FFmpeg
|
avfilter.h
Go to the documentation of this file.
112 #define AV_PERM_REUSE 0x08 ///< can output the buffer multiple times, with the same contents each time
504 * @param cmd the command to process, for handling simplicity all commands must be alphanumeric only
506 * @param res a buffer with size res_size where the filter(s) can return a response. This must not change when the command is not supported.
513 int (*process_command)(AVFilterContext *, const char *cmd, const char *arg, char *res, int res_len, int flags);
772 avfilter_get_video_buffer_ref_from_arrays(uint8_t * const data[4], const int linesize[4], int perms,
821 #define AVFILTER_CMD_FLAG_ONE 1 ///< Stop once a filter understood the command (for target=all for example), fast filters are favored automatically
822 #define AVFILTER_CMD_FLAG_FAST 2 ///< Only execute command when its fast (like a video out that supports contrast adjustment in hw)
828 int avfilter_process_command(AVFilterContext *filter, const char *cmd, const char *arg, char *res, int res_len, int flags);
1003 char *resample_lavr_opts; ///< libavresample options to use for the auto-inserted resample filters
1007 char *aresample_swr_opts; ///< swr options to use for the auto-inserted aresample filters, Access ONLY through AVOptions
1209 * @param cmd the command to sent, for handling simplicity all commands must be alphanumeric only
1216 int avfilter_graph_send_command(AVFilterGraph *graph, const char *target, const char *cmd, const char *arg, char *res, int res_len, int flags);
1226 * @param cmd the command to sent, for handling simplicity all commands must be alphanummeric only
1233 int avfilter_graph_queue_command(AVFilterGraph *graph, const char *target, const char *cmd, const char *arg, int flags, double ts);
void avfilter_graph_set_auto_convert(AVFilterGraph *graph, unsigned flags)
Enable or disable automatic format conversion inside the graph.
Definition: avfiltergraph.c:135
void avfilter_link_set_closed(AVFilterLink *link, int closed)
Set the closed field of a link.
Definition: avfilter.c:160
int avfilter_graph_queue_command(AVFilterGraph *graph, const char *target, const char *cmd, const char *arg, int flags, double ts)
Queue a command for one or more filter instances.
Definition: avfiltergraph.c:1092
int avfilter_graph_config(AVFilterGraph *graphctx, void *log_ctx)
Check validity and configure all the links and formats in the graph.
Definition: avfiltergraph.c:1044
void avfilter_inout_free(AVFilterInOut **inout)
Free the supplied list of AVFilterInOut and set *inout to NULL.
Definition: graphparser.c:175
struct AVFilterInOut * next
next input/input in the list, NULL if this is the last
Definition: avfilter.h:1134
enum AVMediaType avfilter_pad_get_type(const AVFilterPad *pads, int pad_idx)
Get the type of an AVFilterPad.
Definition: avfilter.c:842
Libavfilter version macros.
int request_samples
Audio only, the destination filter sets this to a non-zero value to request that buffers with the giv...
Definition: avfilter.h:620
void avfilter_graph_free(AVFilterGraph **graph)
Free a graph, destroy its links, and set *graph to NULL.
Definition: avfiltergraph.c:75
external API header
Definition: avisynth_c.h:503
struct AVFilterChannelLayouts * in_channel_layouts
Definition: avfilter.h:610
void avfilter_unref_buffer(AVFilterBufferRef *ref)
Definition: libavfilter/buffer.c:95
char * scale_sws_opts
sws options to use for the auto-inserted scale filters
Definition: avfilter.h:1002
Public dictionary API.
int avfilter_link(AVFilterContext *src, unsigned srcpad, AVFilterContext *dst, unsigned dstpad)
Link two filters together.
Definition: avfilter.c:114
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
char * resample_lavr_opts
libavresample options to use for the auto-inserted resample filters
Definition: avfilter.h:1003
int avfilter_config_links(AVFilterContext *filter)
Negotiate the media format, dimensions, etc of all inputs to a filter.
Definition: avfilter.c:203
const AVFilter * avfilter_next(const AVFilter *prev)
Iterate over all registered filters.
Definition: avfilter.c:424
int avfilter_graph_parse2(AVFilterGraph *graph, const char *filters, AVFilterInOut **inputs, AVFilterInOut **outputs)
Add a graph described by a string to a graph.
