FFmpeg
|
vaapi_h264.c
Go to the documentation of this file.
66 va_pic->flags |= (pic_structure & PICT_TOP_FIELD) ? VA_PICTURE_H264_TOP_FIELD : VA_PICTURE_H264_BOTTOM_FIELD;
68 va_pic->flags |= pic->long_ref ? VA_PICTURE_H264_LONG_TERM_REFERENCE : VA_PICTURE_H264_SHORT_TERM_REFERENCE;
82 int max_size; ///< Max number of reference frames. This is FF_ARRAY_ELEMS(VAPictureParameterBufferH264.ReferenceFrames)
105 if ((temp_va_pic.flags ^ va_pic->flags) & (VA_PICTURE_H264_TOP_FIELD | VA_PICTURE_H264_BOTTOM_FIELD)) {
106 va_pic->flags |= temp_va_pic.flags & (VA_PICTURE_H264_TOP_FIELD | VA_PICTURE_H264_BOTTOM_FIELD);
249 pic_param->seq_fields.bits.residual_colour_transform_flag = h->sps.residual_color_transform_flag; /* XXX: only for 4:4:4 high profile? */
250 pic_param->seq_fields.bits.gaps_in_frame_num_value_allowed_flag = h->sps.gaps_in_frame_num_allowed_flag;
258 pic_param->seq_fields.bits.delta_pic_order_always_zero_flag = h->sps.delta_pic_order_always_zero_flag;
274 pic_param->pic_fields.bits.deblocking_filter_control_present_flag = h->pps.deblocking_filter_parameters_present;
284 memcpy(iq_matrix->ScalingList8x8[0], h->pps.scaling_matrix8[0], sizeof(iq_matrix->ScalingList8x8[0]));
285 memcpy(iq_matrix->ScalingList8x8[1], h->pps.scaling_matrix8[3], sizeof(iq_matrix->ScalingList8x8[0]));
324 slice_param = (VASliceParameterBufferH264 *)ff_vaapi_alloc_slice(avctx->hwaccel_context, buffer, size);
327 slice_param->slice_data_bit_offset = get_bits_count(&h->gb) + 8; /* bit buffer started beyond nal_unit_type */
328 slice_param->first_mb_in_slice = (h->mb_y >> FIELD_OR_MBAFF_PICTURE(h)) * h->mb_width + h->mb_x;
330 slice_param->direct_spatial_mv_pred_flag = h->slice_type == AV_PICTURE_TYPE_B ? h->direct_spatial_mv_pred : 0;
335 slice_param->disable_deblocking_filter_idc = h->deblocking_filter < 2 ? !h->deblocking_filter : h->deblocking_filter;
341 fill_vaapi_RefPicList(slice_param->RefPicList0, h->ref_list[0], h->list_count > 0 ? h->ref_count[0] : 0);
342 fill_vaapi_RefPicList(slice_param->RefPicList1, h->ref_list[1], h->list_count > 1 ? h->ref_count[1] : 0);
346 &slice_param->chroma_weight_l0_flag, slice_param->chroma_weight_l0, slice_param->chroma_offset_l0);
349 &slice_param->chroma_weight_l1_flag, slice_param->chroma_weight_l1, slice_param->chroma_offset_l1);
static int dpb_add(DPB *dpb, Picture *pic)
Append picture to the decoded picture buffer, in a VA API form that merges the second field picture a...
Definition: vaapi_h264.c:92
HW decoding through VA API, Picture.data[3] contains a vaapi_render_state struct which contains the b...
Definition: pixfmt.h:126
int ff_vaapi_render_picture(struct vaapi_context *vactx, VASurfaceID surface)
Definition: vaapi.c:44
int max_size
Max number of reference frames. This is FF_ARRAY_ELEMS(VAPictureParameterBufferH264.ReferenceFrames)
Definition: vaapi_h264.c:82
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
This structure is used to share data between the FFmpeg library and the client video application...
Definition: vaapi.h:50
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
Definition: libavcodec/avcodec.h:130
static int vaapi_h264_decode_slice(AVCodecContext *avctx, const uint8_t *buffer, uint32_t size)
Decode the given H.264 slice with VA API.
Definition: vaapi_h264.c:313
int deblocking_filter_parameters_present
deblocking_filter_parameters_present_flag
Definition: h264.h:225
int ff_h264_get_slice_type(const H264Context *h)
Reconstruct bitstream slice_type.
Definition: h264.c:3894
H.264 / AVC / MPEG4 part10 codec.
void ff_vaapi_common_end_frame(AVCodecContext *avctx)
Common AVHWAccel.end_frame() implementation.
Definition: vaapi.c:179
void * ff_vaapi_alloc_pic_param(struct vaapi_context *vactx, unsigned int size)
Allocate a new picture parameter buffer.
Definition: vaapi.c:133
static int vaapi_h264_end_frame(AVCodecContext *avctx)
End a hardware decoding based frame.
Definition: vaapi_h264.c:290
VASliceParameterBufferBase * ff_vaapi_alloc_slice(struct vaapi_context *vactx, const uint8_t *buffer, uint32_t size)
Allocate a new slice descriptor for the input slice.
Definition: vaapi.c:148
void ff_h264_draw_horiz_band(H264Context *h, int y, int height)
Definition: h264.c:147
static int fill_vaapi_ReferenceFrames(VAPictureParameterBufferH264 *pic_param, H264Context *h)
Fill in VA API reference frames array.
Definition: vaapi_h264.c:123
static void fill_vaapi_plain_pred_weight_table(H264Context *h, int list, unsigned char *luma_weight_flag, short luma_weight[32], short luma_offset[32], unsigned char *chroma_weight_flag, short chroma_weight[32][2], short chroma_offset[32][2])
Fill in prediction weight table.
Definition: vaapi_h264.c:185
void * ff_vaapi_alloc_iq_matrix(struct vaapi_context *vactx, unsigned int size)
Allocate a new IQ matrix buffer.
Definition: vaapi.c:138
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
static VASurfaceID ff_vaapi_get_surface_id(Picture *pic)
Extract VASurfaceID from a Picture.
Definition: vaapi_internal.h:39
static int vaapi_h264_start_frame(AVCodecContext *avctx, av_unused const uint8_t *buffer, av_unused uint32_t size)
Initialize and start decoding a frame with VA API.
Definition: vaapi_h264.c:222
static void init_vaapi_pic(VAPictureH264 *va_pic)
Initialize an empty VA API picture.
Definition: vaapi_h264.c:37
int pic_id
h264 pic_num (short -> no wrap version of pic_num, pic_num & max_pic_num; long -> long_pic_num) ...
Definition: mpegvideo.h:163
static void fill_vaapi_RefPicList(VAPictureH264 RefPicList[32], Picture *ref_list, unsigned int ref_count)
Fill in VA API reference picture lists from the FFmpeg reference picture list.
Definition: vaapi_h264.c:157
VAPictureH264 * va_pics
Pointer to VAPictureParameterBufferH264.ReferenceFrames array.
Definition: vaapi_h264.c:83
Definition: avutil.h:143
static void fill_vaapi_pic(VAPictureH264 *va_pic, Picture *pic, int pic_structure)
Translate an FFmpeg Picture into its VA API form.
Definition: vaapi_h264.c:53
Generated on Tue Mar 25 2025 06:54:30 for FFmpeg by
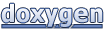