FFmpeg
|
libavfilter/buffer.c
Go to the documentation of this file.
42 static void copy_video_props(AVFilterBufferRefVideoProps *dst, AVFilterBufferRefVideoProps *src) {
misc image utilities
external API header
static void copy_video_props(AVFilterBufferRefVideoProps *dst, AVFilterBufferRefVideoProps *src)
Definition: libavfilter/buffer.c:42
void avfilter_unref_buffer(AVFilterBufferRef *ref)
Definition: libavfilter/buffer.c:95
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
void ff_avfilter_default_free_buffer(AVFilterBuffer *ptr)
default handler for freeing audio/video buffer when there are no references left
Definition: libavfilter/buffer.c:34
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
void av_dict_copy(AVDictionary **dst, AVDictionary *src, int flags)
Copy entries from one AVDictionary struct into another.
Definition: dict.c:176
void av_free(void *ptr)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc(). ...
Definition: mem.c:183
void av_dict_free(AVDictionary **pm)
Free all the memory allocated for an AVDictionary struct and all keys and values. ...
Definition: dict.c:162
int av_get_channel_layout_nb_channels(uint64_t channel_layout)
Return the number of channels in the channel layout.
Definition: channel_layout.c:191
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
external API header
audio channel layout utility functions
void avfilter_copy_buffer_ref_props(AVFilterBufferRef *dst, AVFilterBufferRef *src)
Definition: libavfilter/buffer.c:148
int format
format of the frame, -1 if unknown or unset Values correspond to enum AVPixelFormat for video frames...
Definition: frame.h:134
AVRational sample_aspect_ratio
Sample aspect ratio for the video frame, 0/1 if unknown/unspecified.
Definition: frame.h:154
AVDictionary * av_frame_get_metadata(const AVFrame *frame)
void * av_malloc(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:73
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
common internal and external API header
int avfilter_copy_frame_props(AVFilterBufferRef *dst, const AVFrame *src)
Definition: libavfilter/buffer.c:118
int top_field_first
If the content is interlaced, is top field displayed first.
Definition: frame.h:275
int64_t av_frame_get_pkt_pos(const AVFrame *frame)
AVFilterBufferRef * avfilter_ref_buffer(AVFilterBufferRef *ref, int pmask)
Definition: libavfilter/buffer.c:51
Definition: avutil.h:143
internal API functions
void avfilter_unref_bufferp(AVFilterBufferRef **ref)
Definition: libavfilter/buffer.c:112
Generated on Mon Nov 18 2024 06:51:52 for FFmpeg by
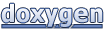