FFmpeg
|
cmdutils.c
Go to the documentation of this file.
int parse_optgroup(void *optctx, OptionGroup *g)
Parse an options group and write results into optctx.
Definition: cmdutils.c:376
Number of sample formats. DO NOT USE if linking dynamically.
Definition: samplefmt.h:63
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
#define AV_CODEC_PROP_INTRA_ONLY
Codec uses only intra compression.
Definition: libavcodec/avcodec.h:531
Definition: libavcodec/avcodec.h:100
int show_decoders(void *optctx, const char *opt, const char *arg)
Print a listing containing all the decoders supported by the program.
Definition: cmdutils.c:1411
Definition: pixfmt.h:67
AVCodec * avcodec_find_encoder(enum AVCodecID id)
Find a registered encoder with a matching codec ID.
Definition: libavcodec/utils.c:2378
static void finish_group(OptionParseContext *octx, int group_idx, const char *arg)
Definition: cmdutils.c:582
Definition: dict.h:80
int show_license(void *optctx, const char *opt, const char *arg)
Print the license of the program to stdout.
Definition: cmdutils.c:1075
void av_log_default_callback(void *ptr, int level, const char *fmt, va_list vl)
Definition: log.c:204
misc image utilities
external API header
int av_parse_time(int64_t *timeval, const char *timestr, int duration)
Parse timestr and return in *time a corresponding number of microseconds.
Definition: parseutils.c:530
if max(w)>1 w=0.9 *w/max(w)
int split_commandline(OptionParseContext *octx, int argc, char *argv[], const OptionDef *options, const OptionGroupDef *groups, int nb_groups)
Split the commandline into an intermediate form convenient for further processing.
Definition: cmdutils.c:678
int av_get_bits_per_pixel(const AVPixFmtDescriptor *pixdesc)
Return the number of bits per pixel used by the pixel format described by pixdesc.
Definition: pixdesc.c:1731
const AVClass * av_opt_child_class_next(const AVClass *parent, const AVClass *prev)
Iterate over potential AVOptions-enabled children of parent.
Definition: opt.c:1278
int opt_loglevel(void *optctx, const char *opt, const char *arg)
Set the libav* libraries log level.
Definition: cmdutils.c:784
int check_stream_specifier(AVFormatContext *s, AVStream *st, const char *spec)
Check if the given stream matches a stream specifier.
Definition: cmdutils.c:1828
#define AVFILTER_FLAG_DYNAMIC_INPUTS
The number of the filter inputs is not determined just by AVFilter.inputs.
Definition: avfilter.h:424
enum AVMediaType avfilter_pad_get_type(const AVFilterPad *pads, int pad_idx)
Get the type of an AVFilterPad.
Definition: avfilter.c:842
void show_banner(int argc, char **argv, const OptionDef *options)
Print the program banner to stderr.
Definition: cmdutils.c:1055
int show_protocols(void *optctx, const char *opt, const char *arg)
Print a listing containing all the protocols supported by the program.
Definition: cmdutils.c:1434
Definition: cmdutils.h:144
Definition: cmdutils.h:256
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
Definition: cmdutils.h:133
struct SwsContext * sws_getContext(int srcW, int srcH, enum AVPixelFormat srcFormat, int dstW, int dstH, enum AVPixelFormat dstFormat, int flags, SwsFilter *srcFilter, SwsFilter *dstFilter, const double *param)
Allocate and return an SwsContext.
Definition: libswscale/utils.c:1502
int show_formats(void *optctx, const char *opt, const char *arg)
Print a listing containing all the formats supported by the program.
Definition: cmdutils.c:1149
Definition: avformat.h:456
void av_log_set_callback(void(*callback)(void *, int, const char *, va_list))
Definition: log.c:279
AVDictionaryEntry * av_dict_get(AVDictionary *m, const char *key, const AVDictionaryEntry *prev, int flags)
Get a dictionary entry with matching key.
Definition: dict.c:39
int av_get_standard_channel_layout(unsigned index, uint64_t *layout, const char **name)
Get the value and name of a standard channel layout.
