FFmpeg
|
mpeg4videodec.c
Go to the documentation of this file.
171 const int vop_ref[4][2]= {{0,0}, {s->width,0}, {0, s->height}, {s->width, s->height}}; // only true for rectangle shapes
206 while((1<<beta )<h) beta++; // there seems to be a typo in the mpeg4 std for the definition of w' and h'
231 // the idea behind this virtual_ref mess is to be able to use shifts later per pixel instead of divides
232 // so the distance between points is converted from w&h based to w2&h2 based which are of the 2^x form
234 + ROUNDED_DIV(((w - w2)*(r*sprite_ref[0][0] - 16*vop_ref[0][0]) + w2*(r*sprite_ref[1][0] - 16*vop_ref[1][0])),w);
236 + ROUNDED_DIV(((w - w2)*(r*sprite_ref[0][1] - 16*vop_ref[0][1]) + w2*(r*sprite_ref[1][1] - 16*vop_ref[1][1])),w);
238 + ROUNDED_DIV(((h - h2)*(r*sprite_ref[0][0] - 16*vop_ref[0][0]) + h2*(r*sprite_ref[2][0] - 16*vop_ref[2][0])),h);
240 + ROUNDED_DIV(((h - h2)*(r*sprite_ref[0][1] - 16*vop_ref[0][1]) + h2*(r*sprite_ref[2][1] - 16*vop_ref[2][1])),h);
798 const int part_a_error= s->pict_type==AV_PICTURE_TYPE_I ? (ER_DC_ERROR|ER_MV_ERROR) : ER_MV_ERROR;
819 av_log(s->avctx, AV_LOG_ERROR, "marker missing after first I partition at %d %d\n", s->mb_x, s->mb_y);
826 av_log(s->avctx, AV_LOG_ERROR, "marker missing after first P partition at %d %d\n", s->mb_x, s->mb_y);
1152 av_log(s->avctx, AV_LOG_ERROR, "texture corrupted at %d %d %d\n", s->mb_x, s->mb_y, s->mb_intra);
1322 s->mb_skipped = s->next_picture.mbskip_table[s->mb_y * s->mb_stride + s->mb_x]; // Note, skiptab=0 if last was GMC
1341 mb_type= MB_TYPE_DIRECT2 | MB_TYPE_SKIP | MB_TYPE_L0L1; //like MB_TYPE_B_DIRECT but no vectors coded
1498 if (s->mb_x + s->mb_y*s->mb_width + 1 > next && (s->avctx->err_recognition & AV_EF_AGGRESSIVE)) {
1634 if(width && height && !(s->width && s->codec_tag == AV_RL32("MP4S"))){ /* they should be non zero but who knows ... */
1647 av_log(s->avctx, AV_LOG_INFO, "MPEG4 OBMC not supported (very likely buggy encoder)\n"); /* OBMC Disable */
1653 if(s->vol_sprite_usage==STATIC_SPRITE) av_log(s->avctx, AV_LOG_ERROR, "Static Sprites not supported\n");
1680 if(get_bits(gb, 4)!=8) av_log(s->avctx, AV_LOG_ERROR, "N-bit not supported\n"); /* bits_per_pixel */
1681 if(s->quant_precision!=5) av_log(s->avctx, AV_LOG_ERROR, "quant precision %d\n", s->quant_precision);
1802 av_log(s->avctx, AV_LOG_ERROR, "Invalid Complexity estimation method %d\n", estimation_method);
1825 if(s->reduced_res_vop) av_log(s->avctx, AV_LOG_ERROR, "reduced resolution VOP not supported\n");
1903 av_log(s->avctx, AV_LOG_WARNING, "Invalid and inefficient vfw-avi packed B frames detected\n");
1939 if(s->pict_type==AV_PICTURE_TYPE_B && s->low_delay && s->vol_control_parameters==0 && !(s->flags & CODEC_FLAG_LOW_DELAY)){
