FFmpeg
|
H.264 / AVC / MPEG4 part10 codec. More...
#include "libavutil/imgutils.h"
#include "libavutil/opt.h"
#include "internal.h"
#include "cabac.h"
#include "cabac_functions.h"
#include "dsputil.h"
#include "error_resilience.h"
#include "avcodec.h"
#include "mpegvideo.h"
#include "h264.h"
#include "h264data.h"
#include "h264chroma.h"
#include "h264_mvpred.h"
#include "golomb.h"
#include "mathops.h"
#include "rectangle.h"
#include "svq3.h"
#include "thread.h"
#include "vdpau_internal.h"
#include "libavutil/avassert.h"
#include <assert.h>
#include "h264_mb_template.c"
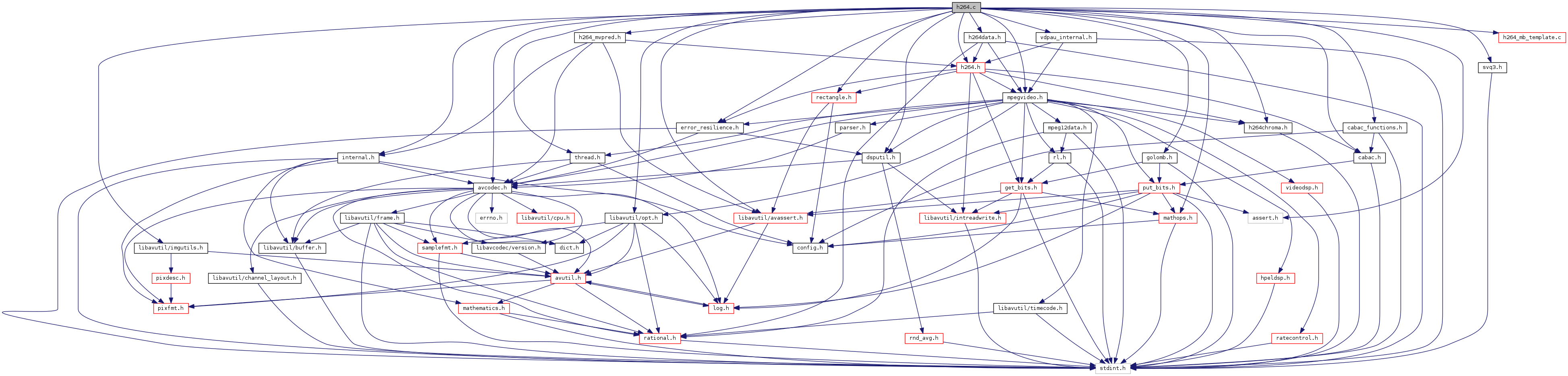
Go to the source code of this file.
Macros | |
#define | UNCHECKED_BITSTREAM_READER 1 |
#define | STARTCODE_TEST |
#define | IN_RANGE(a, b, size) (((a) >= (b)) && ((a) < ((b) + (size)))) |
#define | REBASE_PICTURE(pic, new_ctx, old_ctx) |
#define | copy_fields(to, from, start_field, end_field) |
#define | XCHG(a, b, xchg) |
#define | BITS 8 |
#define | SIMPLE 1 |
#define | BITS 16 |
#define | SIMPLE 0 |
#define | T(x) (x >> 2) | ((x << 2) & 0xF) |
#define | T(x) (x >> 3) | ((x & 7) << 3) |
Functions | |
int | avpriv_h264_has_num_reorder_frames (AVCodecContext *avctx) |
static void | h264_er_decode_mb (void *opaque, int ref, int mv_dir, int mv_type, int(*mv)[2][4][2], int mb_x, int mb_y, int mb_intra, int mb_skipped) |
void | ff_h264_draw_horiz_band (H264Context *h, int y, int height) |
static void | unref_picture (H264Context *h, Picture *pic) |
static void | release_unused_pictures (H264Context *h, int remove_current) |
static int | ref_picture (H264Context *h, Picture *dst, Picture *src) |
static int | alloc_scratch_buffers (H264Context *h, int linesize) |
static int | init_table_pools (H264Context *h) |
static int | alloc_picture (H264Context *h, Picture *pic) |
static int | pic_is_unused (H264Context *h, Picture *pic) |
static int | find_unused_picture (H264Context *h) |
int | ff_h264_check_intra4x4_pred_mode (H264Context *h) |
Check if the top & left blocks are available if needed and change the dc mode so it only uses the available blocks. More... | |
int | ff_h264_check_intra_pred_mode (H264Context *h, int mode, int is_chroma) |
Check if the top & left blocks are available if needed and change the dc mode so it only uses the available blocks. More... | |
const uint8_t * | ff_h264_decode_nal (H264Context *h, const uint8_t *src, int *dst_length, int *consumed, int length) |
Decode a network abstraction layer unit. More... | |
static int | decode_rbsp_trailing (H264Context *h, const uint8_t *src) |
Identify the exact end of the bitstream. More... | |
static int | get_lowest_part_list_y (H264Context *h, Picture *pic, int n, int height, int y_offset, int list) |
static void | get_lowest_part_y (H264Context *h, int refs[2][48], int n, int height, int y_offset, int list0, int list1, int *nrefs) |
static void | await_references (H264Context *h) |
Wait until all reference frames are available for MC operations. More... | |
static av_always_inline void | mc_dir_part (H264Context *h, Picture *pic, int n, int square, int height, int delta, int list, uint8_t *dest_y, uint8_t *dest_cb, uint8_t *dest_cr, int src_x_offset, int src_y_offset, qpel_mc_func *qpix_op, h264_chroma_mc_func chroma_op, int pixel_shift, int chroma_idc) |