Definition: channel_layout.c:250
void av_max_alloc(size_t max)
Set the maximum size that may me allocated in one block.
Definition: mem.c:69
int show_pix_fmts(void *optctx, const char *opt, const char *arg)
Print a listing containing all the pixel formats supported by the program.
Definition: cmdutils.c:1486
AVDictionary * filter_codec_opts(AVDictionary *opts, enum AVCodecID codec_id, AVFormatContext *s, AVStream *st, AVCodec *codec)
Filter out options for given codec.
Definition: cmdutils.c:1836
int av_bprint_finalize(AVBPrint *buf, char **ret_str)
Finalize a print buffer.
Definition: bprint.c:193
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
void uninit_parse_context(OptionParseContext *octx)
Free all allocated memory in an OptionParseContext.
Definition: cmdutils.c:651
const AVCodecDescriptor * avcodec_descriptor_next(const AVCodecDescriptor *prev)
Iterate over all codec descriptors known to libavcodec.
Definition: codec_desc.c:2576
const AVClass * avresample_get_class(void)
Get the AVClass for AVAudioResampleContext.
Definition: libavresample/options.c:108
const AVClass * avcodec_get_class(void)
Get the AVClass for AVCodecContext.
Definition: libavcodec/options.c:239
const char * av_get_channel_description(uint64_t channel)
Get the description of a given channel.
Definition: channel_layout.c:225
int show_codecs(void *optctx, const char *opt, const char *arg)
Print a listing containing all the codecs supported by the program.
Definition: cmdutils.c:1321
Definition: cmdutils.h:241
Public dictionary API.
void log_callback_help(void *ptr, int level, const char *fmt, va_list vl)
Trivial log callback.
Definition: cmdutils.c:95
int opt_default(void *optctx, const char *opt, const char *arg)
Fallback for options that are not explicitly handled, these will be parsed through AVOptions...
Definition: cmdutils.c:483
Definition: samplefmt.h:50
AVOptions.
static unsigned get_codecs_sorted(const AVCodecDescriptor ***rcodecs)
Definition: cmdutils.c:1288
#define AV_CODEC_PROP_LOSSLESS
Codec supports lossless compression.
Definition: libavcodec/avcodec.h:541
int opt_max_alloc(void *optctx, const char *opt, const char *arg)
Definition: cmdutils.c:923
int avformat_match_stream_specifier(AVFormatContext *s, AVStream *st, const char *spec)
Check if the stream st contained in s is matched by the stream specifier spec.
Definition: libavformat/utils.c:4307
trying all byte sequences megabyte in length and selecting the best looking sequence will yield cases to try But first
Definition: rate_distortion.txt:12
#define AV_LOG_SKIP_REPEATED
Skip repeated messages, this requires the user app to use av_log() instead of (f)printf as the 2 woul...
Definition: log.h:219
static void init_parse_context(OptionParseContext *octx, const OptionGroupDef *groups, int nb_groups)
Definition: cmdutils.c:629
void init_opts(void)
Initialize the cmdutils option system, in particular allocate the *_opts contexts.
Definition: cmdutils.c:74
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
void parse_options(void *optctx, int argc, char **argv, const OptionDef *options, void(*parse_arg_function)(void *, const char *))
Definition: cmdutils.c:345
const AVFilter * avfilter_next(const AVFilter *prev)
Iterate over all registered filters.
Definition: avfilter.c:424
const AVClass * avformat_get_class(void)
Get the AVClass for AVFormatContext.
Definition: libavformat/options.c:120
void parse_loglevel(int argc, char **argv, const OptionDef *options)
Find the '-loglevel' option in the command line args and apply it.
Definition: cmdutils.c:459
#define AVFILTER_FLAG_DYNAMIC_OUTPUTS
The number of the filter outputs is not determined just by AVFilter.outputs.
