FFmpeg
|
VC-1 and WMV3 decoder. More...
#include "internal.h"
#include "avcodec.h"
#include "error_resilience.h"
#include "mpegvideo.h"
#include "h263.h"
#include "h264chroma.h"
#include "vc1.h"
#include "vc1data.h"
#include "vc1acdata.h"
#include "msmpeg4data.h"
#include "unary.h"
#include "mathops.h"
#include "vdpau_internal.h"
#include "libavutil/avassert.h"
#include <assert.h>
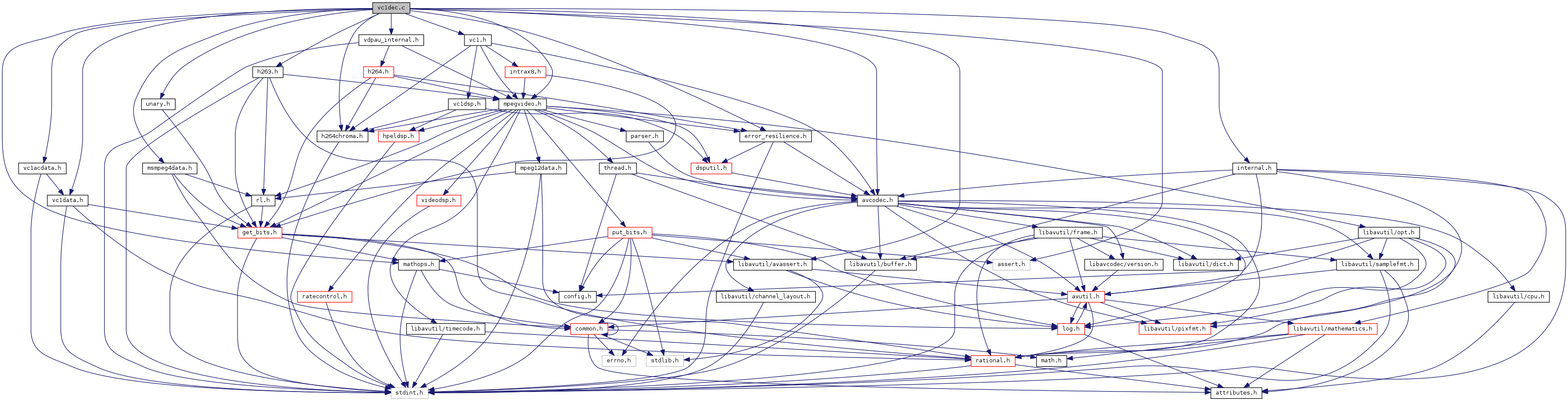
Go to the source code of this file.
Macros | |
#define | MB_INTRA_VLC_BITS 9 |
#define | DC_VLC_BITS 9 |
#define | inc_blk_idx(idx) |
#define | transpose(x) ((x >> 3) | ((x & 7) << 3)) |
Enumerations | |
VC-1 Bitplane decoding | |
| |
enum | Imode { IMODE_RAW, IMODE_NORM2, IMODE_DIFF2, IMODE_NORM6, IMODE_DIFF6, IMODE_ROWSKIP, IMODE_COLSKIP, IMODE_RAW, IMODE_NORM2, IMODE_DIFF2, IMODE_NORM6, IMODE_DIFF6, IMODE_ROWSKIP, IMODE_COLSKIP } |
Imode types. More... | |
Functions | |
static void | init_block_index (VC1Context *v) |
static void | vc1_put_signed_blocks_clamped (VC1Context *v) |
static void | vc1_loop_filter_iblk (VC1Context *v, int pq) |
static void | vc1_loop_filter_iblk_delayed (VC1Context *v, int pq) |
static void | vc1_smooth_overlap_filter_iblk (VC1Context *v) |
static void | vc1_mc_1mv (VC1Context *v, int dir) |
Do motion compensation over 1 macroblock Mostly adapted hpel_motion and qpel_motion from mpegvideo.c. More... | |
static int | median4 (int a, int b, int c, int d) |
static void | vc1_mc_4mv_luma (VC1Context *v, int n, int dir, int avg) |
Do motion compensation for 4-MV macroblock - luminance block. More... | |
static av_always_inline int | get_chroma_mv (int *mvx, int *mvy, int *a, int flag, int *tx, int *ty) |
static void | vc1_mc_4mv_chroma (VC1Context *v, int dir) |
Do motion compensation for 4-MV macroblock - both chroma blocks. More... | |
static void | vc1_mc_4mv_chroma4 (VC1Context *v) |
Do motion compensation for 4-MV field chroma macroblock (both U and V) More... | |
static av_always_inline void | vc1_apply_p_v_loop_filter (VC1Context *v, int block_num) |
static av_always_inline void | vc1_apply_p_h_loop_filter (VC1Context *v, int block_num) |
static void | vc1_apply_p_loop_filter (VC1Context *v) |
static int | vc1_decode_p_mb (VC1Context *v) |
Decode one P-frame MB. More... | |
static int | vc1_decode_p_mb_intfr (VC1Context *v) |
static int | vc1_decode_p_mb_intfi (VC1Context *v) |
static void | vc1_decode_b_mb (VC1Context *v) |
Decode one B-frame MB (in Main profile) More... | |
static void | vc1_decode_b_mb_intfi (VC1Context *v) |
Decode one B-frame MB (in interlaced field B picture) More... | |
static int | vc1_decode_b_mb_intfr (VC1Context *v) |
