FFmpeg
|
avfilter.h File Reference
external API header More...
#include <stddef.h>
#include "libavutil/avutil.h"
#include "libavutil/dict.h"
#include "libavutil/frame.h"
#include "libavutil/log.h"
#include "libavutil/samplefmt.h"
#include "libavutil/pixfmt.h"
#include "libavutil/rational.h"
#include "libavfilter/version.h"
Include dependency graph for avfilter.h:
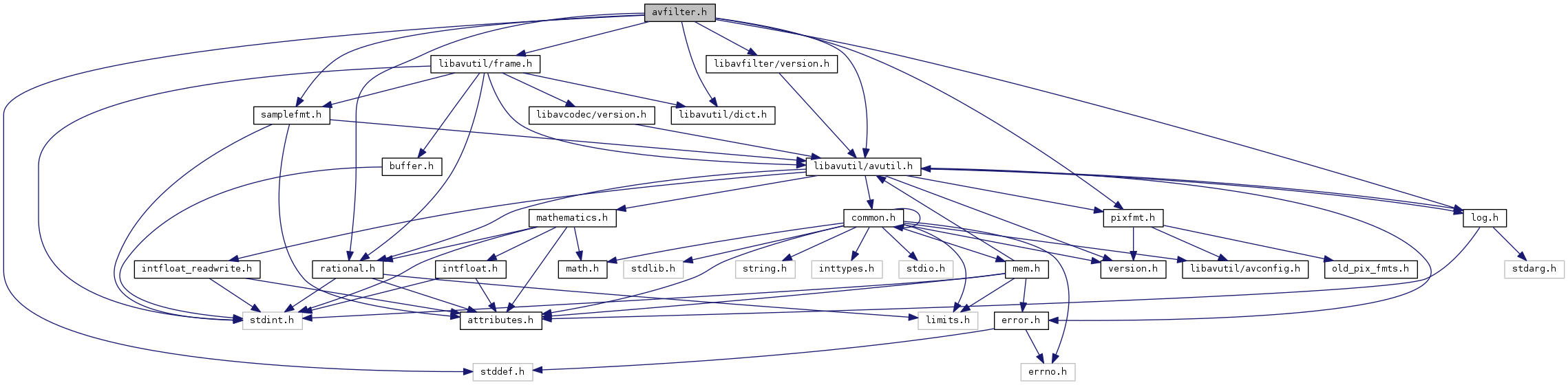
Go to the source code of this file.
Data Structures | |
struct | AVFilter |
Filter definition. More... | |
struct | AVFilterContext |
An instance of a filter. More... | |
struct | AVFilterLink |
A link between two filters. More... | |
struct | AVFilterGraph |
struct | AVFilterInOut |
A linked-list of the inputs/outputs of the filter chain. More... | |
Macros | |
#define | AVFILTER_FLAG_DYNAMIC_INPUTS (1 << 0) |
The number of the filter inputs is not determined just by AVFilter.inputs. More... | |
#define | AVFILTER_FLAG_DYNAMIC_OUTPUTS (1 << 1) |
The number of the filter outputs is not determined just by AVFilter.outputs. More... | |
#define | AVFILTER_CMD_FLAG_ONE 1 |
Stop once a filter understood the command (for target=all for example), fast filters are favored automatically. More... | |
#define | AVFILTER_CMD_FLAG_FAST 2 |
Only execute command when its fast (like a video out that supports contrast adjustment in hw) More... | |
Typedefs | |
typedef struct AVFilterContext | AVFilterContext |
typedef struct AVFilterLink | AVFilterLink |
typedef struct AVFilterPad | AVFilterPad |
typedef struct AVFilterFormats | AVFilterFormats |
typedef struct AVFilter | AVFilter |
Filter definition. More... | |
typedef struct AVFilterGraph | AVFilterGraph |
typedef struct AVFilterInOut | AVFilterInOut |
A linked-list of the inputs/outputs of the filter chain. More... | |
Enumerations | |
enum | { AVFILTER_AUTO_CONVERT_ALL = 0, AVFILTER_AUTO_CONVERT_NONE = -1 } |
Functions | |
unsigned | avfilter_version (void) |
Return the LIBAVFILTER_VERSION_INT constant. More... | |
const char * | avfilter_configuration (void) |
Return the libavfilter build-time configuration. More... | |
const char * | avfilter_license (void) |
Return the libavfilter license. More... | |
attribute_deprecated int | avfilter_ref_get_channels (AVFilterBufferRef *ref) |
Get the number of channels of a buffer reference. More... | |
int | avfilter_pad_count (const AVFilterPad *pads) |
Get the number of elements in a NULL-terminated array of AVFilterPads (e.g. More... | |
const char * | avfilter_pad_get_name (const AVFilterPad *pads, int pad_idx) |
Get the name of an AVFilterPad. More... | |
enum AVMediaType | avfilter_pad_get_type (const AVFilterPad *pads, int pad_idx) |
Get the type of an AVFilterPad. More... | |
int | avfilter_link (AVFilterContext *src, unsigned srcpad, AVFilterContext *dst, unsigned dstpad) |
Link two filters together. More... | |
void | avfilter_link_free (AVFilterLink **link) |
Free the link in *link, and set its pointer to NULL. More... | |
int | avfilter_link_get_channels (AVFilterLink *link) |
Get the number of channels of a link. More... | |
void | avfilter_link_set_closed (AVFilterLink *link, int closed) |
Set the closed field of a link. More... | |
int | avfilter_config_links (AVFilterContext *filter) |
Negotiate the media format, dimensions, etc of all inputs to a filter. More... | |
int | avfilter_process_command (AVFilterContext *filter, const char *cmd, const char *arg, char *res, int res_len, int flags) |
Make the filter instance process a command. More... | |
