FFmpeg
|
libavcodec/h263dec.c
Go to the documentation of this file.
120 if (avctx->codec->id != AV_CODEC_ID_H263 && avctx->codec->id != AV_CODEC_ID_H263P && avctx->codec->id != AV_CODEC_ID_MPEG4)
251 ff_er_add_slice(&s->er, s->resync_mb_x, s->resync_mb_y, s->mb_x+1, s->mb_y, ER_MB_END&part_mask);
255 ff_er_add_slice(&s->er, s->resync_mb_x, s->resync_mb_y, s->mb_x, s->mb_y, ER_MB_ERROR&part_mask);
307 if(s->padding_bug_score > -2 && !s->data_partitioning /*&& (s->divx_version>=0 || !s->resync_marker)*/)
314 if(s->msmpeg4_version || (s->workaround_bugs&FF_BUG_NO_PADDING)){ //FIXME perhaps solve this more cleanly
323 if((s->workaround_bugs&FF_BUG_NO_PADDING) && (s->err_recognition&(AV_EF_BUFFER|AV_EF_AGGRESSIVE)))
329 av_log(s->avctx, AV_LOG_ERROR, "discarding %d junk bits at end, next would be %X\n", left, show_bits(&s->gb, 24));
339 av_log(s->avctx, AV_LOG_ERROR, "slice end not reached but screenspace end (%d left %06X, score= %d)\n",
411 if(s->bitstream_buffer_size && (s->divx_packed || buf_size<20)){ //divx 5.01+/xvid frame reorder
418 if ((ret = ff_MPV_common_init(s)) < 0) //we need the idct permutaton for reading a custom matrix
455 av_log(s->avctx, AV_LOG_WARNING, "Reverting picture dimensions change due to header decoding failure\n");
584 av_log(s->avctx, AV_LOG_DEBUG, "bugs: %X lavc_build:%d xvid_build:%d divx_version:%d divx_build:%d %s\n",
589 if (s->codec_id == AV_CODEC_ID_MPEG4 && s->xvid_build>=0 && avctx->idct_algo == FF_IDCT_AUTO && (av_get_cpu_flags() & AV_CPU_FLAG_MMX)) {
624 if((s->codec_id==AV_CODEC_ID_H263 || s->codec_id==AV_CODEC_ID_H263P || s->codec_id == AV_CODEC_ID_H263I))
667 if ((ret = avctx->hwaccel->start_frame(avctx, s->gb.buffer, s->gb.buffer_end - s->gb.buffer)) < 0)
673 //the second part of the wmv2 header contains the MB skip bits which are stored in current_picture->mb_type
enum AVPixelFormat(* get_format)(struct AVCodecContext *s, const enum AVPixelFormat *fmt)
callback to negotiate the pixelFormat
Definition: libavcodec/avcodec.h:1377
Definition: start.py:1
Definition: pixfmt.h:67
int vol_control_parameters
does the stream contain the low_delay flag, used to workaround buggy encoders
Definition: mpegvideo.h:594
int coded_width
Bitstream width / height, may be different from width/height e.g.
Definition: libavcodec/avcodec.h:1312
av_cold int ff_MPV_common_init(MpegEncContext *s)
init common structure for both encoder and decoder.
Definition: mpegvideo.c:993
void ff_MPV_report_decode_progress(MpegEncContext *s)
Definition: mpegvideo.c:3314
Definition: libavcodec/avcodec.h:173
int msmpeg4_version
0=not msmpeg4, 1=mp41, 2=mp42, 3=mp43/divx3 4=wmv1/7 5=wmv2/8
Definition: mpegvideo.h:639
Definition: parser.h:28
void avcodec_set_dimensions(AVCodecContext *s, int width, int height)
Definition: libavcodec/utils.c:177
HW decoding through VA API, Picture.data[3] contains a vaapi_render_state struct which contains the b...
Definition: pixfmt.h:126
Definition: libavcodec/avcodec.h:255
Definition: libavcodec/avcodec.h:115
mpegvideo header.
av_dlog(ac->avr,"%d samples - audio_convert: %s to %s (%s)\n", len, av_get_sample_fmt_name(ac->in_fmt), av_get_sample_fmt_name(ac->out_fmt), use_generic?ac->func_descr_generic:ac->func_descr)
Definition: libavcodec/avcodec.h:123
int padding_bug_score
used to detect the VERY common padding bug in MPEG4
Definition: mpegvideo.h:601
int ff_h263_decode_mb(MpegEncContext *s, int16_t block[6][64])
Definition: ituh263dec.c:605
int ff_mpeg4_decode_partitions(MpegEncContext *s)
Decode the first and second partition.
Definition: mpeg4videodec.c:795
void av_fast_malloc(void *ptr, unsigned int *size, size_t min_size)
Allocate a buffer, reusing the given one if large enough.
Definition: libavcodec/utils.c:96
void ff_mpeg_draw_horiz_band(MpegEncContext *s, int y, int h)
Definition: mpegvideo.c:2996
int ff_MPV_common_frame_size_change(MpegEncContext *s)
Definition: mpegvideo.c:1171
Definition: libavcodec/avcodec.h:117
#define CODEC_CAP_HWACCEL_VDPAU
Codec can export data for HW decoding (VDPAU).