Decode one B-frame MB (in interlaced frame B picture) More... | |
static void | vc1_decode_i_blocks (VC1Context *v) |
Decode blocks of I-frame. More... | |
static void | vc1_decode_i_blocks_adv (VC1Context *v) |
Decode blocks of I-frame for advanced profile. More... | |
static void | vc1_decode_p_blocks (VC1Context *v) |
static void | vc1_decode_b_blocks (VC1Context *v) |
static void | vc1_decode_skip_blocks (VC1Context *v) |
void | ff_vc1_decode_blocks (VC1Context *v) |
av_cold int | ff_vc1_decode_init_alloc_tables (VC1Context *v) |
av_cold void | ff_vc1_init_transposed_scantables (VC1Context *v) |
static av_cold int | vc1_decode_init (AVCodecContext *avctx) |
Initialize a VC1/WMV3 decoder. More... | |
av_cold int | ff_vc1_decode_end (AVCodecContext *avctx) |
Close a VC1/WMV3 decoder. More... | |
static int | vc1_decode_frame (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt) |
Decode a VC1/WMV3 frame. More... | |
VC1 Macroblock-level functions in Simple/Main Profiles | |
| |
static int | vc1_coded_block_pred (MpegEncContext *s, int n, uint8_t **coded_block_ptr) |
static void | vc1_decode_ac_coeff (VC1Context *v, int *last, int *skip, int *value, int codingset) |
Decode one AC coefficient. More... | |
static int | vc1_decode_i_block (VC1Context *v, int16_t block[64], int n, int coded, int codingset) |
Decode intra block in intra frames - should be faster than decode_intra_block. More... | |
static int | vc1_decode_i_block_adv (VC1Context *v, int16_t block[64], int n, int coded, int codingset, int mquant) |
Decode intra block in intra frames - should be faster than decode_intra_block. More... | |
static int | vc1_decode_intra_block (VC1Context *v, int16_t block[64], int n, int coded, int mquant, int codingset) |
Decode intra block in inter frames - more generic version than vc1_decode_i_block. More... | |
static int | vc1_decode_p_block (VC1Context *v, int16_t block[64], int n, int mquant, int ttmb, int first_block, uint8_t *dst, int linesize, int skip_block, int *ttmb_out) |
Decode P block. More... | |
Variables | |
static const int | offset_table1 [9] = { 0, 1, 2, 4, 8, 16, 32, 64, 128 } |
static const int | offset_table2 [9] = { 0, 1, 3, 7, 15, 31, 63, 127, 255 } |
static const int | size_table [6] = { 0, 2, 3, 4, 5, 8 } |
static const int | offset_table [6] = { 0, 1, 3, 7, 15, 31 } |
static const AVProfile | profiles [] |
static enum AVPixelFormat | vc1_hwaccel_pixfmt_list_420 [] |
AVCodec | ff_vc1_decoder |
VC-1 Block-level functions | |
| |
#define | GET_MQUANT() |
Get macroblock-level quantizer scale. More... | |
#define | GET_MVDATA(_dmv_x, _dmv_y) |
