FFmpeg
|
h263.h
Go to the documentation of this file.
115 const uint8_t *ff_h263_find_resync_marker(MpegEncContext *s, const uint8_t *p, const uint8_t *end);
void ff_h263_encode_picture_header(MpegEncContext *s, int picture_number)
Definition: ituh263enc.c:101
int ff_h263_pred_dc(MpegEncContext *s, int n, int16_t **dc_val_ptr)
Definition: h263.c:100
static void skip_put_bits(PutBitContext *s, int n)
Skip the given number of bits.
Definition: put_bits.h:220
mpegvideo header.
int ff_h263_decode_mb(MpegEncContext *s, int16_t block[6][64])
Definition: ituh263dec.c:605
const uint8_t * ff_h263_find_resync_marker(MpegEncContext *s, const uint8_t *p, const uint8_t *end)
int ff_h263_get_gob_height(MpegEncContext *s)
Get the GOB height based on picture height.
Definition: h263.c:377
int ff_intel_h263_decode_picture_header(MpegEncContext *s)
Definition: intelh263dec.c:25
void ff_init_qscale_tab(MpegEncContext *s)
init s->current_picture.qscale_table from s->lambda_table
Definition: mpegvideo_enc.c:177
bitstream reader API header.
uint8_t ff_h263_static_rl_table_store[2][2][2 *MAX_RUN+MAX_LEVEL+3]
Definition: h263.c:45
av_const int ff_h263_aspect_to_info(AVRational aspect)
Return the 4 bit value that specifies the given aspect ratio.
Definition: ituh263enc.c:87
rl header.
Definition: get_bits.h:63
void ff_clean_h263_qscales(MpegEncContext *s)
modify qscale so that encoding is actually possible in h263 (limit difference to -2..2)
Definition: ituh263enc.c:272
int ff_h263_decode_init(AVCodecContext *avctx)
Definition: libavcodec/h263dec.c:47
void ff_h263_encode_motion(MpegEncContext *s, int val, int f_code)
Definition: ituh263enc.c:654
int ff_h263_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: libavcodec/h263dec.c:348
enum AVPixelFormat ff_h263_hwaccel_pixfmt_list_420[]
Definition: libavcodec/h263dec.c:776
void ff_h263_pred_acdc(MpegEncContext *s, int16_t *block, int n)
Definition: h263.c:228
int av_const h263_get_picture_format(int width, int height)
Return the value of the 3bit "source format" syntax element.
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
void ff_h263_encode_gob_header(MpegEncContext *s, int mb_line)
Encode a group of blocks header.
Definition: ituh263enc.c:246
static int h263_get_motion_length(MpegEncContext *s, int val, int f_code)
Definition: h263.h:120
static void ff_h263_encode_motion_vector(MpegEncContext *s, int x, int y, int f_code)
Definition: h263.h:137
int ff_h263_decode_picture_header(MpegEncContext *s)
Definition: ituh263dec.c:871
int ff_h263_resync(MpegEncContext *s)
Decode the group of blocks / video packet header.
Definition: ituh263dec.c:231
int16_t * ff_h263_pred_motion(MpegEncContext *s, int block, int dir, int *px, int *py)
Definition: h263.c:315
rational numbers
void ff_h263_encode_mb(MpegEncContext *s, int16_t block[6][64], int motion_x, int motion_y)
Definition: ituh263enc.c:453
static int get_p_cbp(MpegEncContext *s, int16_t block[6][64], int motion_x, int motion_y)
Definition: h263.h:148
int ff_h263_decode_gob_header(MpegEncContext *s)
int ff_h263_decode_motion(MpegEncContext *s, int pred, int f_code)
Definition: ituh263dec.c:273
void ff_h263_show_pict_info(MpegEncContext *s)
Print picture info if FF_DEBUG_PICT_INFO is set.
Definition: ituh263dec.c:71
Generated on Wed May 8 2024 06:52:06 for FFmpeg by
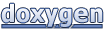