Definition: graphparser.c:379
Definition: dict.c:28
AVRational frame_rate
Frame rate of the stream on the link, or 1/0 if unknown; if left to 0/0, will be automatically be cop...
Definition: avfilter.h:657
int avfilter_link_get_channels(AVFilterLink *link)
Get the number of channels of a link.
Definition: avfilter.c:155
unsigned avfilter_version(void)
Return the LIBAVFILTER_VERSION_INT constant.
Definition: avfilter.c:68
struct AVFilterInOut AVFilterInOut
A linked-list of the inputs/outputs of the filter chain.
AVRational time_base
Define the time base used by the PTS of the frames/samples which will pass through this link...
Definition: avfilter.h:585
struct AVFilterChannelLayouts * out_channel_layouts
Definition: avfilter.h:611
AVFilterFormats * in_formats
Lists of formats and channel layouts supported by the input and output filters respectively.
Definition: avfilter.h:601
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a link
Definition: filter_design.txt:23
Note except for filters that can have queued request_frame does not push and as a the filter_frame method will be called and do the work Legacy the filter_frame method was it was made of draw_slice(that could be called several times on distinct parts of the frame) and end_frame
static av_cold int init_dict(AVFilterContext *ctx, AVDictionary **opts)
Definition: af_aresample.c:46
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
struct AVFilterGraph AVFilterGraph
Definition: avfilter.h:991
static int process_command(AVFilterContext *ctx, const char *cmd, const char *arg, char *res, int res_len, int flags)
Definition: af_atempo.c:1141
AVFrame * partial_buf
Buffer partially filled with samples to achieve a fixed/minimum size.
Definition: avfilter.h:662
AVFilterContext * filter_ctx
filter context associated to this input/output
Definition: avfilter.h:1128
void avfilter_copy_buffer_ref_props(AVFilterBufferRef *dst, AVFilterBufferRef *src)
Definition: libavfilter/buffer.c:148
int avfilter_init_str(AVFilterContext *ctx, const char *args)
Initialize a filter with the supplied parameters.
Definition: avfilter.c:715
int avfilter_insert_filter(AVFilterLink *link, AVFilterContext *filt, unsigned filt_srcpad_idx, unsigned filt_dstpad_idx)
Insert a filter in the middle of an existing link.
Definition: avfilter.c:165
const AVClass * priv_class
A class for the private data, used to access filter private AVOptions.
Definition: avfilter.h:452
AVFilterFormats * in_samplerates
Lists of channel layouts and sample rates used for automatic negotiation.
Definition: avfilter.h:608
AVFilterBufferRef * cur_buf_copy
The buffer reference currently being received across the link by the destination filter.
Definition: avfilter.h:692
int avfilter_graph_request_oldest(AVFilterGraph *graph)
Request a frame on the oldest sink link.
Definition: avfiltergraph.c:1166
int avfilter_process_command(AVFilterContext *filter, const char *cmd, const char *arg, char *res, int res_len, int flags)
Make the filter instance process a command.
Definition: avfilter.c:378
int avfilter_graph_create_filter(AVFilterContext **filt_ctx, AVFilter *filt, const char *name, const char *args, void *opaque, AVFilterGraph *graph_ctx)
Create and add a filter instance into an existing graph.
Definition: avfiltergraph.c:112
const char * avfilter_configuration(void)
Return the libavfilter build-time configuration.
Definition: avfilter.c:73
const char * avfilter_pad_get_name(const AVFilterPad *pads, int pad_idx)
Get the name of an AVFilterPad.
Definition: avfilter.c:837
#define type
Definition: libavfilter/internal.h:40
int(* init_opaque)(AVFilterContext *ctx, void *opaque)
Filter initialization function, alternative to the init() callback.
Definition: avfilter.h:520
int avfilter_init_dict(AVFilterContext *ctx, AVDictionary **options)
Initialize a filter with the supplied dictionary of options.
Definition: avfilter.c:693
attribute_deprecated int avfilter_ref_get_channels(AVFilterBufferRef *ref)
Get the number of channels of a buffer reference.
Definition: audio.c:31
AVFilterInOut * avfilter_inout_alloc(void)
Allocate a single AVFilterInOut entry.