Definition: avfilter.h:430
external API header
Definition: libavcodec/avcodec.h:4535
void show_help_options(const OptionDef *options, const char *msg, int req_flags, int rej_flags, int alt_flags)
Print help for all options matching specified flags.
Definition: cmdutils.c:147
int locate_option(int argc, char **argv, const OptionDef *options, const char *optname)
Return index of option opt in argv or 0 if not found.
Definition: cmdutils.c:409
#define AV_OPT_FLAG_ENCODING_PARAM
a generic parameter which can be set by the user for muxing or encoding
Definition: opt.h:281
AVDictionary ** setup_find_stream_info_opts(AVFormatContext *s, AVDictionary *codec_opts)
Setup AVCodecContext options for avformat_find_stream_info().
Definition: cmdutils.c:1892
AVCodec * avcodec_find_encoder_by_name(const char *name)
Find a registered encoder with the specified name.
Definition: libavcodec/utils.c:2383
int show_help(void *optctx, const char *opt, const char *arg)
Generic -h handler common to all fftools.
Definition: cmdutils.c:1693
static void * av_x_if_null(const void *p, const void *x)
Return x default pointer in case p is NULL.
Definition: avutil.h:250
Main libavdevice API header.
int flags
Option flags that must be set on each option that is applied to this group.
Definition: cmdutils.h:253
libswresample public header
Definition: dict.c:28
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
#define AV_OPT_FLAG_FILTERING_PARAM
a generic parameter which can be set by the user for filtering
Definition: opt.h:287
const char * long_name
Descriptive name for the format, meant to be more human-readable than name.
Definition: avformat.h:384
int show_sample_fmts(void *optctx, const char *opt, const char *arg)
Print a listing containing all the sample formats supported by the program.
Definition: cmdutils.c:1548
Definition: cmdutils.h:281
AVCodec * av_codec_next(const AVCodec *c)
If c is NULL, returns the first registered codec, if c is non-NULL, returns the next registered codec...
Definition: libavcodec/utils.c:128
Definition: swresample_internal.h:69
void av_bprint_init(AVBPrint *buf, unsigned size_init, unsigned size_max)
Init a print buffer.
Definition: bprint.c:68
static int av_bprint_is_complete(AVBPrint *buf)
Test if the print buffer is complete (not truncated).
Definition: bprint.h:166
void av_dict_free(AVDictionary **pm)
Free all the memory allocated for an AVDictionary struct and all keys and values. ...
Definition: dict.c:162
int parse_option(void *optctx, const char *opt, const char *arg, const OptionDef *options)
Parse one given option.
Definition: cmdutils.c:312
Definition: graph2dot.c:48
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
#define PRINT_CODEC_SUPPORTED(codec, field, type, list_name, term, get_name)
Definition: cmdutils.c:1197
#define PIX_FMT_PAL
Pixel format has a palette in data[1], values are indexes in this palette.
Definition: pixdesc.h:90
AVBitStreamFilter * av_bitstream_filter_next(AVBitStreamFilter *f)
Definition: bitstream_filter.c:28
const char * av_get_channel_name(uint64_t channel)
Get the name of a given channel.
Definition: channel_layout.c:214
int show_filters(void *optctx, const char *opt, const char *arg)
Print a listing containing all the filters supported by the program.
Definition: cmdutils.c:1449
size_t av_strlcpy(char *dst, const char *src, size_t size)
Copy the string src to dst, but no more than size - 1 bytes, and null-terminate dst.
Definition: avstring.c:82
int av_parse_cpu_caps(unsigned *flags, const char *s)
Parse CPU caps from a string and update the given AV_CPU_* flags based on that.
Definition: cpu.c:114
const AVCodecDescriptor * avcodec_descriptor_get(enum AVCodecID id)
Definition: codec_desc.c:2566
static const OptionDef * find_option(const OptionDef *po, const char *name)
Definition: cmdutils.c:188
int props
Codec properties, a combination of AV_CODEC_PROP_* flags.
Definition: libavcodec/avcodec.h:524
int sws_isSupportedInput(enum AVPixelFormat pix_fmt)
Return a positive value if pix_fmt is a supported input format, 0 otherwise.
