM *b4, int width)
Definition: dirac_dwt.h:42
Definition: dirac_dwt.h:68
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
Definition: dirac_dwt.h:32
Definition: dirac_dwt.h:66
Definition: dirac_dwt.h:69
Generated on Thu Jun 19 2025 06:53:06 for FFmpeg by
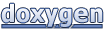