FFmpeg
|
#include "libavutil/opt.h"
#include "error_resilience.h"
#include "internal.h"
#include "mpegvideo.h"
#include "mpeg4video.h"
#include "h263.h"
#include "thread.h"
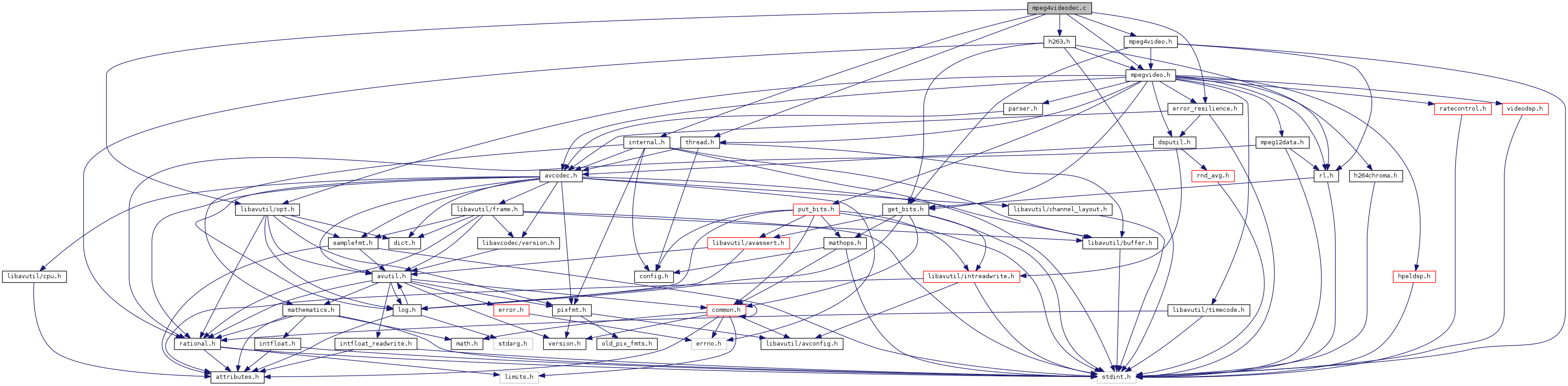
Go to the source code of this file.
Macros | |
#define | UNCHECKED_BITSTREAM_READER 1 |
#define | SPRITE_TRAJ_VLC_BITS 6 |
#define | DC_VLC_BITS 9 |
#define | MB_TYPE_B_VLC_BITS 4 |
Variables | |
static VLC | dc_lum |
static VLC | dc_chrom |
static VLC | sprite_trajectory |
static VLC | mb_type_b_vlc |
static const int | mb_type_b_map [4] |
static const AVProfile | mpeg4_video_profiles [] |
static const AVOption | mpeg4_options [] |
static const AVClass | mpeg4_class |
static const AVClass | mpeg4_vdpau_class |
AVCodec | ff_mpeg4_decoder |
Macro Definition Documentation
#define DC_VLC_BITS 9 |
Definition at line 38 of file mpeg4videodec.c.
Referenced by ff_mpeg4videodec_static_init(), and mpeg4_decode_dc().
#define MB_TYPE_B_VLC_BITS 4 |
Definition at line 39 of file mpeg4videodec.c.
Referenced by ff_mpeg4videodec_static_init(), and mpeg4_decode_mb().
#define SPRITE_TRAJ_VLC_BITS 6 |
Definition at line 37 of file mpeg4videodec.c.
Referenced by ff_mpeg4videodec_static_init(), and mpeg4_decode_sprite_trajectory().
#define UNCHECKED_BITSTREAM_READER 1 |
Definition at line 23 of file mpeg4videodec.c.
Function Documentation
|
static |
Definition at line 2286 of file mpeg4videodec.c.
|
static |
Decode the user data stuff in the header.
Also initializes divx/xvid/lavc_version/build.
Definition at line 1881 of file mpeg4videodec.c.
Referenced by ff_mpeg4_decode_picture_header().
|
static |
Definition at line 1553 of file mpeg4videodec.c.
Referenced by ff_mpeg4_decode_picture_header().
|
static |
Definition at line 1935 of file mpeg4videodec.c.
Referenced by ff_mpeg4_decode_picture_header().
int ff_mpeg4_decode_partitions | ( | MpegEncContext * | s | ) |
Decode the first and second partition.
