FFmpeg
|
vdpau_h264.c
Go to the documentation of this file.
static void vdpau_h264_set_rf(VdpReferenceFrameH264 *rf, Picture *pic, int pic_structure)
Definition: vdpau_h264.c:49
Public libavcodec VDPAU header.
static void vdpau_h264_clear_rf(VdpReferenceFrameH264 *rf)
Definition: vdpau_h264.c:38
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
Definition: libavcodec/avcodec.h:130
int deblocking_filter_parameters_present
deblocking_filter_parameters_present_flag
Definition: h264.h:225
static int vdpau_h264_start_frame(AVCodecContext *avctx, const uint8_t *buffer, uint32_t size)
Definition: vdpau_h264.c:117
static uintptr_t ff_vdpau_get_surface_id(Picture *pic)
Extract VdpVideoSurface from a Picture.
Definition: vdpau_internal.h:32
H.264 / AVC / MPEG4 part10 codec.
This structure is used to share data between the libavcodec library and the client video application...
Definition: vdpau.h:72
external API header
static int vdpau_h264_end_frame(AVCodecContext *avctx)
Definition: vdpau_h264.c:187
static void vdpau_h264_set_reference_frames(AVCodecContext *avctx)
Definition: vdpau_h264.c:66
int ff_vdpau_add_buffer(AVCodecContext *avctx, const uint8_t *buf, uint32_t size)
Definition: vdpau.c:70
#define H264_RF_COUNT
int bitstream_buffers_used
Useful bitstream buffers in the bitstream buffers table.
Definition: vdpau.h:106
HW acceleration through VDPAU, Picture.data[3] contains a VdpVideoSurface.
Definition: pixfmt.h:203
void ff_h264_draw_horiz_band(H264Context *h, int y, int height)
Definition: h264.c:147
int ff_vdpau_common_start_frame(AVCodecContext *avctx, av_unused const uint8_t *buffer, av_unused uint32_t size)
Definition: vdpau.c:41
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
static int vdpau_h264_decode_slice(AVCodecContext *avctx, const uint8_t *buffer, uint32_t size)
Definition: vdpau_h264.c:169
int pic_id
h264 pic_num (short -> no wrap version of pic_num, pic_num & max_pic_num; long -> long_pic_num) ...
Definition: mpegvideo.h:163
Definition: avutil.h:143
Generated on Fri Dec 20 2024 06:56:08 for FFmpeg by
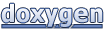