Definition: libswscale/utils.c:201
const int program_birth_year
program birth year, defined by the program for show_banner()
Definition: ffmpeg.c:107
const AVOption * av_opt_find(void *obj, const char *name, const char *unit, int opt_flags, int search_flags)
Look for an option in an object.
Definition: opt.c:1223
enum AVPixelFormat av_pix_fmt_desc_get_id(const AVPixFmtDescriptor *desc)
Definition: pixdesc.c:1797
AVInputFormat * av_find_input_format(const char *short_name)
Find AVInputFormat based on the short name of the input format.
Definition: libavformat/utils.c:248
static void log_callback_report(void *ptr, int level, const char *fmt, va_list vl)
Definition: cmdutils.c:100
int opt_opencl(void *optctx, const char *opt, const char *arg)
#define CODEC_CAP_DRAW_HORIZ_BAND
Decoder can use draw_horiz_band callback.
Definition: libavcodec/avcodec.h:737
external API header
#define AV_OPT_SEARCH_CHILDREN
Search in possible children of the given object first.
Definition: opt.h:538
int show_bsfs(void *optctx, const char *opt, const char *arg)
Print a listing containing all the bit stream filters supported by the program.
Definition: cmdutils.c:1423
static void print_codecs_for_id(enum AVCodecID id, int encoder)
Definition: cmdutils.c:1309
const char * avio_enum_protocols(void **opaque, int output)
Iterate through names of available protocols.
Definition: avio.c:86
#define av_err2str(errnum)
Convenience macro, the return value should be used only directly in function arguments but never stan...
Definition: error.h:110
A list of option groups that all have the same group type (e.g.
Definition: cmdutils.h:274
void show_help_default(const char *opt, const char *arg)
Per-fftool specific help handler.
Definition: ffmpeg_opt.c:2374
#define PIX_FMT_BITSTREAM
All values of a component are bit-wise packed end to end.
Definition: pixdesc.h:91
int av_opencl_set_option(const char *key, const char *val)
Set option in the global OpenCL context.
Definition: opencl.c:320
AVOutputFormat * av_guess_format(const char *short_name, const char *filename, const char *mime_type)
Return the output format in the list of registered output formats which best matches the provided par...
Definition: libavformat/utils.c:188
int opt_timelimit(void *optctx, const char *opt, const char *arg)
Limit the execution time.
Definition: cmdutils.c:949
const AVCodecDescriptor * avcodec_descriptor_get_by_name(const char *name)
Definition: codec_desc.c:2585
AVOutputFormat * av_oformat_next(AVOutputFormat *f)
If f is NULL, returns the first registered output format, if f is non-NULL, returns the next register...
Definition: libavformat/utils.c:119
const char * long_name
Descriptive name for the format, meant to be more human-readable than name.
Definition: avformat.h:468
void av_log_format_line(void *ptr, int level, const char *fmt, va_list vl, char *line, int line_size, int *print_prefix)
Format a line of log the same way as the default callback.
Definition: log.c:196
static char get_media_type_char(enum AVMediaType type)
Definition: cmdutils.c:1256
double av_strtod(const char *numstr, char **tail)
Parse the string in numstr and return its value as a double.
Definition: eval.c:89
external API header
const char * long_name
Descriptive name for the codec, meant to be more human readable than name.
Definition: libavcodec/avcodec.h:2866
const AVClass * priv_class
A class for the private data, used to access filter private AVOptions.
Definition: avfilter.h:452
static const AVCodec * next_codec_for_id(enum AVCodecID id, const AVCodec *prev, int encoder)
Definition: cmdutils.c:1268
const AVRational * supported_framerates
array of supported framerates, or NULL if any, array is terminated by {0,0}
Definition: libavcodec/avcodec.h:2874
int sws_isSupportedOutput(enum AVPixelFormat pix_fmt)
Return a positive value if pix_fmt is a supported output format, 0 otherwise.