static av_always_inline void | mc_part_std (H264Context *h, int n, int square, int height, int delta, uint8_t *dest_y, uint8_t *dest_cb, uint8_t *dest_cr, int x_offset, int y_offset, qpel_mc_func *qpix_put, h264_chroma_mc_func chroma_put, qpel_mc_func *qpix_avg, h264_chroma_mc_func chroma_avg, int list0, int list1, int pixel_shift, int chroma_idc) |
static av_always_inline void | mc_part_weighted (H264Context *h, int n, int square, int height, int delta, uint8_t *dest_y, uint8_t *dest_cb, uint8_t *dest_cr, int x_offset, int y_offset, qpel_mc_func *qpix_put, h264_chroma_mc_func chroma_put, h264_weight_func luma_weight_op, h264_weight_func chroma_weight_op, h264_biweight_func luma_weight_avg, h264_biweight_func chroma_weight_avg, int list0, int list1, int pixel_shift, int chroma_idc) |
static av_always_inline void | prefetch_motion (H264Context *h, int list, int pixel_shift, int chroma_idc) |
static void | free_tables (H264Context *h, int free_rbsp) |
static void | init_dequant8_coeff_table (H264Context *h) |
static void | init_dequant4_coeff_table (H264Context *h) |
static void | init_dequant_tables (H264Context *h) |
int | ff_h264_alloc_tables (H264Context *h) |
Allocate tables. More... | |
static void | clone_tables (H264Context *dst, H264Context *src, int i) |
Mimic alloc_tables(), but for every context thread. More... | |
static int | context_init (H264Context *h) |
Init context Allocate buffers which are not shared amongst multiple threads. More... | |
static int | decode_nal_units (H264Context *h, const uint8_t *buf, int buf_size, int parse_extradata) |
int | ff_h264_decode_extradata (H264Context *h, const uint8_t *buf, int size) |
av_cold int | ff_h264_decode_init (AVCodecContext *avctx) |
static void | copy_picture_range (Picture **to, Picture **from, int count, H264Context *new_base, H264Context *old_base) |
static void | copy_parameter_set (void **to, void **from, int count, int size) |
static int | decode_init_thread_copy (AVCodecContext *avctx) |
static int | h264_slice_header_init (H264Context *, int) |
static int | h264_set_parameter_from_sps (H264Context *h) |
static int | decode_update_thread_context (AVCodecContext *dst, const AVCodecContext *src) |
static int | h264_frame_start (H264Context *h) |
static void | decode_postinit (H264Context *h, int setup_finished) |
Run setup operations that must be run after slice header decoding. More... | |
static av_always_inline void | backup_mb_border (H264Context *h, uint8_t *src_y, uint8_t *src_cb, uint8_t *src_cr, int linesize, int uvlinesize, int simple) |
static av_always_inline void | xchg_mb_border (H264Context *h, uint8_t *src_y, uint8_t *src_cb, uint8_t *src_cr, int linesize, int uvlinesize, int xchg, int chroma444, int simple, int pixel_shift) |
static av_always_inline int | dctcoef_get (int16_t *mb, int high_bit_depth, int index) |
static av_always_inline void | dctcoef_set (int16_t *mb, int high_bit_depth, int index, int value) |
static av_always_inline void | hl_decode_mb_predict_luma (H264Context *h, int mb_type, int is_h264, int simple, int transform_bypass, int pixel_shift, int *block_offset, int linesize, uint8_t *dest_y, int p) |