Get MV differentials. More... | |
static av_always_inline void | get_mvdata_interlaced (VC1Context *v, int *dmv_x, int *dmv_y, int *pred_flag) |
static av_always_inline int | scaleforsame_x (VC1Context *v, int n, int dir) |
static av_always_inline int | scaleforsame_y (VC1Context *v, int i, int n, int dir) |
static av_always_inline int | scaleforopp_x (VC1Context *v, int n) |
static av_always_inline int | scaleforopp_y (VC1Context *v, int n, int dir) |
static av_always_inline int | scaleforsame (VC1Context *v, int i, int n, int dim, int dir) |
static av_always_inline int | scaleforopp (VC1Context *v, int n, int dim, int dir) |
static void | vc1_pred_mv (VC1Context *v, int n, int dmv_x, int dmv_y, int mv1, int r_x, int r_y, uint8_t *is_intra, int pred_flag, int dir) |
Predict and set motion vector. More... | |
static void | vc1_pred_mv_intfr (VC1Context *v, int n, int dmv_x, int dmv_y, int mvn, int r_x, int r_y, uint8_t *is_intra, int dir) |
Predict and set motion vector for interlaced frame picture MBs. More... | |
static void | vc1_interp_mc (VC1Context *v) |
Motion compensation for direct or interpolated blocks in B-frames. More... | |
static av_always_inline int | scale_mv (int value, int bfrac, int inv, int qs) |
static void | vc1_b_mc (VC1Context *v, int dmv_x[2], int dmv_y[2], int direct, int mode) |
Reconstruct motion vector for B-frame and do motion compensation. More... | |
static void | vc1_pred_b_mv (VC1Context *v, int dmv_x[2], int dmv_y[2], int direct, int mvtype) |
static void | vc1_pred_b_mv_intfi (VC1Context *v, int n, int *dmv_x, int *dmv_y, int mv1, int *pred_flag) |
static int | vc1_i_pred_dc (MpegEncContext *s, int overlap, int pq, int n, int16_t **dc_val_ptr, int *dir_ptr) |
Get predicted DC value for I-frames only prediction dir: left=0, top=1. More... | |
static int | vc1_pred_dc (MpegEncContext *s, int overlap, int pq, int n, int a_avail, int c_avail, int16_t **dc_val_ptr, int *dir_ptr) |
Get predicted DC value prediction dir: left=0, top=1. More... | |
Detailed Description
VC-1 and WMV3 decoder.
Definition in file vc1dec.c.
Macro Definition Documentation
#define DC_VLC_BITS 9 |
Definition at line 48 of file vc1dec.c.
Referenced by vc1_decode_i_block(), vc1_decode_i_block_adv(), and vc1_decode_intra_block().
#define GET_MQUANT | ( | ) |
Get macroblock-level quantizer scale.
Definition at line 1055 of file vc1dec.c.
Referenced by vc1_decode_b_mb(), vc1_decode_b_mb_intfi(), vc1_decode_b_mb_intfr(), vc1_decode_i_blocks_adv(), vc1_decode_p_mb(), vc1_decode_p_mb_intfi(), and vc1_decode_p_mb_intfr().
#define GET_MVDATA | ( | _dmv_x, | |
_dmv_y | |||
) |
Get MV differentials.