1957 av_log(s->avctx, AV_LOG_ERROR, "hmm, seems the headers are not complete, trying to guess time_increment_bits\n");
2081 ff_init_scantable(s->dsp.idct_permutation, &s->intra_h_scantable, ff_alternate_horizontal_scan);
2085 if(s->pict_type == AV_PICTURE_TYPE_S && (s->vol_sprite_usage==STATIC_SPRITE || s->vol_sprite_usage==GMC_SPRITE)){
2088 if(s->sprite_brightness_change) av_log(s->avctx, AV_LOG_ERROR, "sprite_brightness_change not supported\n");
2089 if(s->vol_sprite_usage==STATIC_SPRITE) av_log(s->avctx, AV_LOG_ERROR, "static sprite not supported\n");
2120 av_log(s->avctx, AV_LOG_DEBUG, "qp:%d fc:%d,%d %s size:%d pro:%d alt:%d top:%d %spel part:%d resync:%d w:%d a:%d rnd:%d vot:%d%s dc:%d ce:%d/%d/%d time:%"PRId64" tincr:%d\n",
2122 s->pict_type == AV_PICTURE_TYPE_I ? "I" : (s->pict_type == AV_PICTURE_TYPE_P ? "P" : (s->pict_type == AV_PICTURE_TYPE_B ? "B" : "S")),
2124 s->quarter_sample ? "q" : "h", s->data_partitioning, s->resync_marker, s->num_sprite_warping_points,
2125 s->sprite_warping_accuracy, 1-s->no_rounding, s->vo_type, s->vol_control_parameters ? " VOLC" : " ", s->intra_dc_threshold,
2148 if(s->vo_type==0 && s->vol_control_parameters==0 && s->divx_version==-1 && s->picture_number==0){
2149 av_log(s->avctx, AV_LOG_WARNING, "looks like this file was encoded with (divx4/(old)xvid/opendivx) -> forcing low_delay flag\n");
2187 if(gb->size_in_bits==8 && (s->divx_version>=0 || s->xvid_build>=0) || s->codec_tag == AV_RL32("QMP4")){
2333 {"quarter_sample", "1/4 subpel MC", offsetof(MpegEncContext, quarter_sample), FF_OPT_TYPE_INT, {.i64 = 0}, 0, 1, 0},
2334 {"divx_packed", "divx style packed b frames", offsetof(MpegEncContext, divx_packed), FF_OPT_TYPE_INT, {.i64 = 0}, 0, 1, 0},
#define FF_PROFILE_MPEG4_SCALABLE_TEXTURE
Definition: libavcodec/avcodec.h:2710
static unsigned int show_bits_long(GetBitContext *s, int n)
Show 0-32 bits.
Definition: get_bits.h:352
Definition: get_bits.h:69
static int decode_vop_header(MpegEncContext *s, GetBitContext *gb)
Definition: mpeg4videodec.c:1935
int time_increment_bits
number of bits to represent the fractional part of time
Definition: mpegvideo.h:555
#define FF_PROFILE_MPEG4_ADVANCED_CORE
Definition: libavcodec/avcodec.h:2717
Definition: pixfmt.h:67
int vol_control_parameters
does the stream contain the low_delay flag, used to workaround buggy encoders
Definition: mpegvideo.h:594
int last_mv[2][2][2]
last MV, used for MV prediction in MPEG1 & B-frame MPEG4
Definition: mpegvideo.h:433
av_cold void ff_mpeg4videodec_static_init(void)
Definition: mpeg4videodec.c:2260
static int mpeg4_is_resync(MpegEncContext *s)
check if the next stuff is a resync marker or the end.
