FFmpeg
|
vaapi.c
Go to the documentation of this file.
121 static void *alloc_buffer(struct vaapi_context *vactx, int type, unsigned int size, uint32_t *buf_id)
148 VASliceParameterBufferBase *ff_vaapi_alloc_slice(struct vaapi_context *vactx, const uint8_t *buffer, uint32_t size)
169 slice_param = (VASliceParameterBufferBase *)(slice_params + vactx->slice_count * vactx->slice_param_size);
int ff_vaapi_render_picture(struct vaapi_context *vactx, VASurfaceID surface)
Definition: vaapi.c:44
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
This structure is used to share data between the FFmpeg library and the client video application...
Definition: vaapi.h:50
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
void ff_mpeg_draw_horiz_band(MpegEncContext *s, int y, int h)
Definition: mpegvideo.c:2996
static void * alloc_buffer(struct vaapi_context *vactx, int type, unsigned int size, uint32_t *buf_id)
Definition: vaapi.c:121
H.264 / AVC / MPEG4 part10 codec.
void * av_fast_realloc(void *ptr, unsigned int *size, size_t min_size)
Reallocate the given block if it is not large enough, otherwise do nothing.
Definition: libavcodec/utils.c:63
void ff_vaapi_common_end_frame(AVCodecContext *avctx)
Common AVHWAccel.end_frame() implementation.
Definition: vaapi.c:179
void * ff_vaapi_alloc_pic_param(struct vaapi_context *vactx, unsigned int size)
Allocate a new picture parameter buffer.
Definition: vaapi.c:133
static void destroy_buffers(VADisplay display, VABufferID *buffers, unsigned int n_buffers)
Definition: vaapi.c:33
VASliceParameterBufferBase * ff_vaapi_alloc_slice(struct vaapi_context *vactx, const uint8_t *buffer, uint32_t size)
Allocate a new slice descriptor for the input slice.
Definition: vaapi.c:148
#define type
void * ff_vaapi_alloc_iq_matrix(struct vaapi_context *vactx, unsigned int size)
Allocate a new IQ matrix buffer.
Definition: vaapi.c:138
the buffer and buffer reference mechanism is intended to as much as expensive copies of that data while still allowing the filters to produce correct results The data is stored in buffers represented by AVFilterBuffer structures They must not be accessed but through references stored in AVFilterBufferRef structures Several references can point to the same buffer
Definition: filter_design.txt:45
static VASurfaceID ff_vaapi_get_surface_id(Picture *pic)
Extract VASurfaceID from a Picture.
Definition: vaapi_internal.h:39
unsigned int n_slice_buf_ids
Number of effective slice buffer IDs to send to the HW.
Definition: vaapi.h:113
uint8_t * ff_vaapi_alloc_bitplane(struct vaapi_context *vactx, uint32_t size)
Allocate a new bit-plane buffer.
Definition: vaapi.c:143
Generated on Thu Jul 3 2025 06:53:20 for FFmpeg by
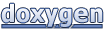