- See also
- MVDATA decoding from 8.3.5.2, p(1)20
- Parameters
-
_dmv_x Horizontal differential for decoded MV _dmv_y Vertical differential for decoded MV
Definition at line 1097 of file vc1dec.c.
Referenced by vc1_decode_b_mb(), and vc1_decode_p_mb().
#define inc_blk_idx | ( | idx | ) |
Referenced by vc1_put_signed_blocks_clamped().
#define MB_INTRA_VLC_BITS 9 |
Definition at line 47 of file vc1dec.c.
Referenced by vc1_decode_i_blocks(), and vc1_decode_i_blocks_adv().
Referenced by avfilter_register_all(), and ff_vc1_init_transposed_scantables().
Enumeration Type Documentation
enum Imode |
Function Documentation
void ff_vc1_decode_blocks | ( | VC1Context * | v | ) |
Definition at line 5124 of file vc1dec.c.
Referenced by decode_wmv9(), vc1_decode_frame(), and vc1_unescape_buffer().
av_cold int ff_vc1_decode_end | ( | AVCodecContext * | avctx | ) |
Close a VC1/WMV3 decoder.
- Warning
- Initial try at using MpegEncContext stuff
Definition at line 5663 of file vc1dec.c.
Referenced by mss2_decode_end(), vc1_decode_frame(), vc1_decode_init(), and vc1_unescape_buffer().
av_cold int ff_vc1_decode_init_alloc_tables | ( | VC1Context * | v | ) |
Definition at line 5433 of file vc1dec.c.
Referenced by vc1_decode_frame(), vc1_decode_init(), vc1_unescape_buffer(), and wmv9_init().
av_cold void ff_vc1_init_transposed_scantables | ( | VC1Context * | v | ) |
Definition at line 5499 of file vc1dec.c.
Referenced by vc1_decode_init(), vc1_unescape_buffer(), and wmv9_init().
|
static |
Definition at line 739 of file vc1dec.c.
Referenced by vc1_mc_4mv_chroma().
|
static |
Definition at line 1135 of file vc1dec.c.
Referenced by vc1_decode_b_mb_intfi(), vc1_decode_b_mb_intfr(), vc1_decode_p_mb_intfi(), and vc1_decode_p_mb_intfr().
|
static |
Definition at line 77 of file vc1dec.c.
Referenced by vc1_decode_b_blocks(), vc1_decode_i_blocks(), vc1_decode_i_blocks_adv(), vc1_decode_p_blocks(), and vc1_decode_skip_blocks().
|
inlinestatic |
Definition at line 546 of file vc1dec.c.
Referenced by get_chroma_mv(), and vc1_mc_4mv_luma().
|
static |
Definition at line 1997 of file vc1dec.c.
Referenced by vc1_decode_b_mb_intfr(), vc1_pred_b_mv(), and vc1_pred_b_mv_intfi().
|
static |
Definition at line 1354 of file vc1dec.c.
Referenced by vc1_pred_mv().
|
static |
Definition at line 1275 of file vc1dec.c.
Referenced by scaleforopp().
|
static |
Definition at line 1302 of file vc1dec.c.
Referenced by scaleforopp().
|
static |
Definition at line 1333 of file vc1dec.c.
Referenced by vc1_pred_mv().
|
static |
Definition at line 1205 of file vc1dec.c.
Referenced by scaleforsame().
|
static |
Definition at line 1238 of file vc1dec.c.
Referenced by scaleforsame().
|
static |
Definition at line 3381 of file vc1dec.c.
Referenced by vc1_apply_p_loop_filter().
|
static |
Definition at line 3441 of file vc1dec.c.
Referenced by vc1_decode_p_blocks().
|
static |
Definition at line 3317 of file vc1dec.c.
Referenced by vc1_apply_p_loop_filter().
|
inlinestatic |
Reconstruct motion vector for B-frame and do motion compensation.
Definition at line 2018 of file vc1dec.c.