Definition: mpeg4videodec.c:117
static int mpeg4_decode_gop_header(MpegEncContext *s, GetBitContext *gb)
Definition: mpeg4videodec.c:1519
int16_t(*[3] ac_val)[16]
used for for mpeg4 AC prediction, all 3 arrays must be continuous
Definition: mpegvideo.h:357
int v_edge_pos
horizontal / vertical position of the right/bottom edge (pixel replication)
Definition: mpegvideo.h:281
#define FF_PROFILE_MPEG4_ADVANCED_SIMPLE
Definition: libavcodec/avcodec.h:2720
void ff_init_rl(RLTable *rl, uint8_t static_store[2][2 *MAX_RUN+MAX_LEVEL+3])
Definition: mpegvideo.c:1304
void ff_mpeg4_pred_ac(MpegEncContext *s, int16_t *block, int n, int dir)
Predict the ac.
Definition: mpeg4videodec.c:58
AVRational sample_aspect_ratio
sample aspect ratio (0 if unknown) That is the width of a pixel divided by the height of the pixel...
Definition: libavcodec/avcodec.h:1505
static int decode_vol_header(MpegEncContext *s, GetBitContext *gb)
Definition: mpeg4videodec.c:1553
Definition: libavcodec/avcodec.h:115
int ff_mpeg4_decode_partitions(MpegEncContext *s)
Decode the first and second partition.
Definition: mpeg4videodec.c:795
mpegvideo header.
the pkt_dts and pkt_pts fields in AVFrame will work as usual Restrictions on codec whose streams don t reset across will not work because their bitstreams cannot be decoded in parallel *The contents of buffers must not be read before ff_thread_await_progress() has been called on them.reget_buffer() and buffer age optimizations no longer work.*The contents of buffers must not be written to after ff_thread_report_progress() has been called on them.This includes draw_edges().Porting codecs to frame threading
int16_t * ff_h263_pred_motion(MpegEncContext *s, int block, int dir, int *px, int *py)
Definition: h263.c:315
#define USES_LIST(a, list)
does this mb use listX, note does not work if subMBs
Definition: mpegvideo.h:156
void ff_clean_intra_table_entries(MpegEncContext *s)
Clean dc, ac, coded_block for the current non-intra MB.
Definition: mpegvideo.c:2625
AVRational time_base
This is the fundamental unit of time (in seconds) in terms of which frame timestamps are represented...
Definition: libavcodec/avcodec.h:1253
int intra_dc_threshold
QP above whch the ac VLC should be used for intra dc.
Definition: mpegvideo.h:595
int mb_num_left
number of MBs left in this video packet (for partitioned Slices only)
Definition: mpegvideo.h:530
#define MV_DIRECT
bidirectional mode where the difference equals the MV of the last P/S/I-Frame (mpeg4) ...
Definition: mpegvideo.h:419
#define av_assert2(cond)
assert() equivalent, that does lie in speed critical code.
Definition: avassert.h:63
static int mpeg4_decode_mb(MpegEncContext *s, int16_t block[6][64])
Definition: mpeg4videodec.c:1176
AVOptions.
#define INIT_VLC_STATIC(vlc, bits, a, b, c, d, e, f, g, static_size)
Definition: get_bits.h:445
#define CODEC_CAP_HWACCEL_VDPAU
Codec can export data for HW decoding (VDPAU).
Definition: libavcodec/avcodec.h:779
int64_t pts
Presentation timestamp in time_base units (time when frame should be shown to user).
Definition: frame.h:159
int no_rounding
apply no rounding to motion compensation (MPEG4, msmpeg4, ...) for b-frames rounding mode is always 0...
Definition: mpegvideo.h:439
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
int showed_packed_warning
flag for having shown the warning about divxs invalid b frames
Definition: mpegvideo.h:539
int codec_tag
internal codec_tag upper case converted from avctx codec_tag
Definition: mpegvideo.h:267
enum AVChromaLocation chroma_sample_location
This defines the location of chroma samples.