Definition: libavcodec/avcodec.h:779
const uint8_t * ff_h263_find_resync_marker(MpegEncContext *s, const uint8_t *p, const uint8_t *end)
uint8_t * extradata
some codecs need / can use extradata like Huffman tables.
Definition: libavcodec/avcodec.h:1242
int no_rounding
apply no rounding to motion compensation (MPEG4, msmpeg4, ...) for b-frames rounding mode is always 0...
Definition: mpegvideo.h:439
int ff_intel_h263_decode_picture_header(MpegEncContext *s)
Definition: intelh263dec.c:25
#define CODEC_CAP_DR1
Codec uses get_buffer() for allocating buffers and supports custom allocators.
Definition: libavcodec/avcodec.h:743
Definition: libavcodec/avcodec.h:124
int codec_tag
internal codec_tag upper case converted from avctx codec_tag
Definition: mpegvideo.h:267
int ff_wmv2_decode_picture_header(MpegEncContext *s)
Definition: wmv2dec.c:113
enum AVChromaLocation chroma_sample_location
This defines the location of chroma samples.
Definition: libavcodec/avcodec.h:1843
static void ff_update_block_index(MpegEncContext *s)
Definition: mpegvideo.h:861
void ff_set_qscale(MpegEncContext *s, int qscale)
set qscale and update qscale dependent variables.
Definition: mpegvideo.c:3300
int has_b_frames
Size of the frame reordering buffer in the decoder.
Definition: libavcodec/avcodec.h:1415
int stream_codec_tag
internal stream_codec_tag upper case converted from avctx stream_codec_tag
Definition: mpegvideo.h:268
void ff_er_add_slice(ERContext *s, int startx, int starty, int endx, int endy, int status)
Add a slice.
Definition: error_resilience.c:772
Multithreading support functions.
#define CODEC_CAP_DELAY
Encoder or decoder requires flushing with NULL input at the end in order to give the complete and cor...
Definition: libavcodec/avcodec.h:770
int ff_combine_frame(ParseContext *pc, int next, const uint8_t **buf, int *buf_size)
Combine the (truncated) bitstream to a complete frame.
Definition: parser.c:214
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
int ff_wmv2_decode_secondary_picture_header(MpegEncContext *s)
Definition: wmv2dec.c:133
Definition: libavcodec/avcodec.h:118
external API header
int ff_flv_decode_picture_header(MpegEncContext *s)
Definition: libavcodec/flvdec.c:36
#define FF_INPUT_BUFFER_PADDING_SIZE
Required number of additionally allocated bytes at the end of the input bitstream for decoding...
Definition: libavcodec/avcodec.h:561
int ff_mpv_export_qp_table(MpegEncContext *s, AVFrame *f, Picture *p, int qp_type)
Definition: mpegvideo.c:2170
enum AVPixelFormat * pix_fmts
array of supported pixel formats, or NULL if unknown, array is terminated by -1
Definition: libavcodec/avcodec.h:2875
void ff_mpeg_er_frame_start(MpegEncContext *s)
int err_recognition
Error recognition; may misdetect some more or less valid parts as errors.
Definition: libavcodec/avcodec.h:2459
#define av_assert1(cond)
assert() equivalent, that does not lie in speed critical code.
Definition: avassert.h:53
#define CODEC_CAP_DRAW_HORIZ_BAND
Decoder can use draw_horiz_band callback.
Definition: libavcodec/avcodec.h:737
int ff_h263_get_gob_height(MpegEncContext *s)
Get the GOB height based on picture height.
Definition: h263.c:377
static int get_consumed_bytes(MpegEncContext *s, int buf_size)
Return the number of bytes consumed for building the current frame.
Definition: libavcodec/h263dec.c:140
int next_p_frame_damaged
set if the next p frame is damaged, to avoid showing trashed b frames
Definition: mpegvideo.h:531
the pkt_dts and pkt_pts fields in AVFrame will work as usual Restrictions on codec whose streams don t reset across will not work because their bitstreams cannot be decoded in parallel *The contents of buffers must not be read before as well as code calling up to before the decode process starts Call ff_thread_finish_setup() afterwards.If some code can't be moved
unsigned int allocated_bitstream_buffer_size
Definition: mpegvideo.h:612
Definition: libavcodec/avcodec.h:121
Definition: mpegvideo.h:53
Definition: libavcodec/avcodec.h:107
int ff_msmpeg4_decode_ext_header(MpegEncContext *s, int buf_size)
Definition: msmpeg4dec.c:551
int(* decode_mb)(struct MpegEncContext *s, int16_t block[6][64])
Definition: mpegvideo.h:702
#define SET_QPEL_FUNC(postfix1, postfix2)
int ff_h263_decode_frame(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: libavcodec/h263dec.c:348
int ff_MPV_frame_start(MpegEncContext *s, AVCodecContext *avctx)
generic function for encode/decode called after coding/decoding the header and before a frame is code...