- Returns
- <0 if error (and sets error type in the error_status_table)
Definition at line 795 of file mpeg4videodec.c.
Referenced by decode_slice().
int ff_mpeg4_decode_picture_header | ( | MpegEncContext * | s, |
GetBitContext * | gb | ||
) |
Decode mpeg4 headers.
- Returns
- <0 if no VOP found (or a damaged one) FRAME_SKIPPED if a not coded VOP is found 0 if a VOP is found
Definition at line 2171 of file mpeg4videodec.c.
Referenced by av_mpeg4_decode_header(), and ff_h263_decode_frame().
int ff_mpeg4_decode_video_packet_header | ( | MpegEncContext * | s | ) |
Decode the next video packet.
- Returns
- <0 if something went wrong
Definition at line 363 of file mpeg4videodec.c.
Referenced by ff_h263_resync().
void ff_mpeg4_pred_ac | ( | MpegEncContext * | s, |
int16_t * | block, | ||
int | n, | ||
int | dir | ||
) |
Predict the ac.
- Parameters
-
n block index (0-3 are luma, 4-5 are chroma) dir the ac prediction direction
Definition at line 58 of file mpeg4videodec.c.
Referenced by ff_msmpeg4_decode_block(), and mpeg4_decode_block().
Definition at line 2260 of file mpeg4videodec.c.
Referenced by decode_init(), and mpeg4video_parse_init().
|
inlinestatic |
Get the average motion vector for a GMC MB.
- Parameters
-
n either 0 for the x component or 1 for y
- Returns
- the average MV for a GMC MB
Definition at line 451 of file mpeg4videodec.c.
Referenced by mpeg4_decode_mb(), and mpeg4_decode_partition_a().
|
inlinestatic |
Decode a block.
- Returns
- <0 if an error occurred
Definition at line 848 of file mpeg4videodec.c.
Referenced by mpeg4_decode_mb(), and mpeg4_decode_partitioned_mb().
|
inlinestatic |
Decode the dc value.
- Parameters
-
n block index (0-3 are luma, 4-5 are chroma) dir_ptr the prediction direction will be stored here
- Returns
- the quantized dc
Definition at line 498 of file mpeg4videodec.c.
Referenced by mpeg4_decode_block(), mpeg4_decode_partition_a(), and mpeg4_decode_partition_b().
|
static |
Definition at line 1519 of file mpeg4videodec.c.
Referenced by ff_mpeg4_decode_picture_header().
|
static |
Definition at line 1176 of file mpeg4videodec.c.
Referenced by decode_init(), and decode_vop_header().
|
static |
Decode first partition.
- Returns
- number of MBs decoded or <0 if an error occurred
Definition at line 543 of file mpeg4videodec.c.
Referenced by ff_mpeg4_decode_partitions().
|
static |
decode second partition.
- Returns
- <0 if an error occurred
Definition at line 708 of file mpeg4videodec.c.
Referenced by ff_mpeg4_decode_partitions().
|
static |
decode partition C of one MB.
- Returns
- <0 if an error occurred
Definition at line 1094 of file mpeg4videodec.c.
Referenced by decode_vop_header().
|
static |
Definition at line 1540 of file mpeg4videodec.c.
Referenced by ff_mpeg4_decode_picture_header().
|
static |
Definition at line 165 of file mpeg4videodec.c.
Referenced by decode_vop_header(), and ff_mpeg4_decode_video_packet_header().
|
inlinestatic |
check if the next stuff is a resync marker or the end.
- Returns
- 0 if not
Definition at line 117 of file mpeg4videodec.c.
Referenced by mpeg4_decode_mb(), and mpeg4_decode_partitioned_mb().
Variable Documentation
|
static |
Definition at line 42 of file mpeg4videodec.c.
|
static |
Definition at line 42 of file mpeg4videodec.c.
AVCodec ff_mpeg4_decoder |
Definition at line 2352 of file mpeg4videodec.c.
|
static |
|
static |
Definition at line 44 of file mpeg4videodec.c.
|
static |
Definition at line 2338 of file mpeg4videodec.c.
Referenced by decode().
|
static |
Definition at line 2332 of file mpeg4videodec.c.
|
static |
Definition at line 2345 of file mpeg4videodec.c.
|
static |
Definition at line 2312 of file mpeg4videodec.c.
|
static |
Definition at line 43 of file mpeg4videodec.c.
Generated on Thu May 8 2025 06:52:58 for FFmpeg by
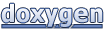