static av_always_inline void | hl_decode_mb_idct_luma (H264Context *h, int mb_type, int is_h264, int simple, int transform_bypass, int pixel_shift, int *block_offset, int linesize, uint8_t *dest_y, int p) |
void | ff_h264_hl_decode_mb (H264Context *h) |
static int | pred_weight_table (H264Context *h) |
static void | implicit_weight_table (H264Context *h, int field) |
Initialize implicit_weight table. More... | |
static void | idr (H264Context *h) |
instantaneous decoder refresh. More... | |
static void | flush_change (H264Context *h) |
static void | flush_dpb (AVCodecContext *avctx) |
static int | init_poc (H264Context *h) |
static void | init_scan_tables (H264Context *h) |
initialize scan tables More... | |
static int | field_end (H264Context *h, int in_setup) |
static int | clone_slice (H264Context *dst, H264Context *src) |
Replicate H264 "master" context to thread contexts. More... | |
int | ff_h264_get_profile (SPS *sps) |
Compute profile from profile_idc and constraint_set?_flags. More... | |
static enum AVPixelFormat | get_pixel_format (H264Context *h, int force_callback) |
static int | init_dimensions (H264Context *h) |
static int | decode_slice_header (H264Context *h, H264Context *h0) |
Decode a slice header. More... | |
int | ff_h264_get_slice_type (const H264Context *h) |
Reconstruct bitstream slice_type. More... | |
static av_always_inline void | fill_filter_caches_inter (H264Context *h, int mb_type, int top_xy, int left_xy[LEFT_MBS], int top_type, int left_type[LEFT_MBS], int mb_xy, int list) |
static int | fill_filter_caches (H264Context *h, int mb_type) |
static void | loop_filter (H264Context *h, int start_x, int end_x) |
static void | predict_field_decoding_flag (H264Context *h) |
static void | decode_finish_row (H264Context *h) |
Draw edges and report progress for the last MB row. More... | |
static void | er_add_slice (H264Context *h, int startx, int starty, int endx, int endy, int status) |
static int | decode_slice (struct AVCodecContext *avctx, void *arg) |
static int | execute_decode_slices (H264Context *h, int context_count) |
Call decode_slice() for each context. More... | |
static int | get_consumed_bytes (int pos, int buf_size) |
Return the number of bytes consumed for building the current frame. More... | |
static int | output_frame (H264Context *h, AVFrame *dst, AVFrame *src) |
static int | decode_frame (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt) |
av_cold void | ff_h264_free_context (H264Context *h) |
Free any data that may have been allocated in the H264 context like SPS, PPS etc. More... | |
static av_cold int | h264_decode_end (AVCodecContext *avctx) |
Variables | |
const uint16_t | ff_h264_mb_sizes [4] = { 256, 384, 512, 768 } |
static const uint8_t | rem6 [QP_MAX_NUM+1] |
static const uint8_t | div6 [QP_MAX_NUM+1] |
static enum AVPixelFormat | h264_hwaccel_pixfmt_list_420 [] |
static enum AVPixelFormat | h264_hwaccel_pixfmt_list_jpeg_420 [] |
static const AVProfile | profiles [] |
static const AVOption | h264_options [] |
static const AVClass | h264_class |
static const AVClass | h264_vdpau_class |
AVCodec | ff_h264_decoder |
Detailed Description
H.264 / AVC / MPEG4 part10 codec.
Definition in file h264.c.
Macro Definition Documentation
Definition at line 1588 of file h264.c.
Referenced by decode_update_thread_context().
