FFmpeg
|
libavfilter/internal.h
Go to the documentation of this file.
struct AVFilterCommand AVFilterCommand
int ff_filter_frame(AVFilterLink *link, AVFrame *frame)
Send a frame of data to the next filter.
Definition: avfilter.c:964
external API header
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining list
Definition: filter_design.txt:23
void ff_avfilter_graph_update_heap(AVFilterGraph *graph, AVFilterLink *link)
Update the position of a link in the age heap.
Definition: avfiltergraph.c:1159
int ff_fmt_is_in(int fmt, const int *fmts)
Tell is a format is contained in the provided list terminated by -1.
Definition: formats.c:254
int ff_parse_pixel_format(enum AVPixelFormat *ret, const char *arg, void *log_ctx)
Parse a pixel format.
Definition: formats.c:579
the mask is usually to keep the same permissions Filters should remove permissions on reference they give to output whenever necessary It can be automatically done by setting the rej_perms field on the output pad Here are a few guidelines corresponding to common then the filter should push the output frames on the output link immediately As an exception to the previous rule if the input frame is enough to produce several output frames then the filter needs output only at least one per link The additional frames can be left buffered in the filter
Definition: filter_design.txt:201
void ff_update_link_current_pts(AVFilterLink *link, int64_t pts)
Definition: avfilter.c:368
int ff_parse_channel_layout(int64_t *ret, const char *arg, void *log_ctx)
Parse a channel layout or a corresponding integer representation.
Definition: formats.c:632
struct AVFilterPool AVFilterPool
int ff_parse_time_base(AVRational *ret, const char *arg, void *log_ctx)
Parse a time base.
Definition: formats.c:609
int64_t * ff_copy_int64_list(const int64_t *const list)
Return a copy of a list of 64-bit integers, or NULL in case of copy failure.
Definition: formats.c:284
void ff_insert_pad(unsigned idx, unsigned *count, size_t padidx_off, AVFilterPad **pads, AVFilterLink ***links, AVFilterPad *newpad)
Insert a new pad.
Definition: avfilter.c:93
AVFilterBufferRef * ff_copy_buffer_ref(AVFilterLink *outlink, AVFilterBufferRef *ref)
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a link
Definition: filter_design.txt:23
static void ff_insert_outpad(AVFilterContext *f, unsigned index, AVFilterPad *p)
Insert a new output pad for the filter.
Definition: libavfilter/internal.h:265
int ff_buffersink_read_compat(AVFilterContext *ctx, AVFilterBufferRef **buf)
Definition: avfilter.h:991
char * ff_get_ref_perms_string(char *buf, size_t buf_size, int perms)
void ff_filter_graph_remove_filter(AVFilterGraph *graph, AVFilterContext *filter)
Remove a filter from a graph;.
Definition: avfiltergraph.c:62
#define type
Definition: libavfilter/internal.h:40
int ff_buffersink_read_samples_compat(AVFilterContext *ctx, AVFilterBufferRef **pbuf, int nb_samples)
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output links
Definition: filter_design.txt:12
void ff_avfilter_default_free_buffer(AVFilterBuffer *buf)
default handler for freeing audio/video buffer when there are no references left
Definition: libavfilter/buffer.c:34
int ff_parse_sample_format(int *ret, const char *arg, void *log_ctx)
Parse a sample format name or a corresponding integer representation.
Definition: formats.c:594
static int filter_frame(AVFilterLink *inlink, AVFrame *insamplesref)
Definition: af_aconvert.c:140
Definition: libavfilter/internal.h:33
static void ff_insert_inpad(AVFilterContext *f, unsigned index, AVFilterPad *p)
Insert a new input pad for the filter.
Definition: libavfilter/internal.h:254
int ff_parse_sample_rate(int *ret, const char *arg, void *log_ctx)
Parse a sample rate.
Definition: formats.c:620
AVFilterContext * ff_filter_alloc(const AVFilter *filter, const char *inst_name)
Allocate a new filter context and return it.
Definition: avfilter.c:495
int needs_fifo
The filter expects a fifo to be inserted on its input link, typically because it has a delay...
Definition: libavfilter/internal.h:140
int ff_request_frame(AVFilterLink *link)
Request an input frame from the filter at the other end of the link.
Definition: avfilter.c:319
Frame requests may need to loop in order to be fulfilled.
Definition: libavfilter/internal.h:338
int * ff_copy_int_list(const int *const list)
Return a copy of a list of integers terminated by -1, or NULL in case of copy failure.
Definition: formats.c:277
Generated on Tue Mar 25 2025 06:54:24 for FFmpeg by
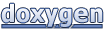