FFmpeg
|
audio.c
Go to the documentation of this file.
47 av_assert0(channels == av_get_channel_layout_nb_channels(link->channel_layout) || !av_get_channel_layout_nb_channels(link->channel_layout));
void * av_mallocz(size_t size)
Allocate a block of size bytes with alignment suitable for all memory accesses (including vectors if ...
Definition: mem.c:205
void av_buffer_unref(AVBufferRef **buf)
Free a given reference and automatically free the buffer if there are no more references to it...
Definition: libavutil/buffer.c:105
int av_samples_get_buffer_size(int *linesize, int nb_channels, int nb_samples, enum AVSampleFormat sample_fmt, int align)
Get the required buffer size for the given audio parameters.
Definition: samplefmt.c:125
external API header
AVBufferRef * buf[AV_NUM_DATA_POINTERS]
AVBuffer references backing the data for this frame.
Definition: frame.h:343
void av_freep(void *arg)
Free a memory block which has been allocated with av_malloc(z)() or av_realloc() and set the pointer ...
Definition: mem.c:198
void ff_avfilter_default_free_buffer(AVFilterBuffer *ptr)
default handler for freeing audio/video buffer when there are no references left
Definition: libavfilter/buffer.c:34
int avcodec_fill_audio_frame(AVFrame *frame, int nb_channels, enum AVSampleFormat sample_fmt, const uint8_t *buf, int buf_size, int align)
Fill AVFrame audio data and linesize pointers.
Definition: libavcodec/utils.c:326
AVFrame * ff_get_audio_buffer(AVFilterLink *link, int nb_samples)
Request an audio samples buffer with a specific set of permissions.
Definition: audio.c:84
int av_get_channel_layout_nb_channels(uint64_t channel_layout)
Return the number of channels in the channel layout.
Definition: channel_layout.c:191
void av_frame_set_channels(AVFrame *frame, int val)
Definition: avutil.h:144
simple assert() macros that are a bit more flexible than ISO C assert().
external API header
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a link
Definition: filter_design.txt:23
audio channel layout utility functions
int av_samples_set_silence(uint8_t **audio_data, int offset, int nb_samples, int nb_channels, enum AVSampleFormat sample_fmt)
Fill an audio buffer with silence.
Definition: samplefmt.c:249
AVFrame * ff_null_get_audio_buffer(AVFilterLink *link, int nb_samples)
get_audio_buffer() handler for filters which simply pass audio along
Definition: audio.c:36
int format
format of the frame, -1 if unknown or unset Values correspond to enum AVPixelFormat for video frames...
Definition: frame.h:134
AVBufferRef * av_buffer_alloc(int size)
Allocate an AVBuffer of the given size using av_malloc().
Definition: libavutil/buffer.c:65
AVFrame *(* get_audio_buffer)(AVFilterLink *link, int nb_samples)
Callback function to get an audio buffer.
Definition: libavfilter/internal.h:84
int av_sample_fmt_is_planar(enum AVSampleFormat sample_fmt)
Check if the sample format is planar.
Definition: samplefmt.c:118
AVFrame * av_frame_alloc(void)
Allocate an AVFrame and set its fields to default values.
Definition: frame.c:95
int avfilter_ref_get_channels(AVFilterBufferRef *ref)
Get the number of channels of a buffer reference.
Definition: audio.c:31
common internal and external API header
AVFrame * ff_default_get_audio_buffer(AVFilterLink *link, int nb_samples)
default handler for get_audio_buffer() for audio inputs
Definition: audio.c:41
void av_frame_free(AVFrame **frame)
Free the frame and any dynamically allocated objects in it, e.g.
Definition: frame.c:108
uint64_t channel_layout
channel layout of current buffer (see libavutil/channel_layout.h)
Definition: avfilter.h:573
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
internal API functions
Generated on Tue Mar 25 2025 06:54:19 for FFmpeg by
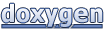