Definition at line 1537 of file h264.c.
Referenced by copy_picture_range().
#define REBASE_PICTURE | ( | pic, | |
new_ctx, | |||
old_ctx | |||
) |
Definition at line 1539 of file h264.c.
Referenced by copy_picture_range(), decode_update_thread_context(), and ff_mpeg_update_thread_context().
#define SIMPLE 1 |
Definition at line 2466 of file h264.c.
Referenced by hl_decode_mb(), and hl_decode_mb_444().
#define STARTCODE_TEST |
Referenced by ff_h264_decode_nal().
Referenced by init_scan_tables().
Referenced by xchg_mb_border().
Function Documentation
|
static |
Definition at line 338 of file h264.c.
Referenced by h264_frame_start().
|
static |
Definition at line 286 of file h264.c.
Referenced by decode_slice_header().
int avpriv_h264_has_num_reorder_frames | ( | AVCodecContext * | avctx | ) |
Definition at line 106 of file h264.c.
Referenced by ff_samples_to_time_base(), and has_decode_delay_been_guessed().
|
static |
Wait until all reference frames are available for MC operations.
- Parameters
-
h the H264 context
Definition at line 701 of file h264.c.
Referenced by hl_motion().
|
static |
Definition at line 2070 of file h264.c.
Referenced by loop_filter().
|
static |
Replicate H264 "master" context to thread contexts.
Definition at line 2865 of file h264.c.
Referenced by decode_slice_header().
|
static |
Mimic alloc_tables(), but for every context thread.
Definition at line 1299 of file h264.c.
Referenced by h264_slice_header_init().
|
static |
Init context Allocate buffers which are not shared amongst multiple threads.
Definition at line 1326 of file h264.c.
Referenced by decode_update_thread_context(), and h264_slice_header_init().
Definition at line 1559 of file h264.c.
Referenced by decode_update_thread_context().
|
static |
Definition at line 1544 of file h264.c.
Referenced by decode_update_thread_context().
|
static |
Definition at line 2254 of file h264.c.
Referenced by hl_decode_mb(), hl_decode_mb_idct_luma(), and hl_decode_mb_predict_luma().
|
static |
Definition at line 2263 of file h264.c.
Referenced by hl_decode_mb_predict_luma().
|
static |
Draw edges and report progress for the last MB row.
Definition at line 4223 of file h264.c.
Referenced by decode_slice().
|
static |
|
static |
|
static |
< thread context
< number of NALs that need decoding before the next frame thread starts
Definition at line 4485 of file h264.c.
Referenced by context_init(), decode_frame(), and ff_h264_decode_extradata().
|
static |
Run setup operations that must be run after slice header decoding.
This includes finding the next displayed frame.
- Parameters
-
h h264 master context setup_finished enough NALs have been read that we can call ff_thread_finish_setup()
Definition at line 1891 of file h264.c.
Referenced by decode_frame(), and decode_nal_units().
|
static |
Identify the exact end of the bitstream.
- Returns
- the length of the trailing, or 0 if damaged
Definition at line 630 of file h264.c.
Referenced by decode_nal_units().
|
static |
Definition at line 4265 of file h264.c.
Referenced by execute_decode_slices().
|
static |
Decode a slice header.
This will also call ff_MPV_common_init() and frame_start() as needed.
- Parameters
-
h h264context h0 h264 master context (differs from 'h' when doing sliced based parallel decoding)
- Returns
- 0 if okay, <0 if an error occurred, 1 if decoding must not be multithreaded
Definition at line 3201 of file h264.c.
Referenced by decode_nal_units().
|
static |
|
static |
Definition at line 4254 of file h264.c.
Referenced by decode_slice().
|
static |
Call decode_slice() for each context.
- Parameters
-
h h264 master context context_count number of contexts to execute
Definition at line 4446 of file h264.c.
Referenced by decode_nal_units().
int ff_h264_alloc_tables | ( | H264Context * | h | ) |
Allocate tables.
needs width/height
Definition at line 1235 of file h264.c.
Referenced by decode_update_thread_context(), h264_slice_header_init(), and svq3_decode_init().
int ff_h264_check_intra4x4_pred_mode | ( | H264Context * | h | ) |
Check if the top & left blocks are available if needed and change the dc mode so it only uses the available blocks.
