FFmpeg
|
avfiltergraph.c
Go to the documentation of this file.
39 {"scale_sws_opts" , "default scale filter options" , OFFSET(scale_sws_opts) , AV_OPT_TYPE_STRING, {.str = NULL}, 0, 0, 0 },
40 {"aresample_swr_opts" , "default aresample filter options" , OFFSET(aresample_swr_opts) , AV_OPT_TYPE_STRING, {.str = NULL}, 0, 0, 0 },
189 "Input pad \"%s\" with type %s of the filter instance \"%s\" of %s not connected to any source\n",
199 "Output pad \"%s\" with type %s of the filter instance \"%s\" of %s not connected to any destination\n",
1062 int avfilter_graph_send_command(AVFilterGraph *graph, const char *target, const char *cmd, const char *arg, char *res, int res_len, int flags)
1070 r=avfilter_graph_send_command(graph, target, cmd, arg, res, res_len, flags | AVFILTER_CMD_FLAG_FAST);
1080 if(!strcmp(target, "all") || (filter->name && !strcmp(target, filter->name)) || !strcmp(target, filter->filter->name)){
1092 int avfilter_graph_queue_command(AVFilterGraph *graph, const char *target, const char *command, const char *arg, int flags, double ts)
1101 if(filter && (!strcmp(target, "all") || !strcmp(target, filter->name) || !strcmp(target, filter->filter->name))){
void avfilter_graph_set_auto_convert(AVFilterGraph *graph, unsigned flags)
Enable or disable automatic format conversion inside the graph.
Definition: avfiltergraph.c:135
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
AVFilterContext * ff_filter_alloc(const AVFilter *filter, const char *inst_name)
Allocate a new filter context and return it.
Definition: avfilter.c:495
const AVPixFmtDescriptor * av_pix_fmt_desc_get(enum AVPixelFormat pix_fmt)
Definition: pixdesc.c:1778
int avfilter_graph_queue_command(AVFilterGraph *graph, const char *target, const char *command, const char *arg, int flags, double ts)
Queue a command for one or more filter instances.
Definition: avfiltergraph.c:1092
Definition: pixfmt.h:67
external API header
int avfilter_graph_config(AVFilterGraph *graphctx, void *log_ctx)
Check validity and configure all the links and formats in the graph.
Definition: avfiltergraph.c:1044
struct AVFilterInOut * next
next input/input in the list, NULL if this is the last
Definition: avfilter.h:1134
void avfilter_graph_free(AVFilterGraph **graph)
Free a graph, destroy its links, and set *graph to NULL.
Definition: avfiltergraph.c:75
Definition: avisynth_c.h:503
void * av_realloc(void *ptr, size_t size)
Allocate or reallocate a block of memory.
Definition: mem.c:141
static int reduce_formats_on_filter(AVFilterContext *filter)
Definition: avfiltergraph.c:575
struct AVFilterChannelLayouts * in_channel_layouts
Definition: avfilter.h:610
static int graph_insert_fifos(AVFilterGraph *graph, AVClass *log_ctx)
Definition: avfiltergraph.c:1006
char * scale_sws_opts
sws options to use for the auto-inserted scale filters
Definition: avfilter.h:1002
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
#define REDUCE_FORMATS(fmt_type, list_type, list, var, nb, add_format)
Definition: avfiltergraph.c:540
static int pick_format(AVFilterLink *link, AVFilterLink *ref)
Definition: avfiltergraph.c:482
const char * class_name
The name of the class; usually it is the same name as the context structure type to which the AVClass...
Definition: log.h:55
AVOptions.
#define FF_LAYOUT2COUNT(l)
Decode a channel count encoded as a channel layout.
Definition: formats.h:108
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
Definition: opt.h:226
enum AVSampleFormat av_get_planar_sample_fmt(enum AVSampleFormat sample_fmt)
Get the planar alternative form of the given sample format.
Definition: samplefmt.c:82
int avfilter_config_links(AVFilterContext *filter)
Negotiate the media format, dimensions, etc of all inputs to a filter.
Definition: avfilter.c:203
void ff_set_common_formats(AVFilterContext *ctx, AVFilterFormats *formats)
A helper for query_formats() which sets all links to the same list of formats.
Definition: formats.c:545
AVFilterFormats * ff_all_formats(enum AVMediaType type)
Return a list of all formats supported by FFmpeg for the given media type.
Definition: formats.c:357
int ff_add_channel_layout(AVFilterChannelLayouts **l, uint64_t channel_layout)
Definition: formats.c:350
void av_bprint_init(AVBPrint *buf, unsigned size_init, unsigned size_max)
Init a print buffer.