Definition: libswscale/utils.c:207
int av_opt_show2(void *obj, void *av_log_obj, int req_flags, int rej_flags)
Show the obj options.
Definition: opt.c:930
Close file fclose(fid)
Descriptor that unambiguously describes how the bits of a pixel are stored in the up to 4 data planes...
Definition: pixdesc.h:55
AVCodec * avcodec_find_decoder(enum AVCodecID id)
Find a registered decoder with a matching codec ID.
Definition: libavcodec/utils.c:2397
char * av_get_sample_fmt_string(char *buf, int buf_size, enum AVSampleFormat sample_fmt)
Generate a string corresponding to the sample format with sample_fmt, or a header if sample_fmt is ne...
Definition: samplefmt.c:91
av_cold void swr_free(SwrContext **ss)
Free the given SwrContext and set the pointer to NULL.
Definition: swresample.c:220
int av_dict_set(AVDictionary **pm, const char *key, const char *value, int flags)
Set the given entry in *pm, overwriting an existing entry.
Definition: dict.c:62
FILE * get_preset_file(char *filename, size_t filename_size, const char *preset_name, int is_path, const char *codec_name)
Get a file corresponding to a preset file.
Definition: cmdutils.c:1778
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
static void expand_filename_template(AVBPrint *bp, const char *template, struct tm *tm)
Definition: cmdutils.c:826
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
int64_t parse_time_or_die(const char *context, const char *timestr, int is_duration)
Parse a string specifying a time and return its corresponding value as a number of microseconds...
Definition: cmdutils.c:135
void * grow_array(void *array, int elem_size, int *size, int new_size)
Realloc array to hold new_size elements of elem_size.
Definition: cmdutils.c:1912
const char * name
Name of the codec described by this descriptor.
Definition: libavcodec/avcodec.h:516
const char * avfilter_pad_get_name(const AVFilterPad *pads, int pad_idx)
Get the name of an AVFilterPad.
Definition: avfilter.c:837
int av_get_cpu_flags(void)
Return the flags which specify extensions supported by the CPU.
Definition: cpu.c:30
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
misc parsing utilities
#define type
size_t av_strlcat(char *dst, const char *src, size_t size)
Append the string src to the string dst, but to a total length of no more than size - 1 bytes...
Definition: avstring.c:92
This struct describes the properties of a single codec described by an AVCodecID. ...
Definition: libavcodec/avcodec.h:508
double parse_number_or_die(const char *context, const char *numstr, int type, double min, double max)
Parse a string and return its corresponding value as a double.
Definition: cmdutils.c:114
void * av_calloc(size_t nmemb, size_t size)
Allocate a block of nmemb * size bytes with alignment suitable for all memory accesses (including vec...
Definition: mem.c:213
int cmdutils_read_file(const char *filename, char **bufptr, size_t *size)
Read the file with name filename, and put its content in a newly allocated 0-terminated buffer...
Definition: cmdutils.c:1736
AVCodec * avcodec_find_decoder_by_name(const char *name)
Find a registered decoder with the specified name.
Definition: libavcodec/utils.c:2402
static int match_group_separator(const OptionGroupDef *groups, int nb_groups, const char *opt)
Definition: cmdutils.c:562
int av_strerror(int errnum, char *errbuf, size_t errbuf_size)
Put a description of the AVERROR code errnum in errbuf.
Definition: error.c:53
static int av_toupper(int c)
Locale-independent conversion of ASCII characters to uppercase.
Definition: avstring.h:206
Main libavformat public API header.
void print_error(const char *filename, int err)
Print an error message to stderr, indicating filename and a human readable description of the error c...
Definition: cmdutils.c:982
#define CODEC_CAP_SLICE_THREADS
Codec supports slice-based (or partition-based) multithreading.
Definition: libavcodec/avcodec.h:814
#define CODEC_CAP_FRAME_THREADS
Codec supports frame-level multithreading.
Definition: libavcodec/avcodec.h:810
#define CODEC_CAP_EXPERIMENTAL
Codec is experimental and is thus avoided in favor of non experimental encoders.