Check if the top & left blocks are available if needed & change the dc mode so it only uses the available blocks.
Definition at line 426 of file h264.c.
Referenced by ff_h264_decode_mb_cabac(), ff_h264_decode_mb_cavlc(), and svq3_decode_mb().
int ff_h264_check_intra_pred_mode | ( | H264Context * | h, |
int | mode, | ||
int | is_chroma | ||
) |
Check if the top & left blocks are available if needed and change the dc mode so it only uses the available blocks.
Check if the top & left blocks are available if needed & change the dc mode so it only uses the available blocks.
Definition at line 473 of file h264.c.
Referenced by ff_h264_decode_mb_cabac(), ff_h264_decode_mb_cavlc(), and svq3_decode_mb().
int ff_h264_decode_extradata | ( | H264Context * | h, |
const uint8_t * | buf, | ||
int | size | ||
) |
Definition at line 1398 of file h264.c.
Referenced by decode_frame(), ff_h264_decode_init(), and h264_parse().
av_cold int ff_h264_decode_init | ( | AVCodecContext * | avctx | ) |
Definition at line 1455 of file h264.c.
Referenced by svq3_decode_init().
const uint8_t* ff_h264_decode_nal | ( | H264Context * | h, |
const uint8_t * | src, | ||
int * | dst_length, | ||
int * | consumed, | ||
int | length | ||
) |
Decode a network abstraction layer unit.
- Parameters
-
consumed is the number of bytes used as input length is the length of the array dst_length is the number of decoded bytes FIXME here or a decode rbsp tailing?
- Returns
- decoded bytes, might be src+1 if no escapes
Definition at line 514 of file h264.c.
Referenced by decode_nal_units(), and parse_nal_units().
void ff_h264_draw_horiz_band | ( | H264Context * | h, |
int | y, | ||
int | height | ||
) |
Definition at line 147 of file h264.c.
Referenced by decode_finish_row(), dxva2_h264_end_frame(), ff_vdpau_add_data_chunk(), get_dct8x8_allowed(), vaapi_h264_end_frame(), and vdpau_h264_end_frame().
av_cold void ff_h264_free_context | ( | H264Context * | h | ) |
Free any data that may have been allocated in the H264 context like SPS, PPS etc.
Definition at line 4978 of file h264.c.
Referenced by close(), ff_h264_decode_init(), h264_decode_end(), and svq3_decode_end().
int ff_h264_get_profile | ( | SPS * | sps | ) |
Compute profile from profile_idc and constraint_set?_flags.
compute profile from sps
- Parameters
-
sps SPS
- Returns
- profile as defined by FF_PROFILE_H264_*
Definition at line 2897 of file h264.c.
Referenced by decode_slice_header(), and parse_nal_units().
int ff_h264_get_slice_type | ( | const H264Context * | h | ) |
Reconstruct bitstream slice_type.
Definition at line 3894 of file h264.c.
Referenced by fill_slice_long(), and vaapi_h264_decode_slice().
void ff_h264_hl_decode_mb | ( | H264Context * | h | ) |
Definition at line 2469 of file h264.c.
Referenced by decode_slice(), h264_er_decode_mb(), and svq3_decode_frame().
|
static |
Definition at line 2804 of file h264.c.
Referenced by decode_frame(), and decode_slice_header().
|
static |
- Returns
- non zero if the loop filter can be skipped
Definition at line 3996 of file h264.c.
Referenced by loop_filter().
|
static |
Definition at line 3912 of file h264.c.
Referenced by fill_filter_caches().
|
static |
Definition at line 403 of file h264.c.
Referenced by h264_frame_start().
|
static |
Definition at line 2632 of file h264.c.
Referenced by decode_slice_header(), and flush_dpb().
|
static |
|
static |
Definition at line 1100 of file h264.c.
Referenced by ff_h264_alloc_tables(), ff_h264_free_context(), and h264_slice_header_init().
|
static |
Return the number of bytes consumed for building the current frame.
Definition at line 4834 of file h264.c.
Referenced by decode_frame().
|
inlinestatic |
Definition at line 645 of file h264.c.
Referenced by get_lowest_part_y().
|
inlinestatic |
Definition at line 658 of file h264.c.