Definition: mpegvideo.c:1493
av_cold int ff_h263_decode_end(AVCodecContext *avctx)
Definition: libavcodec/h263dec.c:129
Definition: libavcodec/avcodec.h:254
int(* end_frame)(AVCodecContext *avctx)
Called at the end of each frame or field picture.
Definition: libavcodec/avcodec.h:3024
Definition: libavcodec/avcodec.h:120
void ff_print_debug_info(MpegEncContext *s, Picture *p, AVFrame *pict)
Definition: mpegvideo.c:2164
void ff_MPV_decode_mb(MpegEncContext *s, int16_t block[12][64])
Definition: mpegvideo.c:2907
HW acceleration through VDPAU, Picture.data[3] contains a VdpVideoSurface.
Definition: pixfmt.h:203
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
AVHWAccel * ff_find_hwaccel(enum AVCodecID codec_id, enum AVPixelFormat pix_fmt)
Return the hardware accelerated codec for codec codec_id and pixel format pix_fmt.
Definition: libavcodec/utils.c:2939
Definition: libavcodec/avcodec.h:270
int ff_h263_decode_picture_header(MpegEncContext *s)
Definition: ituh263dec.c:871
int av_get_cpu_flags(void)
Return the flags which specify extensions supported by the CPU.
Definition: cpu.c:30
Definition: libavcodec/avcodec.h:122
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample they are references to shared objects When the negotiation mechanism computes the intersection of the formats supported at each end of a all references to both lists are replaced with a reference to the intersection And when a single format is eventually chosen for a link amongst the remaining all references to the list are updated That means that if a filter requires that its input and output have the same format amongst a supported all it has to do is use a reference to the same list of formats query_formats can leave some formats unset and return AVERROR(EAGAIN) to cause the negotiation mechanism toagain later.That can be used by filters with complex requirements to use the format negotiated on one link to set the formats supported on another.Buffer references ownership and permissions
int pict_type
AV_PICTURE_TYPE_I, AV_PICTURE_TYPE_P, AV_PICTURE_TYPE_B, ...
Definition: mpegvideo.h:377
av_cold int ff_h263_decode_init(AVCodecContext *avctx)
Definition: libavcodec/h263dec.c:47
int ff_h263_resync(MpegEncContext *s)
Decode the group of blocks / video packet header.
Definition: ituh263dec.c:231
int av_frame_ref(AVFrame *dst, AVFrame *src)
Setup a new reference to the data described by an given frame.
Definition: frame.c:228
int(* decode_slice)(AVCodecContext *avctx, const uint8_t *buf, uint32_t buf_size)
Callback for each slice.
Definition: libavcodec/avcodec.h:3013
int(* start_frame)(AVCodecContext *avctx, const uint8_t *buf, uint32_t buf_size)
Called at the beginning of each frame or field picture.
Definition: libavcodec/avcodec.h:3000
Definition: libavcodec/avcodec.h:174
planar YUV 4:2:0, 12bpp, (1 Cr & Cb sample per 2x2 Y samples)
Definition: pixfmt.h:68
Definition: get_bits.h:54
common internal api header.
int mb_stride
mb_width+1 used for some arrays to allow simple addressing of left & top MBs without sig11 ...
Definition: mpegvideo.h:278
enum AVPixelFormat ff_h263_hwaccel_pixfmt_list_420[]
Definition: libavcodec/h263dec.c:776
int workaround_bugs
Work around bugs in encoders which sometimes cannot be detected automatically.
Definition: libavcodec/avcodec.h:2374
Definition: libavcodec/avcodec.h:119
void ff_vdpau_mpeg4_decode_picture(MpegEncContext *s, const uint8_t *buf, int buf_size)
int workaround_bugs
workaround bugs in encoders which cannot be detected automatically
Definition: mpegvideo.h:266
#define FRAME_SKIPPED
return value for header parsers if frame is not coded
Definition: mpegvideo.h:48
Definition: avutil.h:143
qpel_mc_func put_no_rnd_qpel_pixels_tab[2][16]
Definition: dsputil.h:191
static int decode(AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt)
Definition: crystalhd.c:868
int ff_mpeg4_decode_picture_header(MpegEncContext *s, GetBitContext *gb)
Decode mpeg4 headers.
Definition: mpeg4videodec.c:2171
int ff_msmpeg4_decode_picture_header(MpegEncContext *s)
Definition: msmpeg4dec.c:396
int ff_mpeg4_find_frame_end(ParseContext *pc, const uint8_t *buf, int buf_size)
Find the end of the current frame in the bitstream.
Definition: mpeg4video_parser.c:36
int ff_find_unused_picture(MpegEncContext *s, int shared)
Definition: mpegvideo.c:1453
int ff_h263_find_frame_end(ParseContext *pc, const uint8_t *buf, int buf_size)
Definition: h263_parser.c:30
void ff_MPV_decode_defaults(MpegEncContext *s)
Set the given MpegEncContext to defaults for decoding.
Definition: mpegvideo.c:826
Generated on Wed Jun 18 2025 06:53:01 for FFmpeg by
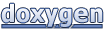