FFmpeg
|
start.py
Go to the documentation of this file.
59 op = optparse.OptionParser()
61 options, args = op.parse_args()
85 html_head = '''<!DOCTYPE html> <html lang="en"> <head><meta charset="utf-8"><title>BrutalizeMe</title> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta name="description" content=""><meta name="author" content=""><!-- Le styles --> <link href="css/bootstrap.css" rel="stylesheet"><style type="text/css">body { padding-top: 20px; padding-bottom: 40px;} /* Custom container */ .container-narrow { margin: 0 auto; max-width: 700px;} .container-narrow > hr { margin: 30px 0;} /* Main marketing message and sign up button */.jumbotron { margin: 60px 0; text-align: center;} .jumbotron h1 { font-size: 72px; line-height: 1; } .jumbotron .btn { font-size: 21px; padding: 14px 24px; } /* Supporting marketing content */ .marketing { margin: 60px 0;} .marketing p + h4 { margin-top: 28px;} .input { border: 1px solid #006;background: #ffc;} </style> <link href="css/bootstrap-responsive.css" rel="stylesheet"> <!-- Fav and touch icons --> <link rel="shortcut icon" href="../assets/ico/favicon.ico"> <link rel="apple-touch-icon-precomposed" sizes="144x144" href="../assets/ico/apple-touch-icon-144-precomposed.png"> <link rel="apple-touch-icon-precomposed" sizes="114x114" href="../assets/ico/apple-touch-icon-114-precomposed.png"> <link rel="apple-touch-icon-precomposed" sizes="72x72" href="../assets/ico/apple-touch-icon-72-precomposed.png"><link rel="apple-touch-icon-precomposed" href="../assets/ico/apple-touch-icon-57-precomposed.png"> <link rel="stylesheet" href="http://code.jquery.com/ui/1.9.1/themes/base/jquery-ui.css"/> <link rel="stylesheet" href="css/styles.css"/> <script src="js/jquery.min.js"></script> <script src="http://code.jquery.com/ui/1.9.1/jquery-ui.js"></script><script type="text/javascript" src="js/main.js"></script> <script type="text/javascript" src="js/infoSongs.js"></script><script type="text/javascript" src="js/genre.js"></script></head><body><div id="dz-root"></div><div class="container-narrow"><hr><div class="jumbotron"> <h1>Results</h1> '''
87 content = '''<div style="text-align:center"> <p> You have chosen <b> %s </b> music </p></div>''' %userinput
88 html_tail = '''</div><div style="margin:0 auto;width:75%;text-align:center"><script type="text/javascript" src="http://mediaplayer.yahoo.com/js"></script><br /> <a href="javascript: history.go(-1)">Back</a> </div></body> </html>'''
96 content = content + '''<div style="text-align:center"><p><a href=" %s ">''' % audio_url + '''<b> %s </b>''' % userinput
110 os.system('./sonic-annotator-0.7-osx-x86_64/sonic-annotator -d vamp:mtg-melodia:melodia:melody Baby.wav -w csv --csv-one-file baby.txt --csv-separator " " ')
115 os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"step1('Baby.wav');exit;\" ")
116 #os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"step1('Baby.wav');exit;\" ")
119 os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"step2('Baby.wav');exit;\" ")
120 #os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"step2('Baby_ps12.wav');exit;\" ")
122 #os.system('/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r "mcc -m filename.m;exit;"')
133 os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"step3('melody_ps5.wav');exit;\"")
134 os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"step3_heavy('melody_ps12.wav');exit;\"")
137 os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"fix_offset('melody.wav','ysbaby_voice_light.wav');exit;\"")
138 os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"fix_offset('melody.wav','ysbaby_voice_heavy.wav');exit;\"")
140 os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"final_mix('ysbaby_voice_light.wav','instrumental.wav');exit;\"")
141 os.system("/Applications/MATLAB_R2012b.app/bin/matlab -nojvm -nosplash -nodisplay -r \"final_mix_heavy('ysbaby_voice_heavy.wav','instrumental.wav');exit;\"")
147 content = content + '''<div style="text-align:center"><p><a href="MIX.wav">''' + '''<b> Light Version </b></div>'''
148 content = content + '''<div style="text-align:center"><p><a href="MIX_heavy.wav">''' + '''<b> Heavy Version </b>'''
249 <response status="ok" version="1.2" xsi:noNamespaceSchemaLocation="http://api.7digital.com/1.2/static/7digitalAPI.xsd">
282 print "getProcessPid:: Unable to obtain process pid. (lsof returned nothing. exitcode: %s)" %str(exitcode)
def get_trackid_from_text_search(title, artistname='')
Definition: start.py:216
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
Definition: start.py:69
Generated on Fri Dec 20 2024 06:56:07 for FFmpeg by
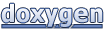