FFmpeg
|
vdpau.c
Go to the documentation of this file.
171 render->bitstream_buffers[render->bitstream_buffers_used].struct_version = VDP_BITSTREAM_BUFFER_VERSION;
211 render->info.h264.mb_adaptive_frame_field_flag = h->sps.mb_aff && !render->info.h264.field_pic_flag;
224 render->info.h264.log2_max_pic_order_cnt_lsb_minus4 = h->sps.poc_type ? 0 : h->sps.log2_max_poc_lsb - 4;
229 render->info.h264.deblocking_filter_control_present_flag = h->pps.deblocking_filter_parameters_present;
231 memcpy(render->info.h264.scaling_lists_4x4, h->pps.scaling_matrix4, sizeof(render->info.h264.scaling_lists_4x4));
232 memcpy(render->info.h264.scaling_lists_8x8[0], h->pps.scaling_matrix8[0], sizeof(render->info.h264.scaling_lists_8x8[0]));
233 memcpy(render->info.h264.scaling_lists_8x8[1], h->pps.scaling_matrix8[3], sizeof(render->info.h264.scaling_lists_8x8[0]));
Public libavcodec VDPAU header.
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
void ff_vdpau_h264_picture_complete(H264Context *h)
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
initialize output if(nPeaks >3)%at least 3 peaks in spectrum for trying to find f0 nf0peaks
void ff_mpeg_draw_horiz_band(MpegEncContext *s, int y, int h)
Definition: mpegvideo.c:2996
int deblocking_filter_parameters_present
deblocking_filter_parameters_present_flag
Definition: h264.h:225
int no_rounding
apply no rounding to motion compensation (MPEG4, msmpeg4, ...) for b-frames rounding mode is always 0...
Definition: mpegvideo.h:439
int refdist_flag
REFDIST syntax element present in II, IP, PI or PP field picture headers.
Definition: vc1.h:213
static uintptr_t ff_vdpau_get_surface_id(Picture *pic)
Extract VdpVideoSurface from a Picture.
Definition: vdpau_internal.h:32
H.264 / AVC / MPEG4 part10 codec.
VdpBitstreamBuffer * bitstream_buffers
The user is responsible for freeing this buffer using av_freep().
Definition: vdpau.h:149
This structure is used to share data between the libavcodec library and the client video application...
Definition: vdpau.h:72
void * av_fast_realloc(void *ptr, unsigned int *size, size_t min_size)
Reallocate the given block if it is not large enough, otherwise do nothing.
Definition: libavcodec/utils.c:63
external API header
int bitstream_buffers_allocated
Allocated size of the bitstream_buffers table.
Definition: vdpau.h:99
Definition: libavcodec/avcodec.h:107
int rangered
RANGEREDFRM (range reduction) syntax element present at frame level.
Definition: vc1.h:198
void ff_vdpau_add_data_chunk(uint8_t *data, const uint8_t *buf, int buf_size)
Definition: vdpau.c:160
int ff_vdpau_mpeg_end_frame(AVCodecContext *avctx)
int ff_vdpau_add_buffer(AVCodecContext *avctx, const uint8_t *buf, uint32_t size)
Definition: vdpau.c:70
int bitstream_buffers_allocated
Describe size/location of the compressed video data.
Definition: vdpau.h:146
void ff_vdpau_h264_set_reference_frames(H264Context *h)
Definition: vdpau.c:92
int bitstream_buffers_used
Useful bitstream buffers in the bitstream buffers table.
Definition: vdpau.h:106
void ff_vdpau_h264_picture_start(H264Context *h)
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
void ff_h264_draw_horiz_band(H264Context *h, int y, int height)
Definition: h264.c:147
int ff_vdpau_common_start_frame(AVCodecContext *avctx, av_unused const uint8_t *buffer, av_unused uint32_t size)
Definition: vdpau.c:41
void ff_vdpau_mpeg_picture_complete(MpegEncContext *s, const uint8_t *buf, int buf_size, int slice_count)
This structure is used as a callback between the FFmpeg decoder (vd_) and presentation (vo_) module...
Definition: vdpau.h:134
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
int pic_id
h264 pic_num (short -> no wrap version of pic_num, pic_num & max_pic_num; long -> long_pic_num) ...
Definition: mpegvideo.h:163
void ff_vdpau_mpeg4_decode_picture(MpegEncContext *s, const uint8_t *buf, int buf_size)
union FFVdpPictureInfo info
picture parameter information for all supported codecs
Definition: vdpau.h:153
#define H264_RF_COUNT
void ff_vdpau_vc1_decode_picture(MpegEncContext *s, const uint8_t *buf, int buf_size)
Generated on Wed May 7 2025 06:52:48 for FFmpeg by
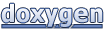