Definition: libavcodec/avcodec.h:796
#define AV_OPT_SEARCH_FAKE_OBJ
The obj passed to av_opt_find() is fake – only a double pointer to AVClass instead of a required poi...
Definition: opt.h:547
Definition: avformat.h:377
void uninit_opts(void)
Uninitialize the cmdutils option system, in particular free the *_opts contexts and their contents...
Definition: cmdutils.c:82
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel layout
Definition: filter_design.txt:12
AVInputFormat * av_iformat_next(AVInputFormat *f)
If f is NULL, returns the first registered input format, if f is non-NULL, returns the next registere...
Definition: libavformat/utils.c:113
int av_opt_get_key_value(const char **ropts, const char *key_val_sep, const char *pairs_sep, unsigned flags, char **rkey, char **rval)
Extract a key-value pair from the beginning of a string.
Definition: opt.c:1120
printf("static const uint8_t my_array[100] = {\n")
static void prepare_app_arguments(int *argc_ptr, char ***argv_ptr)
Definition: cmdutils.c:257
static int write_option(void *optctx, const OptionDef *po, const char *opt, const char *arg)
Definition: cmdutils.c:263
void show_help_children(const AVClass *class, int flags)
Show help for all options with given flags in class and all its children.
Definition: cmdutils.c:176
int show_layouts(void *optctx, const char *opt, const char *arg)
Print a listing containing all the standard channel layouts supported by the program.
Definition: cmdutils.c:1519
#define GET_ARG(arg)
int avfilter_pad_count(const AVFilterPad *pads)
Get the number of elements in a NULL-terminated array of AVFilterPads (e.g.
Definition: avfilter.c:440
int opt_cpuflags(void *optctx, const char *opt, const char *arg)
Definition: cmdutils.c:937
OpenCL wrapper.
int read_yesno(void)
Return a positive value if a line read from standard input starts with [yY], otherwise return 0...
Definition: cmdutils.c:1725
const AVFilterPad * outputs
NULL terminated list of outputs. NULL if none.
Definition: avfilter.h:446
union OptionDef::@1 u
void sws_freeContext(struct SwsContext *swsContext)
Free the swscaler context swsContext.
Definition: libswscale/utils.c:1860
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
int show_version(void *optctx, const char *opt, const char *arg)
Print the version of the program to stdout.
Definition: cmdutils.c:1066
void av_force_cpu_flags(int arg)
Disables cpu detection and forces the specified flags.
Definition: cpu.c:25
AVFilter * avfilter_get_by_name(const char *name)
Get a filter definition matching the given name.
Definition: avfilter.c:391
Definition: swscale_internal.h:259
Definition: avutil.h:146
int av_opt_set(void *obj, const char *name, const char *val, int search_flags)
Definition: opt.c:252
const AVClass * sws_get_class(void)
Get the AVClass for swsContext.
Definition: libswscale/options.c:78
const char program_name[]
program name, defined by the program for show_version().
Definition: ffmpeg.c:106
static void show_help_codec(const char *name, int encoder)
Definition: cmdutils.c:1557
static int compare_codec_desc(const void *a, const void *b)
Definition: cmdutils.c:1279
int show_encoders(void *optctx, const char *opt, const char *arg)
Print a listing containing all the encoders supported by the program.
Definition: cmdutils.c:1417
simple arithmetic expression evaluator
const AVPixFmtDescriptor * av_pix_fmt_desc_next(const AVPixFmtDescriptor *prev)
Iterate over all pixel format descriptors known to libavutil.
Definition: pixdesc.c:1785
static void add_opt(OptionParseContext *octx, const OptionDef *opt, const char *key, const char *val)
Definition: cmdutils.c:617
void av_bprint_chars(AVBPrint *buf, char c, unsigned n)
Append char c n times to a print buffer.
Definition: bprint.c:116
Generated on Fri Jul 4 2025 06:54:36 for FFmpeg by
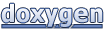