Referenced by await_references().
|
static |
Definition at line 2972 of file h264.c.
Referenced by decode_slice_header().
|
static |
|
static |
Definition at line 112 of file h264.c.
Referenced by context_init().
|
static |
Definition at line 1795 of file h264.c.
Referenced by decode_slice_header().
|
static |
Definition at line 2917 of file h264.c.
Referenced by decode_slice_header(), and decode_update_thread_context().
|
static |
Definition at line 3089 of file h264.c.
Referenced by decode_slice_header(), and decode_update_thread_context().
|
static |
Definition at line 2387 of file h264.c.
Referenced by hl_decode_mb(), and hl_decode_mb_444().
|
static |
Definition at line 2272 of file h264.c.
Referenced by hl_decode_mb(), and hl_decode_mb_444().
|
static |
instantaneous decoder refresh.
Definition at line 2619 of file h264.c.
Referenced by decode_nal_units(), flush_change(), and h264_probe().
|
static |
Initialize implicit_weight table.
- Parameters
-
field 0/1 initialize the weight for interlaced MBAFF -1 initializes the rest
Definition at line 2555 of file h264.c.
Referenced by decode_slice_header().
|
static |
Definition at line 1192 of file h264.c.
Referenced by init_dequant_tables().
|
static |
Definition at line 1165 of file h264.c.
Referenced by init_dequant_tables().
|
static |
Definition at line 1218 of file h264.c.
Referenced by decode_slice_header(), and ff_h264_alloc_tables().
|
static |
Definition at line 3054 of file h264.c.
Referenced by decode_slice_header().
|
static |
Definition at line 2690 of file h264.c.
Referenced by decode_slice_header().
|
static |
initialize scan tables
Definition at line 2770 of file h264.c.
Referenced by h264_slice_header_init().
|
static |
Definition at line 311 of file h264.c.
Referenced by alloc_picture().
|
static |
Definition at line 4141 of file h264.c.
Referenced by decode_slice(), and xmv_read_extradata().
|
static |
Definition at line 809 of file h264.c.
Referenced by mc_part_std(), and mc_part_weighted().
|
static |
|
static |
|
static |
Definition at line 4844 of file h264.c.
Referenced by decode_frame().
|
inlinestatic |
Definition at line 394 of file h264.c.
Referenced by find_unused_picture().
|
static |
Definition at line 2488 of file h264.c.
Referenced by decode_slice_header().
|
static |
Definition at line 4210 of file h264.c.
Referenced by decode_slice().
|
static |
Definition at line 1076 of file h264.c.
Referenced by hl_motion().
|
static |
Definition at line 225 of file h264.c.
Referenced by decode_update_thread_context(), h264_frame_start(), MPV_motion_internal(), and MPV_motion_lowres().
|
static |
Definition at line 212 of file h264.c.
Referenced by decode_slice_header(), and h264_frame_start().
|
static |
Definition at line 191 of file h264.c.
Referenced by alloc_picture(), decode_update_thread_context(), find_unused_picture(), flush_dpb(), free_tables(), h264_decode_end(), h264_frame_start(), ref_picture(), and release_unused_pictures().
|
static |
Definition at line 2166 of file h264.c.
Referenced by hl_decode_mb(), and hl_decode_mb_444().
Variable Documentation
|
static |
Definition at line 64 of file h264.c.
Referenced by init_dequant4_coeff_table(), and init_dequant8_coeff_table().
AVCodec ff_h264_decoder |
const uint16_t ff_h264_mb_sizes[4] = { 256, 384, 512, 768 } |
Definition at line 54 of file h264.c.
Referenced by ff_h264_decode_mb_cabac(), ff_h264_decode_mb_cavlc(), and hl_decode_mb().
|
static |
Definition at line 5026 of file h264.c.
Referenced by decode().
|
static |
Definition at line 72 of file h264.c.
Referenced by get_pixel_format().
|
static |
Definition at line 89 of file h264.c.
Referenced by get_pixel_format().
|
static |
|
static |
|
static |
|
static |
Definition at line 56 of file h264.c.
Referenced by init_dequant4_coeff_table(), and init_dequant8_coeff_table().
Generated on Tue Jan 21 2025 06:52:33 for FFmpeg by
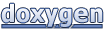