Referenced by vc1_decode_b_mb(), and vc1_decode_b_mb_intfi().
|
inlinestatic |
Definition at line 2468 of file vc1dec.c.
Referenced by vc1_decode_i_blocks(), and vc1_decode_i_blocks_adv().
|
static |
Decode one AC coefficient.
- Parameters
-
v The VC1 context last Last coefficient skip How much zero coefficients to skip value Decoded AC coefficient value codingset set of VLC to decode data
- See also
- 8.1.3.4
Definition at line 2504 of file vc1dec.c.
Referenced by vc1_decode_i_block(), vc1_decode_i_block_adv(), vc1_decode_intra_block(), and vc1_decode_p_block().
|
static |
Definition at line 5040 of file vc1dec.c.
Referenced by ff_vc1_decode_blocks().
|
static |
Decode one B-frame MB (in Main profile)
Definition at line 4037 of file vc1dec.c.
Referenced by vc1_decode_b_blocks().
|
static |
Decode one B-frame MB (in interlaced field B picture)
Definition at line 4189 of file vc1dec.c.
Referenced by vc1_decode_b_blocks().
|
static |
Decode one B-frame MB (in interlaced frame B picture)
Definition at line 4342 of file vc1dec.c.
Referenced by vc1_decode_b_blocks().
|
static |
|
static |
Decode intra block in intra frames - should be faster than decode_intra_block.
- Parameters
-
v VC1Context block block to decode [in] n subblock index coded are AC coeffs present or not codingset set of VLC to decode data
Definition at line 2570 of file vc1dec.c.
Referenced by vc1_decode_i_blocks().
|
static |
Decode intra block in intra frames - should be faster than decode_intra_block.
- Parameters
-
v VC1Context block block to decode [in] n subblock number coded are AC coeffs present or not codingset set of VLC to decode data mquant quantizer value for this macroblock
Definition at line 2733 of file vc1dec.c.
Referenced by vc1_decode_i_blocks_adv().
|
static |
Decode blocks of I-frame.
Definition at line 4691 of file vc1dec.c.
Referenced by ff_vc1_decode_blocks().
|
static |
Decode blocks of I-frame for advanced profile.
Definition at line 4831 of file vc1dec.c.
Referenced by ff_vc1_decode_blocks().
|
static |
|
static |
Decode intra block in inter frames - more generic version than vc1_decode_i_block.
- Parameters
-
v VC1Context block block to decode [in] n subblock index coded are AC coeffs present or not mquant block quantizer codingset set of VLC to decode data
Definition at line 2945 of file vc1dec.c.
Referenced by vc1_decode_b_mb(), vc1_decode_b_mb_intfi(), vc1_decode_b_mb_intfr(), vc1_decode_p_mb(), vc1_decode_p_mb_intfi(), and vc1_decode_p_mb_intfr().
|
static |
Decode P block.
Definition at line 3155 of file vc1dec.c.
Referenced by vc1_decode_b_mb(), vc1_decode_b_mb_intfi(), vc1_decode_b_mb_intfr(), vc1_decode_p_mb(), vc1_decode_p_mb_intfi(), and vc1_decode_p_mb_intfr().
|
static |
Definition at line 4965 of file vc1dec.c.
Referenced by ff_vc1_decode_blocks().
|
static |
Decode one P-frame MB.
Definition at line 3468 of file vc1dec.c.
Referenced by vc1_decode_p_blocks().
|
static |
Definition at line 3918 of file vc1dec.c.
Referenced by vc1_decode_p_blocks().
|
static |
Definition at line 3707 of file vc1dec.c.
Referenced by vc1_decode_p_blocks().
|
static |
Definition at line 5103 of file vc1dec.c.
Referenced by ff_vc1_decode_blocks().
|
inlinestatic |
Get predicted DC value for I-frames only prediction dir: left=0, top=1.
