FFmpeg
|
vdpau_internal.h
Go to the documentation of this file.
mpegvideo header.
void ff_vdpau_h264_picture_complete(H264Context *h)
static uintptr_t ff_vdpau_get_surface_id(Picture *pic)
Extract VdpVideoSurface from a Picture.
Definition: vdpau_internal.h:32
H.264 / AVC / MPEG4 part10 codec.
void ff_vdpau_add_data_chunk(uint8_t *data, const uint8_t *buf, int buf_size)
Definition: vdpau.c:160
int ff_vdpau_mpeg_end_frame(AVCodecContext *avctx)
int ff_vdpau_add_buffer(AVCodecContext *avctx, const uint8_t *buf, uint32_t buf_size)
Definition: vdpau.c:70
void ff_vdpau_h264_set_reference_frames(H264Context *h)
Definition: vdpau.c:92
void ff_vdpau_h264_picture_start(H264Context *h)
void ff_vdpau_mpeg_picture_complete(MpegEncContext *s, const uint8_t *buf, int buf_size, int slice_count)
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
void ff_vdpau_mpeg4_decode_picture(MpegEncContext *s, const uint8_t *buf, int buf_size)
int ff_vdpau_common_start_frame(AVCodecContext *avctx, const uint8_t *buffer, uint32_t size)
void ff_vdpau_vc1_decode_picture(MpegEncContext *s, const uint8_t *buf, int buf_size)
Generated on Wed May 7 2025 06:52:48 for FFmpeg by
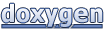