Definition: bprint.c:68
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip please avoid problems through this extra level of scrutiny For cosmetics only commits you should e g by running git config global user name My Name git config global user email my email which is either set in your personal configuration file through git config core editor or set by one of the following environment VISUAL or EDITOR Log messages should be concise but descriptive Explain why you made a change
Definition: git-howto.txt:153
Definition: log.h:36
int av_get_channel_layout_nb_channels(uint64_t channel_layout)
Return the number of channels in the channel layout.
Definition: channel_layout.c:191
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
struct AVFilterChannelLayouts * out_channel_layouts
Definition: avfilter.h:611
int ff_add_format(AVFilterFormats **avff, int64_t fmt)
Add fmt to the list of media formats contained in *avff.
Definition: formats.c:344
AVFilterFormats * in_formats
Lists of formats and channel layouts supported by the input and output filters respectively.
Definition: avfilter.h:601
static void heap_bubble_down(AVFilterGraph *graph, AVFilterLink *link, int index)
Definition: avfiltergraph.c:1137
external API header
size_t av_strlcpy(char *dst, const char *src, size_t size)
Copy the string src to dst, but no more than size - 1 bytes, and null-terminate dst.
Definition: avstring.c:82
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a link
Definition: filter_design.txt:23
static int graph_config_formats(AVFilterGraph *graph, AVClass *log_ctx)
Configure the formats of all the links in the graph.
Definition: avfiltergraph.c:937
audio channel layout utility functions
static void sanitize_channel_layouts(void *log, AVFilterChannelLayouts *l)
Definition: avfiltergraph.c:242
static void swap_sample_fmts_on_filter(AVFilterContext *filter)
Definition: avfiltergraph.c:813
#define av_err2str(errnum)
Convenience macro, the return value should be used only directly in function arguments but never stan...
Definition: error.h:110
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
enum AVSampleFormat av_get_packed_sample_fmt(enum AVSampleFormat sample_fmt)
Get the packed alternative form of the given sample format.
Definition: samplefmt.c:73
static int graph_check_validity(AVFilterGraph *graph, AVClass *log_ctx)
Check for the validity of graph.
Definition: avfiltergraph.c:176
#define AVFILTER_CMD_FLAG_ONE
Stop once a filter understood the command (for target=all for example), fast filters are favored auto...
Definition: avfilter.h:821
Definition: avfilter.h:991
AVFilterChannelLayouts * ff_all_channel_layouts(void)
Construct an empty AVFilterChannelLayouts/AVFilterFormats struct – representing any channel layout (...
Definition: formats.c:402
int avfilter_init_str(AVFilterContext *filter, const char *args)
Initialize a filter with the supplied parameters.
Definition: avfilter.c:715
int av_get_bytes_per_sample(enum AVSampleFormat sample_fmt)
Return number of bytes per sample.
Definition: samplefmt.c:104
int avfilter_insert_filter(AVFilterLink *link, AVFilterContext *filt, unsigned filt_srcpad_idx, unsigned filt_dstpad_idx)
Insert a filter in the middle of an existing link.
Definition: avfilter.c:165
enum AVPixelFormat avcodec_find_best_pix_fmt_of_2(enum AVPixelFormat dst_pix_fmt1, enum AVPixelFormat dst_pix_fmt2, enum AVPixelFormat src_pix_fmt, int has_alpha, int *loss_ptr)
Find the best pixel format to convert to given a certain source pixel format and a selection of two d...
Definition: imgconvert.c:218
AVFilterFormats * in_samplerates
Lists of channel layouts and sample rates used for automatic negotiation.
Definition: avfilter.h:608
static void heap_bubble_up(AVFilterGraph *graph, AVFilterLink *link, int index)
Definition: avfiltergraph.c:1120
AVFilterChannelLayouts * ff_merge_channel_layouts(AVFilterChannelLayouts *a, AVFilterChannelLayouts *b)
Return a channel layouts/samplerates list which contains the intersection of the layouts/samplerates ...
Definition: formats.c:166
AVFilterFormats * ff_merge_formats(AVFilterFormats *a, AVFilterFormats *b, enum AVMediaType type)
Return a format list which contains the intersection of the formats of a and b.
Definition: formats.c:92
static int command(AVFilterContext *ctx, const char *cmd, const char *arg, char *res, int res_len, int flags)
Definition: vf_drawtext.c:596
void ff_avfilter_graph_update_heap(AVFilterGraph *graph, AVFilterLink *link)
Update the position of a link in the age heap.
Definition: avfiltergraph.c:1159
void ff_channel_layouts_unref(AVFilterChannelLayouts **ref)
Remove a reference to a channel layouts list.
Definition: formats.c:473
int avfilter_graph_request_oldest(AVFilterGraph *graph)
Request a frame on the oldest sink link.
Definition: avfiltergraph.c:1166
int avfilter_process_command(AVFilterContext *filter, const char *cmd, const char *arg, char *res, int res_len, int flags)
Make the filter instance process a command.
