FFmpeg
|
#include "libavutil/attributes.h"
#include "libavcodec/avcodec.h"
#include "libavcodec/dsputil.h"
#include "libavcodec/bit_depth_template.c"
#include "libavcodec/hpeldsp.h"
#include "libavcodec/rnd_avg.h"
#include "dsputil_sh4.h"
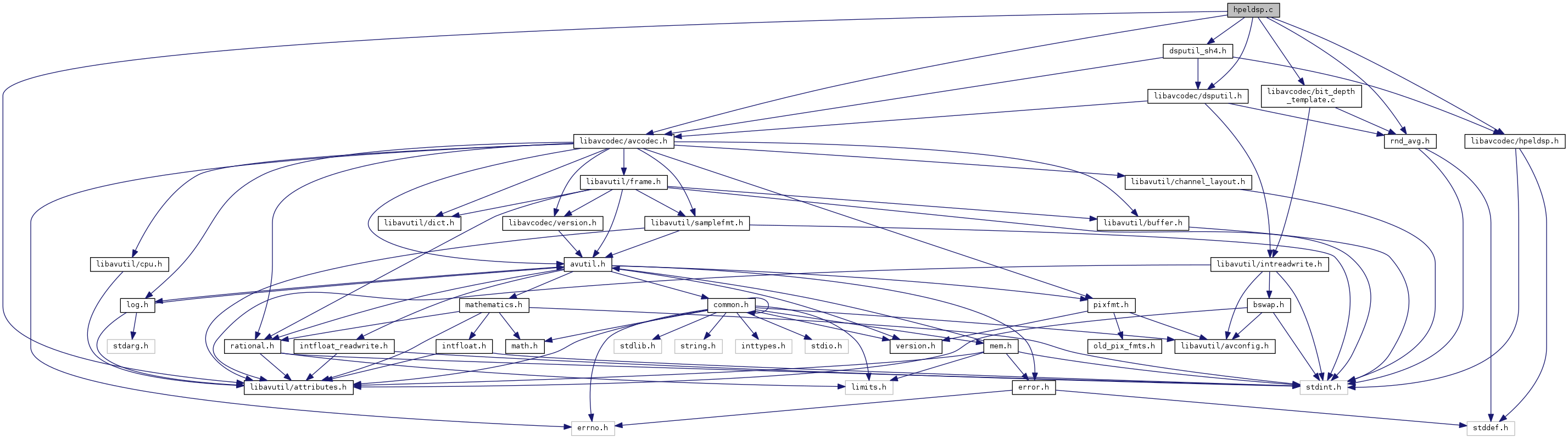
Go to the source code of this file.
Macros | |
#define | LP(p) *(uint32_t*)(p) |
#define | LPC(p) *(const uint32_t*)(p) |
#define | UNPACK(ph, pl, tt0, tt1) |
#define | rnd_PACK(ph, pl, nph, npl) ph + nph + (((pl + npl + BYTE_VEC32(0x02))>>2) & BYTE_VEC32(0x03)) |
#define | no_rnd_PACK(ph, pl, nph, npl) ph + nph + (((pl + npl + BYTE_VEC32(0x01))>>2) & BYTE_VEC32(0x03)) |
#define | MERGE1(a, b, ofs) (ofs==0)?a:( ((a)>>(8*ofs))|((b)<<(32-8*ofs)) ) |
#define | MERGE2(a, b, ofs) (ofs==3)?b:( ((a)>>(8*(ofs+1)))|((b)<<(32-8*(ofs+1))) ) |
#define | put(d, s) d = s |
#define | avg(d, s) d = rnd_avg32(s,d) |
#define | OP_C4(ofs) |
#define | OP_C40() |
#define | OP put |
#define | OP avg |
#define | OP_C(ofs, sz, avg2) |
#define | OP_C0(sz, avg2) |
#define | OP_X(ofs, sz, avg2) |
#define | OP_Y0(sz, avg2) |
#define | OP_Y(ofs, sz, avg2) |
#define | OP_X0(sz, avg2) OP_X(0,sz,avg2) |
#define | OP_XY0(sz, PACK) OP_XY(0,sz,PACK) |
#define | OP_XY(ofs, sz, PACK) |
#define | DEFFUNC(prefix, op, rnd, xy, sz, OP_N, avgfunc) |
#define | OP put |
#define | OP avg |
#define | ff_put_no_rnd_pixels8_o ff_put_rnd_pixels8_o |
#define | ff_put_no_rnd_pixels16_o ff_put_rnd_pixels16_o |
#define | ff_avg_no_rnd_pixels16_o ff_avg_rnd_pixels16_o |
Functions | |
static void | put_pixels4_c (uint8_t *dest, const uint8_t *ref, const int stride, int height) |
static void | avg_pixels4_c (uint8_t *dest, const uint8_t *ref, const int stride, int height) |
av_cold void | ff_hpeldsp_init_sh4 (HpelDSPContext *c, int flags) |
Macro Definition Documentation
Definition at line 268 of file sh4/hpeldsp.c.