void | avfilter_register_all (void) |
Initialize the filter system. More... | |
int | avfilter_register (AVFilter *filter) |
Register a filter. More... | |
AVFilter * | avfilter_get_by_name (const char *name) |
Get a filter definition matching the given name. More... | |
const AVFilter * | avfilter_next (const AVFilter *prev) |
Iterate over all registered filters. More... | |
int | avfilter_init_str (AVFilterContext *ctx, const char *args) |
Initialize a filter with the supplied parameters. More... | |
int | avfilter_init_dict (AVFilterContext *ctx, AVDictionary **options) |
Initialize a filter with the supplied dictionary of options. More... | |
void | avfilter_free (AVFilterContext *filter) |
Free a filter context. More... | |
int | avfilter_insert_filter (AVFilterLink *link, AVFilterContext *filt, unsigned filt_srcpad_idx, unsigned filt_dstpad_idx) |
Insert a filter in the middle of an existing link. More... | |
const AVClass * | avfilter_get_class (void) |
AVFilterGraph * | avfilter_graph_alloc (void) |
Allocate a filter graph. More... | |
AVFilterContext * | avfilter_graph_alloc_filter (AVFilterGraph *graph, const AVFilter *filter, const char *name) |
Create a new filter instance in a filter graph. More... | |
AVFilterContext * | avfilter_graph_get_filter (AVFilterGraph *graph, char *name) |
Get a filter instance with name name from graph. More... | |
int | avfilter_graph_create_filter (AVFilterContext **filt_ctx, AVFilter *filt, const char *name, const char *args, void *opaque, AVFilterGraph *graph_ctx) |
Create and add a filter instance into an existing graph. More... | |
void | avfilter_graph_set_auto_convert (AVFilterGraph *graph, unsigned flags) |
Enable or disable automatic format conversion inside the graph. More... | |
int | avfilter_graph_config (AVFilterGraph *graphctx, void *log_ctx) |
Check validity and configure all the links and formats in the graph. More... | |
void | avfilter_graph_free (AVFilterGraph **graph) |
Free a graph, destroy its links, and set *graph to NULL. More... | |
AVFilterInOut * | avfilter_inout_alloc (void) |
Allocate a single AVFilterInOut entry. More... | |
void | avfilter_inout_free (AVFilterInOut **inout) |
Free the supplied list of AVFilterInOut and set *inout to NULL. More... | |
int | avfilter_graph_parse (AVFilterGraph *graph, const char *filters, AVFilterInOut **inputs, AVFilterInOut **outputs, void *log_ctx) |
Add a graph described by a string to a graph. More... | |
int | avfilter_graph_parse2 (AVFilterGraph *graph, const char *filters, AVFilterInOut **inputs, AVFilterInOut **outputs) |
Add a graph described by a string to a graph. More... | |
int | avfilter_graph_send_command (AVFilterGraph *graph, const char *target, const char *cmd, const char *arg, char *res, int res_len, int flags) |
Send a command to one or more filter instances. More... | |
int | avfilter_graph_queue_command (AVFilterGraph *graph, const char *target, const char *cmd, const char *arg, int flags, double ts) |
Queue a command for one or more filter instances. More... | |
char * | avfilter_graph_dump (AVFilterGraph *graph, const char *options) |
Dump a graph into a human-readable string representation. More... | |
int | avfilter_graph_request_oldest (AVFilterGraph *graph) |
Request a frame on the oldest sink link. More... | |
Detailed Description
external API header
Definition in file avfilter.h.
Generated on Wed Jun 18 2025 06:53:11 for FFmpeg by
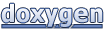