Definition: avfilter.c:378
int avfilter_graph_create_filter(AVFilterContext **filt_ctx, AVFilter *filt, const char *name, const char *args, void *opaque, AVFilterGraph *graph_ctx)
Create and add a filter instance into an existing graph.
Definition: avfiltergraph.c:112
int(* query_formats)(AVFilterContext *)
Queries formats/layouts supported by the filter and its pads, and sets the in_formats/in_chlayouts fo...
Definition: avfilter.h:495
#define AVFILTER_CMD_FLAG_FAST
Only execute command when its fast (like a video out that supports contrast adjustment in hw) ...
Definition: avfilter.h:822
void ff_formats_unref(AVFilterFormats **ref)
If *ref is non-NULL, remove *ref as a reference to the format list it currently points to...
Definition: formats.c:468
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
#define type
size_t av_strlcat(char *dst, const char *src, size_t size)
Append the string src to the string dst, but to a total length of no more than size - 1 bytes...
Definition: avstring.c:92
Definition: libavfilter/internal.h:40
static void swap_channel_layouts_on_filter(AVFilterContext *filter)
Definition: avfiltergraph.c:716
void * av_calloc(size_t nmemb, size_t size)
Allocate a block of nmemb * size bytes with alignment suitable for all memory accesses (including vec...
Definition: mem.c:213
const char * av_get_media_type_string(enum AVMediaType media_type)
Return a string describing the media_type enum, NULL if media_type is unknown.
Definition: libavutil/utils.c:64
static int query_formats(AVFilterGraph *graph, AVClass *log_ctx)
Definition: avfiltergraph.c:319
void ff_set_common_samplerates(AVFilterContext *ctx, AVFilterFormats *samplerates)
Definition: formats.c:533
static int graph_config_links(AVFilterGraph *graph, AVClass *log_ctx)
Configure all the links of graphctx.
Definition: avfiltergraph.c:214
static void swap_channel_layouts(AVFilterGraph *graph)
Definition: avfiltergraph.c:805
#define MERGE_DISPATCH(field, statement)
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output links
Definition: filter_design.txt:12
The official guide to swscale for confused that consecutive non overlapping rectangles of slice_bottom special converter These generally are unscaled converters of common formats
Definition: swscale.txt:33
uint64_t channel_layout
channel layout of current buffer (see libavutil/channel_layout.h)
Definition: avfilter.h:573
int avfilter_graph_send_command(AVFilterGraph *graph, const char *target, const char *cmd, const char *arg, char *res, int res_len, int flags)
Send a command to one or more filter instances.
Definition: avfiltergraph.c:1062
static void swap_samplerates_on_filter(AVFilterContext *filter)
Definition: avfiltergraph.c:635
char * aresample_swr_opts
swr options to use for the auto-inserted aresample filters, Access ONLY through AVOptions ...
Definition: avfilter.h:1007
void ff_filter_graph_remove_filter(AVFilterGraph *graph, AVFilterContext *filter)
Remove a filter from a graph;.
Definition: avfiltergraph.c:62
AVFilterContext * avfilter_graph_alloc_filter(AVFilterGraph *graph, const AVFilter *filter, const char *name)
Create a new filter instance in a filter graph.
Definition: avfiltergraph.c:140
AVFilterContext * avfilter_graph_get_filter(AVFilterGraph *graph, char *name)
Get a filter instance with name name from graph.
Definition: avfiltergraph.c:231
Definition: avutil.h:143
void ff_set_common_channel_layouts(AVFilterContext *ctx, AVFilterChannelLayouts *layouts)
A helper for query_formats() which sets all links to the same list of channel layouts/sample rates...
Definition: formats.c:526
int needs_fifo
The filter expects a fifo to be inserted on its input link, typically because it has a delay...
Definition: libavfilter/internal.h:140
int ff_request_frame(AVFilterLink *link)
Request an input frame from the filter at the other end of the link.
Definition: avfilter.c:319
const char * av_get_pix_fmt_name(enum AVPixelFormat pix_fmt)
Return the short name for a pixel format, NULL in case pix_fmt is unknown.
Definition: pixdesc.c:1700
static int filter_query_formats(AVFilterContext *ctx)
Definition: avfiltergraph.c:257
AVFilterFormats * ff_merge_samplerates(AVFilterFormats *a, AVFilterFormats *b)
Definition: formats.c:139
internal API functions
AVFilter * avfilter_get_by_name(const char *name)
Get a filter definition matching the given name.
Definition: avfilter.c:391
static int ff_avfilter_graph_config_pointers(AVFilterGraph *graph, AVClass *log_ctx)
Definition: avfiltergraph.c:964
int64_t current_pts
Current timestamp of the link, as defined by the most recent frame(s), in AV_TIME_BASE units...
Definition: avfilter.h:640
Generated on Wed May 7 2025 06:52:38 for FFmpeg by
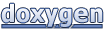