- Parameters
-
s MpegEncContext overlap flag indicating that overlap filtering is used pq integer part of picture quantizer [in] n block index in the current MB dc_val_ptr Pointer to DC predictor dir_ptr Prediction direction for use in AC prediction
Definition at line 2326 of file vc1dec.c.
Referenced by vc1_decode_i_block().
|
static |
Motion compensation for direct or interpolated blocks in B-frames.
Definition at line 1861 of file vc1dec.c.
Referenced by vc1_b_mc(), and vc1_decode_b_mb_intfr().
|
static |
Definition at line 170 of file vc1dec.c.
Referenced by vc1_decode_b_blocks(), and vc1_decode_i_blocks().
|
static |
Definition at line 197 of file vc1dec.c.
Referenced by vc1_decode_i_blocks_adv().
|
static |
Do motion compensation over 1 macroblock Mostly adapted hpel_motion and qpel_motion from mpegvideo.c.
Definition at line 345 of file vc1dec.c.
Referenced by vc1_b_mc(), vc1_decode_b_mb_intfr(), vc1_decode_p_mb(), vc1_decode_p_mb_intfi(), and vc1_decode_p_mb_intfr().
|
static |
Do motion compensation for 4-MV macroblock - both chroma blocks.
Definition at line 794 of file vc1dec.c.
Referenced by vc1_decode_b_mb_intfi(), vc1_decode_p_mb(), and vc1_decode_p_mb_intfi().
|
static |
Do motion compensation for 4-MV field chroma macroblock (both U and V)
Definition at line 959 of file vc1dec.c.
Referenced by vc1_decode_b_mb_intfr(), and vc1_decode_p_mb_intfr().
|
static |
Do motion compensation for 4-MV macroblock - luminance block.
Definition at line 559 of file vc1dec.c.
Referenced by vc1_decode_b_mb_intfi(), vc1_decode_b_mb_intfr(), vc1_decode_p_mb(), vc1_decode_p_mb_intfi(), and vc1_decode_p_mb_intfr().
|
inlinestatic |
Definition at line 2047 of file vc1dec.c.
Referenced by vc1_decode_b_mb().
|
inlinestatic |
Definition at line 2260 of file vc1dec.c.
Referenced by vc1_decode_b_mb_intfi().
|
inlinestatic |
Get predicted DC value prediction dir: left=0, top=1.
- Parameters
-
s MpegEncContext overlap flag indicating that overlap filtering is used pq integer part of picture quantizer [in] n block index in the current MB a_avail flag indicating top block availability c_avail flag indicating left block availability dc_val_ptr Pointer to DC predictor dir_ptr Prediction direction for use in AC prediction
Definition at line 2391 of file vc1dec.c.
Referenced by vc1_decode_i_block_adv(), and vc1_decode_intra_block().
|
inlinestatic |
Predict and set motion vector.
Definition at line 1380 of file vc1dec.c.
Referenced by vc1_decode_p_mb(), vc1_decode_p_mb_intfi(), and vc1_pred_b_mv_intfi().
|
inlinestatic |
Predict and set motion vector for interlaced frame picture MBs.
Definition at line 1634 of file vc1dec.c.
Referenced by vc1_decode_b_mb_intfr(), and vc1_decode_p_mb_intfr().
|
static |
Definition at line 91 of file vc1dec.c.
Referenced by vc1_decode_i_blocks_adv().
|
static |
Definition at line 265 of file vc1dec.c.
Referenced by vc1_decode_i_blocks_adv().
Variable Documentation
AVCodec ff_vc1_decoder |
|
static |
Definition at line 3315 of file vc1dec.c.
Referenced by escape130_decode_frame(), and seq_parse_frame_data().
|
static |
Definition at line 52 of file vc1dec.c.
Referenced by get_mvdata_interlaced().
|
static |
Definition at line 53 of file vc1dec.c.
Referenced by get_mvdata_interlaced().
|
static |
|
static |
Generated on Wed Jul 2 2025 06:53:47 for FFmpeg by
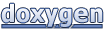