Definition: libavcodec/avcodec.h:1843
#define FF_PROFILE_MPEG4_BASIC_ANIMATED_TEXTURE
Definition: libavcodec/avcodec.h:2712
static int ff_mpeg4_pred_dc(MpegEncContext *s, int n, int level, int *dir_ptr, int encoding)
Predict the dc.
Definition: mpeg4video.h:129
static void ff_update_block_index(MpegEncContext *s)
Definition: mpegvideo.h:861
void ff_set_qscale(MpegEncContext *s, int qscale)
set qscale and update qscale dependent variables.
Definition: mpegvideo.c:3300
static int mpeg4_decode_sprite_trajectory(MpegEncContext *s, GetBitContext *gb)
Definition: mpeg4videodec.c:165
int16_t * dc_val[3]
used for mpeg4 DC prediction, all 3 arrays must be continuous
Definition: mpegvideo.h:350
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
void ff_er_add_slice(ERContext *s, int startx, int starty, int endx, int endy, int status)
Add a slice.
Definition: error_resilience.c:772
Multithreading support functions.
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
Definition: opt.h:237
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
#define FF_PROFILE_MPEG4_SIMPLE_SCALABLE
Definition: libavcodec/avcodec.h:2706
#define FF_PROFILE_MPEG4_ADVANCED_REAL_TIME
Definition: libavcodec/avcodec.h:2714
#define GET_RL_VLC(level, run, name, gb, table, bits,max_depth, need_update)
Definition: get_bits.h:490
Definition: get_bits.h:63
#define ONLY_IF_THREADS_ENABLED(x)
Define a function with only the non-default version specified.
Definition: libavutil/internal.h:162
int ff_h263_decode_init(AVCodecContext *avctx)
Definition: libavcodec/h263dec.c:47
uint8_t ff_mpeg4_static_rl_table_store[3][2][2 *MAX_RUN+MAX_LEVEL+3]
Definition: mpeg4video.c:27
int ff_h263_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: libavcodec/h263dec.c:348
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
#define CODEC_CAP_DRAW_HORIZ_BAND
Decoder can use draw_horiz_band callback.
Definition: libavcodec/avcodec.h:737
static int mpeg4_decode_dc(MpegEncContext *s, int n, int *dir_ptr)
Decode the dc value.
Definition: mpeg4videodec.c:498
static int mpeg4_decode_block(MpegEncContext *s, int16_t *block, int n, int coded, int intra, int rvlc)
Decode a block.
Definition: mpeg4videodec.c:848
enum AVPixelFormat ff_h263_hwaccel_pixfmt_list_420[]
Definition: libavcodec/h263dec.c:776
static av_always_inline int get_vlc2(GetBitContext *s, VLC_TYPE(*table)[2], int bits, int max_depth)
Parse a vlc code.
Definition: get_bits.h:524
const int16_t ff_mpeg4_default_non_intra_matrix[64]
Definition: mpeg4data.h:348
int(* decode_mb)(struct MpegEncContext *s, int16_t block[6][64])
Definition: mpegvideo.h:702
uint8_t * mbintra_table
used to avoid setting {ac, dc, cbp}-pred stuff to zero on inter MB decoding
Definition: mpegvideo.h:361
int ff_mpeg_update_thread_context(AVCodecContext *dst, const AVCodecContext *src)
Definition: mpegvideo.c:658
static int mpeg4_decode_partitioned_mb(MpegEncContext *s, int16_t block[6][64])
decode partition C of one MB.
Definition: mpeg4videodec.c:1094
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
FIXME Range Coding of cr are mx and my are Motion Vector top and top right vectors is used as motion vector prediction the used motion vector is the sum of the predictor and(mvx_diff, mvy_diff)*mv_scale Intra DC Predicton block[y][x] dc[1]
Definition: snow.txt:392
static int get_xbits(GetBitContext *s, int n)
read mpeg1 dc style vlc (sign bit + mantisse with no MSB).