#define ff_avg_no_rnd_pixels16_o ff_avg_rnd_pixels16_o |
Definition at line 316 of file sh4/hpeldsp.c.
Referenced by ff_hpeldsp_init_sh4().
#define ff_put_no_rnd_pixels16_o ff_put_rnd_pixels16_o |
Definition at line 315 of file sh4/hpeldsp.c.
Referenced by ff_hpeldsp_init_sh4().
#define ff_put_no_rnd_pixels8_o ff_put_rnd_pixels8_o |
Definition at line 314 of file sh4/hpeldsp.c.
Referenced by ff_hpeldsp_init_sh4().
#define LP | ( | p | ) | *(uint32_t*)(p) |
Definition at line 32 of file sh4/hpeldsp.c.
#define LPC | ( | p | ) | *(const uint32_t*)(p) |
Definition at line 33 of file sh4/hpeldsp.c.
Definition at line 45 of file sh4/hpeldsp.c.
Definition at line 46 of file sh4/hpeldsp.c.
#define no_rnd_PACK | ( | ph, | |
pl, | |||
nph, | |||
npl | |||
) | ph + nph + (((pl + npl + BYTE_VEC32(0x01))>>2) & BYTE_VEC32(0x03)) |
Definition at line 42 of file sh4/hpeldsp.c.
#define OP put |
Definition at line 298 of file sh4/hpeldsp.c.
#define OP avg |
Definition at line 298 of file sh4/hpeldsp.c.
#define OP put |
Definition at line 298 of file sh4/hpeldsp.c.
#define OP avg |
Definition at line 298 of file sh4/hpeldsp.c.
#define OP_C | ( | ofs, | |
sz, | |||
avg2 | |||
) |
#define OP_C0 | ( | sz, | |
avg2 | |||
) |
Definition at line 123 of file sh4/hpeldsp.c.
#define OP_C4 | ( | ofs | ) |
Definition at line 56 of file sh4/hpeldsp.c.
Referenced by avg_pixels4_c(), and put_pixels4_c().
#define OP_C40 | ( | ) |
Definition at line 64 of file sh4/hpeldsp.c.
Referenced by avg_pixels4_c(), and put_pixels4_c().
#define OP_X | ( | ofs, | |
sz, | |||
avg2 | |||
) |
Definition at line 224 of file sh4/hpeldsp.c.
#define OP_XY | ( | ofs, | |
sz, | |||
PACK | |||
) |
Definition at line 226 of file sh4/hpeldsp.c.
#define OP_XY0 | ( | sz, | |
PACK | |||
) | OP_XY(0,sz,PACK) |
Definition at line 225 of file sh4/hpeldsp.c.
#define OP_Y | ( | ofs, | |
sz, | |||
avg2 | |||
) |
Definition at line 186 of file sh4/hpeldsp.c.
#define OP_Y0 | ( | sz, | |
avg2 | |||
) |
Definition at line 159 of file sh4/hpeldsp.c.
#define rnd_PACK | ( | ph, | |
pl, | |||
nph, | |||
npl | |||
) | ph + nph + (((pl + npl + BYTE_VEC32(0x02))>>2) & BYTE_VEC32(0x03)) |
Definition at line 41 of file sh4/hpeldsp.c.
#define UNPACK | ( | ph, | |
pl, | |||
tt0, | |||
tt1 | |||
) |
Definition at line 36 of file sh4/hpeldsp.c.
Function Documentation
|
static |
Definition at line 88 of file sh4/hpeldsp.c.
av_cold void ff_hpeldsp_init_sh4 | ( | HpelDSPContext * | c, |
int | flags | ||
) |
Definition at line 318 of file sh4/hpeldsp.c.
Referenced by ff_hpeldsp_init().
|
static |
Definition at line 74 of file sh4/hpeldsp.c.
Generated on Sat Feb 22 2025 06:54:47 for FFmpeg by
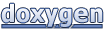