FFmpeg
|
h264_parser.c
Go to the documentation of this file.
71 while(i<next_avc && !((~*(const uint64_t*)(buf+i) & (*(const uint64_t*)(buf+i) - 0x0101010101010101ULL)) & 0x8080808080808080ULL))
74 while(i<next_avc && !((~*(const uint32_t*)(buf+i) & (*(const uint32_t*)(buf+i) - 0x01010101U)) & 0x80808080U))
361 /* if((state&0xFFFFFF1F) == 0x101 || (state&0xFFFFFF1F) == 0x102 || (state&0xFFFFFF1F) == 0x105){
363 if((state&0xFFFFFF00) == 0x100 && (state&0xFFFFFF1F) != 0x107 && (state&0xFFFFFF1F) != 0x108 && (state&0xFFFFFF1F) != 0x109){
5: top field, bottom field, top field repeated, in that order
Definition: h264.h:142
int sei_cpb_removal_delay
cpb_removal_delay in picture timing SEI message, see H.264 C.1.2
Definition: h264.h:608
const uint8_t * ff_h264_decode_nal(H264Context *h, const uint8_t *src, int *dst_length, int *consumed, int length)
Decode a network abstraction layer unit.
Definition: h264.c:514
Definition: parser.h:28
int ff_h264_get_profile(SPS *sps)
Compute profile from profile_idc and constraint_set?_flags.
Definition: h264.c:2897
int dts_ref_dts_delta
Offset of the current timestamp against last timestamp sync point in units of AVCodecContext.time_base.
Definition: libavcodec/avcodec.h:3842
Definition: libavcodec/avcodec.h:130
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
Definition: h264.h:112
const uint8_t * avpriv_find_start_code(const uint8_t *p, const uint8_t *end, uint32_t *state)
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
int ff_combine_frame(ParseContext *pc, int next, const uint8_t **buf, int *buf_size)
Combine the (truncated) bitstream to a complete frame.
Definition: parser.c:214
Definition: libavcodec/avcodec.h:3883
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
static int parse_nal_units(AVCodecParserContext *s, AVCodecContext *avctx, const uint8_t *buf, int buf_size)
Parse NAL units of found picture and decode some basic information.
Definition: h264_parser.c:141
int ff_h264_decode_picture_parameter_set(H264Context *h, int bit_length)
Decode PPS.
Definition: h264_ps.c:567
Note except for filters that can have queued request_frame does not push and as a the filter_frame method will be called and do the work Legacy the filter_frame method was split
Definition: filter_design.txt:263
Definition: libavcodec/avcodec.h:3747
static unsigned get_ue_golomb_long(GetBitContext *gb)
Read an unsigned Exp-Golomb code in the range 0 to UINT32_MAX-1.
Definition: golomb.h:85
int pts_dts_delta
Presentation delay of current frame in units of AVCodecContext.time_base.
Definition: libavcodec/avcodec.h:3856
Definition: h264.h:110
H264 / AVC / MPEG4 part10 codec data table
static int get_ue_golomb_31(GetBitContext *gb)
read unsigned exp golomb code, constraint to a max of 31.
Definition: golomb.h:100
static int h264_split(AVCodecContext *avctx, const uint8_t *buf, int buf_size)
Definition: h264_parser.c:351
Definition: h264.h:109
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
6: bottom field, top field, bottom field repeated, in that order
Definition: h264.h:143
av_cold void ff_h264_free_context(H264Context *h)
Free any data that may have been allocated in the H264 context like SPS, PPS etc. ...
Definition: h264.c:4978
Definition: get_bits.h:54
common internal api header.
int ff_h264_decode_extradata(H264Context *h, const uint8_t *buf, int size)
Definition: h264.c:1398
Definition: h264.h:105
int repeat_pict
This field is used for proper frame duration computation in lavf.
Definition: libavcodec/avcodec.h:3765
static int h264_parse(AVCodecParserContext *s, AVCodecContext *avctx, const uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size)
Definition: h264_parser.c:290
exp golomb vlc stuff
Definition: h264.h:111
int key_frame
Set by parser to 1 for key frames and 0 for non-key frames.
Definition: libavcodec/avcodec.h:3796
int dts_sync_point
Synchronization point for start of timestamp generation.
Definition: libavcodec/avcodec.h:3827
static int ff_h264_find_frame_end(H264Context *h, const uint8_t *buf, int buf_size)
Definition: h264_parser.c:36
int sei_dpb_output_delay
dpb_output_delay in picture timing SEI message, see H.264 C.2.2
Definition: h264.h:603
Generated on Wed May 7 2025 06:52:41 for FFmpeg by
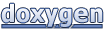