Definition: get_bits.h:212
#define FF_PROFILE_MPEG4_ADVANCED_SCALABLE_TEXTURE
Definition: libavcodec/avcodec.h:2718
MPEG4 HW decoding with VDPAU, data[0] contains a vdpau_render_state struct which contains the bitstre...
Definition: pixfmt.h:134
const int16_t ff_mpeg4_default_intra_matrix[64]
Definition: mpeg4data.h:337
int allocate_progress
Whether to allocate progress for frame threading.
Definition: libavcodec/internal.h:78
static unsigned int get_bits_long(GetBitContext *s, int n)
Read 0-32 bits.
Definition: get_bits.h:306
int ff_mpeg4_set_direct_mv(MpegEncContext *s, int mx, int my)
Definition: mpeg4video.c:121
static int mpeg4_decode_partition_a(MpegEncContext *s)
Decode first partition.
Definition: mpeg4videodec.c:543
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
int ff_mpeg4_get_video_packet_prefix_length(MpegEncContext *s)
Definition: mpeg4video.c:29
int mv[2][4][2]
motion vectors for a macroblock first coordinate : 0 = forward 1 = backward second " : depend...
Definition: mpegvideo.h:431
int b8_stride
2*mb_width+1 used for some 8x8 block arrays to allow simple addressing
Definition: mpegvideo.h:279
int ff_mpeg4_decode_picture_header(MpegEncContext *s, GetBitContext *gb)
Decode mpeg4 headers.
Definition: mpeg4videodec.c:2171
Definition: get_bits.h:54
common internal api header.
int mb_stride
mb_width+1 used for some arrays to allow simple addressing of left & top MBs without sig11 ...
Definition: mpegvideo.h:278
#define CODEC_CAP_FRAME_THREADS
Codec supports frame-level multithreading.
Definition: libavcodec/avcodec.h:810
static int mpeg4_decode_partition_b(MpegEncContext *s, int mb_count)
decode second partition.
Definition: mpeg4videodec.c:708
void ff_init_scantable(uint8_t *permutation, ScanTable *st, const uint8_t *src_scantable)
Definition: dsputil.c:110
struct AVCodecInternal * internal
Private context used for internal data.
Definition: libavcodec/avcodec.h:1178
static int mpeg4_decode_profile_level(MpegEncContext *s, GetBitContext *gb)
Definition: mpeg4videodec.c:1540
int ff_mpeg4_decode_video_packet_header(MpegEncContext *s)
Decode the next video packet.
Definition: mpeg4videodec.c:363
int ff_h263_decode_motion(MpegEncContext *s, int pred, int f_code)
Definition: ituh263dec.c:273
#define FF_PROFILE_MPEG4_SIMPLE_STUDIO
Definition: libavcodec/avcodec.h:2719
static int decode_user_data(MpegEncContext *s, GetBitContext *gb)
Decode the user data stuff in the header.
Definition: mpeg4videodec.c:1881
int workaround_bugs
workaround bugs in encoders which cannot be detected automatically
Definition: mpegvideo.h:266
ScanTable inter_scantable
if inter == intra then intra should be used to reduce tha cache usage
Definition: mpegvideo.h:295
#define FRAME_SKIPPED
return value for header parsers if frame is not coded
Definition: mpegvideo.h:48
Definition: avutil.h:143
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
#define FF_PROFILE_MPEG4_ADVANCED_CODING
Definition: libavcodec/avcodec.h:2716
static int get_amv(MpegEncContext *s, int n)
Get the average motion vector for a GMC MB.
Definition: mpeg4videodec.c:451
#define FF_PROFILE_MPEG4_CORE_SCALABLE
Definition: libavcodec/avcodec.h:2715
#define FF_PROFILE_MPEG4_SIMPLE_FACE_ANIMATION
Definition: libavcodec/avcodec.h:2711
Generated on Wed Jun 18 2025 06:53:04 for FFmpeg by
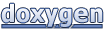