FFmpeg
|
allfilters.c
Go to the documentation of this file.
Definition: af_biquads.c:77
external API header
static void super2xsai(AVFilterContext *ctx, uint8_t *src, int src_linesize, uint8_t *dst, int dst_linesize, int width, int height)
Definition: vf_super2xsai.c:56
Definition: af_biquads.c:72
static void drawtext(AVFrame *pic, int x, int y, int ftid, const uint8_t *color, const char *fmt,...)
Definition: f_ebur128.c:186
#define COPY(condition)
static void copy(LZOContext *c, int cnt)
Copies bytes from input to output buffer with checking.
Definition: lzo.c:79
static int resample(ResampleContext *c, void *dst, const void *src, int *consumed, int src_size, int dst_size, int update_ctx)
Definition: libavresample/resample.c:332
#define transpose(x)
Definition: af_biquads.c:75
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
Definition: af_biquads.c:79
void ff_opencl_register_filter_kernel_code_all(void)
Definition: opencl_allkernels.c:34
Note except for filters that can have queued request_frame does not push and as a the filter_frame method will be called and do the work Legacy the filter_frame method was split
Definition: filter_design.txt:263
Definition: af_biquads.c:80
static int noise(AVBitStreamFilterContext *bsfc, AVCodecContext *avctx, const char *args, uint8_t **poutbuf, int *poutbuf_size, const uint8_t *buf, int buf_size, int keyframe)
Definition: noise_bsf.c:28
Definition: af_biquads.c:74
Definition: af_biquads.c:73
Definition: af_biquads.c:81
#define PAD
Definition: af_biquads.c:78
#define COLOR(x)
Generated on Tue Feb 18 2025 06:56:12 for FFmpeg by
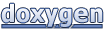