Definition: graphparser.c:170
int avfilter_copy_frame_props(AVFilterBufferRef *dst, const AVFrame *src)
Definition: libavfilter/buffer.c:118
rational numbers
char * avfilter_graph_dump(AVFilterGraph *graph, const char *options)
Dump a graph into a human-readable string representation.
Definition: graphdump.c:153
uint64_t channel_layout
channel layout of current buffer (see libavutil/channel_layout.h)
Definition: avfilter.h:573
int avfilter_graph_send_command(AVFilterGraph *graph, const char *target, const char *cmd, const char *arg, char *res, int res_len, int flags)
Send a command to one or more filter instances.
Definition: avfiltergraph.c:1062
static int filter_frame(AVFilterLink *inlink, AVFrame *insamplesref)
Definition: af_aconvert.c:140
char * aresample_swr_opts
swr options to use for the auto-inserted aresample filters, Access ONLY through AVOptions ...
Definition: avfilter.h:1007
Definition: libavfilter/internal.h:33
A Quick Description Of Rate Distortion Theory We want to encode a video
Definition: rate_distortion.txt:3
pixel format definitions
AVFilterContext * avfilter_graph_alloc_filter(AVFilterGraph *graph, const AVFilter *filter, const char *name)
Create a new filter instance in a filter graph.
Definition: avfiltergraph.c:140
int avfilter_pad_count(const AVFilterPad *pads)
Get the number of elements in a NULL-terminated array of AVFilterPads (e.g.
Definition: avfilter.c:440
const AVFilterPad * outputs
NULL terminated list of outputs. NULL if none.
Definition: avfilter.h:446
AVFilterBufferRef * avfilter_ref_buffer(AVFilterBufferRef *ref, int pmask)
Definition: libavfilter/buffer.c:51
AVFilterContext * avfilter_graph_get_filter(AVFilterGraph *graph, char *name)
Get a filter instance with name name from graph.
Definition: avfiltergraph.c:231
Note except for filters that can have queued request_frame does not push and as a the filter_frame method will be called and do the work Legacy the filter_frame method was it was made of start_frame
Definition: filter_design.txt:263
AVFilter * avfilter_get_by_name(const char *name)
Get a filter definition matching the given name.
Definition: avfilter.c:391
these buffered frames must be flushed immediately if a new input produces new the filter must not call request_frame to get more It must just process the frame or queue it The task of requesting more frames is left to the filter s request_frame method or the application If a filter has several inputs
Definition: filter_design.txt:216
void avfilter_link_free(AVFilterLink **link)
Free the link in *link, and set its pointer to NULL.
Definition: avfilter.c:145
int64_t current_pts
Current timestamp of the link, as defined by the most recent frame(s), in AV_TIME_BASE units...
Definition: avfilter.h:640
void avfilter_unref_bufferp(AVFilterBufferRef **ref)
Definition: libavfilter/buffer.c:112
MUSIC TECHNOLOGY GROUP UNIVERSITAT POMPEU FABRA Free Non Commercial Binary License Agreement UNIVERSITAT POMPEU OR INDICATING ACCEPTANCE BY SELECTING THE ACCEPT BUTTON ON ANY DOWNLOAD OR INSTALL YOU ACCEPT THE TERMS OF THE LICENSE SUMMARY TABLE Software MELODIA Melody Extraction vamp plug in Licensor Music Technology Group Universitat Pompeu Plaça de la Spain Permitted purposes Non commercial internal research and validation and educational purposes only All commercial uses in a production either internal or are prohibited by this license and require an additional commercial exploitation license TERMS AND CONDITIONS SOFTWARE Software means the software programs identified herein in binary any other machine readable any updates or error corrections provided by and any user programming guides and other documentation provided to you by UPF under this Agreement LICENSE Subject to the terms and conditions of this UPF grants you a royalty free
Definition: MELODIA - License.txt:16
int avfilter_graph_parse(AVFilterGraph *graph, const char *filters, AVFilterInOut **inputs, AVFilterInOut **outputs, void *log_ctx)
Add a graph described by a string to a graph.
Definition: graphparser.c:447
Generated on Thu May 29 2025 06:52:45 for FFmpeg by
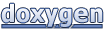