FFmpeg
|
ituh263dec.c
Go to the documentation of this file.
209 const uint8_t *ff_h263_find_resync_marker(MpegEncContext *s, const uint8_t *av_restrict p, const uint8_t *av_restrict end)
1141 if (s->pict_type == AV_PICTURE_TYPE_I && s->codec_tag == AV_RL32("ZYGO") && get_bits_left(&s->gb) >= 85 + 13*3*16 + 50){
static int h263_decode_gob_header(MpegEncContext *s)
Decode the group of blocks header or slice header.
Definition: ituh263dec.c:152
void ff_init_rl(RLTable *rl, uint8_t static_store[2][2 *MAX_RUN+MAX_LEVEL+3])
Definition: mpegvideo.c:1304
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
static int h263_decode_block(MpegEncContext *s, int16_t *block, int n, int coded)
Definition: ituh263dec.c:442
Definition: libavcodec/avcodec.h:115
mpegvideo header.
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
int16_t * ff_h263_pred_motion(MpegEncContext *s, int block, int dir, int *px, int *py)
Definition: h263.c:315
#define USES_LIST(a, list)
does this mb use listX, note does not work if subMBs
Definition: mpegvideo.h:156
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
int mb_num_left
number of MBs left in this video packet (for partitioned Slices only)
Definition: mpegvideo.h:530
#define MV_DIRECT
bidirectional mode where the difference equals the MV of the last P/S/I-Frame (mpeg4) ...
Definition: mpegvideo.h:419
#define av_assert2(cond)
assert() equivalent, that does lie in speed critical code.
Definition: avassert.h:63
#define INIT_VLC_STATIC(vlc, bits, a, b, c, d, e, f, g, static_size)
Definition: get_bits.h:445
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
int no_rounding
apply no rounding to motion compensation (MPEG4, msmpeg4, ...) for b-frames rounding mode is always 0...
Definition: mpegvideo.h:439
char av_get_picture_type_char(enum AVPictureType pict_type)
Return a single letter to describe the given picture type pict_type.
Definition: libavutil/utils.c:76
int codec_tag
internal codec_tag upper case converted from avctx codec_tag
Definition: mpegvideo.h:267
void ff_set_qscale(MpegEncContext *s, int qscale)
set qscale and update qscale dependent variables.
Definition: mpegvideo.c:3300
int ff_h263_decode_motion(MpegEncContext *s, int pred, int f_code)
Definition: ituh263dec.c:273
int64_t av_gcd(int64_t a, int64_t b)
Return the greatest common divisor of a and b.
Definition: mathematics.c:55
static int h263_get_modb(GetBitContext *gb, int pb_frame, int *cbpb)
Definition: ituh263dec.c:587
external API header
Definition: get_bits.h:63
common internal API header
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
Definition: libavcodec/avcodec.h:108
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
static int h263p_decode_umotion(MpegEncContext *s, int pred)
Definition: ituh263dec.c:311
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
uint8_t ff_h263_static_rl_table_store[2][2][2 *MAX_RUN+MAX_LEVEL+3]
Definition: h263.c:45
int ff_h263_resync(MpegEncContext *s)
Decode the group of blocks / video packet header.
Definition: ituh263dec.c:231
int ff_mpeg4_set_direct_mv(MpegEncContext *s, int mx, int my)
Definition: mpeg4video.c:121
int ff_h263_decode_picture_header(MpegEncContext *s)
Definition: ituh263dec.c:871
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
int ff_mpeg4_get_video_packet_prefix_length(MpegEncContext *s)
Definition: mpeg4video.c:29
int mv[2][4][2]
motion vectors for a macroblock first coordinate : 0 = forward 1 = backward second " : depend...
Definition: mpegvideo.h:431
int b8_stride
2*mb_width+1 used for some 8x8 block arrays to allow simple addressing
Definition: mpegvideo.h:279
Definition: get_bits.h:54
int mb_stride
mb_width+1 used for some arrays to allow simple addressing of left & top MBs without sig11 ...
Definition: mpegvideo.h:278
void ff_h263_pred_acdc(MpegEncContext *s, int16_t *block, int n)
Definition: h263.c:228
static int get_unary(GetBitContext *gb, int stop, int len)
Get unary code of limited length.
Definition: unary.h:33
void ff_h263_show_pict_info(MpegEncContext *s)
Print picture info if FF_DEBUG_PICT_INFO is set.
Definition: ituh263dec.c:71
int ff_mpeg4_decode_video_packet_header(MpegEncContext *s)
Decode the next video packet.
Definition: mpeg4videodec.c:363
void ff_flv2_decode_ac_esc(GetBitContext *gb, int *level, int *run, int *last)
Definition: libavcodec/flvdec.c:25
const uint8_t * ff_h263_find_resync_marker(MpegEncContext *s, const uint8_t *av_restrict p, const uint8_t *av_restrict end)
Find the next resync_marker.
Definition: ituh263dec.c:209
int ff_h263_decode_mb(MpegEncContext *s, int16_t block[6][64])
Definition: ituh263dec.c:605
Generated on Thu May 29 2025 06:52:49 for FFmpeg by
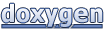