FFmpeg
|
parser.c
Go to the documentation of this file.
153 index = s->parser->parser_parse(s, avctx, (const uint8_t **)poutbuf, poutbuf_size, buf, buf_size);
236 void* new_buffer = av_fast_realloc(pc->buffer, &pc->buffer_size, (*buf_size) + pc->index + FF_INPUT_BUFFER_PADDING_SIZE);
251 void* new_buffer = av_fast_realloc(pc->buffer, &pc->buffer_size, next + pc->index + FF_INPUT_BUFFER_PADDING_SIZE);
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
Definition: libavcodec/avcodec.h:100
void(* parser_close)(AVCodecParserContext *s)
Definition: libavcodec/avcodec.h:3891
memory handling functions
int64_t next_frame_offset
Definition: libavcodec/avcodec.h:3753
Definition: parser.h:28
int(* split)(AVCodecContext *avctx, const uint8_t *buf, int buf_size)
Definition: libavcodec/avcodec.h:3892
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
int64_t cur_frame_pos[AV_PARSER_PTS_NB]
Position of the packet in file.
Definition: libavcodec/avcodec.h:3863
int dts_ref_dts_delta
Offset of the current timestamp against last timestamp sync point in units of AVCodecContext.time_base.
Definition: libavcodec/avcodec.h:3842
int ff_mpeg4video_split(AVCodecContext *avctx, const uint8_t *buf, int buf_size)
Definition: parser.c:288
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
int av_parser_change(AVCodecParserContext *s, AVCodecContext *avctx, uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size, int keyframe)
Definition: parser.c:169
#define CODEC_FLAG_GLOBAL_HEADER
Place global headers in extradata instead of every keyframe.
Definition: libavcodec/avcodec.h:714
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
void ff_fetch_timestamp(AVCodecParserContext *s, int off, int remove)
Fetch timestamps for a specific byte within the current access unit.
Definition: parser.c:88
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
void * av_fast_realloc(void *ptr, unsigned int *size, size_t min_size)
Reallocate the given block if it is not large enough, otherwise do nothing.
Definition: libavcodec/utils.c:63
int ff_combine_frame(ParseContext *pc, int next, const uint8_t **buf, int *buf_size)
Combine the (truncated) bitstream to a complete frame.
Definition: parser.c:214
int64_t cur_frame_dts[AV_PARSER_PTS_NB]
Definition: libavcodec/avcodec.h:3778
int(* parser_parse)(AVCodecParserContext *s, AVCodecContext *avctx, const uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size)
Definition: libavcodec/avcodec.h:3887
Definition: libavcodec/avcodec.h:3883
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
int overread
the number of bytes which where irreversibly read from the next frame
Definition: parser.h:35
int av_parser_parse2(AVCodecParserContext *s, AVCodecContext *avctx, uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size, int64_t pts, int64_t dts, int64_t pos)
Parse a packet.
Definition: parser.c:112
int64_t convergence_duration
Time difference in stream time base units from the pts of this packet to the point at which the outpu...
Definition: libavcodec/avcodec.h:3815
int64_t cur_frame_end[AV_PARSER_PTS_NB]
Definition: libavcodec/avcodec.h:3788
int64_t cur_frame_pts[AV_PARSER_PTS_NB]
Definition: libavcodec/avcodec.h:3777
Definition: libavcodec/avcodec.h:3747
int pts_dts_delta
Presentation delay of current frame in units of AVCodecContext.time_base.
Definition: libavcodec/avcodec.h:3856
#define CODEC_FLAG2_LOCAL_HEADER
Place global headers at every keyframe instead of in extradata.
Definition: libavcodec/avcodec.h:723
int cur_frame_start_index
Definition: libavcodec/avcodec.h:3775
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int(* parser_init)(AVCodecParserContext *s)
Definition: libavcodec/avcodec.h:3886
#define PARSER_FLAG_FETCHED_OFFSET
Set if the parser has a valid file offset.
Definition: libavcodec/avcodec.h:3784
int64_t cur_frame_offset[AV_PARSER_PTS_NB]
Definition: libavcodec/avcodec.h:3776
int key_frame
Set by parser to 1 for key frames and 0 for non-key frames.
Definition: libavcodec/avcodec.h:3796
int dts_sync_point
Synchronization point for start of timestamp generation.
Definition: libavcodec/avcodec.h:3827
Generated on Tue Jul 1 2025 06:52:28 for FFmpeg by
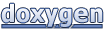